Importing Existing VPC in AWS CDK
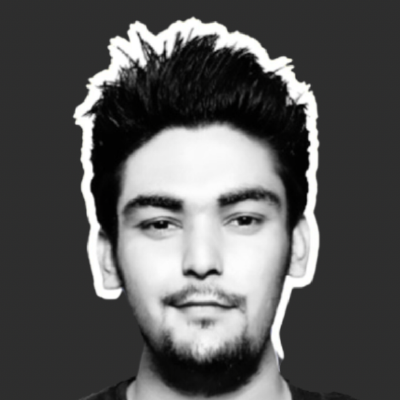
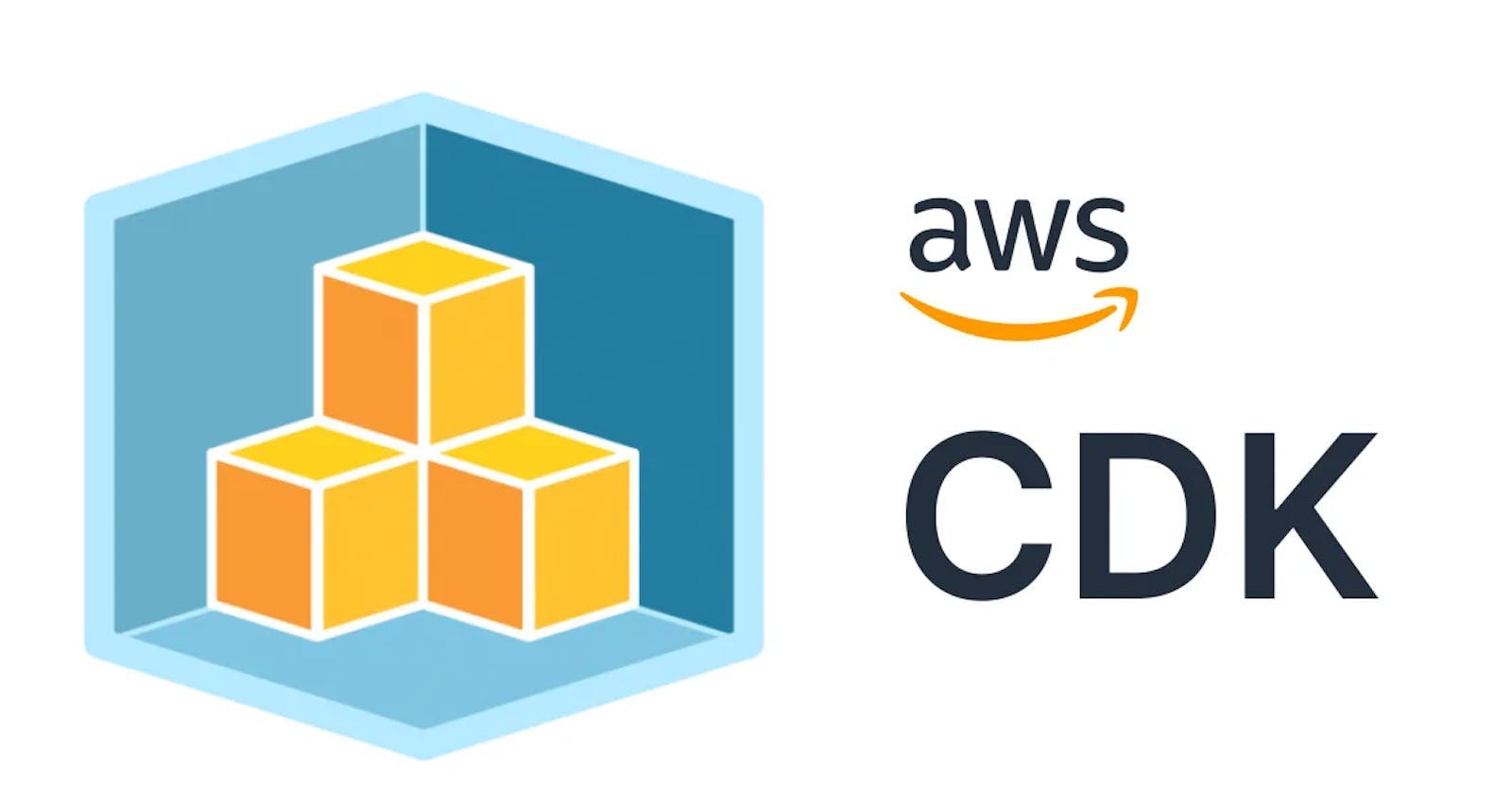
Managing network infrastructure efficiently is crucial for deploying scalable and secure applications on AWS. AWS Cloud Development Kit (CDK) offers a powerful way to define cloud infrastructure using familiar programming languages. This guide provides a comprehensive approach to importing an existing Virtual Private Cloud (VPC) into your AWS CDK project.
