WebSockets vs. Server-Sent Events (SSE) vs. WebRTC
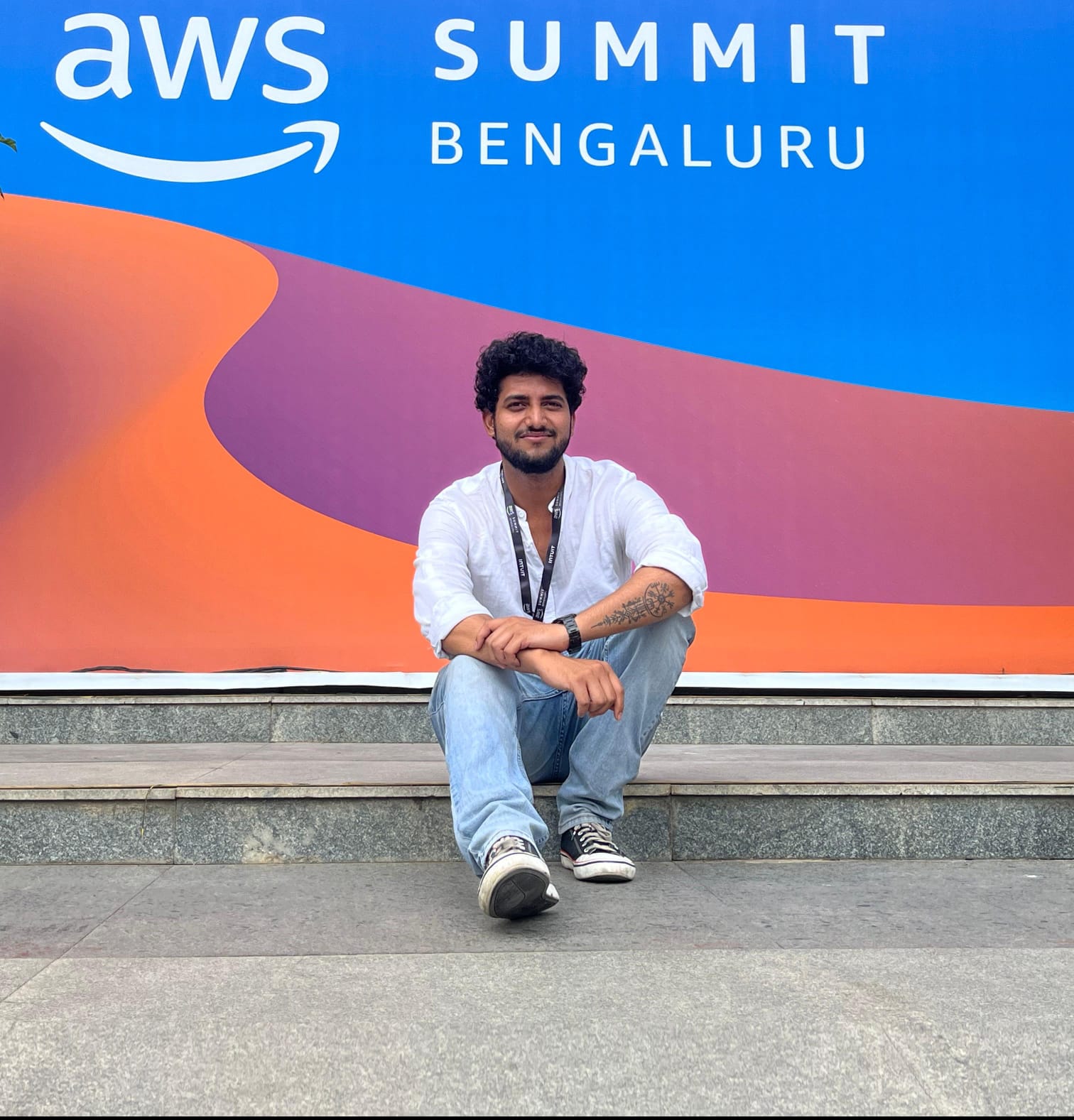
In today’s fast-paced digital world, real-time communication is a key component of many web applications. Whether it's live messaging, notifications, or video calls, delivering instant updates and responses can make or break user experience. To achieve this, web developers have a few tools at their disposal: WebSockets, Server-Sent Events (SSE), and WebRTC. Each has its own strengths and ideal use cases, but which one is the best fit for your project?
In this blog, we’ll break down the differences between these three technologies, give you some easy-to-understand examples, and help you choose the right one for your next web app.
WebSockets: Two-Way Communication for Real-Time Interactions
WebSockets are all about speed and two-way communication. Unlike regular HTTP requests that only allow the client (browser) to request data from the server, WebSockets let both the client and the server send messages to each other as soon as they have something to say. This makes WebSockets perfect for scenarios where fast, back-and-forth interaction is needed, like:
Real-time chat applications
Online multiplayer games
Collaborative tools (like Google Docs)
Why Use WebSockets?
