Java Interview Questions
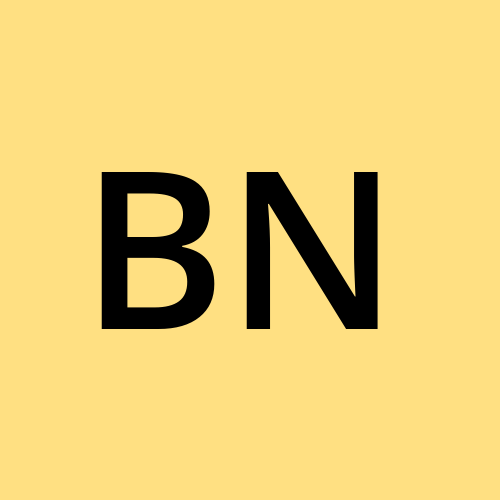
String:
What is the difference between
String
,StringBuilder
, andStringBuffer
in Java?Explain how
String
is immutable in Java. What are the benefits of immutability?How does the
intern()
method work withString
objects?What will be the output of the following code?
String s1 = "abc"; String s2 = new String("abc"); System.out.println(s1 == s2); System.out.println(s1.equals(s2));
Can you explain how the
split()
method works in theString
class? Provide an example.How can you reverse a string in Java without using a built-in reverse function?
What is the difference between
==
andequals()
when comparing strings?How does Java handle string concatenation internally? What are the performance implications?
What will be the output of the following code?
String str = "Java"; str.concat("Programming"); System.out.println(str);
Explain the concept of
String Pool
in Java. How does it optimize memory usage?How does the
substring()
method work in Java? What is the time complexity ofsubstring()
?How can you convert a
String
to anint
and vice versa in Java?What is the difference between
String.valueOf()
andtoString()
?How does the
replace()
method work in theString
class? Provide an example.Explain how you can check if a
String
is a palindrome in Java.What is the difference between
trim()
andstrip()
methods in Java?How can you convert a
String
to achar[]
in Java? Provide an example.How can you efficiently concatenate a large number of strings in Java?
What is the difference between
String.intern()
and a regular string in the pool?Can you explain how string immutability improves performance in Java?
What is
String.intern()
method, and when would you use it?How does
StringBuilder
internally manage memory, and how does it differ fromStringBuffer
?What is the output of the following code, and why?
String a = "abc"; String b = "abc"; String c = new String("abc"); System.out.println(a == b); System.out.println(a == c);
Tracing: What will be the output of the following code?
String s = "Java"; s = s.concat(" is fun"); System.out.println(s);
Coding: Write a Java program to find the first non-repeating character in a given string.
OOPs Concepts:
What is the difference between method overloading and method overriding? Can we override a static method? Why or why not?
Can a constructor be final or static in Java? Why or why not?
Can you have an abstract class without any abstract methods?
How can we achieve multiple inheritance in Java?
What will happen if a class has two interfaces with default methods having the same method signature? How would you resolve the conflict?
Can you overload a constructor in Java? If yes, how is it different from method overloading?
What is the difference between encapsulation and abstraction in Java OOP?
Can a private method be inherited in Java?
Can you explain polymorphism with an example in Java?
How does Java handle object creation with respect to constructor chaining?
How does the
super
keyword work in Java? Provide a use case.Explain the significance of the
instanceof
keyword in Java. Can it be used with interfaces?What is the significance of the
this
keyword? Can we use it inside static methods?What is the difference between composition and inheritance? Which is preferred in Java?
How does the Java Virtual Machine (JVM) distinguish between overloaded methods?
Can we override a private or static method in Java? Why or why not?
What is a copy constructor? Does Java have it by default?
What is the difference between an interface and an abstract class? Can a class be both abstract and final?
How do access modifiers affect method overriding and inheritance?
What is object slicing, and how does it occur in Java?
What is composition over inheritance? Why is it recommended in design patterns?
How is the
@Override
annotation useful in Java? What happens if you don't use it?Can you explain what shadowing is in Java with respect to variables?
What is a bounded type parameter in generics, and why is it useful?
What is method overriding covariant return type, and why is it important in Java?
Coding: Write code to demonstrate method overriding in Java. Create a
Parent
class and aChild
class, and override a method to show polymorphism.
Static:
Can you access non-static members from a static context? Why not? Provide an example.
