The Octopus’s Tentacles
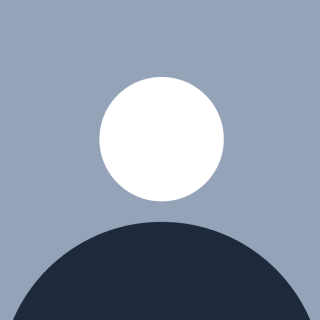
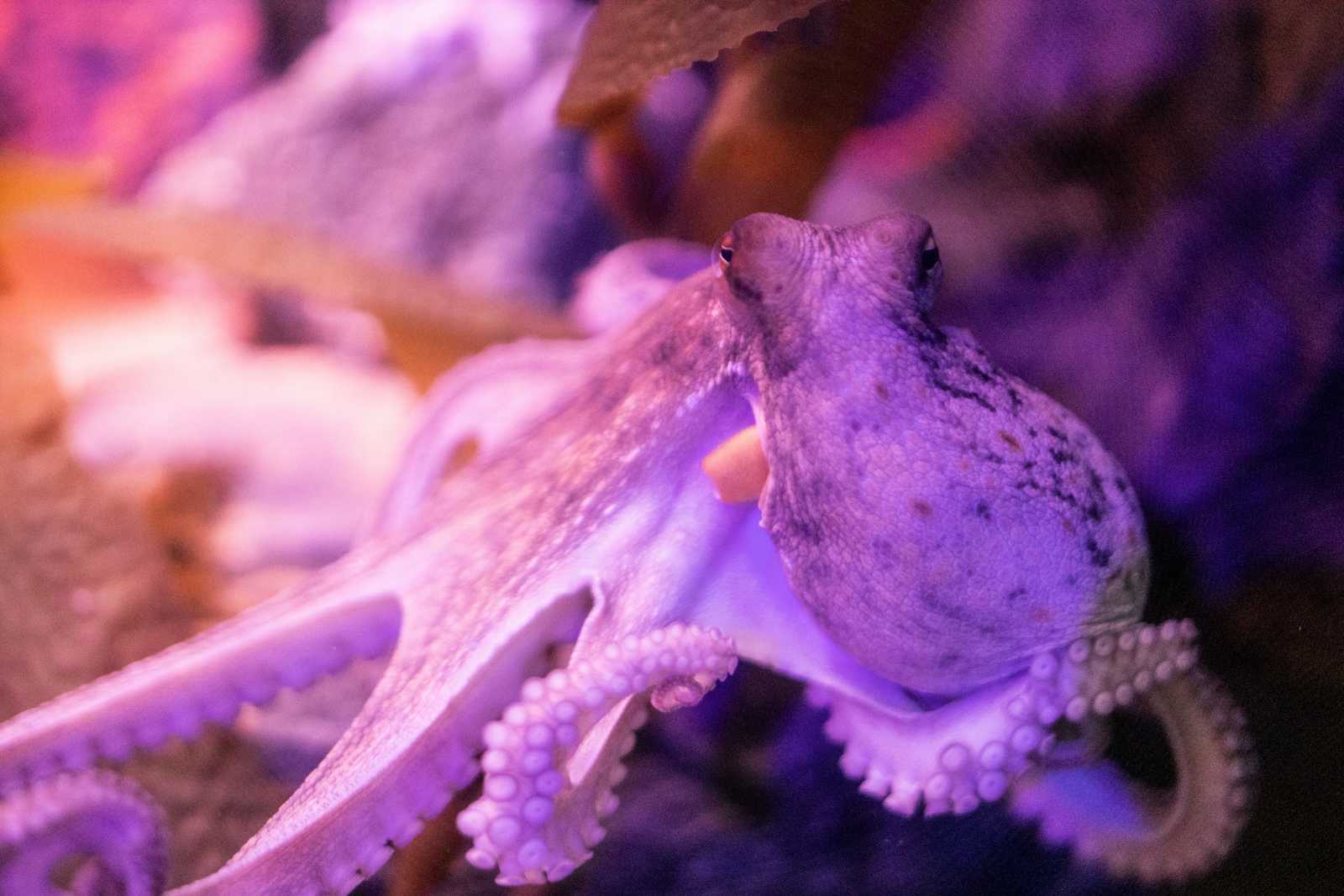
Picture an octopus efficiently using its tentacles to manage multiple tasks at the same time—each tentacle works on a part of the task, eventually bringing everything together. This is similar to how Radix Sort operates: by sorting elements one digit at a time, starting from the least significant digit and moving up to the most significant. This non-comparative sorting algorithm is highly efficient for sorting integers, and it uses the structure of the numbers to sort rather than comparing them directly.
