8 Essential Code Snippets to Master the WordPress Block Editor

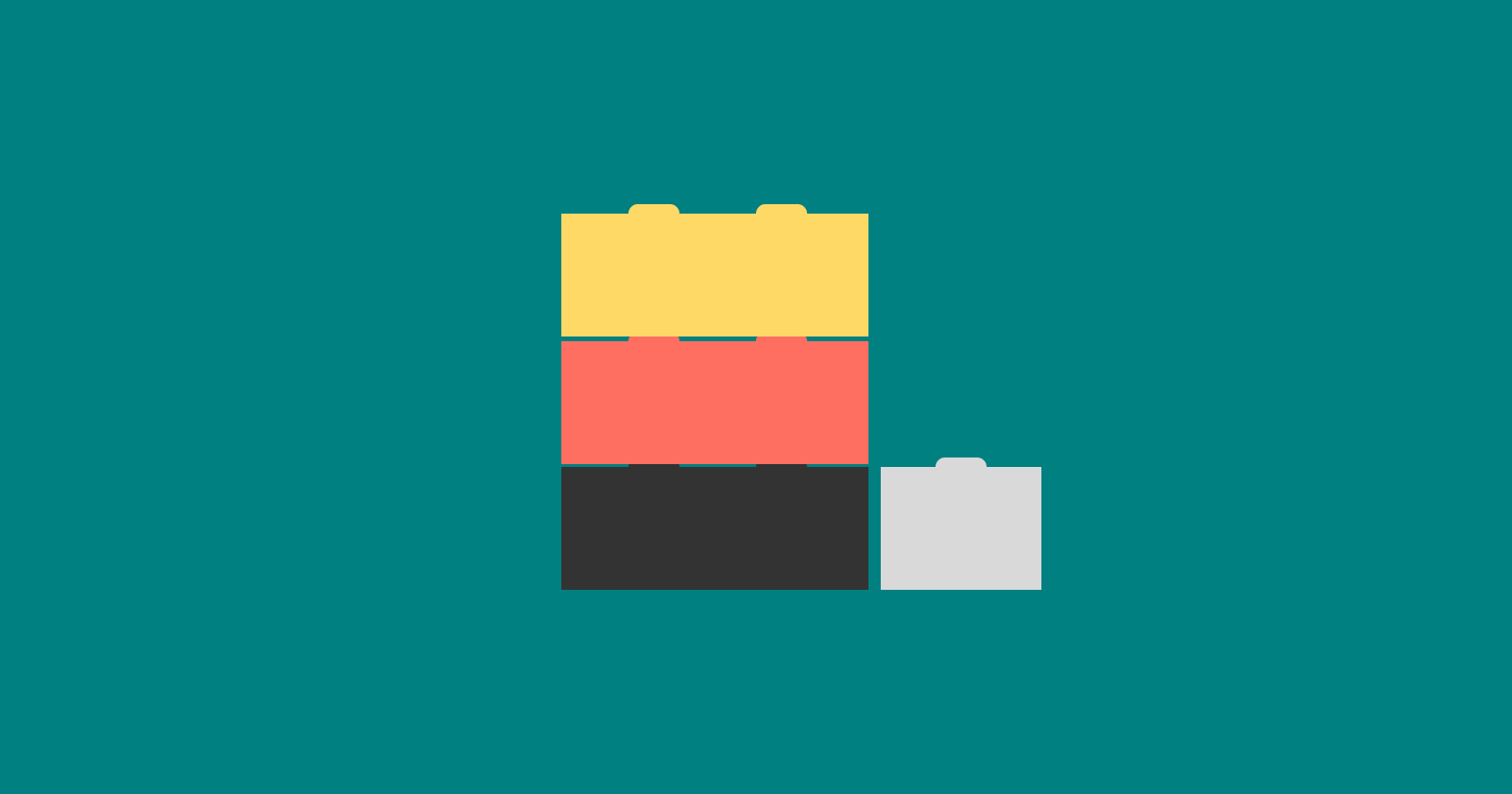
Ah, the Block Editor (formerly Gutenberg) — a source of joy for some and mild frustration for others. Whether you're still getting the hang of it or looking to make it work the way you want, these code snippets will help you navigate some common issues.
(I’ve gathered these trusty code snippets from some of my older blog posts. Some might be a bit old, but they might still come in handy.)
1. Busting Cache in getEntityRecords
When working with dynamic blocks that rely on fetching posts or data, you may encounter issues where outdated data is cached, making your content appear stale. This can be particularly problematic when you’re fetching frequently updated content. The getEntityRecords
cache in the Block Editor can sometimes keep old data around longer than you’d like.
