Explore More RobotJS Examples to Supercharge Your Task Automation!

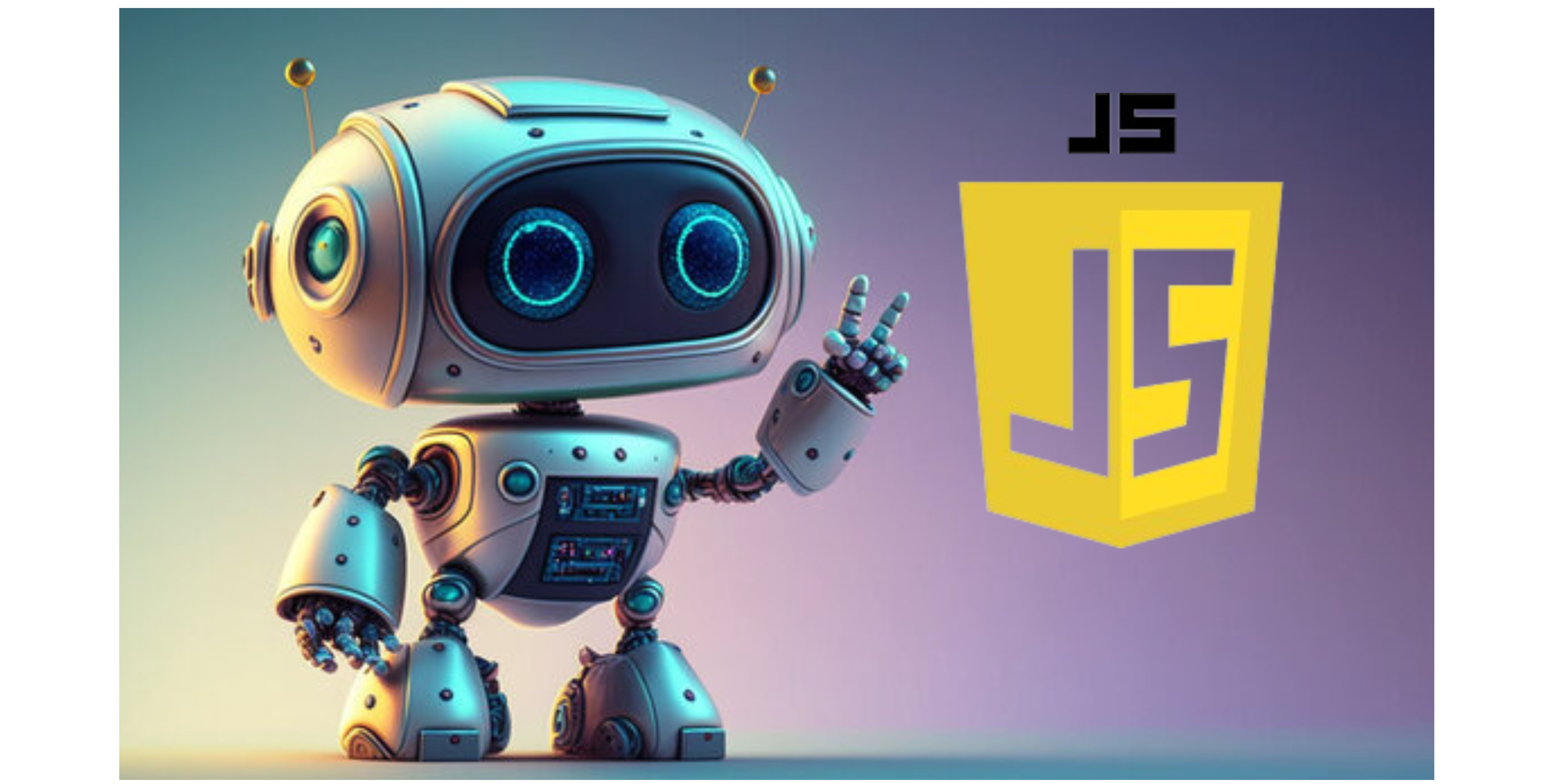
Here are more RobotJS examples to help you explore the different ways you can automate tasks using this powerful library :
1. Mouse Movement and Clicks
Example 1: Move Mouse to Specific Coordinates
You can move the mouse to a specific position on the screen using its coordinates.
const robot = require('robotjs');
// Move the mouse to (100, 100) on the screen
robot.moveMouse(100, 100);
Example 2: Smooth Mouse Movement
RobotJS also supports smooth mouse movements, simulating how a human might move the mouse.
robot.moveMouseSmooth(500, 500);
Example 3: Perform a Right-Click
You can simulate a right-click using RobotJS.