In this article, we’ll explore how Radix Sort works step by step, understand its advantages, and dive into some fun challenges. Let the octopus guide you through this clever sorting algorithm!
Simple Radix Sort – The Octopus’s Tentacles at Work
Radix Sort 101 – Sorting Digit by Digit
Radix Sort works by sorting elements based on each digit or place value, starting with the least significant digit (LSD) and progressing to the most significant digit (MSD). It uses a stable sorting algorithm, like counting sort, to handle each digit.
Step-by-Step Example with Code
Let’s take a list of octopus arm lengths in millimeters: [170, 45, 75, 90, 802, 24]
.
Sort by the Least Significant Digit (Ones Place):
Starting with the least significant digit (the ones place), we sort the numbers.
After sorting by the ones place:
List becomes:[170, 90, 802, 24, 45, 75]
Sort by the Tens Digit:
Now, sort the numbers based on the tens place.
After sorting by the tens place:
List becomes:[802, 24, 45, 75, 170, 90]
Sort by the Hundreds Digit:
Finally, sort by the hundreds place.
After sorting by the hundreds place:
List becomes:[24, 45, 75, 90, 170, 802]
Final Sorted List:
After sorting by all digits, the final sorted list is:
[24, 45, 75, 90, 170, 802]
.
Code Snippets for Simple Radix Sort
def counting_sort(arr, exp):
n = len(arr)
output = [0] * n
count = [0] * 10
for i in range(n):
index = arr[i] // exp
count[index % 10] += 1
for i in range(1, 10):
count[i] += count[i - 1]
i = n - 1
while i >= 0:
index = arr[i] // exp
output[count[index % 10] - 1] = arr[i]
count[index % 10] -= 1
i -= 1
for i in range(n):
arr[i] = output[i]
def radix_sort(arr):
max_num = max(arr)
exp = 1
while max_num // exp > 0:
counting_sort(arr, exp)
exp *= 10
return arr
# Example usage
octopus_lengths = [170, 45, 75, 90, 802, 24]
print(radix_sort(octopus_lengths))
function countingSort(arr, exp) {
let n = arr.length;
let output = new Array(n).fill(0);
let count = new Array(10).fill(0);
for (let i = 0; i < n; i++) {
let index = Math.floor(arr[i] / exp);
count[(index % 10)]++;
}
for (let i = 1; i < 10; i++) {
count[i] += count[i - 1];
}
for (let i = n - 1; i >= 0; i--) {
let index = Math.floor(arr[i] / exp);
output[count[(index % 10)] - 1] = arr[i];
count[(index % 10)]--;
}
for (let i = 0; i < n; i++) {
arr[i] = output[i];
}
}
function radixSort(arr) {
let max = Math.max(...arr);
for (let exp = 1; Math.floor(max / exp) > 0; exp *= 10) {
countingSort(arr, exp);
}
return arr;
}
// Example usage
let octopusLengths = [170, 45, 75, 90, 802, 24];
console.log(radixSort(octopusLengths));
#include <iostream>
using namespace std;
void countingSort(int arr[], int n, int exp) {
int output[n];
int count[10] = {0};
for (int i = 0; i < n; i++)
count[(arr[i] / exp) % 10]++;
for (int i = 1; i < 10; i++)
count[i] += count[i - 1];
for (int i = n - 1; i >= 0; i--) {
output[count[(arr[i] / exp) % 10] - 1] = arr[i];
count[(arr[i] / exp) % 10]--;
}
for (int i = 0; i < n; i++)
arr[i] = output[i];
}
void radixSort(int arr[], int n) {
int max = arr[0];
for (int i = 1; i < n; i++)
if (arr[i] > max)
max = arr[i];
for (int exp = 1; max / exp > 0; exp *= 10)
countingSort(arr, n, exp);
}
int main() {
int octopusLengths[] = {170, 45, 75, 90, 802, 24};
int n = sizeof(octopusLengths) / sizeof(octopusLengths[0]);
radixSort(octopusLengths, n);
for (int i = 0; i < n; i++)
cout << octopusLengths[i] << " ";
return 0;
}
Optimizing Radix Sort – The Octopus’s Efficiency
Radix Sort is already a highly efficient algorithm for sorting integers, but there are ways to optimize it:
Use a Stable Sorting Algorithm:
For each digit sorting step, it’s important to use a stable sorting algorithm like counting sort, which preserves the order of elements with the same digit value. This ensures that the relative order of equal elements is maintained across different digits.Base Optimization:
Instead of always using base 10 (decimal), you can optimize Radix Sort by using different bases, such as base 2 or base 16, depending on the data structure and system architecture.
