Exploring Event-Driven Architecture with AWS: Key Patterns, Use Cases, and Implementations
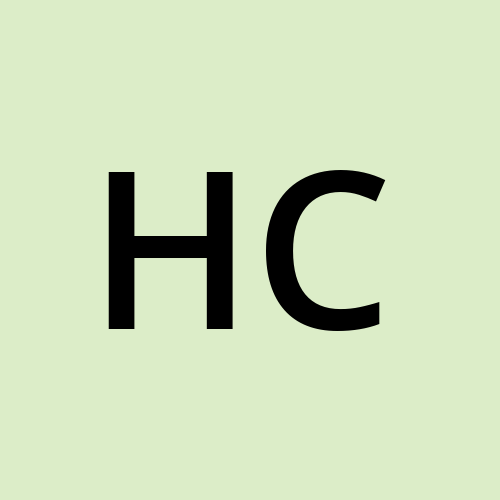
Table of contents
- Introduction
- Example Use Cases:
- Event-Driven Architecture Patterns
- 1. Publish-Subscribe (Pub/Sub)
- 2. Event Sourcing
- 3. Command Query Responsibility Segregation (CQRS)
- 4. Event Notification
- 5. Saga
- 6. Orchestration
- 7. Choreography
- 8. Event-Carried State Transfer
- 9. Event Stream Processing
- 10. Event Collaboration
- 11. Dead Letter Queue
- 12. Event Versioning
- 13. Projections
- 14. Event Replay
- Conclusion
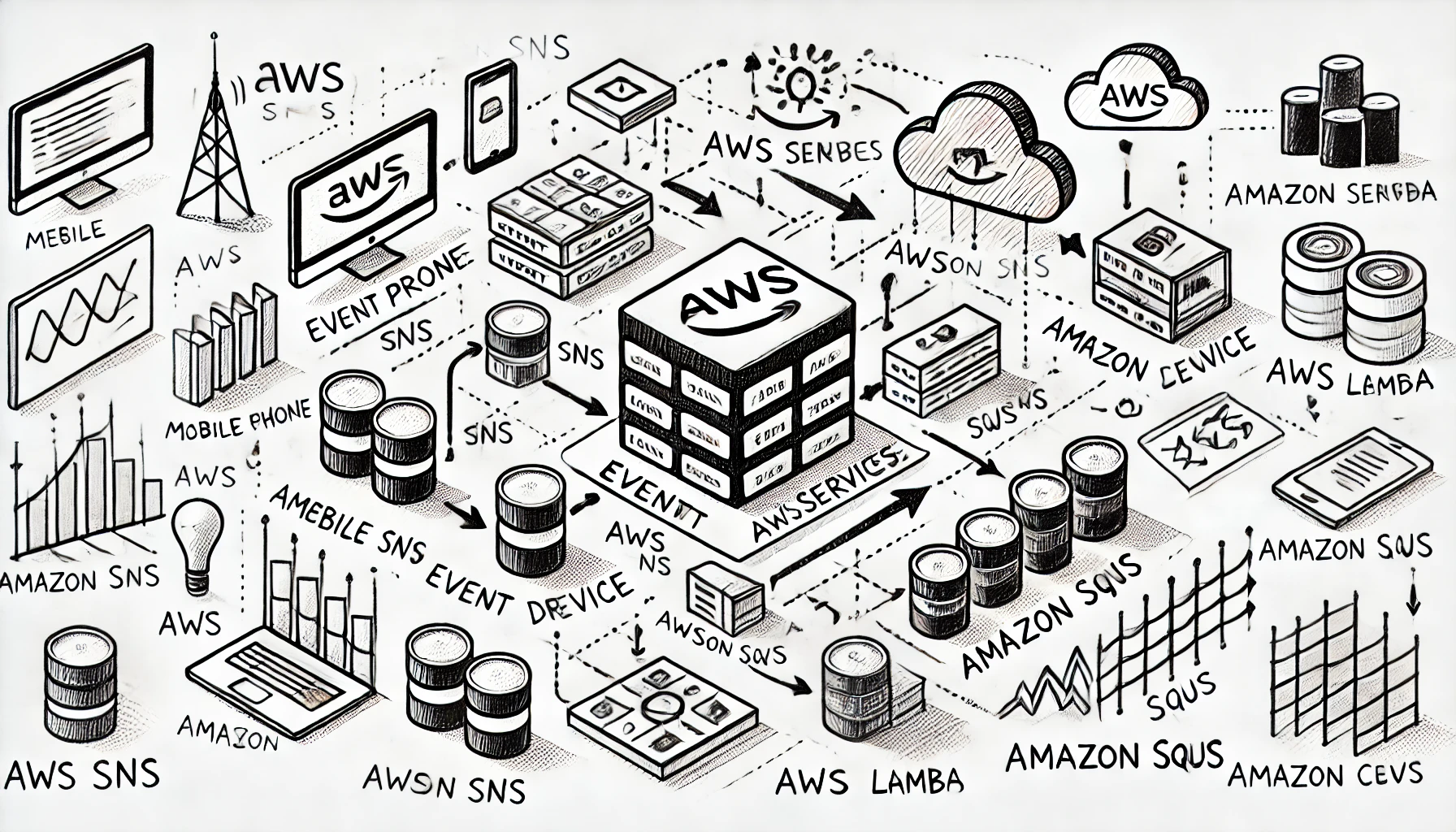
Introduction
Event-Driven Architecture (EDA) patterns are important for a variety of reasons, especially in modern distributed systems where flexibility, scalability, and real-time processing are key. Here’s why EDA patterns are needed:
Reduced Tight Coupling: EDA allows systems to decouple producers and consumers of data. Components don’t need to directly know about each other, enabling independent development, deployment, and scaling.
Improved Flexibility: Since components communicate through events, changes to one part of the system don’t necessarily break or require updates to other parts.
Easier Horizontal Scaling: Event-driven systems can scale more easily because new consumers can be added without impacting the producers. The system can handle more events by scaling individual components.
Asynchronous Processing: With EDA, systems can process events asynchronously, making it easier to handle high loads and distribute tasks across multiple services.
Timely Response to Events: EDA supports real-time data processing, enabling systems to react immediately to changes or events, such as user actions or sensor data.
Event Streams: Continuous streams of events allow for near-instantaneous processing, improving user experience and system responsiveness.
Isolation of Failures: EDA allows components to fail independently without bringing down the whole system. If an event consumer fails, the system can retry the event without affecting other consumers or producers.
Graceful Degradation: When a service is temporarily down, events can be queued and processed later, which helps systems maintain some level of functionality during failures.
Easier Addition of New Functionality: New functionality can be added by simply introducing new event consumers, without altering existing producers.