Full-duplex communication: Both client and server can send messages anytime.
Low-latency: Great for real-time data transfer where speed is crucial.
Client Side Code
// Create a new WebSocket connection
const socket = new WebSocket('wss://example.com/socket');
// When the connection opens, send a message to the server
socket.addEventListener('open', () => {
console.log('Connected to the server');
socket.send('Hello, Server!');
});
// Listen for messages from the server
socket.addEventListener('message', (event) => {
console.log('Message from server:', event.data);
});
// Handle connection closure
socket.addEventListener('close', () => {
console.log('Connection closed');
});
Server Side Code
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', ws => {
console.log('Client connected');
// Respond to messages from the client
ws.on('message', message => {
console.log('Received:', message);
ws.send('Hello, Client!');
});
// Handle connection closure
ws.on('close', () => {
console.log('Client disconnected');
});
});
Server-Sent Events (SSE): Simple, One-Way Updates
Server-Sent Events (SSE) are great when you only need the server to send updates to the client without expecting the client to send much back. Think of it as the server constantly pushing information to the browser. This is perfect for live updates that don’t need to go both ways, like:
Real-time news feeds
Live sports scores
Stock price updates
Why Use SSE?
One-way communication: The server pushes data to the client.
Easy to implement: SSE uses standard HTTP, making it simple to set up.
Automatic reconnection: If the connection drops, the browser will automatically try to reconnect.
Client Side Code
// Set up an EventSource to receive updates from the server
const eventSource = new EventSource('/events');
// Listen for incoming messages
eventSource.onmessage = (event) => {
console.log('New message from server:', event.data);
};
// Handle errors (e.g., if the connection is lost)
eventSource.onerror = (error) => {
console.error('Error with SSE:', error);
};
Server Side Code
const http = require('http');
http.createServer((req, res) => {
if (req.url === '/events') {
// Set headers to enable SSE
res.writeHead(200, {
'Content-Type': 'text/event-stream',
'Cache-Control': 'no-cache',
'Connection': 'keep-alive',
});
// Send an update every second
setInterval(() => {
res.write(`data: ${new Date().toLocaleTimeString()}\n\n`);
}, 1000);
}
}).listen(8080, () => console.log('SSE server running on port 8080'));
WebRTC: Peer-to-Peer Magic for Media and Data
WebRTC is a little different from WebSockets and SSE. It’s designed for peer-to-peer (P2P) communication, meaning you can connect two users directly without needing a central server to handle the data. This makes it the go-to option for things like:
Video calls (e.g., Zoom, Google Meet)
File sharing between users
Multiplayer games where players interact directly
Why Use WebRTC?
Peer-to-peer connections: Allows users to communicate directly, reducing server load.
Low-latency media streaming: Ideal for video, audio, and real-time data sharing.
Secure: WebRTC encrypts data by default.
Client Side Code
// Create RTCPeerConnection for both peers
const peer1 = new RTCPeerConnection();
const peer2 = new RTCPeerConnection();
// Create a data channel on peer1
const dataChannel = peer1.createDataChannel('chat');
dataChannel.onopen = () => console.log('Data channel open');
dataChannel.onmessage = (event) => console.log('Message from Peer 2:', event.data);
// Exchange offer and answer to establish connection
peer1.createOffer().then(offer => {
peer1.setLocalDescription(offer);
peer2.setRemoteDescription(offer);
peer2.createAnswer().then(answer => {
peer2.setLocalDescription(answer);
peer1.setRemoteDescription(answer);
});
});
// Send a message once the connection is established
dataChannel.send('Hello Peer 2!');
Comparison at a Glance:
Feature | Websockets | Server Sent Events (SSE) | WebRTC |
Communication Direction | Two-way (full-duplex) | One-way (server to client) | Peer-to-Peer |
Use Cases (best for) | Live chat, gaming, collaboration | Live Updates, notifications | P2P apps, Video Calls, file sharing |
Latency | Very Low Latency | Slightly higher | Optimized for low latency |
Connection Setup | Persistent Connection | Persistent |
(Retries built-in) | Requires signalling server |
Summary (Which one should you choose)
Go with WebSockets if you need fast, two-way communication like in a live chat or online game. It’s the best option for frequent back-and-forth messaging.
Use SSE if you just need to push real-time updates from the server to the client, like live scores or stock prices. SSE is simple, reliable, and easy to set up.
Pick WebRTC if your application involves peer-to-peer communication, such as video conferencing or file sharing. WebRTC is optimized for low-latency, real-time media sharing.
