Exploring Composite Types in Go: Arrays and Slices
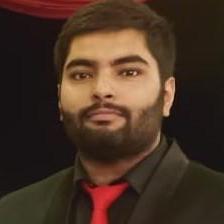
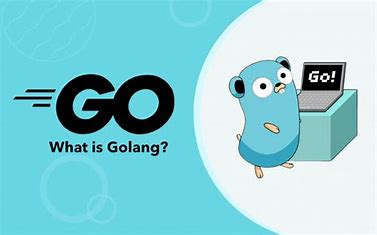
In my journey of learning Go, I've recently delved into composite types, specifically arrays and slices. These structures allow for more complex data management and manipulation, and understanding them is crucial for efficient programming in Go. Here’s what I learned.
Arrays
An array in Go is a fixed-size collection of elements of the same type. Here’s how they work:
Declaring an Array: You specify the size of the array and the type of elements it will hold. For example.
var myArray [3]int
In this case,
myArray
can hold three integers, and by default, all elements are initialized to zero.
Initializing an Array: You can also declare and initialize an array simultaneously.
This creates an array with specified values.var myInitializedArray = [3]int{10, 30, 54}
This creates an array with specified values.
Sparse Arrays: Go allows you to create sparse arrays, where most elements are zero. Here’s an example.
var sparseArray = [12]int{1, 5: 4, 6, 10: 100, 15}
This indicates that
sparseArray[0] = 1
,sparseArray[5] = 4
,sparseArray[6] = 0
,sparseArray[10] = 100
, andsparseArray[11] = 15
Array Literals: You can use the
...
syntax to let Go determine the size of the arrayvar arrayLiteral = [...]int{10, 20, 30}
Length of an Array: Go provides a built-in function to get the length of an array
fmt.Println(len(myInitializedArray))
Slices
Slices are a more flexible alternative to arrays in Go. They are dynamically-sized and more versatile.
Declaring a Slice: You can declare a slice without specifying a size.
var mySlice = []int{10, 20, 30}
Appending to a Slice: You can easily add elements to a slice.
var mySlice []int mySlice = append(mySlice, 10) mySlice = append(mySlice, 20)
Capacity: Slices have a capacity that can grow as you append elements. Here’s an example.
fmt.Println(mySlice, len(mySlice), cap(mySlice)) mySlice = append(mySlice, 30) fmt.Println(mySlice, len(mySlice), cap(mySlice))
Creating Slices with
make
: Themake
function allows you to specify the length and capacity.mySlice = make([]int, 5) // Length and capacity of 5
Slicing Slices: You can create new slices from existing slices using slicing syntax.
originalSlice := []string{"a", "b", "c", "d"} newSlice := originalSlice[1:3] // This will contain "b" and "c"
Overlapping Slices: Slices can overlap in memory, which can lead to unexpected behavior. Modifying one slice can affect another if they share the same underlying array.
x := []string{"a", "b", "c", "d"} y := x[:2] fmt.Println(cap(x), cap(y)) y = append(y, "z") fmt.Println("x:", x) fmt.Println("y:", y) fmt.Println()
Copying Slices: You can copy the contents of one slice to another using the
copy
function.sourceSlice := []int{1, 2, 3, 4} destinationSlice := make([]int, 4) copy(destinationSlice, sourceSlice)
Converting Arrays to Slices: You can easily convert an array to a slice.
array := [4]int{1, 2, 3, 4} slice := array[:]
Next Up
In my next phase of learning Go, I will focus on maps and structs. Maps are essential for storing key-value pairs, enabling efficient data retrieval, while structs allow me to create complex data types by grouping related variables. Understanding these composite types will deepen my knowledge of data structures in Go and enhance my ability to build robust applications.
Subscribe to my newsletter
Read articles from Sahal Imran directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
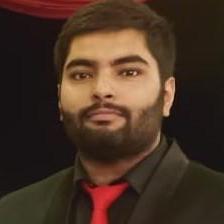