10 Best AI Tools for Ruby on Rails 8 Development in 2024

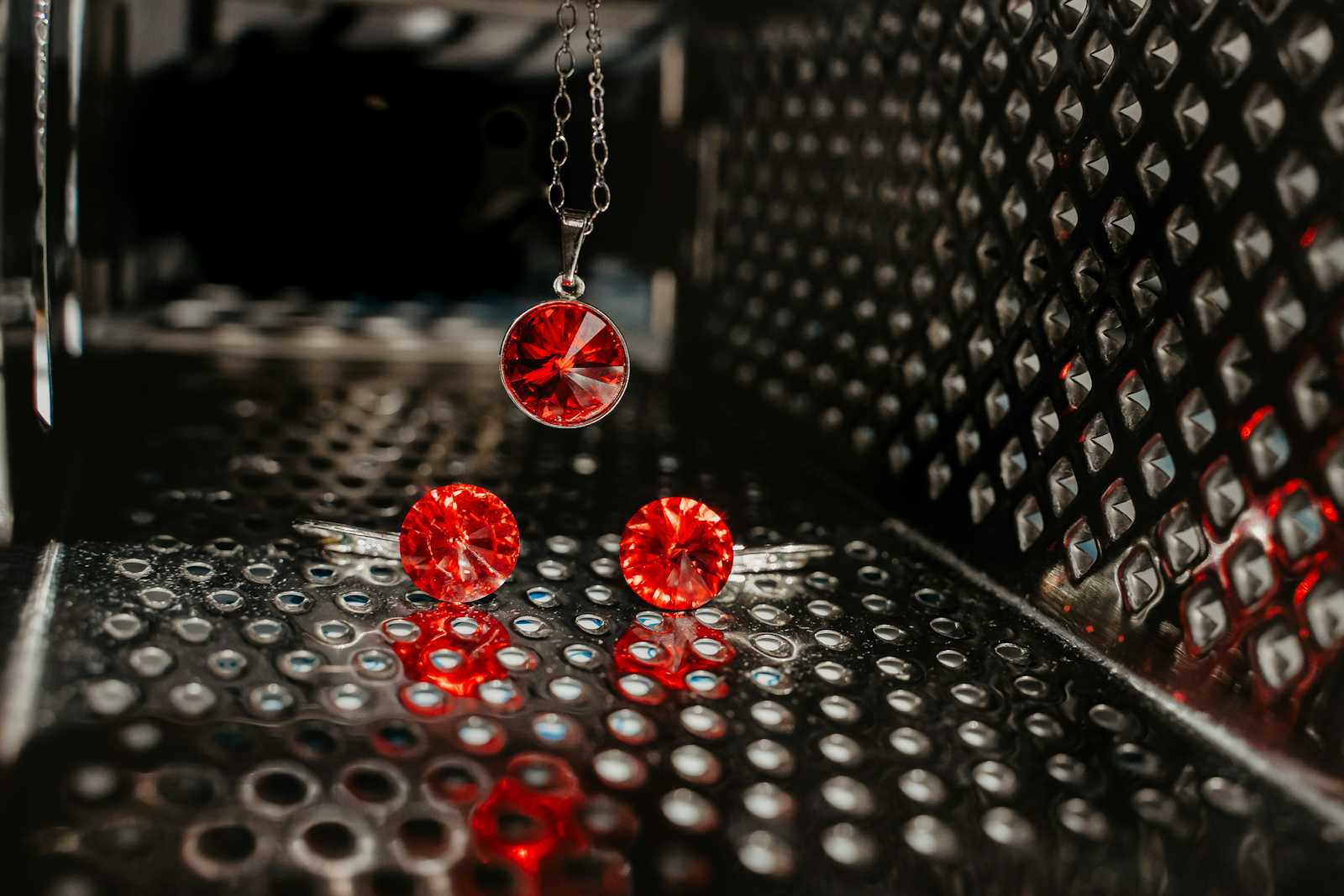
Artificial Intelligence (AI) has reshaped how developers approach software development, offering automation, predictive capabilities, and efficiency boosts.