// Move to position (300, 300) and perform a right-click
robot.moveMouse(300, 300);
robot.mouseClick('right');
2. Keyboard Automation
Example 1: Type a String
RobotJS allows you to simulate typing on the keyboard, making it ideal for automating text input tasks.
// Type out a string like a user would
robot.typeString('Hello, this is RobotJS!');
Example 2: Press a Key Combination (e.g., Ctrl + C)
You can simulate pressing key combinations, such as copying text.
// Simulate pressing Ctrl + C (copy)
robot.keyTap('c', ['control']);
Example 3: Simulate Holding and Releasing Keys
If you want to simulate holding down a key, RobotJS allows you to do that as well.
// Hold down the "control" key, press "a" and then release "control"
robot.keyToggle('control', 'down');
robot.keyTap('a'); // Select all (Ctrl + A)
robot.keyToggle('control', 'up');
3. Pixel Color Detection
Example 1: Get the Color of a Pixel
You can get the color of a specific pixel on the screen, which can be useful for image recognition tasks.
// Get the mouse position and detect the color of the pixel at that location
const mouse = robot.getMousePos();
const color = robot.getPixelColor(mouse.x, mouse.y);
console.log(`The color at (${mouse.x}, ${mouse.y}) is #${color}`);
Example 2: Screenshot a Region and Detect Color
RobotJS can take a screenshot of a portion of the screen and check the pixel colors.
// Take a screenshot of a region (100x100 pixels) starting from (0, 0)
const img = robot.screen.capture(0, 0, 100, 100);
// Get the color of a pixel in the screenshot
const color = img.colorAt(50, 50); // Get the color at position (50, 50) in the image
console.log(`The color at (50, 50) in the screenshot is #${color}`);
4. Screenshots
Example 1: Capture a Screenshot of the Entire Screen
RobotJS can take full-screen or partial screenshots.
const screenSize = robot.getScreenSize();
const screenshot = robot.screen.capture(0, 0, screenSize.width, screenSize.height);
// Save screenshot or process the data
console.log(`Screenshot size: ${screenshot.width}x${screenshot.height}`);
5. Delays Between Actions
Example 1: Add Delays Between Actions
You can slow down your automation by adding delays between actions to simulate human-like interaction.
// Move the mouse and add a delay before clicking
robot.moveMouse(400, 400);
robot.setMouseDelay(200); // Add a delay of 200 milliseconds
robot.mouseClick();
Example 2: Delay Between Typing Characters
To simulate a real typing experience, you can add a delay between each keystroke.
robot.setKeyboardDelay(500); // Delay of 500 milliseconds between key presses
robot.typeString('Typing with delays...');
6. Screen Size and Mouse Position
Example 1: Get Screen Size
If you want your automation to adjust based on the screen size, RobotJS can provide the current screen dimensions.
const screenSize = robot.getScreenSize();
console.log(`Screen size: ${screenSize.width}x${screenSize.height}`);
Example 2: Get Current Mouse Position
You can track the current position of the mouse and use it for further automation.
const mousePos = robot.getMousePos();
console.log(`Mouse is at: (${mousePos.x}, ${mousePos.y})`);
7. Scroll Automation
Example 1: Scroll the Mouse Wheel
RobotJS can also scroll the mouse wheel up or down, simulating how users scroll through web pages or applications.
// Scroll down by 10 units
robot.scrollMouse(0, 10);
// Scroll up by 5 units
robot.scrollMouse(0, -5);
8. Working with Coordinates
Example 1: Move Mouse Relative to Its Current Position
You can move the mouse relative to its current position rather than absolute screen coordinates.
// Get the current mouse position
const { x, y } = robot.getMousePos();
// Move the mouse 100 pixels right and 50 pixels down from its current position
robot.moveMouse(x + 100, y + 50);
Conclusion
These examples should give you a solid understanding of what you can do with RobotJS. Whether you need to automate repetitive tasks, simulate user input, or create bots, RobotJS provides a simple yet powerful API to control your desktop environment with JavaScript. Explore these examples and combine them to create more complex automation workflows!
Subscribe to my newsletter
Read articles from Payal Porwal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Payal Porwal
Payal Porwal
Hi there, tech enthusiasts! I'm a passionate Software Developer driven by a love for continuous learning and innovation. I thrive on exploring new tools and technologies, pushing boundaries, and finding creative solutions to complex problems. What You'll Find Here On my Hashnode blog, I share: 🚀 In-depth explorations of emerging technologies 💡 Practical tutorials and how-to guides 🔧Insights on software development best practices 🚀Reviews of the latest tools and frameworks 💡 Personal experiences from real-world projects. Join me as we bridge imagination and implementation in the tech world. Whether you're a seasoned pro or just starting out, there's always something new to discover! Let’s connect and grow together! 🌟