This snippet shows you how to bust the cache for getEntityRecords
, ensuring that the content on your site is always up-to-date, especially after creating or editing posts.
wp.data.dispatch('core').receiveEntityRecords('postType', 'post', [], {}, true);
2. Hooking into the Save Action
In some situations, you may want to trigger additional actions whenever a post is saved, such as updating external services, sending notifications, or performing some custom processing. Unfortunately, the Block Editor doesn’t provide a simple hook to intercept the save action by default.
This snippet listens for the post-saving process and allows you to hook into that event, enabling you to run custom code every time a post is saved, ensuring you can automate processes as needed without manual intervention.
import { select, subscribe } from '@wordpress/data';
subscribe(() => {
const isSavingPost = select('core/editor').isSavingPost();
const isAutosavingPost = select('core/editor').isAutosavingPost();
if (isAutosavingPost && !isSavingPost) {
return;
}
// Your custom action here
});
3. Creating Custom Block Categories
With a growing number of blocks in the Block Editor, it can become increasingly difficult to locate specific blocks, especially custom ones you’ve developed. By default, all blocks are placed into general categories, making it harder to organise them.
This snippet demonstrates how to create a custom block category, allowing you to organise your blocks better. It not only improves the workflow for developers but also helps users find and use your blocks more efficiently.
function matt_watson_block_categories($categories) {
return array_merge($categories, [
[
'slug' => 'matt-watson-blocks',
'title' => __('Matt Watson Blocks', 'mattwatson'),
],
]);
}
add_filter('block_categories_all', 'matt_watson_block_categories', 10, 2);
4. Fetching Posts with getEntityRecord
Fetching individual posts by their ID in the Block Editor can be tricky for developers accustomed to using the get_post()
function in classic WordPress. The Block Editor uses getEntityRecord()
instead, which offers a more modern approach for fetching data.
This snippet shows how to use getEntityRecord()
to retrieve a specific post by its ID in the Block Editor, making the transition from classic WordPress development smoother.
const post = wp.data.select('core').getEntityRecord('postType', 'post', 42);
5. Querying Posts with getEntityRecords
If you’ve ever missed the flexibility of WP_Query
when working in the Block Editor, getEntityRecords()
can provide a similar experience. This function allows you to query posts and custom post types using parameters such as post status or pagination.
This snippet demonstrates how to retrieve posts based on a specific status and limit the number of posts returned, offering an efficient way to handle content querying in the Block Editor.
const posts = wp.data.select('core').getEntityRecords('postType', 'post', {
status: 'draft',
per_page: 2,
});
6. Updating Post Meta with editPost
In the past, updating post meta in WordPress typically involved using PHP’s update_post_meta()
function. With the Block Editor, you can now update post meta directly using the editPost
function from JavaScript.
This snippet shows how to update the metadata of a post within the Block Editor interface, keeping your workflow modern and efficient without needing to switch back to server-side PHP.
wp.data.dispatch('core/editor').editPost({
meta: {
matt_watson_meta_key_example: 'Example Value',
},
});
7. Find Posts with Specific Blocks in WordPress
If you need to identify posts containing a specific block, this snippet can help. It runs a WP_Query
to search for posts that include a block by its namespace and block name. This can be useful when you want to apply changes or track down posts that contain a certain block across your site.
$posts = new WP_Query([
'post_type' => 'any',
'post_status' => 'any',
's' => 'wp:some-block-namespace/block-name',
]);
8. Add Font Family Support to the Core Heading Block
Extending core blocks can improve design flexibility without needing to create new custom blocks. By using the block_type_metadata_settings
filter, this snippet enables font family support for the core Heading block. It modifies the block settings to allow users to select custom font families, making your Heading blocks more versatile in terms of typography options.
function add_font_family_support(array $settings, array $metadata) {
if ($metadata['name'] !== 'core/heading') {
return $settings;
}
$settings['supports']['typography']['__experimentalFontFamily'] = true;
$settings['supports']['typography']['__experimentalDefaultControls']['fontFamily'] = true;
return $settings;
}
add_filter('block_type_metadata_settings', 'add_font_family_support', 10, 2);
Whether you're fixing outdated data in blocks or customising your Block Editor environment, these snippets will make your work easier and more efficient. A well-optimised Block Editor can significantly improve your workflow, saving you time and frustration in the process.
Subscribe to my newsletter
Read articles from Matt Watson directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Matt Watson
Matt Watson
Hello there! I’m Matt Watson — a developer, father, and husband, remotely coding from the charming landscapes of Yorkshire, UK. I’ve been crafting wonderful things with WordPress since 2006 and spinning webs (the good kind) since 1996 — yes, I survived the dial-up era! Solving (and occasionally committing) WordPress-based crimes, I juggle code by day and dad jokes by night. Remote worker since 2014, I’ve mastered the art of debugging in my slippers while keeping the coffee industry in business.