Python Solutions for Parsing JSON and YAML in Cloud

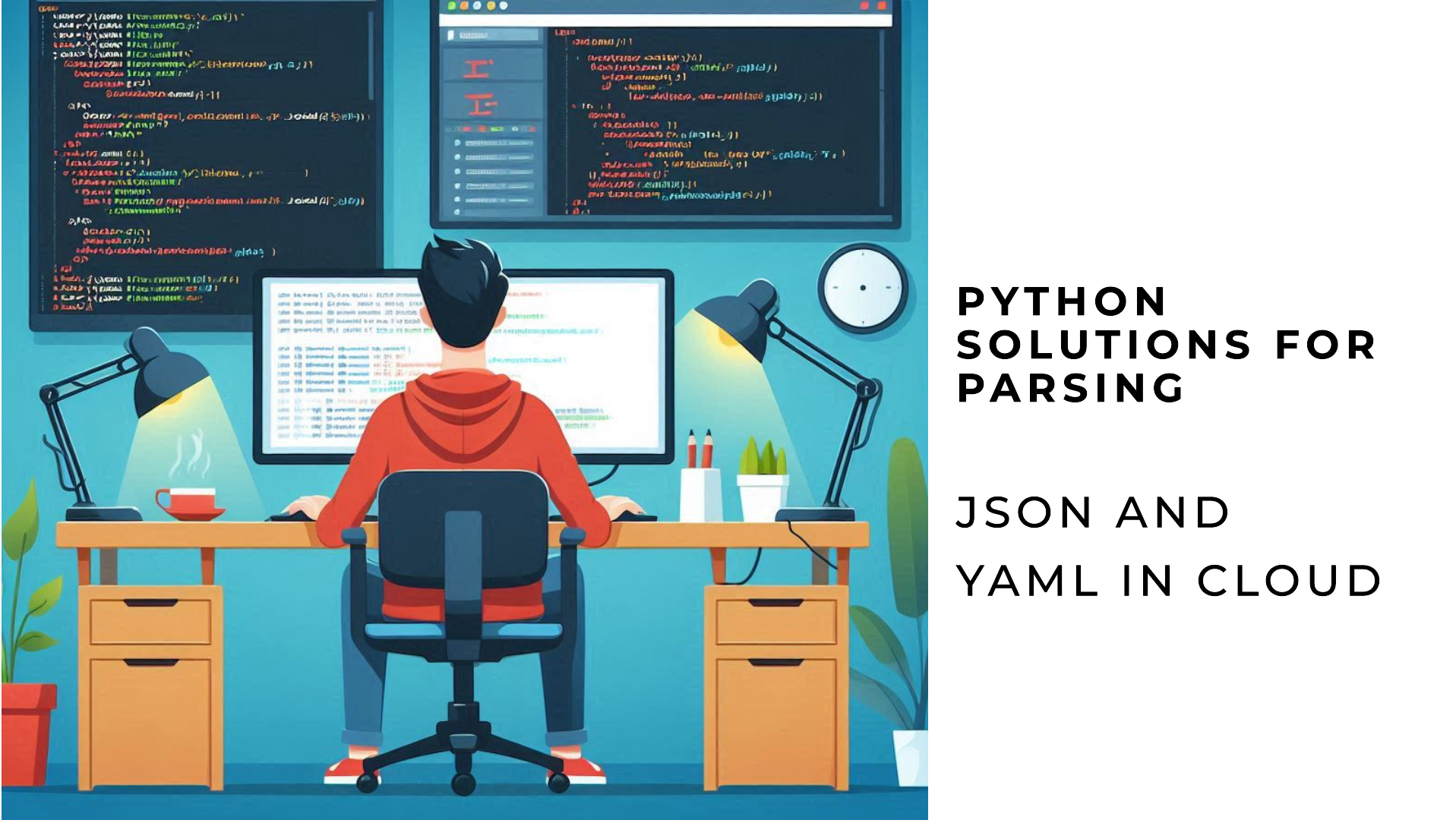
Working with JSON and YAML Files in Python Using Libraries
When with JSON and YAML files in Python, understanding the relevant libraries is important for parsing, processing, and managing file data. Alongside JSON and YAML, other libraries like os and sys help handle the file system and command-line arguments, which are essential for scripting and automation.
1. Python Libraries Overview
os: Provides functions for interacting with the operating system (file handling, directory management, etc.).
sys: Provides access to system-specific parameters and functions (command-line arguments, exiting scripts, etc.).
json: Built-in Python library to handle JSON data (parsing, generating, etc.).
yaml: External library for handling YAML data (needs to be installed via pip).
What Are JSON and YAML Files?
1. JSON (JavaScript Object Notation):
JSON is a lightweight data-interchange format, often used for web APIs, configuration files, or storing data in a structured format.
File Extension: .json
Typical Usage: Configuration files, API responses, and data exchange between client-server systems.
2. YAML (YAML Ain't Markup Language):
YAML is a human-readable data serialization language, popular in configuration files for infrastructure tools like Kubernetes and Docker.
File Extension: .yaml or .yml
Typical Usage: Configuration files, API responses, and data exchange between client-server systems.
