The Dolphin's Divide-and-Conquer
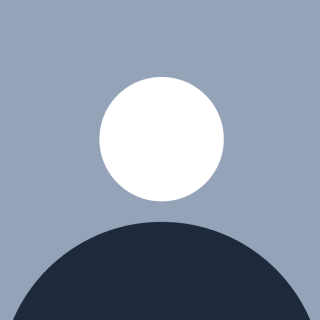
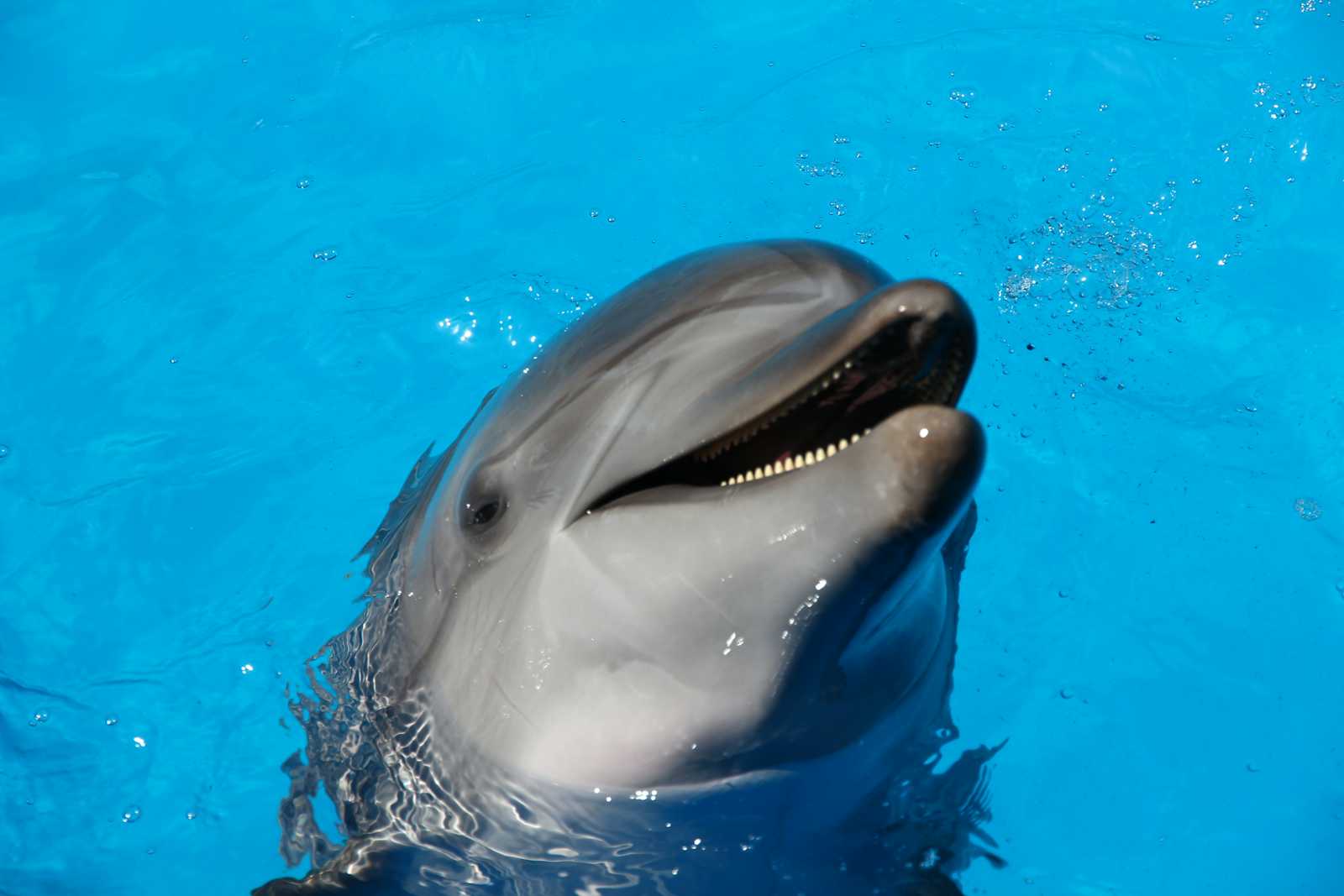
Imagine a group of dolphins working together to catch fish. Instead of chasing after every fish, they divide the task, working in smaller teams to round up the fish before coming together to share the catch. This approach is efficient and strategic, and it mirrors how Merge Sort works.