For Ruby on Rails 8, which already emphasizes developer productivity and simplicity, AI tools can unlock even more potential.
In this post, we’ll explore the top 10 AI tools that can supercharge your Ruby on Rails 8 development experience.
1. GitHub Copilot
GitHub Copilot, developed by GitHub in collaboration with OpenAI, is one of the most powerful AI-driven code completion tools available. It integrates directly into your favorite IDEs like VS Code or JetBrains, offering intelligent code suggestions as you type.
Features:
Context-aware code suggestions
Auto-completes entire blocks of code
Works with multiple programming languages, including Ruby
Example:
# Creating a new Rails model with validations
class User < ApplicationRecord
validates :name, presence: true
validates :email, presence: true, uniqueness: true
end
With GitHub Copilot, the validation lines would be auto-suggested as you define the model, saving time on repetitive code.
2. Cursor AI
Cursor AI is a code-focused AI tool designed to assist developers by offering real-time code reviews and suggestions. It not only improves your code quality but also helps refactor existing code for better performance.
Features:
Real-time code analysis and suggestions
Identifies code inefficiencies
Refactoring assistance for performance improvement
Example:
# Inefficient code
@users = User.all
@users.each do |user|
user.update_attribute(:active, true)
end
# Refactored code using Cursor AI suggestion
User.update_all(active: true)
Cursor AI would suggest refactoring this loop to a more efficient method, reducing database calls and improving performance.
3. ChatGPT
ChatGPT by OpenAI is a powerful conversational AI that can be an invaluable assistant when debugging, researching, or brainstorming solutions.
Integrated with Ruby on Rails, it can help explain complex code snippets, suggest improvements, and provide solutions to issues.
Features:
Debugging assistance through conversation
Code generation based on natural language prompts
Offers explanations of Rails-specific functionalities
Example:
Ask ChatGPT: "How can I optimize a slow database query in Rails?"
ChatGPT would suggest using tools like the includes
method to minimize N+1 query issues.
# Before optimization
@posts = Post.all
@posts.each do |post|
puts post.comments.count
end
# After ChatGPT suggestion
@posts = Post.includes(:comments).all
@posts.each do |post|
puts post.comments.size
end
4. Claude AI
Claude AI, developed by Anthropic, offers a thoughtful and comprehensive approach to AI-powered development.
Claude’s design focuses on offering safe and reliable AI suggestions for developers, making it useful for refactoring code or coming up with scalable solutions.
Features:
Comprehensive analysis of code quality
Offers safe suggestions for refactoring
Helps scale application architecture
Example:
Claude might suggest breaking down a monolithic controller into smaller, more manageable services to follow the Single Responsibility Principle (SRP).
# Before refactor
class UsersController < ApplicationController
def index
@users = User.all
end
def send_newsletter
NewsletterService.new.deliver_to(@users)
end
end
# After Claude AI suggestion
class UsersController < ApplicationController
def index
@users = User.all
end
end
class NewsletterController < ApplicationController
def send_newsletter
NewsletterService.new.deliver_to(User.all)
end
end
5. Tabnine
Tabnine is another AI code assistant that offers lightning-fast code completions for Ruby on Rails development.
With a focus on both speed and accuracy, it integrates seamlessly with various editors, providing instant suggestions for both small and large projects.
Features:
Offers AI-powered auto-completion
Works offline for privacy-conscious teams
Provides context-aware suggestions
Example:
# Define a method in a Rails service class
class EmailService
def send_welcome_email(user)
# Tabnine suggests this method's implementation based on project context
WelcomeMailer.with(user: user).deliver_later
end
end
6. CodeT5
CodeT5, developed by Salesforce AI Research, is an open-source transformer model for code understanding and generation. Its robust capabilities allow developers to use it for various code-related tasks, such as summarization, code generation, and bug fixing.