With this breakdown, you’re now equipped to decide which real-time communication tool works best for your project. Whether you're pushing live updates, building a chat app, or setting up a video call, knowing the strengths of WebSockets, SSE, and WebRTC will make a huge difference in your app's performance and scalability.
Happy coding!
Subscribe to my newsletter
Read articles from Brijesh Pandey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
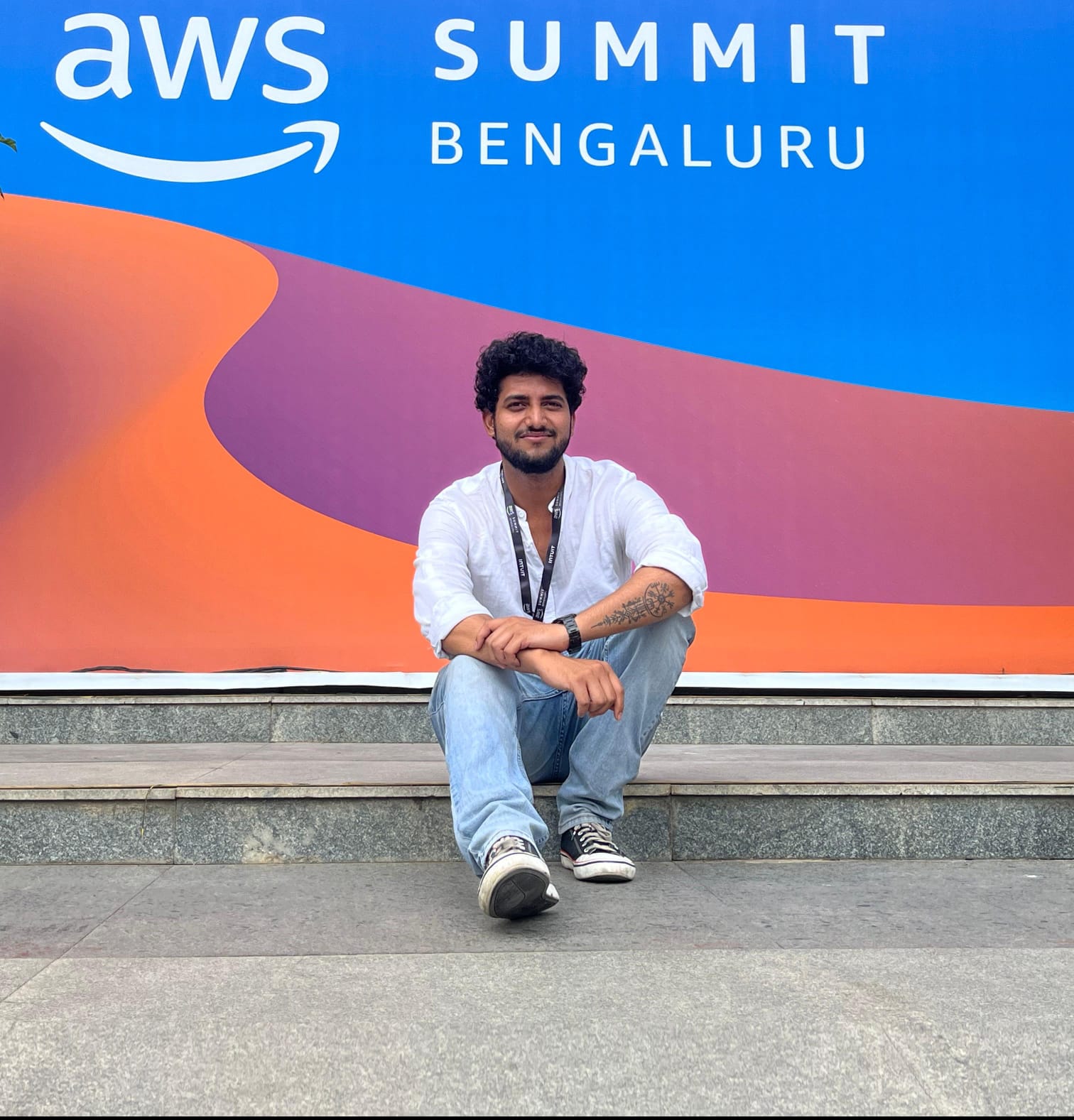
Brijesh Pandey
Brijesh Pandey
I'm a passionate software engineer with a strong background in web development, including both frontend and backend technologies. I'm particularly excited about the potential of Web 3.0 and enjoy working on cutting-edge projects in this space. Beyond coding, I'm an adventurous individual who loves to travel and explore new places, cultures, and cuisines. I'm also athletic and enjoy playing football, cricket, and badminton. I'm always up for a challenge and believe in trying new things!