What is the output of the following code?
class Test { static { System.out.println("Static block"); } Test() { System.out.println("Constructor"); } public static void main(String[] args) { Test obj = new Test(); Test obj2 = new Test(); } }
Explain the difference between a static block, static method, and static variable in Java.
What happens if you declare a static method in a subclass that overrides a static method in the superclass?
Can we access the
this
keyword inside a static method? Why or why not?Can you create a static class inside a non-static class? If yes, explain how it works.
Can we declare static methods inside an interface? How are they different from default methods?
What is the output when you call a static method from a null object reference?
Can a static block throw an exception?
Can static methods be synchronized in Java?
Can we have multiple static blocks in a Java class? How are they executed?
Explain the lifecycle of static variables in Java.
Can a static method access instance variables in any scenario?
What is the significance of static import? Provide an example.
Can static methods be abstract? Why or why not?
How does JVM initialize static variables when a class is loaded?
Can we serialize static fields in Java?
Can a static method in Java call another static method without using an object? Why or why not?
What is a static factory method? How does it differ from constructors?
Can we declare static variables inside static methods? Explain with an example.
How does the JVM manage the lifecycle of static variables in memory?
What is the static import feature in Java, and when would you use it?
Explain the role of static initialization blocks when working with constant values.
Tracing: Given the following code, what will be the output?
class A { static { System.out.println("Static A"); } A() { System.out.println("Constructor A"); } } class B extends A { static { System.out.println("Static B"); } B() { System.out.println("Constructor B"); } } public class Test { public static void main(String[] args) { B b = new B(); } }
Coding: Write code to demonstrate static block execution in a class with inheritance. Explain the order in which static blocks and constructors are executed.
Final:
What is the significance of the
final
keyword in Java? Can you give examples of final for variables, methods, and classes?Can you change the object that a final reference variable points to? Explain with an example.
What happens if you declare a method as final and try to override it?
What is the difference between immutability and using the final keyword?
Can we declare a final method in an abstract class?
What will happen if we declare a class as both final and abstract?
Can you declare an interface method as final? Why or why not?
Can final variables be initialized later? If yes, explain with an example.
Can a final variable be modified inside a constructor?
What is the use of a blank final variable in Java?
Can we declare a final variable without initializing it? Provide an example.
Can we change the reference of a final object if the object is mutable?
How does the final keyword work with inheritance? Provide an example.
What happens if you declare a constructor as final in Java?
Can final methods be overloaded? If yes, how?
What are the performance implications of the final keyword in Java?
Can you explain the difference between the final keyword and the immutability of an object?
Why is it a good practice to use final variables in multi-threaded programs?
Can a
final
method be overridden by a subclass if it is inside an anonymous class?What are the implications of having a
final
class implementing an interface?Can we declare a method
final
andabstract
together? Why or why not?Why is the
final
keyword important for constants in Java?Tracing: What will be the output of the following code?
class Test { final int a = 10; void display() { a = 20; } public static void main(String[] args) { Test obj = new Test(); obj.display(); } }
Coding: Write code to demonstrate how a final method cannot be overridden in a subclass.
Coding: Write a Java program to demonstrate that a final variable can only be assigned once and cannot be changed later.
Abstract Classes and Interfaces:
Can you have a constructor in an abstract class? If yes, when is it invoked?
What is the difference between an abstract class and an interface? When should you use one over the other?
Can an abstract class have a
main()
method? What will happen if we run it?Can a class implement multiple interfaces? Can it extend multiple classes? Why or why not?
If an interface has 100 methods, do you need to implement all methods in the implementing class? Explain.
Can an abstract class implement an interface without providing an implementation for all methods?
Can we have static methods in an interface? How are they different from default methods?
What is the purpose of having private methods in interfaces?
What happens if two interfaces have methods with the same signature but different return types, and a class implements both interfaces?
Can a non-abstract class extend an abstract class without implementing any abstract methods?
Can an abstract class extend another abstract class?
Can we mark an abstract class as final in Java?
What happens if a class does not implement all abstract methods of its abstract parent class?
Can an interface have instance variables? Why or why not?
What is the diamond problem in Java, and how does the interface solve it?
Can you instantiate an abstract class? If not, how can you use its constructor?
What are the differences between default and static methods in an interface?
Can an abstract class have private methods? Why would you do this?