Real-time Analytics and Monitoring: Events provide a stream of data that can be monitored and analyzed in real-time, enabling dynamic insights and immediate reactions to business-critical changes.
Natural Fit for Microservices: EDA patterns are often a key enabler for microservices architecture because they allow services to communicate in a loosely coupled manner.
Autonomous Services: With EDA, each microservice can work independently and react to the events it cares about, promoting autonomy and reducing dependencies.
Correlating and Enriching Events: EDA allows systems to process and correlate multiple events, combining them into meaningful actions. Complex event processing is vital in areas like fraud detection, IoT, or monitoring systems.
Event-Triggered Operations: Resources are utilized only when necessary, as operations are triggered by events. This leads to more efficient use of computing resources compared to systems where services are continuously running or polling for changes.
Example Use Cases:
E-commerce: Reacting to user actions, like adding items to a cart, placing orders, and notifying inventory or delivery systems.
IoT: Real-time sensor data processing and actions like triggering alarms, updates, or responses based on environmental changes.
Financial Systems: Detecting and responding to real-time transactions, stock price changes, or fraud detection.
Event-driven architecture patterns provide a robust framework for building scalable, responsive, and loosely coupled systems, making them essential for modern cloud-native and distributed applications.
This guide covers key patterns in event-driven architecture, providing insights into their workings, benefits, real-world applications, and implementation considerations.
Event-Driven Architecture Patterns
1. Publish-Subscribe (Pub/Sub)
Description
A messaging pattern where senders (publishers) categorize messages into classes and send them to an intermediary message broker. Receivers (subscribers) express interest in classes and receive relevant messages.
Real-Life Example
Social media platform using Pub/Sub for real-time analytics.
AWS Services
Amazon SNS (Simple Notification Service): Acts as the message broker, where publishers send messages and subscribers receive them.
Amazon SQS (Simple Queue Service): Subscribers can be SQS queues to decouple processing.
AWS Lambda: Lambda functions can also act as subscribers to process messages in real time.
