Swapping the value of a and b without using a temporary variable.
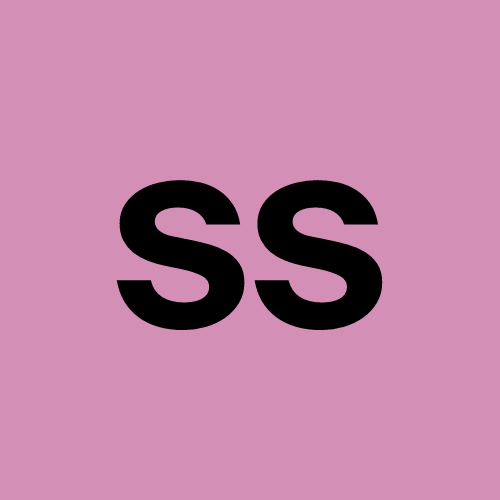
The most obvious way to perform a swap is to use a temporary C variable; however, there are also some unique methods to swap values without using any extra memory. In this article, we will see two different and efficient ways to swap without a temporary variable.
1)Starting with XOR
XOR (Exclusive OR) is a bitwise operator that operates on the binary forms of numbers. The XOR operator compares each corresponding bit of two numbers and returns 1
if the bits are different and 0
if they are the same.
XOR Table:
A | B | A ^ B |
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 0 |
Some Useful Properties:
A ^ A = 0
A ^ 0 = A
A ^ B ^ B = A
Code to Swap Variables Using XOR
#include <stdio.h>
int main() {
int a, b;
printf("Enter two numbers:\n");
scanf("%d %d", &a, &b);
printf("Before swapping: a = %d, b = %d\n", a, b);
// Swap using XOR
a = a ^ b; // Step 1: a holds the XOR of a and b
b = a ^ b; // Step 2: b now holds the original value of a
a = a ^ b; // Step 3: a now holds the original value of b
// Display swapped values
printf("After swapping: a = %d, b = %d\n", a, b);
return 0;
}
Explanation of XOR Logic:
Let’s assume:
a = 2
(binary:0010
)b = 3
(binary:0011
)Step 1:
a = a ^ b
a = 2 ^ 3 = 0010 ^ 0011 = 0001 (a now holds 1)
Step 2: b = a ^ b
b = 1 ^ 3 = 0001 ^ 0011 = 0010 (b now holds 2, the original value of a)
Step 3: a = a ^ b
a = 1 ^ 2 = 0001 ^ 0010 = 0101 (a now holds 3, the original value of b)
Output:
Enter two integers:
2 3
Before swapping: a = 2, b = 3
After swapping: a = 3, b = 2
Second Method: Swapping Using Arithmetic Operations.
Arithmetic operations can also be used to swap two variables without the need for a temporary variable.
#include <stdio.h>
int main() {
int a, b;
printf("Enter two integers:\n");
scanf("%d %d", &a, &b);
// Swap using arithmetic operations
a = a + b; // Step 1: a holds the sum of a and b
b = a - b; // Step 2: b now holds the original value of a
a = a - b; // Step 3: a now holds the original value of b
// Display swapped values
printf("After swapping: a = %d, b = %d\n", a, b);
return 0;
}
Output:
Enter two integers:
2 3
Before swapping: a = 2, b = 3
After swapping: a = 3, b = 3
Conclusion:
XOR Swap Method:
Pros:
No overflow issues (works with any integer size).
Good use of bitwise operations.
Cons:
lil bit confusing to beginners.
Doesn't work if the variables refer to the same memory location (e.g.,
a = b
).
Arithmetic Swap Method:
Pros:
- Simple and easy to understand.
Cons:
- Might cause an overflow if the sum of
a
andb
exceeds the range of the integer type.
- Might cause an overflow if the sum of
Subscribe to my newsletter
Read articles from Sonal Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
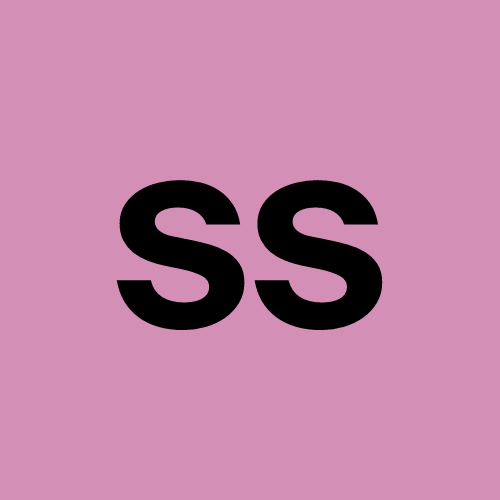