Prerequisites
AWS CDK Installed: Ensure you have the AWS CDK installed. If not, follow the installation guide.
Existing VPC: An existing VPC in your AWS account that you intend to import.
Configured AWS Credentials: Ensure your AWS credentials are properly configured.
Overview
Importing an existing VPC into AWS CDK involves using the fromLookup
static method provided by the Vpc
construct. This method allows CDK to reference a VPC that already exists in your AWS environment. It's essential to explicitly specify the stack environment (account and region) to enable CDK to locate the VPC accurately.
Setting Up the CDK Application
Defining the Stack Environment
Start by explicitly setting the stack's environment with the AWS account and region. This step ensures that CDK performs the lookup in the correct context.
// bin/app.ts
import * as cdk from 'aws-cdk-lib';
import { ImportVpcStack } from '../lib/import-vpc-stack';
const app = new cdk.App();
new ImportVpcStack(app, 'import-vpc-stack', {
env: {
region: 'us-east-1', // specify your AWS region
account: '123456789012', // replace with your AWS account ID
},
});
Importing an Existing VPC by Name
To import a VPC by its name, utilize the fromLookup
method and provide the VPC name. This approach is straightforward and leverages the VPC's unique name within your AWS environment.
// lib/import-vpc-stack.ts
import * as ec2 from 'aws-cdk-lib/aws-ec2';
import * as lambda from 'aws-cdk-lib/aws-lambda';
import { NodejsFunction } from 'aws-cdk-lib/aws-lambda-nodejs';
import * as cdk from 'aws-cdk-lib';
import * as path from 'path';
export class ImportVpcStack extends cdk.Stack {
constructor(scope: cdk.App, id: string, props: cdk.StackProps) {
super(scope, id, props);
// import VPC by Name
const myVpc = ec2.Vpc.fromLookup(this, 'external-vpc', {
vpcName: 'YOUR_VPC_NAME', // replace with your VPC name
});
console.log('VPC ID:', myVpc.vpcId);
console.log('VPC CIDR Block:', myVpc.vpcCidrBlock);
// create a Lambda function within the imported VPC
const myFunction = new NodejsFunction(this, 'function', {
vpc: myVpc,
runtime: lambda.Runtime.NODEJS_20_X,
handler: 'handler',
entry: path.join(__dirname, '/../lambdas/lambda/index.js'),
allowPublicSubnet: true,
});
}
}
Additional Lookup Options
The fromLookup
method provides several options to identify the VPC:
isDefault: Boolean value to match the default VPC.
tags: Key-value pairs to filter the VPC based on tags.
vpcId: Directly specify the VPC ID.
These options offer flexibility in selecting the appropriate VPC, especially in environments with multiple VPCs.
Viewing Synthesized CloudFormation
After defining your stack, you can inspect the synthesized CloudFormation template using:
npx aws-cdk synth
or
cdk synth
Initially, placeholder values appear until CDK performs the VPC lookup and fetches the actual values. This synthesis helps verify that the imported VPC is correctly referenced in your stack.
Deploying the Stack
Deploy your CDK stack to provision resources. Upon successful deployment, the Lambda function will be deployed within the imported VPC.
npx aws-cdk deploy
or
cdk deploy
You can verify the deployment by checking the Lambda console, which should indicate that the function resides within the specified VPC.
cdk.context.json
file to optimize future deployments by avoiding repeated lookups. This caching includes values like VPC ID, CIDR blocks, and subnet groups.Refreshing Cached VPC Values
If the VPC's configuration changes, you need to refresh the cached values to reflect these updates in your CDK stack. Follow these steps to reset the cache:
Identify the Cache Key or Number:
npx aws-cdk context or cdk context
This command lists all cached context values with their corresponding keys and numbers.