Merge Sort is a divide-and-conquer algorithm that breaks down a large unsorted list into smaller sublists, sorts each sublist, and then merges them back together to form a sorted list. By breaking the task into manageable pieces, Merge Sort is both efficient and powerful, especially for large datasets.
In this article, we’ll explore how Merge Sort works, understand its advantages, and dive into some fun challenges!
Simple Merge Sort – The Dolphins' Method
Merge Sort 101 – Divide, Conquer, and Merge
Merge Sort works by dividing the unsorted list into smaller sublists, sorting those sublists, and then merging them back together. Like dolphins working in smaller teams before regrouping, Merge Sort makes the sorting process much easier by breaking it down.
Step-by-Step Example with Code
Let’s take a list of fish sizes: [12, 3, 8, 5, 7, 2]
.
Divide the List:
The first step is to divide the list into two halves:[12, 3, 8]
and[5, 7, 2]
.Divide Again:
Each of those halves is further divided:[12, 3]
,[8]
,[5, 7]
, and[2]
.Sort and Conquer:
Each smaller list is sorted:
[12, 3]
becomes[3, 12]
[5, 7]
is already sorted.Merge the Sorted Sublists:
Now, merge the smaller sorted sublists:
[3, 12]
and[8]
are merged to form[3, 8, 12]
.
[5, 7]
and[2]
are merged to form[2, 5, 7]
.Final Merge:
The two larger sorted sublists[3, 8, 12]
and[2, 5, 7]
are merged to form the fully sorted list:
Final list becomes:[2, 3, 5, 7, 8, 12]
.
Code Snippets for Simple Merge Sort
def merge_sort(arr):
if len(arr) > 1:
mid = len(arr) // 2
left_half = arr[:mid]
right_half = arr[mid:]
merge_sort(left_half)
merge_sort(right_half)
i = j = k = 0
while i < len(left_half) and j < len(right_half):
if left_half[i] < right_half[j]:
arr[k] = left_half[i]
i += 1
else:
arr[k] = right_half[j]
j += 1
k += 1
while i < len(left_half):
arr[k] = left_half[i]
i += 1
k += 1
while j < len(right_half):
arr[k] = right_half[j]
j += 1
k += 1
return arr
# Example usage
fish_sizes = [12, 3, 8, 5, 7, 2]
print(merge_sort(fish_sizes))
function mergeSort(arr) {
if (arr.length <= 1) {
return arr;
}
const mid = Math.floor(arr.length / 2);
const left = mergeSort(arr.slice(0, mid));
const right = mergeSort(arr.slice(mid));
return merge(left, right);
}
function merge(left, right) {
let result = [], i = 0, j = 0;
while (i < left.length && j < right.length) {
if (left[i] < right[j]) {
result.push(left[i]);
i++;
} else {
result.push(right[j]);
j++;
}
}
return result.concat(left.slice(i)).concat(right.slice(j));
}
// Example usage
let fishSizes = [12, 3, 8, 5, 7, 2];
console.log(mergeSort(fishSizes));
#include <iostream>
using namespace std;
void merge(int arr[], int l, int m, int r) {
int n1 = m - l + 1;
int n2 = r - m;
int L[n1], R[n2];
for (int i = 0; i < n1; i++)
L[i] = arr[l + i];
for (int i = 0; i < n2; i++)
R[i] = arr[m + 1 + i];
int i = 0, j = 0, k = l;
while (i < n1 && j < n2) {
if (L[i] <= R[j]) {
arr[k] = L[i];
i++;
} else {
arr[k] = R[j];
j++;
}
k++;
}
while (i < n1) {
arr[k] = L[i];
i++;
k++;
}
while (j < n2) {
arr[k] = R[j];
j++;
k++;
}
}
void mergeSort(int arr[], int l, int r) {
if (l >= r) {
return;
}
int m = l + (r - l) / 2;
mergeSort(arr, l, m);
mergeSort(arr, m + 1, r);
merge(arr, l, m, r);
}
int main() {
int fishSizes[] = {12, 3, 8, 5, 7, 2};
int n = sizeof(fishSizes) / sizeof(fishSizes[0]);
mergeSort(fishSizes, 0, n - 1);
for (int i = 0; i < n; i++)
cout << fishSizes[i] << " ";
return 0;
}
Optimizing Merge Sort – Fine-Tuning the Dolphins' Strategy
Can We Make Merge Sort Faster?
