Activity #22: Research: Python Flask
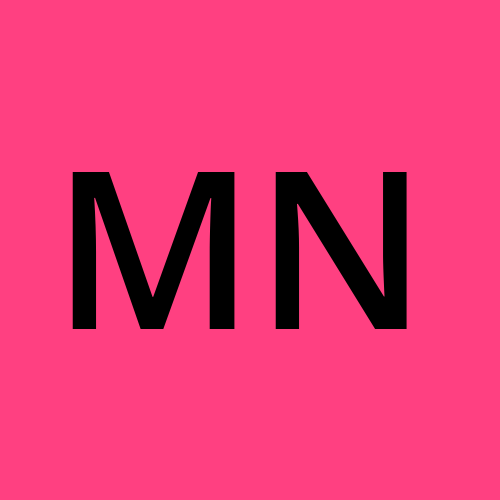
What is Flask?
Flask is a lightweight and easy-to-use web framework for Python. It’s designed to be simple and flexible, providing the essential tools to build web applications without requiring large overhead.
It’s called a "micro-framework" because it doesn’t require specific libraries or tools by default, although you can add extensions as needed.
Installing Flask
Before you can use Flask, you’ll need to install it using
pip
. Open your terminal or command prompt and run:pip install Flask
After installation, you can verify it by checking the version:
python -m flask --version
Creating Your First Flask Application
Here’s a simple step-by-step guide to creating your first Flask app:
Create a New Project Folder:
- Create a folder where you will keep your Flask project.
Example:
mkdir flask_app
cd flask_app
Create a Python File:
- Inside the
flask_app
folder, create a file namedapp.py
.
- Inside the
Basic Flask Application Code:
- Open
app.py
and write the following code to create a basic Flask application:
- Open
from flask import Flask
# Create a Flask app instance
app = Flask(__name__)
# Define a route
@app.route('/')
def home():
return "Hello, Flask!"
# Run the application
if __name__ == '__main__':
app.run(debug=True)
Explanation:
Flask(__name__)
: Initializes the Flask application.@app.route('/')
: Sets up a route to handle requests to the homepage (/
).return "Hello, Flask!"
: Returns this message when visiting the homepage.app.run(debug=True)
: Starts the web server in debug mode so you can see real-time changes.
Running the Flask Application
Run the Flask application by executing the following command:
python app.py
After running the command, Flask will start a development server. You should see an output like this:
* Running on http://127.0.0.1:5000/ (Press CTRL+C to quit)
Open your browser and go to
http://127.0.0.1:5000/
. You should see "Hello, Flask!" displayed on the page.
Routing in Flask
Flask allows you to define different routes (URLs) that map to specific functions in your application. Here’s how you can add more routes:
Update
app.py
@app.route('/about') def about(): return "This is the About page." @app.route('/contact') def contact(): return "Contact us at contact@example.com"
Now, visiting
/about
and/contact
in your browser will show different messages.Go to:
http://127.0.0.1:5000/about
→ This will display: "This is the About page."Go to:
http://127.0.0.1:5000/contact
→ This will display: "Contact us at contact@example.com"
Dynamic Routing
You can also create dynamic routes that take parameters. For example, you can display a personalized greeting by capturing a username from the URL:
@app.route('/user/<name>') def user(name): return f"Hello, {name}!"
If you visit
http://127.0.0.1:5000/user/Monet
, you will see "Hello, Monet!".
Rendering HTML Templates
- Flask allows you to serve HTML files using templates. To do this, you need to create a folder called
templates
where your HTML files will be stored.
Create a Folder for Templates:
- Inside your project folder, create a
templates
folder and create an HTML file inside it. For example, createindex.html
:
- Inside your project folder, create a
<!-- templates/index.html -->
<html>
<head>
<title>Flask App</title>
</head>
<body>
<h1>Welcome to Flask!</h1>
</body>
</html>
Modify Your Flask Code to Render Templates:
from flask import Flask, render_template app = Flask(__name__) @app.route('/') def home(): return render_template('index.html')
Now when you visit the homepage, Flask will serve the
index.html
file.
Handling Forms and POST Requests
- Flask supports handling form data and POST requests. Here’s an example where a user submits their name through a form:
Create a Form in HTML (
form.html
):<!-- templates/form.html --> <form method="POST"> Name: <input type="text" name="name"> <input type="submit"> </form>
Update Your Flask Application to Handle the Form:
from flask import Flask, render_template, request app = Flask(__name__) @app.route('/form', methods=['GET', 'POST']) def form(): if request.method == 'POST': name = request.form['name'] return f"Hello, {name}!" return render_template('form.html')
Now, when you visit
/form
, you’ll see the form. After submitting your name, it will display a personalized greeting.Open your browser and go to
http://127.0.0.1:5000/form
.
Using Flask Extensions
Flask has many extensions that add more features to your app, such as authentication, database handling, etc. Some common extensions include:
Flask-SQLAlchemy for database management.
Flask-WTF for working with forms.
Flask-Login for user authentication.
You can install them via pip:
pip install Flask-SQLAlchemy
pip install Flask-WTF
pip install Flask-Login
Subscribe to my newsletter
Read articles from Monette Nicolas directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
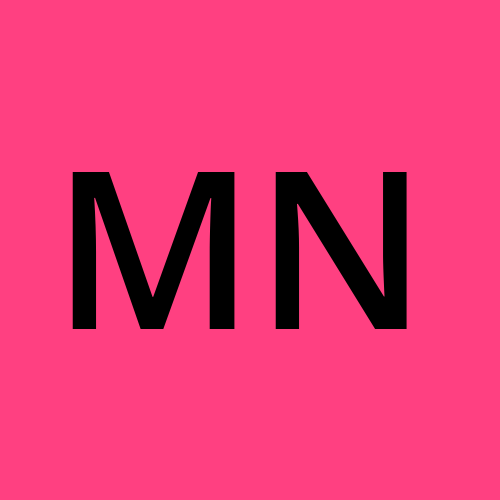