How does the
@FunctionalInterface
annotation work in Java, and why is it useful?Can an interface have a constructor? Explain with reasoning.
What is the difference between a
default
method in an interface and a normal method in a class?Can abstract classes implement interfaces? If yes, what is the significance?
Tracing: What will be the output of the following code?
interface A { void display(); } class B implements A { public void display() { System.out.println("Display from B"); } } class C extends B { public void display() { System.out.println("Display from C"); } } public class Test { public static void main(String[] args) { A obj = new C(); obj.display(); } }
Coding: Write a program to demonstrate multiple inheritance using interfaces.
Coding: Create an abstract class
Shape
with a methodcalculateArea()
. Implement two subclassesCircle
andRectangle
that calculate the area of the respective shapes.
Exception Handling:
What is the difference between checked and unchecked exceptions in Java? Provide examples.
Can you catch more than one type of exception in a single catch block? How?
What is the difference between
throw
andthrows
in exception handling?What will happen if you throw an exception in the finally block?
Explain the difference between
Error
andException
in Java.Can you override a method that throws a checked exception to throw an unchecked exception instead?
What happens if an exception is not handled in a multi-threaded application?
Can a constructor throw an exception? If yes, what will happen?
Can you catch an exception thrown by a static block?
How does the JVM handle exceptions when they are not caught by any catch block?
Can we have a try block without a catch block? Provide an example.
Can you rethrow an exception in Java? If yes, explain how.
What happens when an exception occurs in a catch block?
Explain the purpose of the
finally
block. Will it always be executed?Can we write multiple
finally
blocks for a single try-catch block?What is the difference between checked and unchecked exceptions in Java?
How does the
throw
keyword differ from thethrows
keyword in Java?Can a
catch
block throw an exception? Explain how you would handle this.How would you handle exceptions in Java without using
try-catch
?What is
SuppressedException
in Java, and how is it used?Tracing: What will be the output of the following code?
public class Test { public static void main(String[] args) { try { System.out.println(10 / 0); } catch (Exception e) { System.out.println("Caught Exception"); } finally { System.out.println("Finally block executed"); } } }
Coding: Write a program to demonstrate custom exception handling by creating a
InvalidAgeException
that throws an exception if the age is below 18.Coding: Write code to demonstrate how to rethrow an exception from a catch block.
Coding: Write a program to demonstrate the order of exception handling when a method throws multiple exceptions.
Coding: Write a program to demonstrate exception handling when an exception occurs inside a catch block.
Collections:
What is the difference between
ArrayList
andLinkedList
in terms of performance and internal implementation?Explain the difference between
HashSet
andTreeSet
. In what scenarios would you use each?How does the
HashMap
work internally? Explain the role ofhashCode()
andequals()
methods.What is the difference between
HashMap
andHashtable
? Why isHashtable
considered legacy?How does
ConcurrentHashMap
differ fromHashMap
in terms of thread safety and performance?Explain the differences between
List
,Set
, andMap
interfaces.What is the difference between
fail-fast
andfail-safe
iterators? Give examples of collections that use each type.Can you explain the concept of "resize" in
ArrayList
? How does it impact performance?What is the difference between
Comparable
andComparator
? When would you use one over the other?What is a
PriorityQueue
in Java? Provide an example of its usage.Can you sort a
HashMap
? If so, how? Provide an example.How does
LinkedHashMap
maintain the order of elements, and how does it differ fromHashMap
?Explain how the
remove()
method works inArrayList
. What happens if you try to remove an element during iteration?How can you make a collection immutable in Java? Provide examples.
What are the performance implications of using a
TreeMap
versus aHashMap
?How does the
ConcurrentLinkedQueue
work, and in which scenarios is it useful?Can you create a synchronized
ArrayList
orHashMap
? If yes, how?What is the difference between
LinkedHashSet
andHashSet
?How does
ConcurrentSkipListMap
differ from aTreeMap
in terms of concurrency?What are the pros and cons of using a
LinkedHashMap
over aHashMap
?What is
EnumMap
, and why is it optimized for use with enumerations?How does a
TreeSet
maintain order internally, and what are its performance implications?What are the differences between
SynchronousQueue
andArrayBlockingQueue
?Tracing: What will be the output of the following code?
public class Test { public static void main(String[] args) { Set<String> set = new HashSet<>(); set.add("one"); set.add("two"); set.add("three"); set.add("one"); for (String s : set) { System.out.println(s); } } }
Coding: Write a Java program to remove duplicates from an
ArrayList
of integers without using any built-in functions.
