Day 2: Superpowers and Secret Identities 🦸♂️🦸♀️
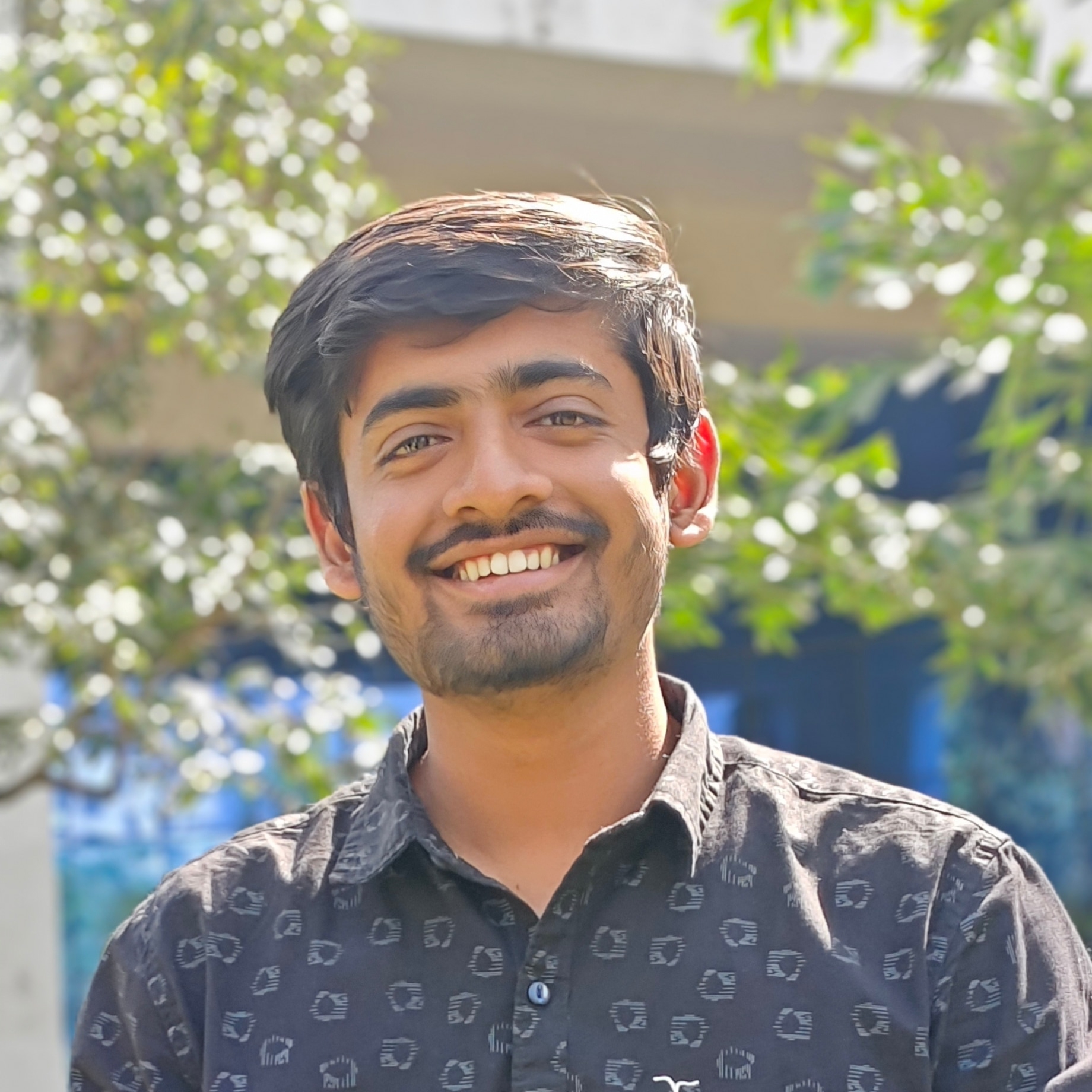
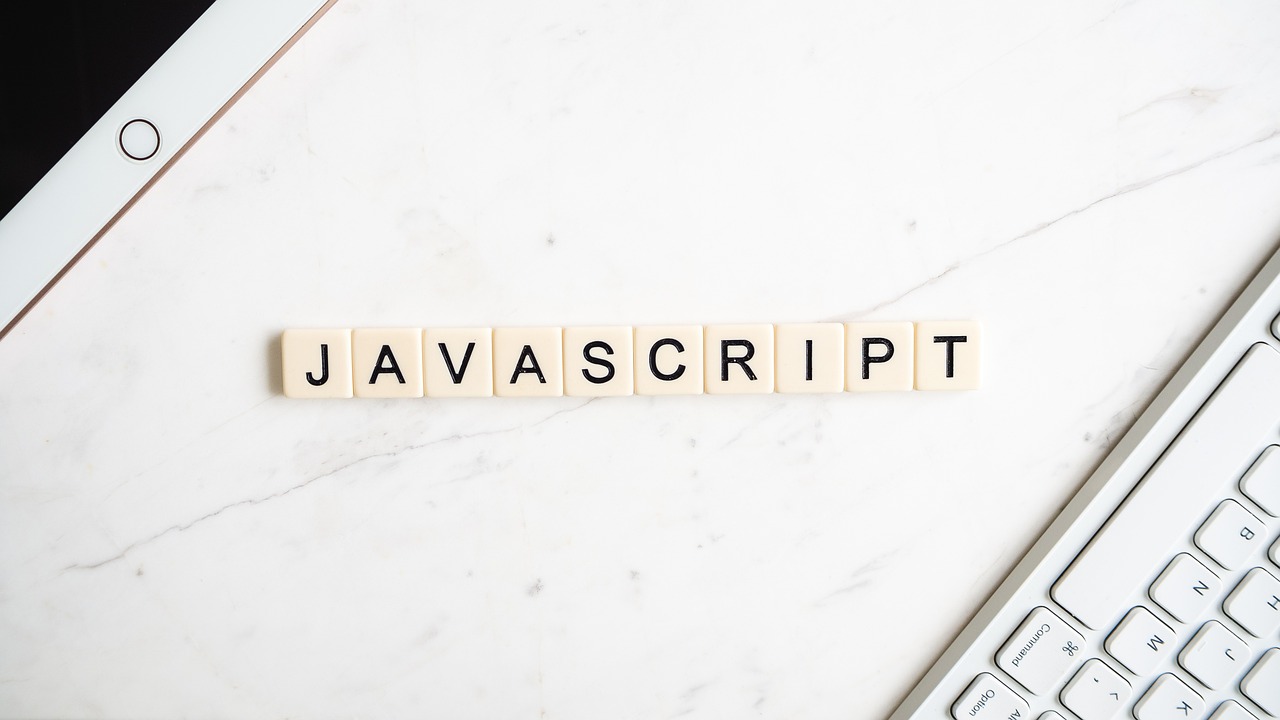
Today’s Goal: Learn how to use var
, let
, and const
through fun examples about superheroes and their secret identities! 🌟
1. Intro: Variables as Superhero Secret Identities
Imagine you’re a superhero! 🦸♂️ But, to keep your identity safe, you use variables to store your powers and secret name. Different heroes have different rules – some can change their powers, some can’t! That’s where var
, let
, and const
come in.
2. Meet the Superhero Variables: var
, let
, and const
Here’s how they work in our superhero world:
Variable Type | Can Change (Reassign)? | Scope | Best Fit For |
var | Yes 🌀 | Function | Old-school superheroes (a bit unpredictable!) 🏰 |
let | Yes 🔄 | Block | Changing powers (like Spiderman learning new tricks) 🕸️ |
const | No 🚫 | Block | Permanent things (like Captain America's shield 🛡️) |
3. Fun Code Examples – Superhero Style!
🏰 1. var
– The Old-School Hero
Think of var
as a hero from the 1950s – still works, but sometimes behaves unexpectedly!
javascriptCopy codevar heroName = 'Iron Man'; // Hero name
console.log(heroName); // Output: Iron Man
var heroName = 'War Machine'; // Re-declared!
console.log(heroName); // Output: War Machine 🤯 (Wait, what?!)
