Activity 31: Python Flask Data Structure
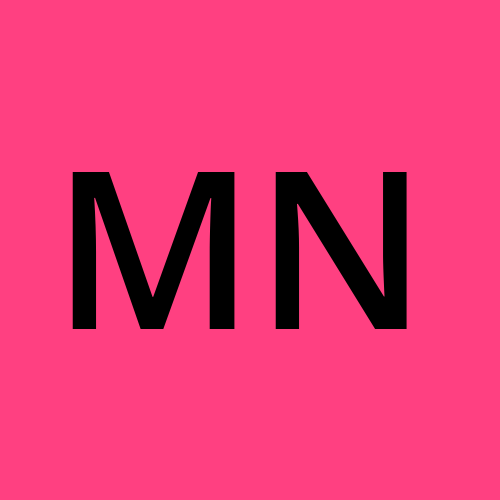
Project Structure
The index.html file serves as the user interface for managing a student list within the web application. It features a form designed for adding new students, along with a table that displays the list of students, complete with headers for organization.
Each entry in the table includes actions that allow users to interact with the student data effectively, such as editing names, deleting student records, and selecting specific students for further actions.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<h1>Add Student</h1>
<form method="POST" action="/add">
<input type="text" name="name" placeholder="Input Student" required >
<button type="submit">Add</button>
</form>
<br>
<br>
<table>
<thead>
<tr>
<th>index</th>
<th>Name</th>
<th>Action</th>
</tr>
</thead>
<tbody>
{% for index, student in students %}
<tr>
<td>{{index}}</td>
<td>{{student}}</td>
<td >
<form method="POST" action="/edit_student/{{ index }}">
<input type="text" name="new_name" value="{{student}}" required/>
<button type="submit">Submit</button>
<a href="/delete/{{student}}" >Delete With Student Name</a>
<a href="/delete-with-index/{{index}}">Delete With Index</a>
<a href="/select/{{student}}">Select Student</a>
</form>
</td>
</tr>
{% endfor %}
</tbody>
</table>
</body>
</html>
Importing Flask Library
- This code snippet initializes a Flask web application by importing components from the Flask library, instantiating the
Flask
class, and creating the main application object, enabling developers to define routes, handle requests, and manage URL redirection.
from flask import Flask, render_template, request, redirect, url_for;
app = Flask(__name__)
student_name (List Data Structure)
- The
student_name
variable in Python is a list data structure that stores a sequence of student names, allowing easy manipulation and retrieval. Its order and flexibility make it an efficient management tool.
student_name = ["Spongebob", "Jimmy Neutron", "Alice"]
Fetch Student List Function
- The
fetch_student_list
function in a Flask web application generates a list of student names and indices using theenumerate
function. It then creates astudent_with_index
list, which is displayed dynamically on the webpage using an HTML template.
@app.route('/')
def fetch_student_list():
student_with_index = list(enumerate(student_name))
return render_template('index.html', students = student_with_index)
add Student Function
- The
add_student
function in Flask handles POST requests for adding students. It retrieves the student’s name from the form data, appends it to thestudent_name
list, and then redirects the user to thefetch_student_list
route for immediate updates.
Copy@app.route('/add', methods=['POST'])
def add_student():
name = request.form.get('name')
if name:
student_name.append(name)
return redirect(url_for('fetch_student_list'))
Delete Student with name Function
- The
delete_student
function is a Flask route handler that deletes a student from thestudent_name
list using a URL parameter. It retrieves the name and removes it, then redirects the user back to thefetch_student_list
route.
@app.route('/delete/<string:name>')
def delete_student(name):
student_name.remove(name)
return redirect(url_for('fetch_student_list'))
Select Student
- The
selected_student
function is a Flask route handler that selects a student by name using a URL parameter. It retrieves the student’s name and prints it to the console, allowing debugging or logging purposes. The function could be expanded for more details.
Copy@app.route('/select/<string:name>')
def selected_student(name):
print("selected " , name);
return redirect(url_for('fetch_student_list'))
Edit Students
- The
edit_student
function in Flask allows users to update a student’s name in thestudent_name
list based on their index. It retrieves a new name from the submitted form and updates it at the specified index, ensuring seamless user interaction.
@app.route('/edit_student/<int:index>', methods = ['POST'])
def edit_student(index):
new_name = request.form.get("new_name")
student_name[index] = new_name
return redirect(url_for('fetch_student_list'))
running the python code including the index
The if __name__ == ‘__main__’:
ensures code runs directly when executed, not as a module. It starts Flask web application with app.run
(debug=True)
, enabling debug mode for development, enabling troubleshooting and local testing.
if __name__ == '__main__':
app.run(debug=True)
This is the complete code for “app.py.”
from flask import Flask, render_template, request, redirect, url_for;
app = Flask(__name__)
student_name = ["Spongebob", "Jimmy Neutron", "Alice"]
@app.route('/')
def fetch_student_list():
student_with_index = list(enumerate(student_name))
return render_template('index.html', students = student_with_index)
@app.route('/add', methods=['POST'])
def add_student():
name = request.form.get('name')
if name:
student_name.append(name)
return redirect(url_for('fetch_student_list'))
@app.route('/delete/<string:name>')
def delete_student(name):
student_name.remove(name)
return redirect(url_for('fetch_student_list'))
@app.route('/delete-with-index/<int:index>')
def delete_student_with_index(index):
student_name.pop(index)
return redirect(url_for('fetch_student_list'))
@app.route('/select/<string:name>')
def selected_student(name):
print("selected " , name);
return redirect(url_for('fetch_student_list'))
@app.route('/edit_student/<int:index>', methods = ['POST'])
def edit_student(index):
new_name = request.form.get("new_name")
student_name[index] = new_name
return redirect(url_for('fetch_student_list'))
if __name__ == '__main__':
app.run(debug=True)
After creating the “index.html” and “app.py” files, the web application will enable users to manage a student list effectively. Users can view, add, edit, select, and delete student names, with the application dynamically updating the list. This setup ensures a smooth and interactive experience for managing student information.
After developing the app.py and index.html files, the next step is to upload them to GitHub. This involves initializing a Git repository, staging the files, committing the changes, linking to a new GitHub repository, and pushing the changes to make the code accessible and version-controlled.
Link to my GitHub Repository
https://github.com/MonetForProgrammingPurposes/Python-Flask-Data-Structure
Subscribe to my newsletter
Read articles from Monette Nicolas directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
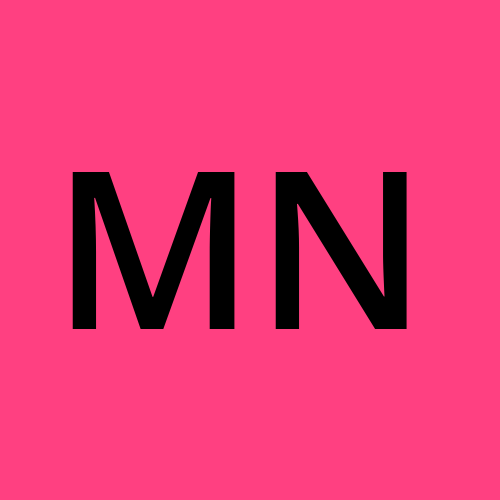