Threads and Concurrency:
What is the difference between
Thread
andRunnable
in Java? Which one is preferred?Explain the concept of synchronization in Java. What are the different ways to achieve thread safety?
What is a
volatile
keyword in Java, and how is it different fromsynchronized
?Can you explain the purpose of the
join()
method in thread management?How does the
wait()
,notify()
, andnotifyAll()
methods work in thread communication? Provide a use case.What is the difference between
ExecutorService
and creating your own threads usingnew Thread()
?Explain the concept of deadlock in Java. How can you prevent it?
What is the difference between a
ReentrantLock
and a synchronized block in Java?What is the purpose of the
ThreadLocal
class in Java? When would you use it?What is the
Callable
interface in Java, and how is it different fromRunnable
?Explain the difference between
CyclicBarrier
andCountDownLatch
. Provide an example.What is a
ForkJoinPool
in Java, and how does it help in parallel processing?What is the difference between
sleep()
andyield()
methods in thread management?How does Java handle thread starvation and fairness in thread scheduling?
Can you explain the concept of a daemon thread in Java? How is it different from a user thread?
What are
Atomic
classes in Java's concurrency package? Provide examples.What is a
Semaphore
in Java, and how is it used in thread synchronization?What is the difference between
Future
andCompletableFuture
in Java?What are the advantages of using
ForkJoinPool
over a traditionalThreadPoolExecutor
?How does the
AtomicInteger
class help avoid synchronization issues?Explain the
ThreadLocal
class and give a scenario where it's beneficial.What is the purpose of
CountDownLatch
and how does it differ fromCyclicBarrier
?What is the
Semaphore
class, and when would you use it?Tracing: What will be the output of the following code?
public class Test { public static void main(String[] args) { Thread t = new Thread(() -> { for (int i = 0; i < 5; i++) { System.out.println(i); } }); t.start(); t.run(); } }
Coding: Write a Java program to demonstrate a producer-consumer problem using
wait()
andnotify()
methods.
JVM (Java Virtual Machine) Tricky Questions:
What is the difference between the JVM, JDK, and JRE?
How does the JVM load a class, and what is the significance of classloaders in Java?
Explain the structure of the JVM memory model (Heap, Stack, Method Area, and more). How does the JVM allocate memory for objects and methods?
What is the difference between the young generation and the old generation in the JVM memory?
Explain how the garbage collection process works in Java. What are the different types of garbage collectors available?
What is the difference between serial, parallel, and CMS garbage collectors in the JVM?
What is a memory leak in Java, and how can it occur despite automatic garbage collection?
What is the role of the Just-In-Time (JIT) compiler in the JVM? How does it optimize the execution of bytecode?
What is the permgen space in older versions of Java, and how does it differ from the metaspace in newer versions (Java 8+)?
Explain what happens during a full garbage collection (GC) cycle in the JVM. What are its implications for performance?
What is the
OutOfMemoryError: PermGen space
? How can you resolve this error?What is the purpose of the
-Xms
and-Xmx
JVM options? When should you adjust these settings?What is the role of the
-XX:+UseG1GC
flag in JVM? How does G1GC differ from other collectors?How do
soft references
,weak references
, andphantom references
affect garbage collection in Java?What is the difference between heap memory and stack memory in Java?
How does the JVM handle multithreading with respect to memory visibility and synchronization?
What is a class file in Java, and what kind of information does it contain about the bytecode?
What is
escape analysis
in the JVM, and how does it affect object allocation and garbage collection?How does the JVM handle native methods, and what is the significance of the
JNI (Java Native Interface)
?What are JVM tuning parameters, and how can you optimize memory usage for a large Java application?
Memory Management Tricky Questions:
What is the difference between strong, weak, soft, and phantom references in Java?
What are memory leaks, and how can you identify and prevent them in Java applications?
Explain how the JVM’s garbage collector works. What triggers a garbage collection event?
What are the different memory regions in Java (e.g., Heap, Stack, Metaspace), and what is stored in each?
What happens when the JVM runs out of heap memory?
What is the difference between minor GC and major (full) GC in the JVM?
Explain how to use memory profiling tools like
VisualVM
orJConsole
to monitor and troubleshoot memory issues.What is the use of the
finalize()
method in Java, and why is it considered deprecated?How does the JVM allocate memory for an object during object creation?
