Code to Container: Deploying Apps with Docker in VS Code
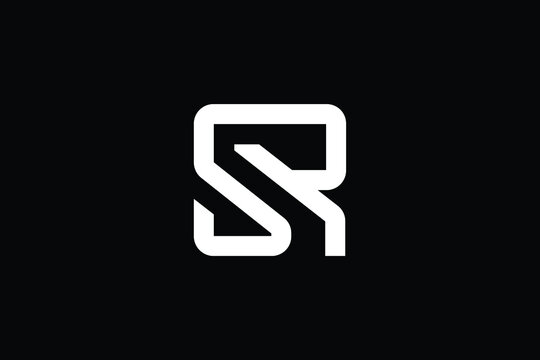
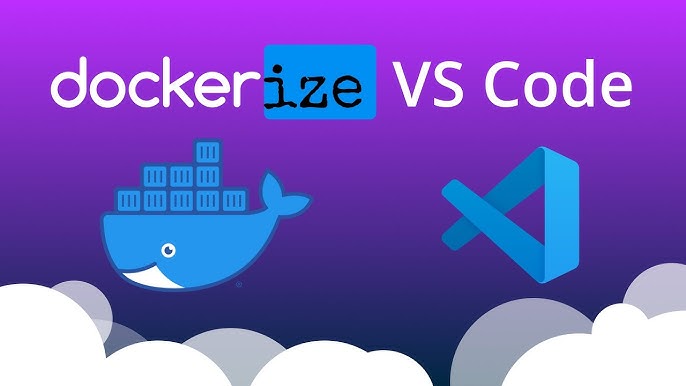
Step 1: Install Docker
Windows/Mac: Install Docker Desktop.
Install the docker application with recommended suggestions.
After Installation, start docker and ensure it’s running.
Step 2. Install Docker Extension in VS Code
Open VS Code.
Go to the Extensions view by clicking the Extensions icon on the Activity Bar or pressing
Ctrl + Shift + X
.In the search bar, type "Docker" and look for the Docker extension by Microsoft.
Click Install to add the Docker extension to your VS Code.
Once installed, a Docker icon should appear in the left-hand sidebar.
Step 3. Create a Dockerfile for Your Application
In your project folder, create a file named
Dockerfile
(without any extension).Define your Docker build steps. Below is the sample template to build the docker image form the file.
# Base Image
FROM <base image>
# Set the working directory
WORKDIR /app
#Copy the code from host to docker container
COPY <source host> <destination container>
#Required Libraries/ Install dependencies (This step defines the steps to be taken before building the image)
RUN npm install
RUN pip install --no-cache-dir -r requirements.txt
# Expose the port your app will run on (e.g.3000)
EXPOSE 3000
# Command to run the application
CMD ["node", "app.js"]
CMD ["python", "app.py"]
- Save the file. You can customize this
Dockerfile
based on your application’s technology stack.
Step 4: Build the Docker Image
Now that you have the Dockerfile, you can build your Docker image using VS Code.
Open the Command Palette by pressing
Ctrl + Shift + P
.Type
Docker: Build Image
and select it.If prompted, select the
Dockerfile
from your workspace.Provide a name for the image (e.g.,
my-app:latest
).
You can also build the image from the terminal in VS Code using:
docker build -t image-name:tag . #docker build -t my-app:latest . >> -t: tag to image >> .: current directory
Step 5: Run the Docker Image
Once the image is built, you can run it in a container.
Open the Command Palette again (
Ctrl + Shift + P
).Type
Docker: Run
and select it.Choose the image you built (
my-app:latest
).Specify the port mappings (e.g.,
3000:3000
if your app is running on port 3000).Optionally, specify environment variables or other configurations.
Alternatively, you can run it via the terminal:
docker run -d -p 3000:3000 my-app:latest #docker run -d -p 3000:3000 my-app:latest >> -d: detached mode >> -p: publish/expose/bind the port >> 3000:3000: host port: container port >> my-app: Image name >> latest: tag
Step 6: View and Manage Docker Containers in VS Code
After running the container, you can manage it using the Docker extension.
Click on the Docker icon on the left sidebar.
Under Containers, you’ll see the running container.
Right-click on the container to access options like Stop, Restart, View Logs, and Attach Shell.
Step 7: Pushing Images to Docker Hub
If you want to push your image to Docker Hub:
Open the Command Palette and type
Docker: Push
.Choose the image you want to push.
Enter your Docker Hub credentials if required.
Alternatively, use the terminal:
docker tag <image-id> username/repo-name:tag
#docker tag bee42b9f165c sakshipathak/my-to-do-app:latest
docker push username/repo-name:tag
#docker push sakshipathak/my-to-do-app:latest
Step 8: Stopping and Removing Containers
To stop and remove running containers:
In the Docker extension panel, right-click on the container and select Stop.
To remove the container, right-click again and select Remove.
You can also do this from the terminal:
docker stop container-id docker rm container-id
Subscribe to my newsletter
Read articles from SAKSHI RISHIPATHAK directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
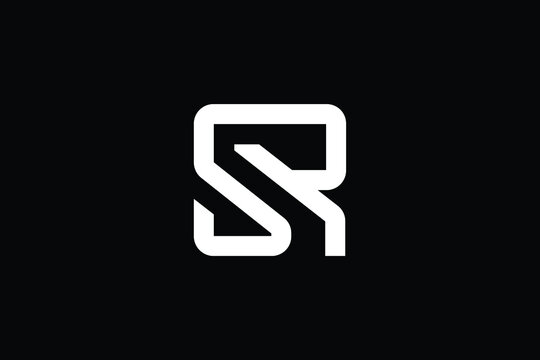
SAKSHI RISHIPATHAK
SAKSHI RISHIPATHAK
I'm an experienced Software Development Engineer in Test (SDET) deeply passionate about DevOps practices. My focus lies in ensuring software quality through rigorous testing and automation. I leverage my background in software engineering to integrate DevOps principles, aiming to optimize efficiency and enhance collaboration across teams. I'm dedicated to continuous improvement and excited to contribute to advancing DevOps methodologies.