📝 Why avoid var
?
It lets you redeclare variables, which might confuse you.
It has function scope, not block scope, so it’s harder to manage in modern code.
🕸️ 2. let
– The New Age Hero (Spiderman)
With let
, the hero can change powers as they grow. Think about Spiderman – he discovers new abilities over time!
javascriptCopy codelet heroName = 'Peter Parker'; // Secret identity
heroName = 'Spiderman'; // New identity when wearing the suit
console.log(heroName); // Output: Spiderman 🕸️
Block Scope Example:
javascriptCopy codeif (true) {
let heroName = 'Miles Morales'; // Only inside this block
console.log(heroName); // Output: Miles Morales 🕸️
}
console.log(heroName); // Error: heroName is not defined (outside block)
🛡️ 3. const
– The Unchanging Hero (Captain America’s Shield)
With const
, some things never change. Captain America’s shield 🛡️, for example, is always with him.
javascriptCopy codeconst heroWeapon = 'Shield';
console.log(heroWeapon); // Output: Shield 🛡️
// Trying to change it
heroWeapon = 'Mjolnir'; // Error: Assignment to constant variable
Using const
with Objects:
Even though the reference can’t change, you can still modify properties inside the object.
javascriptCopy codeconst hero = { name: 'Thor', weapon: 'Mjolnir' };
hero.weapon = 'Stormbreaker'; // Changing the weapon inside the object
console.log(hero); // Output: { name: 'Thor', weapon: 'Stormbreaker' } ⚡
4. Challenge: Create Your Own Hero 🦸♂️
Your task today is to use variables to describe a superhero! 🎨 Store their name, powers, and weapon using the appropriate variable types.
Sample Code:
javascriptCopy codelet heroName = 'Bruce Wayne'; // Secret identity
const heroAlias = 'Batman'; // Permanent alias 🦇
let superpowers = ['Martial Arts', 'Gadgets', 'Stealth'];
console.log(`${heroAlias}, also known as ${heroName}, has these powers: ${superpowers}`);
Your Challenge:
Create a hero with 3 superpowers using an array.
Switch their identity (like from Clark Kent to Superman) with
let
.Use
const
to store something they can’t change (like their main weapon or symbol).
5. Quiz Time! 🧠
Which of the following lines will cause an error?
javascriptCopy codeconst weapon = 'Shield'; weapon = 'Sword';
javascriptCopy codelet hero = 'Flash'; hero = 'Reverse Flash';
javascriptCopy codevar alias = 'Iron Man'; var alias = 'War Machine';
Answer: Option 1 will cause an error because const
variables cannot be reassigned.
6. Recap: What Did You Learn Today?
var
is like an old-school hero – works but can be confusing.let
is a modern hero that can change their abilities.const
is for things that stay the same, like a shield or an unbreakable weapon.