What is a thread stack in Java? How does the JVM allocate memory for each thread?
What is a
memory barrier
, and how does it work in multithreaded applications?Can you explain how memory alignment works in the JVM? Why is it important for performance?
How does the JVM handle class metadata in the heap (especially in versions after Java 8)?
What are the consequences of not properly releasing native resources in Java?
What is the difference between direct memory and heap memory, and when would you use direct memory?
What is the
java.lang.OutOfMemoryError: Metaspace
error, and how would you debug and resolve it?What is
GC tuning
, and how do you approach it for applications with large datasets or high throughput?How can you manage the lifecycle of large objects in memory to avoid frequent full GCs?
What are the benefits and risks of using
-XX:+UseCompressedOops
in the JVM?How does
Escape Analysis
help in optimizing memory usage in Java?
Java 8 new features
Lambdas & Functional Interfaces:
What is a lambda expression in Java, and how does it differ from an anonymous inner class?
Can you explain the syntax of lambda expressions and provide examples for different use cases (e.g., single line, multi-line)?
How do lambda expressions contribute to functional programming in Java?
What is the
@FunctionalInterface
annotation, and what are its rules? Can you have multiple abstract methods in a functional interface?Can a lambda expression capture variables from the surrounding context? What are the restrictions on captured variables?
What is the difference between a lambda expression and a method reference in Java?
What happens if a functional interface method throws a checked exception? How can you handle that in a lambda expression?
How does type inference work in lambda expressions? Can you explicitly specify the types of parameters?
Can lambda expressions access static and instance variables from their enclosing class?
What are the performance implications of using lambda expressions compared to anonymous inner classes?
Can you create recursive lambdas? How would you implement them?
Explain how lambdas improve the readability and maintainability of code. Can you give an example?
What is the difference between
Predicate
,Function
,Supplier
, andConsumer
interfaces?Can you use a lambda expression to implement the
Runnable
interface? Provide a code example.What happens if you use
this
inside a lambda expression? What does it refer to?Can you overload a method that takes lambda expressions? What rules apply in such cases?
How does the
effectively final
concept apply to variables used in lambda expressions?What are method references, and how are they different from lambdas? Provide examples.
Can lambda expressions be used in synchronized blocks or methods?
How are lambdas represented internally in the JVM? Explain the
invokedynamic
instruction related to lambdas.
Streams API:
What is the Streams API in Java 8, and how does it differ from traditional collections processing?
Can you explain the difference between
intermediate
andterminal
operations in a stream? Provide examples.What is the significance of laziness in streams, and how does it affect performance?
What is the difference between
map()
andflatMap()
in streams?Explain how
filter()
,map()
, andreduce()
work in the Streams API.How does the Streams API handle parallel processing? How do you make a stream parallel?
What is the difference between
findAny()
andfindFirst()
in the Streams API?Explain the difference between
forEach()
andforEachOrdered()
in streams.What is the difference between
collect()
andreduce()
methods in streams? Provide use cases for each.How do you convert a stream back into a collection, like a
List
or aSet
?What are the potential pitfalls of using parallel streams? How does it impact thread safety and performance?
Explain how
groupingBy()
andpartitioningBy()
collectors work in streams.What is a
Spliterator
, and how does it differ from anIterator
in streams?Can streams be reused after a terminal operation? Why or why not?
What is short-circuiting in streams, and how do
limit()
andfindFirst()
demonstrate it?What is the difference between a sequential stream and a parallel stream? How do you convert one to another?
Can a stream have infinite data? How would you process such streams?
What is a
Stream
pipeline, and how does it improve the clarity of code in functional programming?How can you use streams to find the sum of an array of integers?
What are
Collectors
, and how do they work with the Streams API? Provide examples of commonly used collectors.