2. Parsing JSON File in Python
lets suppose we have a services.json file that holds cloud service providers with their corresponding services.
To parse a JSON file in Python, you can use the built-in json library.
Example: Parsing services.json File
import json
import os
# Check if the file exists
if os.path.exists('services.json'):
# Open and read the JSON file
with open('services.json', 'r') as json_file:
data = json.load(json_file)
# Extract and print service names
for provider, details in data['cloud_services'].items():
print(f"{provider} : {details['service']}")
else:
print("File not found!")
In this example, the JSON file is parsed into a Python dictionary, and the service names of each cloud provider are printed using the keys "aws", "azure", and "gcp".
3. Parsing YAML File in Python
lets suppose we have a services.yaml file that contains the same information as the JSON file but is written in a more concise, readable format.
To parse a YAML file, the yaml library must be installed using pip:
pip install pyyaml
Example: Parsing a services.yaml File and Converting it to JSON
import yaml
import os
import json
# Check if the file exists
if os.path.exists('services.yaml'):
# Open and read the YAML file
with open('services.yaml', 'r') as yaml_file:
data = yaml.safe_load(yaml_file)
# Convert to JSON format (just for visualizing purposes)
json_data = json.dumps(data, indent=2)
print(json_data)
else:
print("File not found!")
Here, the YAML file is parsed and converted into a Python dictionary, which is then printed in a JSON format. The contents of YAML and JSON files are similar, but YAML is generally more human-readable, making it ideal for configuration files.
4. Using os and sys Libraries
os Library:
The os library is used for interacting with the file system, including operations like checking if a file exists, renaming files, creating directories, and more.
Check if a file exists:
import os
if os.path.exists('services.json'):
print("File exists!")
else:
print("File not found!")
Get the current working directory:
print(os.getcwd())
sys Library:
The sys library is useful for interacting with system parameters, including command-line arguments, exiting scripts, and handling standard input/output.
- Access command-line arguments:
import sys
if len(sys.argv) > 1:
print(f"First argument: {sys.argv[1]}")
else:
print("No arguments passed!")
- Exit the script:
import sys
sys.exit("Exiting the script with this message.")
5. Real-Life Example of JSON and YAML in DevOps
In DevOps, configuration management often involves working with JSON and YAML files. For example, you might deal with:
Infrastructure as Code (IaC) tools like Terraform, which outputs configurations in JSON.
Kubernetes or Docker Compose configurations, which use YAML extensively for defining infrastructure and services.
1. Parsing a JSON Configuration File (aws_config.json)
Let's first create a JSON configuration file named aws_config.json to include multiple AWS services like EC2, S3, and Lambda, with additional configuration details such as region, instance types, and storage options.
Python Code for Parsing JSON:
import json
import os
# Check if the JSON file exists
if os.path.exists('aws_config.json'):
# Open and read the JSON file
with open('aws_config.json', 'r') as json_file:
data = json.load(json_file)
# Extracting AWS details
aws_region = data['services']['aws']['region']
ec2_instance_type = data['services']['aws']['ec2']['instance_type']
ec2_instance_count = data['services']['aws']['ec2']['instance_count']
s3_buckets = data['services']['aws']['s3']['buckets']
lambda_function_count = data['services']['aws']['lambda']['function_count']
print(f"AWS Region: {aws_region}")
print(f"EC2 Instance Type: {ec2_instance_type}, Count: {ec2_instance_count}")
print(f"S3 Buckets: {', '.join(s3_buckets)}")
print(f"Lambda Function Count: {lambda_function_count}")
else:
print("aws_config.json file not found!")
2. Parsing a YAML Configuration File (aws_config.yaml)
Next, create a YAML configuration file named aws_config.yaml:
We’ll make similar modifications to the YAML file, including multiple AWS services with details like EC2 instance type, S3 buckets, and Lambda functions.
Python Code for Parsing YAML:
import yaml
import os
# Check if the YAML file exists
if os.path.exists('aws_config.yaml'):
# Open and read the YAML file
with open('aws_config.yaml', 'r') as yaml_file:
data = yaml.safe_load(yaml_file)
# Extracting AWS details
aws_region = data['services']['aws']['region']
ec2_instance_type = data['services']['aws']['ec2']['instance_type']
ec2_instance_count = data['services']['aws']['ec2']['instance_count']
s3_buckets = data['services']['aws']['s3']['buckets']
lambda_function_count = data['services']['aws']['lambda']['function_count']
print(f"AWS Region: {aws_region}")
print(f"EC2 Instance Type: {ec2_instance_type}, Count: {ec2_instance_count}")
print(f"S3 Buckets: {', '.join(s3_buckets)}")
print(f"Lambda Function Count: {lambda_function_count}")
else:
print("aws_config.yaml file not found!")
By mastering these file formats and understanding how to parse them using Python, you’ll be well-prepared to handle the configuration of cloud resources, manage infrastructure, and automate tasks as a DevOps engineer.
Subscribe to my newsletter
Read articles from Muzammil Jan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Muzammil Jan
Muzammil Jan
Software Engineering student at Dha Suffa University, Karachi. Exploring the world of DevOps & Cloud! Love learning & giving back to open source communities. Connect with me on https://www.linkedin.com/in/muzammiljan/