The Lobster’s Hierarchy
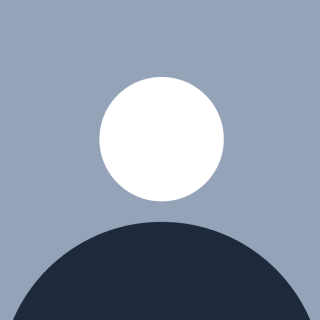
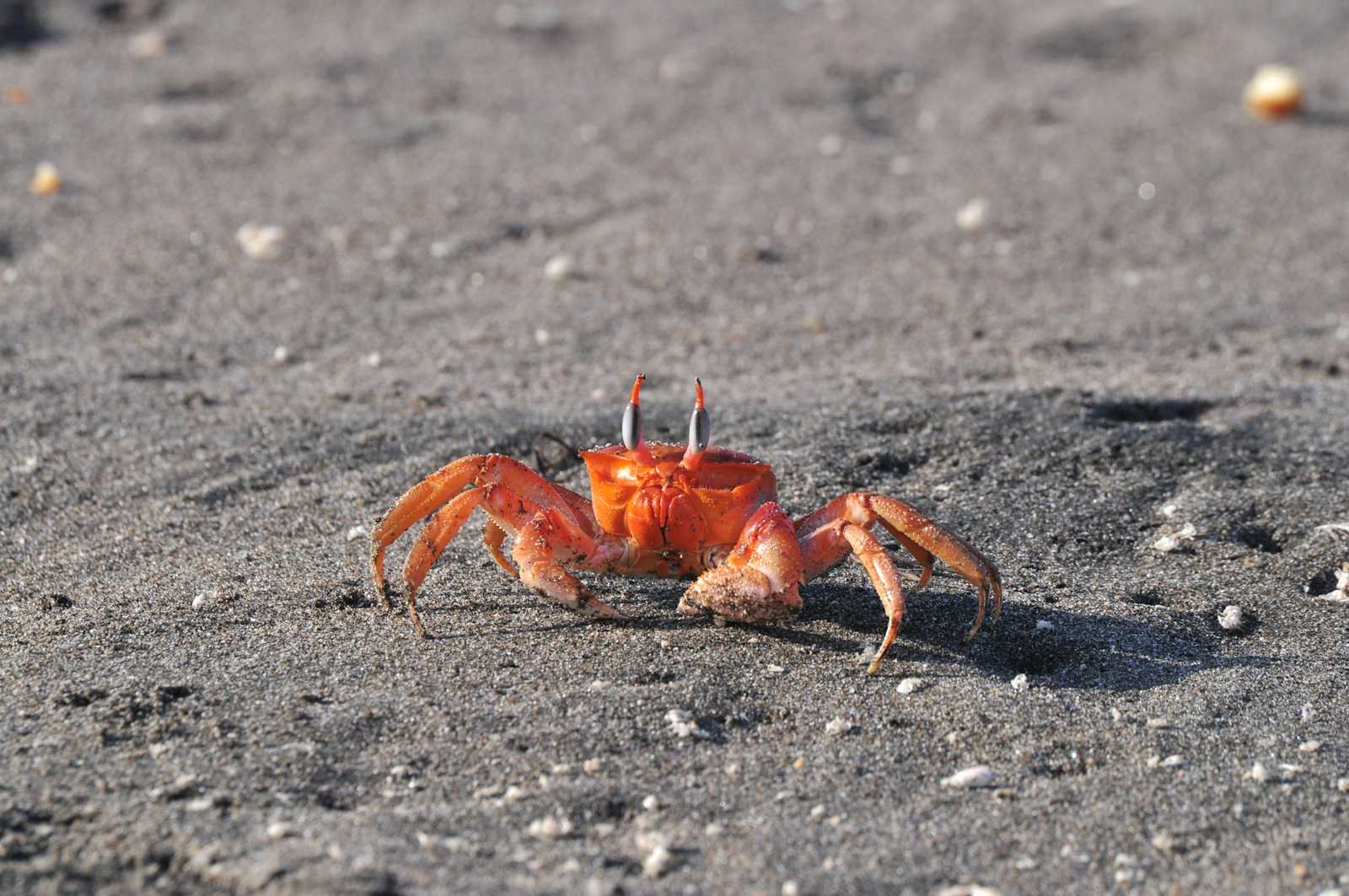
Imagine a lobster navigating its way through the complex environment of the ocean floor. The lobster efficiently manages its movement and makes decisions based on structure and hierarchy. This is exactly how Heap Sort works—by organizing data into a structured tree, Heap Sort allows you to efficiently extract the largest (or smallest) element, much like the lobster methodically chooses its path.