Implementation
Use SNS to publish a message when a social media post is created. Subscribers (analytics service, notification service) receive the event and react accordingly.
Benefits
Decoupling of publishers and subscribers
Scalability and flexibility
2. Event Sourcing
Description
Stores the state of a system as a sequence of events rather than just the current state.
Real-Life Example
Banking system for account management and auditing.
AWS Services
Amazon DynamoDB: Store events in DynamoDB as immutable records.
Amazon Kinesis: Stream the events for real-time processing.
AWS Lambda: To replay events and reconstruct state.
Implementation
Store every state change (deposit, withdrawal) in DynamoDB as events.
Use Kinesis to stream those events to different consumers.
Consumers replay the events via Lambda to rebuild the current account balance.
Benefits
Complete audit trail
Historical state reconstruction
3. Command Query Responsibility Segregation (CQRS)
Description
Separates read and write operations for a data store, using different models for updating and reading information.
Real-Life Example
E-commerce platform with separate models for order processing and product viewing.
AWS Services
Amazon DynamoDB: For the write model.
DynamoDB Streams: To propagate changes.
Amazon Elasticsearch Service (OpenSearch): For the read model.
Implementation
Store write operations (order creation) in DynamoDB.
Use DynamoDB Streams to trigger a Lambda function that updates a read-optimized OpenSearch index.
The read model (product catalog) is served by OpenSearch, optimized for fast querying.
Benefits
Optimized read and write performance
Scalability of read and write sides independently
4. Event Notification
Description
Services notify each other of events without passing complex state, typically containing minimal information.
Real-Life Example
E-commerce order system notifying inventory and shipping services of new orders.
AWS Services
Amazon SNS: For broadcasting notifications.
AWS Lambda: For processing events.
Implementation
Publish an OrderPlaced event via SNS.
Inventory and shipping services receive the event and act accordingly (reduce stock, prepare shipment).
Benefits
Simple and lightweight communication
Reduced coupling between services
5. Saga
Description
Manages data consistency across microservices in distributed transaction scenarios through a sequence of local transactions.
Real-Life Example
Travel booking system coordinating flight, hotel, and car rental reservations.
AWS Services
AWS Step Functions: To manage a saga with compensating transactions.
AWS Lambda: For executing individual steps in the saga.
Implementation
When an order is placed, reserve inventory, process payment, and trigger shipping in a Step Function.
If payment fails, trigger a compensating transaction to release inventory.
Benefits
Maintains data consistency in distributed systems
Handles complex workflows across services
6. Orchestration
Description
Uses a central orchestrator to manage and direct the overall process flow in a system.
Real-Life Example
Loan approval process with a central service coordinating credit checks, income verification, and risk assessment.
AWS Services
AWS Step Functions: As the orchestrator.
AWS Lambda: For processing tasks in the workflow.
Implementation
Use Step Functions to orchestrate the loan approval process, coordinating credit checks, income verification, and risk assessment.
Each step invokes a Lambda function.
Benefits
Centralized control and visibility of processes
Easier to manage complex workflows
7. Choreography
Description
Services work independently, reacting to events from other services without central coordination.
Real-Life Example
E-commerce order processing where services react to events from other services to complete the order flow.
AWS Services
Amazon SNS: Publish events.
AWS Lambda: Each service reacts to events and emits new ones.
Implementation
The OrderPlaced event triggers the payment service to process the payment.
The PaymentProcessed event triggers the shipping service to prepare the shipment.
Benefits
Increased service autonomy
Better scalability in some scenarios
8. Event-Carried State Transfer
Description
The event itself contains the necessary state information, reducing inter-service queries.
Real-Life Example
Customer profile updates where the event contains all updated fields.
AWS Services
Amazon EventBridge: For routing events with state.
AWS Lambda: For processing the state contained in the event.
Implementation
When a customer profile is updated, publish an event with all the new profile fields.
EventBridge routes this event to all services that need to update their records (e.g., notifications, billing).
Benefits
Reduces inter-service queries
Simplifies event consumers
9. Event Stream Processing
Description
Analyzes and acts on real-time data streams, treating events as a continuous flow of data.
Real-Life Example
Real-time fraud detection in financial transactions.
AWS Services
Amazon Kinesis: To process real-time streams of data.
AWS Lambda: To act on incoming data streams.
Implementation
Use Kinesis to stream financial transaction data in real time.