Optional:
What is
Optional
in Java 8, and why was it introduced?How does
Optional
help in avoidingNullPointerException
?What is the difference between
Optional.of()
,Optional.ofNullable()
, andOptional.empty()
?What is the purpose of the
orElse()
,orElseGet()
, andorElseThrow()
methods inOptional
?How can you chain
Optional
operations usingmap()
andflatMap()
? Provide examples.What happens if you call
get()
on an emptyOptional
? How should you handle it?What is the difference between
isPresent()
andifPresent()
inOptional
?How does
Optional
relate to streams, and how can you convert anOptional
to a stream?Can you use
Optional
in method parameters? Is it considered good practice? Why or why not?How does
Optional
improve code readability and reduce boilerplate code for null checks?What is the difference between
Optional.map
()
andOptional.flatMap()
?How can you combine two
Optional
values?Is
Optional
serializable? Why or why not?Can you use
Optional
with primitive types? If not, what alternatives are provided by Java?How does
Optional
fit into the overall functional programming paradigm introduced in Java 8?Can you return an
Optional
from a method? How does this improve code safety?Why is
Optional
not designed to be used for fields in Java classes?What is a typical use case for
Optional
when dealing with collections?Explain how
Optional
can be used to express the absence of a value in functional APIs.What are the performance implications of using
Optional
extensively in your code?
Default Methods in Interfaces:
What are default methods in interfaces, and why were they introduced in Java 8?
Can you override a default method in an implementing class? What happens if you don’t?
What happens if a class implements two interfaces with conflicting default methods? How do you resolve it?
Can a default method call other methods from the same interface? Provide an example.
Can a default method be
synchronized
? Why or why not?Why can’t a default method be static or final?
Can a default method access instance variables in the implementing class?
What is the difference between default methods and abstract methods in interfaces?
How do default methods impact backward compatibility with older Java versions?
Can a default method in an interface call a static method from the same interface?
How do default methods contribute to the multiple inheritance problem in Java?
What is the significance of the
super
keyword when calling a default method from an interface?Can you declare a default method as
private
? Why or why not?Can a default method be used in an interface that already has abstract methods?
How do default methods enable evolution of interfaces in large libraries like the JDK?
Explain the use case for default methods in functional programming scenarios in Java.
What are the implications of default methods for multiple inheritance in Java?
Can you create a default method in an interface that calls another interface’s default method?
Can you use default methods to provide a base implementation for commonly used methods?
How do default methods interact with lambda expressions and functional interfaces?
New Date and Time API (java.time):
What are the limitations of the old
java.util.Date
andjava.util.Calendar
classes, and how does the new Date/Time API improve upon them?What is the difference between
LocalDate
,LocalTime
, andLocalDateTime
classes in Java 8?How can you parse and format dates using
DateTimeFormatter
in Java 8?What is
ZonedDateTime
, and how does it handle time zones?How do you calculate the difference between two dates using the new Date/Time API?
What is the purpose of
Instant
in Java 8, and how is it used for timestamp management?How do you convert between
LocalDate
andLocalDateTime
?What are
Period
andDuration
classes, and how do they differ?Can you explain the immutability of the Date/Time classes in Java 8 and why it’s important?
What is
TemporalAdjuster
, and how is it used for date manipulation?How do you handle leap years and daylight savings time with the new API?
Can you explain the significance of
Clock
and how it can be used for time manipulation in tests?How do you compare two dates using the new Date/Time API?
What are the advantages of
java.time
package classes overjava.sql.Date
for database interactions?How does the
ZoneId
class work, and how do you use it to represent time zones?How can you convert the new date/time API to
java.util.Date
and vice versa?What are the key improvements of
DateTimeFormatter
overSimpleDateFormat
?What are
ChronoUnit
andChronoField
, and how are they used for date manipulations?How do
MonthDay
andYearMonth
classes work, and how do they differ fromLocalDate
?What is the
OffsetDateTime
class, and how does it handle UTC offsets?
Subscribe to my newsletter
Read articles from Bikash Nishank directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
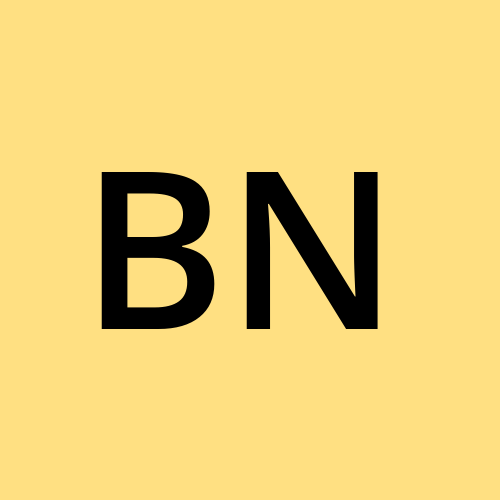