Activity 25 : Research Use Cases of Python Dictionaries Data Structures
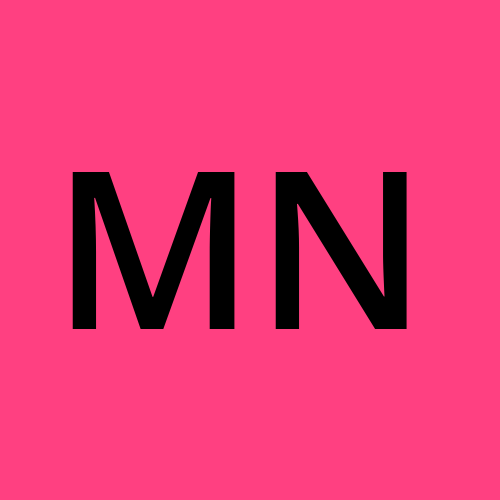
A dictionary in Python is an unordered, mutable, and indexed collection of key-value pairs. Dictionaries are widely used in programming due to their ability to quickly retrieve, update, and organize data based on unique keys. Below are some of the most common and important use cases for dictionaries in Python, accompanied by detailed explanations and code examples.
1. Mapping and Storing Key-Value Pairs
Use Case: When you need to associate values with unique keys for easy lookup.
Example:
student_grades = { "Alice": 85, "Bob": 90, "Charlie": 95 } # Accessing a value by key print(student_grades["Alice"]) # Output: 85
Explanation: Dictionaries are perfect for storing and mapping data where each key corresponds to a unique value. In the example above, student names are the keys, and their respective grades are the values. The key can be used to quickly retrieve the associated value.
Common Operations:
Adding or updating a key-value pair:
student_grades["David"] = 88 student_grades["Alice"] = 87 # Update Alice's grade print(student_grades) # Output: {'Alice': 87, 'Bob': 90, 'Charlie': 95, 'David': 88}
Checking if a key exists:
if "Charlie" in student_grades: print("Charlie's grade:", student_grades["Charlie"]) # Output: Charlie's grade: 95
2. Storing Configuration or Settings
Use Case: When you need to store configuration options or settings in a structured way.
Example:
config = { "theme": "dark", "language": "English", "auto_save": True } # Accessing settings print(config["theme"]) # Output: dark
Explanation: Dictionaries are useful for representing configuration settings. In the example, the
config
dictionary stores application settings such as the theme, language, and auto-save preference. This structure is easy to modify and can be used to configure different parts of an application.Common Operations:
Modifying settings:
config["language"] = "Spanish" print(config) # Output: {'theme': 'dark', 'language': 'Spanish', 'auto_save': True}
Setting default values:
backup_setting = config.get("backup", False) print(backup_setting) # Output: False (since "backup" does not exist in config)
3. Counting Occurrences of Items
Use Case: When you need to count how often items appear in a collection.
Example:
words = ["apple", "banana", "apple", "orange", "banana", "apple"] word_count = {} for word in words: if word in word_count: word_count[word] += 1 else: word_count[word] = 1 print(word_count) # Output: {'apple': 3, 'banana': 2, 'orange': 1}
Explanation: Dictionaries are highly efficient for counting occurrences of items. In the above example, the
word_count
dictionary tracks how often each word appears in thewords
list. Every word is used as a key, and its value is the number of times it appears.Common Operations:
Using the
collections.Counter
class (an optimized version):from collections import Counter word_count = Counter(words) print(word_count) # Output: Counter({'apple': 3, 'banana': 2, 'orange': 1})
4. Grouping Data by a Common Key
Use Case: When you need to group data based on some attribute or key.
Example:
employees = [ {"name": "Alice", "department": "HR"}, {"name": "Bob", "department": "Engineering"}, {"name": "Charlie", "department": "Engineering"}, {"name": "David", "department": "HR"} ] department_groups = {} for employee in employees: dept = employee["department"] if dept not in department_groups: department_groups[dept] = [] department_groups[dept].append(employee["name"]) print(department_groups) # Output: {'HR': ['Alice', 'David'], 'Engineering': ['Bob', 'Charlie']}
Explanation: In this example, dictionaries are used to group employees by department. The department names serve as keys, and each key holds a list of employee names. This technique is especially useful when you want to organize or categorize data based on a shared attribute.
