The Shark's Precision
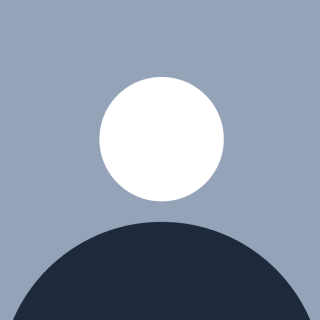
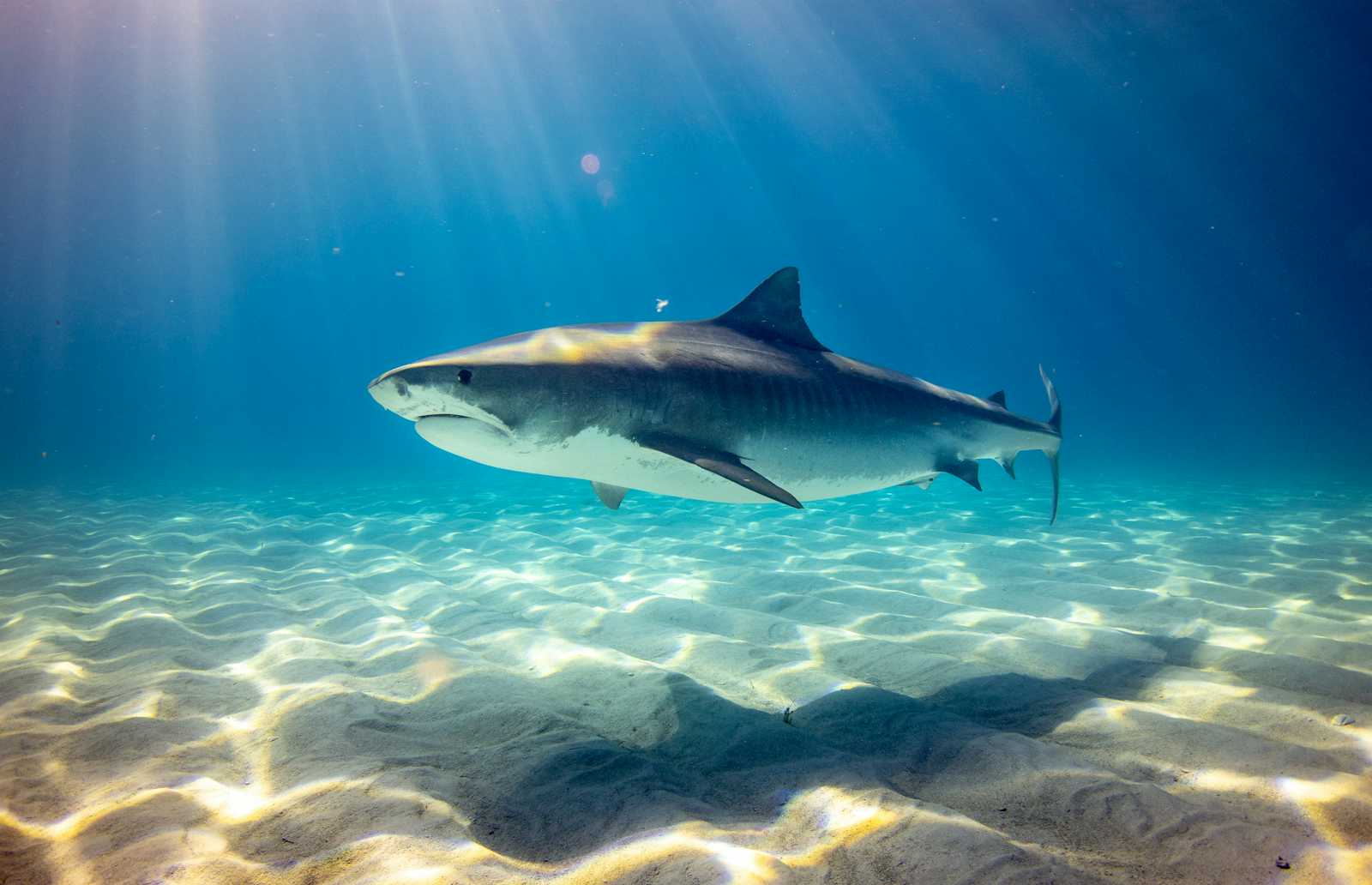
How Sharks Teach Us to Sort Efficiently with Quick Sort
Imagine a shark swimming swiftly through the ocean, making quick, precise decisions to capture its prey. This kind of speed and accuracy is reflected in the way Quick Sort works. Instead of slowly comparing every element, Quick Sort picks a pivot and partitions the list into smaller and larger elements. By dividing and conquering, it sorts elements faster than many other algorithms.