In this article, we’ll explore how Heap Sort uses a binary heap structure to sort data, optimize it for different use cases, and solve some fun challenges. The lobster will guide you through this methodical sorting process!
Simple Heap Sort – The Lobster’s Structured Approach
Heap Sort 101 – Building and Sorting with a Binary Heap
Heap Sort works by building a binary heap from the input data and then repeatedly extracting the largest element (in the case of a max heap) or the smallest element (in a min heap) to sort the data. The heap structure ensures that the largest (or smallest) element is always at the root, making it easy to extract and sort.
Step-by-Step Example with Code
Let’s take a list of lobster sizes: [20, 10, 15, 30, 5]
.
Build the Max Heap:
Start by converting the list into a max heap so that the largest element is at the root.
After heapifying the list, it becomes:[30, 20, 15, 10, 5]
.Extract the Maximum:
The largest element (30
) is swapped with the last element in the heap and removed. The heap is then adjusted to restore the max heap property.
List becomes:[20, 10, 15, 5]
(sorted:[30]
).Repeat the Process:
Continue extracting the maximum element and adjusting the heap. After extracting20
, the heap becomes[15, 10, 5]
(sorted:[20, 30]
).Final Sorted List:
After repeating this process, the final sorted list is:
[5, 10, 15, 20, 30]
.
Code Snippets for Simple Heap Sort
def heapify(arr, n, i):
largest = i
left = 2 * i + 1
right = 2 * i + 2
if left < n and arr[left] > arr[largest]:
largest = left
if right < n and arr[right] > arr[largest]:
largest = right
if largest != i:
arr[i], arr[largest] = arr[largest], arr[i]
heapify(arr, n, largest)
def heap_sort(arr):
n = len(arr)
for i in range(n // 2 - 1, -1, -1):
heapify(arr, n, i)
for i in range(n - 1, 0, -1):
arr[i], arr[0] = arr[0], arr[i]
heapify(arr, i, 0)
return arr
# Example usage
lobster_sizes = [20, 10, 15, 30, 5]
print(heap_sort(lobster_sizes))
function heapify(arr, n, i) {
let largest = i;
let left = 2 * i + 1;
let right = 2 * i + 2;
if (left < n && arr[left] > arr[largest]) {
largest = left;
}
if (right < n && arr[right] > arr[largest]) {
largest = right;
}
if (largest !== i) {
[arr[i], arr[largest]] = [arr[largest], arr[i]];
heapify(arr, n, largest);
}
}
function heapSort(arr) {
let n = arr.length;
for (let i = Math.floor(n / 2) - 1; i >= 0; i--) {
heapify(arr, n, i);
}
for (let i = n - 1; i > 0; i--) {
[arr[0], arr[i]] = [arr[i], arr[0]];
heapify(arr, i, 0);
}
return arr;
}
// Example usage
let lobsterSizes = [20, 10, 15, 30, 5];
console.log(heapSort(lobsterSizes));
#include <iostream>
using namespace std;
void heapify(int arr[], int n, int i) {
int largest = i;
int left = 2 * i + 1;
int right = 2 * i + 2;
if (left < n && arr[left] > arr[largest])
largest = left;
if (right < n && arr[right] > arr[largest])
largest = right;
if (largest != i) {
swap(arr[i], arr[largest]);
heapify(arr, n, largest);
}
}
void heapSort(int arr[], int n) {
for (int i = n / 2 - 1; i >= 0; i--)
heapify(arr, n, i);
for (int i = n - 1; i > 0; i--) {
swap(arr[0], arr[i]);
heapify(arr, i, 0);
}
}
int main() {
int lobsterSizes[] = {20, 10, 15, 30, 5};
int n = sizeof(lobsterSizes) / sizeof(lobsterSizes[0]);
heapSort(lobsterSizes, n);
for (int i = 0; i < n; i++)
cout << lobsterSizes[i] << " ";
return 0;
}
Optimizing Heap Sort – Fine-Tuning the Lobster’s Strategy
Making Heap Sort Even More Efficient
Heap Sort is already efficient with O(n log n) time complexity, but there are a few ways we can optimize it:
Heapify in Bottom-Up Order:
Instead of starting at the top, starting the heapify process from the leaves and working upwards can reduce the number of comparisons and swaps, making it faster.In-Place Sorting:
Heap Sort is an in-place sorting algorithm, which means it doesn’t require extra space for sorting beyond the input array, making it more memory-efficient.
