Mastering Error Handling in Shell Scripting: Essential Techniques for Robust Automation ๐

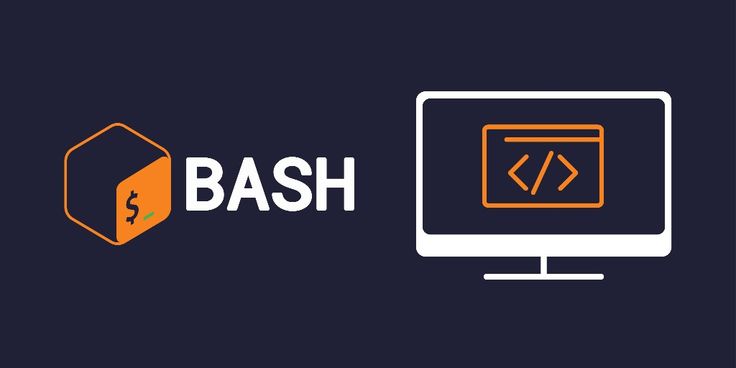
๐ Day 11 Task: Error Handling in Shell Scripting ๐จ
In today's DevOps journey, we focus on error handling in shell scriptingโa crucial skill to make sure our scripts are robust, reliable, and ready for the real world! ๐ Whether you're automating backups, managing services, or processing logs, handling errors effectively ensures your scripts can recover from failures gracefully.
Let's dive into the essentials of error handling and explore how you can use different methods to keep your scripts in check! ๐จโ๐ป๐ฉโ๐ป
๐งโ๐ซ Learning Objectives:
Understand exit status codes ๐ข๐ด
Use
if
statements to handle errorsImplement
trap
to perform cleanup when errors happen unexpectedlyRedirect errors to files for better debugging ๐
Create custom error messages to make troubleshooting easier
๐ Topics Covered:
1. Exit Status โ
Every command returns an exit status in Linux. If a command runs successfully, it returns 0; if it fails, it returns a non-zero status. You can check these exit statuses to control the flow of your script.
2. if
Statements for Error Handling โ๏ธ
Use if
statements to check the success or failure of commands. Based on this, you can perform error handling, take corrective action, or print meaningful error messages.
3. trap
for Cleanup ๐งน
When something unexpected happens, you don't want your system left in an unclean state. The trap
command is used to catch signals and perform cleanup tasks like removing temporary files or closing resources.
4. Redirecting Errors ๐
Sometimes, you want to redirect error messages to a file (for logging or troubleshooting) or even discard them if they're not critical. You can do this using redirection.
5. Custom Error Messages ๐
Improve user experience by creating helpful error messages. Instead of just "Error," tell users what went wrong and how they can possibly fix it.
๐ง Tasks (with Examples!):
๐ Task 1: Checking Exit Status
Write a script that tries to create a directory and checks if it succeeded. If the directory creation fails, print an error message.
Example:
#!/bin/bash
mkdir /tmp/mytestdir
if [ $? -ne 0 ]; then
echo "โ Failed to create directory /tmp/mytestdir"
else
echo "โ
Directory /tmp/mytestdir created successfully!"
fi
This script creates a directory at /tmp/mytestdir
and checks if the exit status is 0 (success). If it's not, it prints an error message. Simple, right? ๐
๐ Task 2: Using if
Statements for Multiple Commands
Extend the previous task by adding more commands, like creating a file inside the directory. Use if
statements to check the success of each command.
Example:
#!/bin/bash
mkdir /tmp/mytestdir
if [ $? -ne 0 ]; then
echo "โ Failed to create directory /tmp/mytestdir"
else
touch /tmp/mytestdir/myfile.txt
if [ $? -ne 0 ]; then
echo "โ Failed to create file /tmp/mytestdir/myfile.txt"
else
echo "โ
File /tmp/mytestdir/myfile.txt created successfully!"
fi
fi
Now the script checks the success of each step before proceeding, making it more robust.
๐ Task 3: Using trap
for Cleanup
Use the trap
command to automatically clean up a temporary file when the script exits, whether successfully or due to an error.
Example:
#!/bin/bash
tempfile=$(mktemp) # Creates a temporary file
trap "rm -f $tempfile" EXIT # Deletes the temporary file when the script exits
echo "This is a temporary file." > $tempfile
cat $tempfile
# Simulating an error
exit 1
Here, if the script exits unexpectedly (like in the case of an error), the trap
command will automatically delete the temporary file. No more leftover files! ๐งน
๐ Task 4: Redirecting Errors
Redirect error messages to a file instead of cluttering up your terminal.
Example:
#!/bin/bash
cat non_existent_file.txt 2> error.log
This script tries to read a non-existent file, but instead of printing the error message on the terminal, it redirects the error to error.log
.
๐ Task 5: Custom Error Messages
Modify the scripts to include custom error messages. These provide more context and can guide users to troubleshoot the issue.
Example:
#!/bin/bash
mkdir /tmp/mydir
if [ $? -ne 0 ]; then
echo "โ Error: Could not create /tmp/mydir. Please check if you have the right permissions."
fi
Custom error messages make troubleshooting easier by giving users specific information about what went wrong and how to possibly fix it.
๐ Key Takeaways:
Always check the exit status of critical commands in your scripts.
Use
if
statements to handle errors and perform conditional operations.Employ
trap
to handle unexpected exits and clean up resources.Redirect error output to logs for debugging.
Create clear and informative error messages to make life easier for users!
By following these practices, you'll make your scripts more resilient and reliable, preparing you for more advanced DevOps automation tasks! ๐ช
๐ก Conclusion:
Error handling is a crucial part of any script, ensuring that your automation flows smoothly even when things go wrong. Understanding these techniques will help you create scripts that can handle the real-world complexities of DevOps and system administration. ๐
Subscribe to my newsletter
Read articles from Saksham Kamble directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