Merge Sort is already an efficient algorithm, but there are a few areas where we can make it even better:
Memory Optimization: Merge Sort requires additional space to store the left and right halves. By using an in-place merge sort, we can reduce the memory overhead.
Switch to Insertion Sort for Small Lists: When sorting smaller sublists (e.g., fewer than 10 elements), Insertion Sort can be faster than Merge Sort. This hybrid approach speeds up sorting by using Merge Sort for larger lists and Insertion Sort for smaller sublists.
In-Place Merge Sort Optimization
def merge_sort_in_place(arr, start, end):
if end - start > 1:
mid = (start + end) // 2
merge_sort_in_place(arr, start, mid)
merge_sort_in_place(arr, mid, end)
merge_in_place(arr, start, mid, end)
def merge_in_place(arr, start, mid, end):
left = arr[start:mid]
right = arr[mid:end]
i = j = 0
k = start
while i < len(left) and j < len(right):
if left[i] < right[j]:
arr[k] = left[i]
i += 1
else:
arr[k] = right[j]
j += 1
k += 1
while i < len(left):
arr[k] = left[i]
i += 1
k += 1
while j < len(right):
arr[k] = right[j]
j += 1
k += 1
# Example usage
fish_sizes = [12, 3, 8, 5, 7, 2]
merge_sort_in_place(fish_sizes, 0, len(fish_sizes))
print(fish_sizes)
Time and Space Complexity – How Efficient is Merge Sort?
Time and Space Complexity Explained
Time Complexity:
- Worst Case (O(n log n)): Merge Sort consistently performs well even with large datasets, making O(n log n) comparisons regardless of whether the list is already sorted or not. The "log n" comes from the repeated division of the list into halves.
Space Complexity:
- Space Complexity (O(n)): Merge Sort requires extra space to store the temporary sublists (left and right halves) during the merging process, leading to a space complexity of O(n). However, with in-place Merge Sort, this can be reduced.
Drawbacks and Real-World Applications
Drawbacks of Merge Sort
Memory Usage: The additional space required for storing temporary arrays makes Merge Sort less efficient in memory-constrained environments, particularly with large datasets.
Not In-Place: By default, Merge Sort is not an in-place sorting algorithm, meaning it requires extra space to hold the divided lists.
Real-World Applications of Merge Sort
Sorting Linked Lists: Merge Sort is highly efficient for sorting linked lists due to its non-reliance on random access of data.
External Sorting: Merge Sort is often used in external sorting, such as sorting data on disk, because of its consistent O(n log n) time complexity and efficiency when handling large datasets.
Stable Sort: Since Merge Sort is a stable algorithm (it preserves the relative order of equal elements), it is useful in applications where this property is important, such as databases or file systems.
Challenge Time – Test Your Skills!
Fun Challenges with Merge Sort
Challenge: Sorting a Pod of Dolphins
You’re organizing dolphins based on their swimming speeds. Use Merge Sort to arrange their speeds:[32, 45, 28, 16, 50, 22]
.Challenge: Merge Two Schools of Fish
Two schools of fish need to be merged into a single sorted list. Use Merge Sort to combine the lists:[18, 24, 30]
and[12, 20, 25]
.
Think you’ve mastered Merge Sort? Try the challenges and share your solutions!
The Dolphins Have Mastered Merge Sort!
By using the smart strategy of divide and conquer, dolphins (and you) can now sort through large datasets efficiently. You’ve learned how to implement and optimize Merge Sort, making it a powerful tool in your sorting arsenal. Stay tuned for the next step in your journey
Ready for the next algorithm? Subscribe to stay updated on Quick Sort!
Subscribe to my newsletter
Read articles from gayatri kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by