In this article, we’ll explore how Quick Sort uses pivots to organize data efficiently, optimize it for different scenarios, and solve fun challenges. You’ll also learn why the shark is the perfect animal to represent Quick Sort’s speed and precision.
Simple Quick Sort – The Shark’s Speedy Approach
Quick Sort 101 – Sorting with Precision and Pivots
Quick Sort works by selecting a pivot element from the list and partitioning the other elements into two groups: one group with elements smaller than the pivot and one with elements larger. The pivot is then placed in its correct sorted position, and this process is repeated for each group. This efficient partitioning is why Quick Sort is so fast—like a shark quickly finding its target.
Step-by-Step Example with Code
Let’s take a list of fish weights: [15, 3, 9, 7, 2, 10]
.
Select a Pivot:
The shark selects the last element,10
, as the pivot.Partition the List:
All the fish smaller than10
are moved to the left side, and all the fish larger than10
stay on the right side. After partitioning, the list looks like this:[3, 9, 7, 2, 10, 15]
. The pivot10
is now in its correct position.Recursively Sort the Left and Right Groups:
The left group
[3, 9, 7, 2]
is partitioned again. The pivot2
is selected, and the list becomes[2, 3, 7, 9]
.The right group
[15]
contains only one element, so no further sorting is needed.
Final Sorted List:
After recursively sorting both sides, the final sorted list is:
[2, 3, 7, 9, 10, 15]
.
Code Snippets for Simple Quick Sort
def quick_sort(arr):
if len(arr) <= 1:
return arr
pivot = arr[-1]
left = [x for x in arr[:-1] if x <= pivot]
right = [x for x in arr[:-1] if x > pivot]
return quick_sort(left) + [pivot] + quick_sort(right)
# Example usage
fish_weights = [15, 3, 9, 7, 2, 10]
print(quick_sort(fish_weights))
function quickSort(arr) {
if (arr.length <= 1) {
return arr;
}
let pivot = arr[arr.length - 1];
let left = arr.filter(x => x < pivot);
let right = arr.filter(x => x > pivot);
return [...quickSort(left), pivot, ...quickSort(right)];
}
// Example usage
let fishWeights = [15, 3, 9, 7, 2, 10];
console.log(quickSort(fishWeights));
#include <iostream>
using namespace std;
int partition(int arr[], int low, int high) {
int pivot = arr[high];
int i = (low - 1);
for (int j = low; j < high; j++) {
if (arr[j] < pivot) {
i++;
swap(arr[i], arr[j]);
}
}
swap(arr[i + 1], arr[high]);
return (i + 1);
}
void quickSort(int arr[], int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
quickSort(arr, low, pi - 1);
quickSort(arr, pi + 1, high);
}
}
int main() {
int fishWeights[] = {15, 3, 9, 7, 2, 10};
int n = sizeof(fishWeights) / sizeof(fishWeights[0]);
quickSort(fishWeights, 0, n - 1);
for (int i = 0; i < n; i++)
cout << fishWeights[i] << " ";
return 0;
}
Optimizing Quick Sort – Even Faster Sharks
Making Quick Sort Even Quicker
Quick Sort is already one of the fastest sorting algorithms, but there are ways to make it even more efficient:
Choosing a Better Pivot:
Instead of always choosing the last element as the pivot, a better strategy is to choose the median of the first, middle, and last elements. This helps avoid poor pivot choices, especially when the list is already sorted or nearly sorted.Tail Recursion Optimization:
Tail recursion can be optimized to reduce the overhead of recursive function calls, improving Quick Sort’s performance.
