🚀 Understanding React-Redux: A Beginner's Guide to State Management
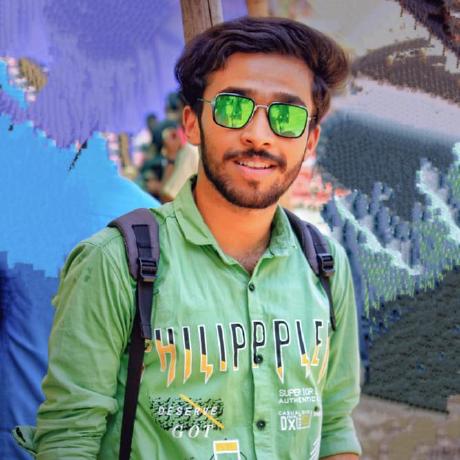
In the world of React, managing state efficiently is key to building scalable and maintainable applications. That's where React-Redux comes in—a powerful tool that helps you handle complex state management with ease.
đź§ What is Redux?
Redux is a predictable state container for JavaScript apps, designed to manage the state of your entire application in a single store. It's especially useful for large-scale apps where managing state across components can become tricky.
đź”— Why React-Redux?
React-Redux is the official binding library that allows your React components to interact with the Redux store. It provides a clean, declarative API to connect your components with the Redux state, ensuring a smoother developer experience.
đź› Key Concepts:
Store: The single source of truth that holds your entire application’s state.
Actions: Plain JavaScript objects that describe what happened.
Reducers: Pure functions that take the current state and action, returning the next state.
Dispatch: The method used to send actions to the store.
Selectors: Functions that allow you to extract specific pieces of state from the store.
⚙️ Getting Started with React-Redux
Let’s break down the steps to integrate Redux with a React app:
Install Redux and React-Redux:
npm install redux react-redux
Create a Redux Store:
javascriptCopy codeimport { createStore } from 'redux'; import rootReducer from './reducers'; const store = createStore(rootReducer);
Wrap Your App with the
Provider
:javascriptCopy codeimport { Provider } from 'react-redux'; import store from './store'; function App() { return ( <Provider store={store}> <YourMainComponent /> </Provider> ); }
Connect Components to the Store:
javascriptCopy codeimport { useSelector, useDispatch } from 'react-redux'; const MyComponent = () => { const stateValue = useSelector((state) => state.someValue); const dispatch = useDispatch(); return ( <div> <p>{stateValue}</p> <button onClick={() => dispatch({ type: 'SOME_ACTION' })}> Update Value </button> </div> ); };
🚀 Advantages of React-Redux
Predictable State: You have a clear structure and process for handling application state.
Time-Travel Debugging: Redux DevTools allows you to step through dispatched actions and see how your state evolves over time.
Easier Testing: Pure functions in reducers make unit testing straightforward.
Code Organization: Keeps your business logic separate from UI components.
đź’ˇ Final Thoughts
React-Redux is a great tool for managing the state of larger applications, but it may be overkill for smaller apps. If you’re dealing with deeply nested state or shared data across multiple components, React-Redux can streamline your workflow and help you maintain cleaner, more scalable code.
Subscribe to my newsletter
Read articles from Supratim Dey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
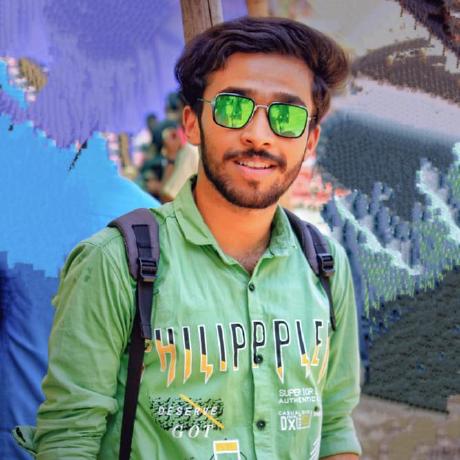