How to style child elements in Bootstrap Components
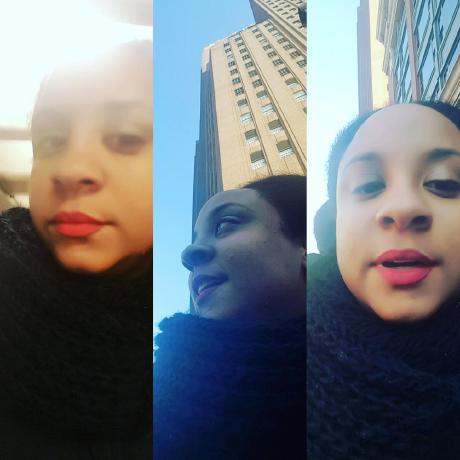
During my time at Teachers Pay Teachers (TpT) on the Easel team, we once had a Customer-Delight-o-than or rather a sprint where we only focused on implementing features and changes to Easel that our users requested.
I took on the seemingly easy task of changing the hover state of the cursor when the user hovered over checkboxes and text in our filter section.
I tried everything in my tool belt. I tried targeting the text directly. No change. I tried using the ::before
and ::after
pseudo-elements. Fail.
Finally, after struggling, Googling, and collaborating/pairing with other engineers on this ticket, I discovered that the issue with this problem was that Bootstrap components like Form.Check
use custom classes that can complicate styling their children elements. Because I wanted to target the checkboxes and text nested within this component, I needed to find a way to select these child components using an unconventional approach ensuring the cursor style was applied.
Basically, I had to target the child component or prop label by nesting the label selector within the custom class and applying the desired style change there.
The Form.Check
component looks like this in code:
<Form.Check
type="switch"
id="custom-switch"
label="Check this switch"
/>
Nesting the label in CSS looked like this:
.checkbox {
& label {
cursor: pointer;
}
}
Why Use the Ampersand (&
)?
In the nested CSS structure, the ampersand (&
) acts as a reference to the parent selector—in this case, .checkbox
. It’s particularly useful when you want to style an element based on its context within the parent. In this example, & label
tells the CSS processor to combine .checkbox
with the label
selector, resulting in .checkbox label
, applying the desired style only within that scope.
Without the ampersand, the following CSS would work, but it would apply the style to any label
inside .checkbox
regardless of hierarchy
.checkbox {
label {
cursor: pointer;
}
}
While both work, the ampersand provides cleaner, more maintainable code, especially when working with larger, more complex projects.
Why targeting child components in Bootstrap is so difficult
Bootstrap’’s components are highly structured, with many nested elements and predefined classes. This design choice promotes reusability and consistency (which is why we love Bootstrap and similar libraries in the first place). However, this consistency can make it difficult to customize styles within the Bootstrap components (classic Bootstrap downside). Bootstrap’s default CSS often applies to parent components, leaving developers tasked with manually adjusting the style of child components by nesting selectors or using other creative and advanced CSS techniques.
Other child components you may want to customize in Bootstrap
Form.Group and Input Elements
The
Form.Group
component is often used to group form elements such as labels and inputs. Due to its nested structure, targeting both the label and input fields can be tricky. For example, changing the hover state or styling the input alongside its label might require targeting the child elements in a similar nested fashion. You may need to use something like:.form-group { & label { font-weight: bold; } & input { border-color: blue; } }
This is necessary when you want consistent styling across the entire group of form elements.
2. Dropdowns
Bootstrap dropdown components can also pose challenges because the toggle button and the dropdown menu are separate elements. If you want to style both, such as changing the button's hover state and the dropdown menu’s appearance at the same time, you might need to nest the selectors or override specific Bootstrap classes:
.dropdown {
& .dropdown-toggle {
color: red;
}
& .dropdown-menu {
background-color: blue;
}
}
The nested nature of these elements means that conventional CSS approaches might not always work smoothly.
3. Card
Bootstrap Card
components can also require creative styling approaches when you want to target both the card body and the footer or header at once. Cards often contain multiple nested elements like .card-body
, .card-header
, and .card-footer
, and applying hover effects or uniform styles might involve using nested selectors:
.card {
& .card-header, & .card-footer {
background-color: lightgray;
}
& .card-body {
color: darkgray;
}
}
Without using this approach, you may struggle to consistently apply styles across different sections of the card.
4. Nav and Nav.Link
The Nav
and Nav.Link
components are another area where nested structures can complicate styling. For example, changing the hover state for both the Nav
container and its child links often requires a combination of custom classes and nested CSS:
.nav {
& .nav-link {
color: blue;
&:hover {
background-color: lightblue;
}
}
}
This approach becomes necessary because the default Bootstrap classes often apply limited styles to only the immediate children, leaving deeper elements untouched.
5. Accordion
The Accordion
component in Bootstrap uses a nested structure with .accordion-item
, .accordion-header
, and .accordion-body
elements. Styling all parts of the accordion at once, such as changing the background color when expanded, can require nested selectors:
.accordion {
& .accordion-header {
font-size: 1.2rem;
}
& .accordion-body {
padding: 15px;
}
}
The interaction between the expanding/collapsing feature and its internal structure might also require workarounds to ensure proper styling.
Further reading:
MDN Web Docs: Nesting Selector, https://developer.mozilla.org/en-US/docs/Web/CSS/Nesting_selector
Sass: Nest Rules, https://sass-lang.com/documentation/style-rules/
Bootstrap Components, https://getbootstrap.com/docs/5.0/customize/components/
Mastering Flexbox, https://css-tricks.com/snippets/css/a-guide-to-flexbox/
Mastering CSS Grid, https://css-tricks.com/snippets/css/complete-guide-grid/
Common Bootstrap Pitfalls, https://www.smashingmagazine.com/2013/03/customizing-bootstrap/
CSS Specificity, https://developer.mozilla.org/en-US/docs/Web/CSS/Specificity
Understanding Pseudo-elements, https://developer.mozilla.org/en-US/docs/Web/CSS/Pseudo-elements
About the Author: Nicole Gathany is a freelance web developer and software engineer who loves Product and loves puns.
Subscribe to my newsletter
Read articles from Nicole Gathany directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
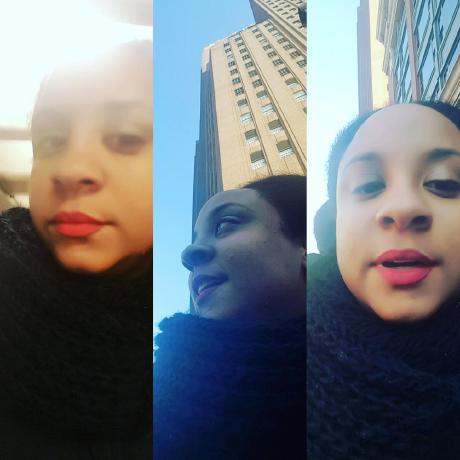
Nicole Gathany
Nicole Gathany
I am a people-centered software engineer with a past life in public health and reproductive justice. I'm using this blog to combine my love for tech and communication.