Reset the Cache:
npx aws-cdk context --reset KEY_OR_NUMBER or cdk context --reset KEY_OR_NUMBER
Replace
KEY_OR_NUMBER
with the appropriate identifier from the previous step.Synthesize Again:
npx aws-cdk synth or cdk synth
CDK will perform a fresh lookup for the VPC, updating the
cdk.context.json
file with the latest values.
Importing the Default VPC
To import the default VPC, set the isDefault
option to true
in the fromLookup
method. Since there is only one default VPC per account and region, this configuration is sufficient for CDK to identify it.
// lib/import-vpc-stack.ts
const defaultVpc = ec2.Vpc.fromLookup(this, 'default-vpc-id', {
isDefault: true,
});
Ensure that the stack environment is explicitly set to help CDK locate the default VPC accurately.
Importing an Existing VPC by Tags
Tag-based lookup allows you to import a VPC by specifying key-value pairs. This method is particularly useful when managing multiple VPCs with distinct tags.
Tag Your VPC: Add a tag to your VPC, for example:
Key:
app
Value:
web
Import the VPC Using Tags:
// lib/import-vpc-stack.ts const myVpc = ec2.Vpc.fromLookup(this, 'by-tags-id', { tags: { app: 'web', }, });
This approach ensures that CDK imports the VPC matching the specified tag criteria.
Importing an Existing VPC by VPC ID
Directly specifying the VPC ID provides a precise method to import a VPC without ambiguity.
// lib/cdk-starter-stack.ts
const myVpc = ec2.Vpc.fromLookup(this, 'external-vpc', {
vpcId: 'vpc-091234567ee1234567', // replace with your VPC ID
});
This method is straightforward and eliminates the need for additional lookup parameters.
Considerations for Importing Existing VPCs
When importing existing VPCs into your CDK stacks, consider the following limitations and best practices:
Explicit Environment Specification:
Requirement: Always set the stack's environment (
account
andregion
).Reason: CDK needs to know where to perform the VPC lookup accurately.
Immutable Imported VPCs:
Limitation: Imported VPCs cannot be modified by CDK.
Implication: Ensure that the VPC configuration meets your requirements before importing.
Context Caching:
Behavior: After the initial lookup, VPC details are cached in
cdk.context.json
.Action: If the VPC's configuration changes, reset the cache using the
--reset
flag to perform a fresh lookup.
Cache Reset Procedure:
npx aws-cdk context --reset CONTEXT_NUMBER_OR_KEY or cdk context --reset CONTEXT_NUMBER_OR_KEY
Replace
CONTEXT_NUMBER_OR_KEY
with the appropriate identifier from thecdk.context.json
file.
Summary
Importing an existing VPC into AWS CDK is a powerful feature that enables seamless integration of your existing network infrastructure with CDK-managed resources. By leveraging methods such as fromLookup
with various lookup options, you can efficiently reference and utilize your VPCs within your CDK applications. Always remember to manage context caching appropriately and adhere to best practices to maintain a robust and maintainable infrastructure as code setup.
Subscribe to my newsletter
Read articles from Mikaeel Khalid directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
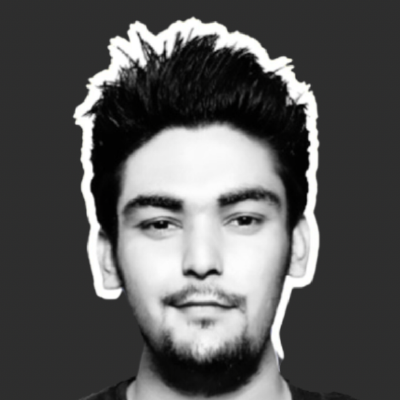
Mikaeel Khalid
Mikaeel Khalid
I am a Software and Certified AWS & GCP Engineer, I am passionate about solving complex technical problems. My goal here is to help others by sharing my learning journey.