Process the stream with Lambda to detect fraud based on transaction patterns.
Benefits
Real-time insights and actions
Handles high-volume, high-velocity data
10. Event Collaboration
Description
Multiple services collaborate by sharing events to complete a business process.
Real-Life Example
Insurance claim processing involving multiple departments.
AWS Services
Amazon SNS/SQS: For event publishing and consumption.
AWS Lambda: To process events and complete business processes.
Implementation
An insurance claim processing system where each department (claims, payments, legal) subscribes to an SNS topic.
Each department works on the event and publishes updates to an SNS topic.
Benefits
Enables complex business processes across services
Promotes loose coupling
11. Dead Letter Queue
Description
A service that stores messages that other services were unable to process for later analysis or reprocessing.
Real-Life Example
E-commerce system storing failed payment processing attempts for later retry.
AWS Services
Amazon SQS: For dead letter queue management.
AWS Lambda: To monitor and process dead-lettered messages.
Implementation
Set up an SQS Dead Letter Queue for failed payment events in an e-commerce system.
Use a Lambda function to analyze failed payments and retry processing.
Benefits
Prevents data loss from failed processing
Aids in system debugging and monitoring
12. Event Versioning
Description
Strategies for handling changes in event schemas over time.
Real-Life Example
Evolving customer data schema in a CRM system.
AWS Services
Amazon SNS: For publishing versioned events.
AWS Lambda: To handle multiple event versions.
Implementation
Publish UserUpdated v2 event with a new schema.
Older services can continue using UserUpdated v1 while newer services use v2.
Benefits
Allows system evolution without breaking existing consumers
Supports backward and forward compatibility
13. Projections
Description
Creating read-optimized views of event-sourced data.
Real-Life Example
Generating monthly account statements from transaction events.
AWS Services
Amazon DynamoDB: Store events.
AWS Lambda: To create read-optimized views of the data.
Implementation
Use DynamoDB to store events and Lambda to create projections like monthly account statements.
Queries for projections are optimized for specific use cases.
Benefits
Optimizes read performance
Allows for specialized views of data
14. Event Replay
Description
Reconstructing application state by replaying stored events.
Real-Life Example
Debugging production issues by replaying events to reproduce the problem.
AWS Services:
Amazon S3: Store events for replay.
AWS Lambda: To replay events and reconstruct state.
Implementation
Store all events in S3.
Replay events using Lambda to debug a production issue or reconstruct system state.
Benefits
Aids in debugging and testing
Supports system recovery and data reconstruction
Conclusion
These patterns form a comprehensive toolkit for designing and implementing event-driven architectures. Each pattern addresses specific challenges in distributed systems and can be combined to create robust, scalable, and responsive architectures.
When implementing these patterns, consider your specific use case, scalability requirements, and the complexity trade-offs each pattern introduces. Start with simpler patterns and evolve your architecture as your understanding and requirements grow.
Remember, the goal is not to use every pattern, but to select and combine patterns that best solve your particular challenges and help you build better software systems. I will explore implementations of each pattern in upcoming articles and will provide a demonstration on their practical applications, I look forward to your feedback.
Subscribe to my newsletter
Read articles from Harshwardhan Choudhary directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
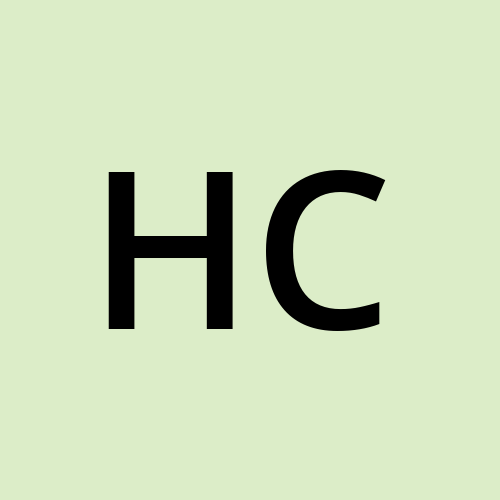
Harshwardhan Choudhary
Harshwardhan Choudhary
Passionate cloud architect specializing in AWS serverless architectures and infrastructure as code. I help organizations build and scale their cloud infrastructure using modern DevOps practices. With expertise in AWS Lambda, Terraform, and data engineering, I focus on creating efficient, cost-effective solutions. Currently based in the Netherlands, working on projects that push the boundaries of cloud computing and automation.