7. Share Your Hero! 🦸♂️
Tweet your superhero description using your favorite variables and share with the hashtag #JavaScriptHeroes. Example:
“Day 2 of my JS journey: Created a hero using
let
andconst
. Meet Spiderman 🕸️! #JavaScriptHeroes #SuperFunLearning”
Next up: Day 3 – Data Types Party 🎉 where we’ll learn how to store everything from numbers to emojis in JavaScript! Keep up the amazing work! 🚀
Subscribe to my newsletter
Read articles from Risharth pardeshi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
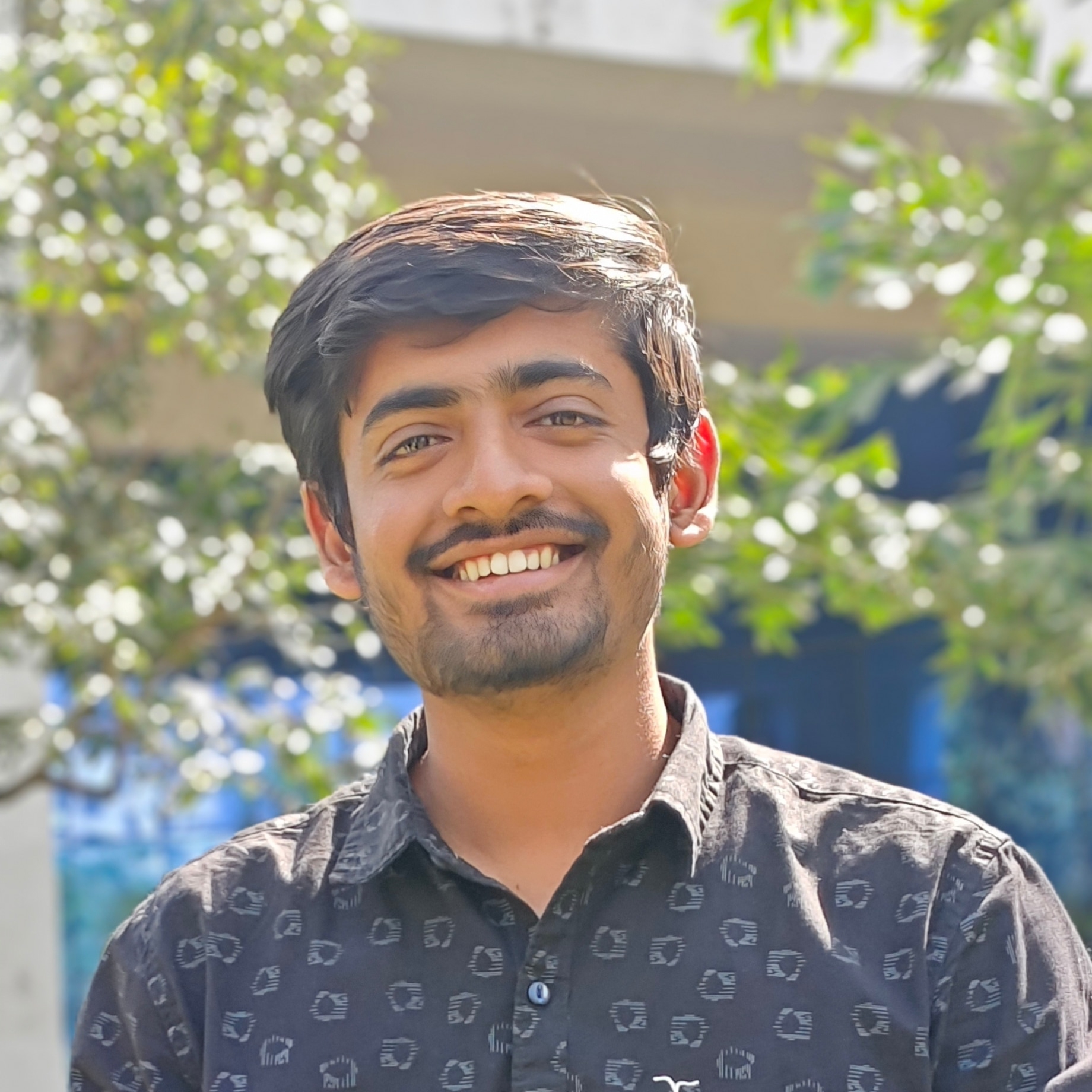
Risharth pardeshi
Risharth pardeshi
I hope this message finds you well. I am Risharth Pardeshi I am passionate about Computer Science and Engineering, and I have a strong foundation in this field. I graduated with a Bachelor of Engineering from the Laxmi Narayan College of Technology in Indore, India, in July 2024, with a CGPA of 7.98. Here is a brief overview of my technical strengths: Languages: C++, HTML, CSS, JavaScript, SQL Frameworks: React.js, Next.js, Tailwind CSS, Node.js Databases/OS: MySQL, MongoDB, Firebase, Linux Technical Skills: Data Structures and Algorithms, Git, DBMS, OS, Object-Oriented Programming, Computer Networking, Vercel, Postman