Step-by-Step Example of Median-of-Three Pivot Selection
Let’s use the same list [15, 3, 9, 7, 2, 10]
, but this time we’ll select the pivot as the median of the first, middle, and last elements:
Select the Pivot:
The first element is15
, the middle element is9
, and the last element is10
. The median of these three is10
, so10
is selected as the pivot.Partition the List:
After partitioning, the list looks like this:[3, 9, 7, 2, 10, 15]
. The pivot10
is in the correct position.Recursively Sort:
- The left group
[3, 9, 7, 2]
is sorted recursively, and the right group[15]
is already sorted.
- The left group
def quick_sort_median(arr):
if len(arr) <= 1:
return arr
first, middle, last = arr[0], arr[len(arr)//2], arr[-1]
pivot = sorted([first, middle, last])[1]
arr.remove(pivot)
left = [x for x in arr if x <= pivot]
right = [x for x in arr if x > pivot]
return quick_sort_median(left) + [pivot] + quick_sort_median(right)
# Example usage
fish_weights = [15, 3, 9, 7, 2, 10]
print(quick_sort_median(fish_weights))
Time and Space Complexity – How Efficient is Quick Sort?
Time and Space Complexity Explained Simply
Time Complexity:
Average Case (O(n log n)): Quick Sort performs best when the pivot is well-chosen, dividing the list into two roughly equal halves. In this case, the algorithm runs in O(n log n) time.
Worst Case (O(n²)): If the pivot is poorly chosen (for example, always the smallest or largest element), Quick Sort may degrade to O(n²) time complexity.
Space Complexity:
- Space Complexity (O(log n)): Quick Sort is an in-place algorithm, meaning it sorts the list within the original array without needing extra space. However, the recursion depth can be as large as O(log n) in the average case.
Drawbacks and Real-World Applications
Drawbacks of Quick Sort
Worst-Case Time Complexity: Quick Sort can degrade to O(n²) if the pivot is consistently poorly chosen, such as when the list is already sorted.
Recursive Nature: Quick Sort’s recursion depth can become problematic for very large datasets if not optimized with techniques like tail recursion.
Real-World Applications of Quick Sort
Efficient Sorting of Large Datasets: Quick Sort is ideal for large datasets, where its average-case O(n log n) time complexity provides excellent performance.
In-Place Sorting: Since Quick Sort doesn’t require additional memory beyond the original array, it’s efficient in memory-constrained environments.
Performance-Critical Systems: Quick Sort is often the go-to choice in performance-critical systems, such as real-time applications and embedded systems, where speed is crucial.
Challenge Time – Test Your Skills!
Fun Challenges with Quick Sort
Challenge: Sorting Shark Sizes
A group of sharks is being measured for a study, and their sizes need to be sorted quickly. Use Quick Sort to arrange their sizes:[18, 5, 23, 9, 12, 15]
.Challenge: Partition a School of Fish
You’re tasked with partitioning a school of fish into two groups: smaller fish and larger fish. The pivot fish weighs8
. Use Quick Sort’s partitioning method to divide the school into two groups:[4, 9, 2, 8, 12, 7]
.Challenge: Find the Fastest Swimmers
Out of a group of swimmers, you need to find the top 3 fastest ones. Use Quick Sort to sort their swim times and select the top 3:[12.5, 9.2, 10.1, 11.4, 13.3, 8.9]
.
Think you’ve got Quick Sort down? Try the challenges and share your solutions!
The Shark’s Quick Precision in Sorting
With Quick Sort’s smart use of pivots and partitioning, you now know how to sort efficiently and handle large datasets with precision and speed—just like a shark targeting its prey in the ocean. You’ve mastered the basics, explored optimizations, and solved some fun challenges using Quick Sort.
Ready for the next challenge? Subscribe to stay updated on Heap Sort!
Subscribe to my newsletter
Read articles from gayatri kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by