Features:
Code summarization for better readability
Auto-generates boilerplate code
Suggests solutions for common bugs
Example:
# Summarizing a complex method using CodeT5
def complex_method(a, b)
result = (a * b) + (a / b)
return result if a > 10
a + b
end
CodeT5 can generate a concise summary, making it easier for developers to understand what the method does at a glance.
7. OpenAI Codex
OpenAI Codex, the underlying model for GitHub Copilot, can also be used separately to interact directly via an API.
This can be useful for building custom integrations or automating complex workflows in a Ruby on Rails environment.
Features:
Code generation via API
Custom integration possibilities for specialized tasks
Supports multiple programming languages, including Ruby
Example:
With OpenAI Codex, you can write a script that automates CRUD operations in Rails:
# Automatically generate CRUD methods for a resource
codex.generate_crud('Product')
8. Sourcery
Sourcery is a specialized AI tool that focuses on improving code quality by suggesting cleaner, more readable code. It performs static analysis on your Ruby codebase and suggests refactoring options to align with best practices.
Features:
Code refactoring for better readability
Enforces code quality standards
Works seamlessly with Ruby
Example:
# Before Sourcery
def total_price(items)
sum = 0
items.each do |item|
sum += item.price
end
sum
end
# After Sourcery refactoring
def total_price(items)
items.sum(&:price)
end
9. Codiga
Codiga is an AI-driven code analysis tool that integrates with your CI/CD pipeline, ensuring that your code is error-free before merging. It analyzes your Rails code in real-time and provides actionable feedback on code quality, security vulnerabilities, and performance issues.
Features:
Real-time code quality analysis
Security and performance checks
Integrates with CI/CD tools like Jenkins and GitLab
Example:
Codiga could flag security vulnerabilities in a method like this:
# Codiga suggests using parameterized queries to avoid SQL injection
User.where("email = ?", params[:email])
10. DeepCode
DeepCode is an AI tool that focuses on identifying security vulnerabilities in your codebase by analyzing it in real-time. It's particularly useful for Rails developers dealing with sensitive data or needing to ensure high levels of security.
Features:
AI-driven code scanning for vulnerabilities
Real-time feedback on potential security risks
Works with GitHub and GitLab
Has a VSCode extension
Example:
DeepCode would highlight potential security risks in the following code:
# DeepCode flags the raw query as a security risk
User.find_by_sql("SELECT * FROM users WHERE email = '#{params[:email]}'")
Instead, DeepCode would suggest using ActiveRecord’s parameterized queries to prevent SQL injection:
User.where("email = ?", params[:email])
Bonus: Codeium AI
Codeium AI is an AI-driven code completion and code quality tool designed to enhance your coding workflow.
Unlike traditional code completion tools, Codeium AI focuses on generating smart, context-aware code suggestions while also offering insights into potential improvements in your code structure and logic. It's particularly useful for improving productivity and minimizing errors in Ruby on Rails development.
Features:
Advanced code suggestions and auto-completions
Real-time code quality analysis
Works seamlessly with Ruby on Rails projects
Example:
# Writing a controller action with Codium AI
class ProductsController < ApplicationController
def create
@product = Product.new(product_params)
if @product.save
redirect_to @product, notice: 'Product was successfully created.'
else
render :new
end
end
end
Conclusion
In 2024, AI tools like GitHub Copilot, ChatGPT, Cursor AI, and Codeium AI are revolutionizing Ruby on Rails 8 development, offering enhanced productivity, optimized code quality, and improved security.
By integrating these tools into your development workflow, you can automate mundane tasks, reduce errors, and focus on building innovative, high-quality software faster than ever.
I personally use Codeium in my VSCode to get code suggestions.
Subscribe to my newsletter
Read articles from BestWeb Ventures directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

BestWeb Ventures
BestWeb Ventures
From cutting-edge web and app development, using Ruby on Rails, since 2005 to data-driven digital marketing strategies, we build, operate, and scale your MVP (Minimum Viable Product) for sustainable growth in today's competitive landscape.