5. Caching and Memoization
Use Case: Optimizing expensive function calls by caching previously computed results.
Example:
fibonacci_cache = {} def fibonacci(n): if n in fibonacci_cache: return fibonacci_cache[n] if n == 0: value = 0 elif n == 1: value = 1 else: value = fibonacci(n-1) + fibonacci(n-2) fibonacci_cache[n] = value return value print(fibonacci(10)) # Output: 55
Explanation: Dictionaries can be used to cache the results of expensive function calls to avoid redundant computations. In the example above, the Fibonacci sequence is computed and stored in the
fibonacci_cache
dictionary, speeding up future requests for the same Fibonacci number.Common Operations:
- Storing computed values: The result of each computation is stored in the dictionary with the function input (
n
) as the key and the computed value as the value.
- Storing computed values: The result of each computation is stored in the dictionary with the function input (
6. Representing Objects or Entities
Use Case: Representing entities like users or products with multiple attributes.
Example:
user = { "name": "Alice", "age": 30, "email": "alice@example.com" } # Accessing attributes print(user["name"]) # Output: Alice
Explanation: Dictionaries are excellent for representing objects or entities with multiple attributes. In this example, the
user
dictionary contains the user's name, age, and email. This structure mirrors how JSON objects are represented and is often used in web development and APIs.Common Operations:
Adding new attributes:
user["phone"] = "123-456-7890" print(user) # Output: {'name': 'Alice', 'age': 30, 'email': 'alice@example.com', 'phone': '123-456-7890'}
7. Efficient Lookups in Large Datasets
Use Case: When you need fast, constant-time lookups for data retrieval.
Example:
inventory = { "item_001": {"name": "Laptop", "price": 1200}, "item_002": {"name": "Smartphone", "price": 800}, "item_003": {"name": "Tablet", "price": 500} } item = inventory.get("item_002", "Item not found") print(item) # Output: {'name': 'Smartphone', 'price': 800}
Explanation: Dictionaries provide an efficient way to look up values based on keys, especially when working with large datasets. In the example, each product in an inventory is identified by a unique product ID, and looking up an item by its ID is fast and direct, regardless of the size of the dataset.
8. Handling JSON Data
Use Case: When working with JSON data from APIs or configuration files.
Example:
import json json_data = '{"name": "Alice", "age": 30, "city": "New York"}' user = json.loads(json_data) print(user["name"]) # Output: Alice
Explanation: Python dictionaries are often used when dealing with JSON data because JSON objects map directly to Python dictionaries. In the example, the
json.loads()
method converts a JSON string into a Python dictionary, allowing easy access to the values.Common Operations:
Converting dictionaries to JSON:
json_output = json.dumps(user)
print(json_output) # Output: {"name": "Alice", "age": 30, "city": "New York"}
Reference
https://www.freecodecamp.org/news/python-dictionary-data-structure-explained/
https://www.dataquest.io/blog/python-dictionaries/
Subscribe to my newsletter
Read articles from Monette Nicolas directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
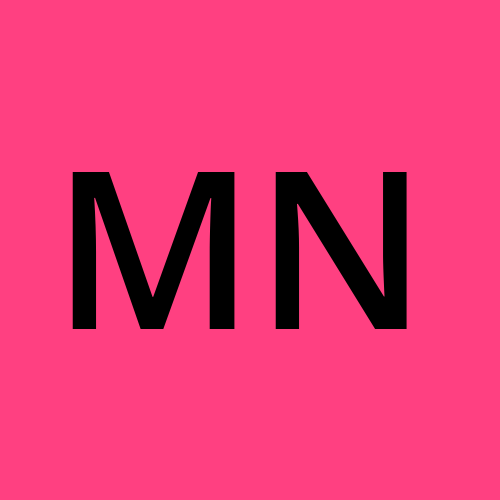