Time and Space Complexity – Understanding Radix Sort’s Efficiency
Time Complexity:
Best, Worst, and Average Case (O(d × (n + k))): Radix Sort consistently runs in O(d × (n + k)) time, where:
n
is the number of elements,k
is the range of the input values (e.g., base 10 for decimal numbers),and
d
is the number of digits in the largest number. This makes Radix Sort highly efficient for integers and large datasets.
Space Complexity:
- Space Complexity (O(n + k)): Radix Sort requires additional space for the output array and the counting array used by counting sort, resulting in O(n + k) space complexity.
Drawbacks and Real-World Applications
Drawbacks of Radix Sort
Restricted to Specific Data Types: Radix Sort is primarily limited to sorting integers or fixed-length strings. It’s not as versatile as comparison-based sorts like Quick Sort or Merge Sort, which can handle more complex data types.
Space Complexity: While efficient in terms of time, Radix Sort requires additional memory for the counting array and output array, which can be a limitation in memory-constrained systems.
Real-World Applications of Radix Sort
Sorting Large Integers: Radix Sort is highly efficient for sorting large datasets of integers, making it useful in applications like sorting ID numbers or transaction data.
Fixed-Length String Sorting: Radix Sort can be adapted to sort fixed-length strings, such as sorting a list of words or codes where each item has the same number of characters. This is useful in applications like sorting postal codes, DNA sequences, or license plates.
Sorting Large Integers: Radix Sort is highly efficient for sorting large datasets of integers, such as telephone numbers, social security numbers, or transaction IDs, where comparison-based sorts might be less efficient.
Processing Log Files: In systems where log files generate large numbers of timestamped entries, Radix Sort can efficiently sort these entries by timestamp when they are represented as integers.
Challenge Time – Test Your Skills!
Challenge: Sorting Octopus Tentacle Lengths
You have a list of octopus tentacle lengths in millimeters:
[123, 45, 67, 890, 12, 456]
. Use Radix Sort to arrange them in ascending order.Challenge: Organizing Barcode Numbers
A warehouse needs to sort a large number of products by their barcode numbers (assuming they are numerical and have the same length). Given the barcodes
[1023, 5123, 3012, 4123, 2034, 1123]
, use Radix Sort to sort them efficiently.Challenge: Sorting DNA Sequences
Biologists are analyzing DNA sequences represented by numerical codes of equal length. Use Radix Sort to sort the sequences:
[3214, 2134, 1324, 4231, 2413, 3142]
.
Think you've mastered Radix Sort? Try the challenges and share your solutions!
The Octopus’s Multitasking Mastery in Sorting
Just like an octopus using its multiple tentacles to handle different tasks simultaneously, Radix Sort efficiently sorts data by processing each digit one at a time. You've learned how Radix Sort works, its advantages, and its applications in sorting large datasets of integers or fixed-length strings. By mastering Radix Sort, you've added a powerful non-comparative sorting algorithm to your toolkit. Stay tuned for our next sorting adventure.
Ready for the next algorithm? Subscribe to stay updated on Bucket Sort!
Subscribe to my newsletter
Read articles from gayatri kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by