Python Code for Optimized Heap Sort
def heapify_optimized(arr, n, i):
largest = i
left = 2 * i + 1
right = 2 * i + 2
if left < n and arr[left] > arr[largest]:
largest = left
if right < n and arr[right] > arr[largest]:
largest = right
if largest != i:
arr[i], arr[largest] = arr[largest], arr[i]
heapify_optimized(arr, n, largest)
def heap_sort_optimized(arr):
n = len(arr)
for i in range(n // 2 - 1, -1, -1):
heapify_optimized(arr, n, i)
for i in range(n - 1, 0, -1):
arr[i], arr[0] = arr[0], arr[i]
heapify_optimized(arr, i, 0)
return arr
Time and Space Complexity – How Efficient is Heap Sort?
Time Complexity:
Best Case (O(n log n)): Heap Sort consistently performs well regardless of the input order, making O(n log n) comparisons and swaps in all cases.
Worst Case (O(n log n)): Even in the worst-case scenario, Heap Sort’s time complexity remains O(n log n) due to the structured nature of the heap.
Space Complexity:
- Space Complexity (O(1)): Heap Sort is an in-place sorting algorithm, meaning it requires a constant amount of extra space beyond the input array, making it memory-efficient.
Drawbacks and Real-World Applications
Drawbacks of Heap Sort
Not a Stable Sort: Heap Sort is not stable, meaning it doesn’t preserve the relative order of equal elements.
Complex Heapify Operation: The heapify operation can be complex to implement, making it harder to optimize for certain use cases compared to other sorting algorithms.
Real-World Applications of Heap Sort
Priority Queues: Heap Sort is ideal for implementing priority queues, where elements are served based on their priority (e.g., in scheduling systems). Since heaps maintain the largest (or smallest) element at the root, it’s easy to retrieve the highest-priority item efficiently.
Memory-Constrained Systems: Because Heap Sort is an in-place algorithm and doesn't require additional space beyond the input array, it’s a great choice for systems with limited memory.
Streamlining Multi-Tasking Operations: Heap Sort can be used to optimize task scheduling systems where tasks are prioritized based on importance or deadlines.
Challenge Time – Test Your Skills!
Challenge: Sorting Lobster Sizes
A marine research team is cataloging lobsters based on their sizes, and they need to quickly sort the data. Use Heap Sort to arrange the lobster sizes:[25, 14, 8, 30, 18, 5]
.Challenge: Implement a Priority Queue
You’ve been asked to design a basic priority queue using Heap Sort. The tasks and their respective priorities are:[("Task A", 3), ("Task B", 1), ("Task C", 2)]
. Sort the tasks by priority.Challenge: Find the Largest Lobster
Out of a group of lobsters, find the largest one using Heap Sort:[22, 11, 19, 8, 30, 15]
. Extract the largest lobster and sort the rest.
Think you’ve got Heap Sort down? Try the challenges and share your solutions!
The Lobster’s Structured Approach to Sorting
Lobsters are efficient creatures, navigating complex environments with precision, just as Heap Sort builds and manipulates a structured heap to sort data. You’ve now mastered Heap Sort and learned how to optimize it for different use cases. Stay tuned for our next sorting algorithm.
Ready for the next challenge? Subscribe to stay updated on Radix Sort!
Subscribe to my newsletter
Read articles from gayatri kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by