Day 3: Data Types Party ๐
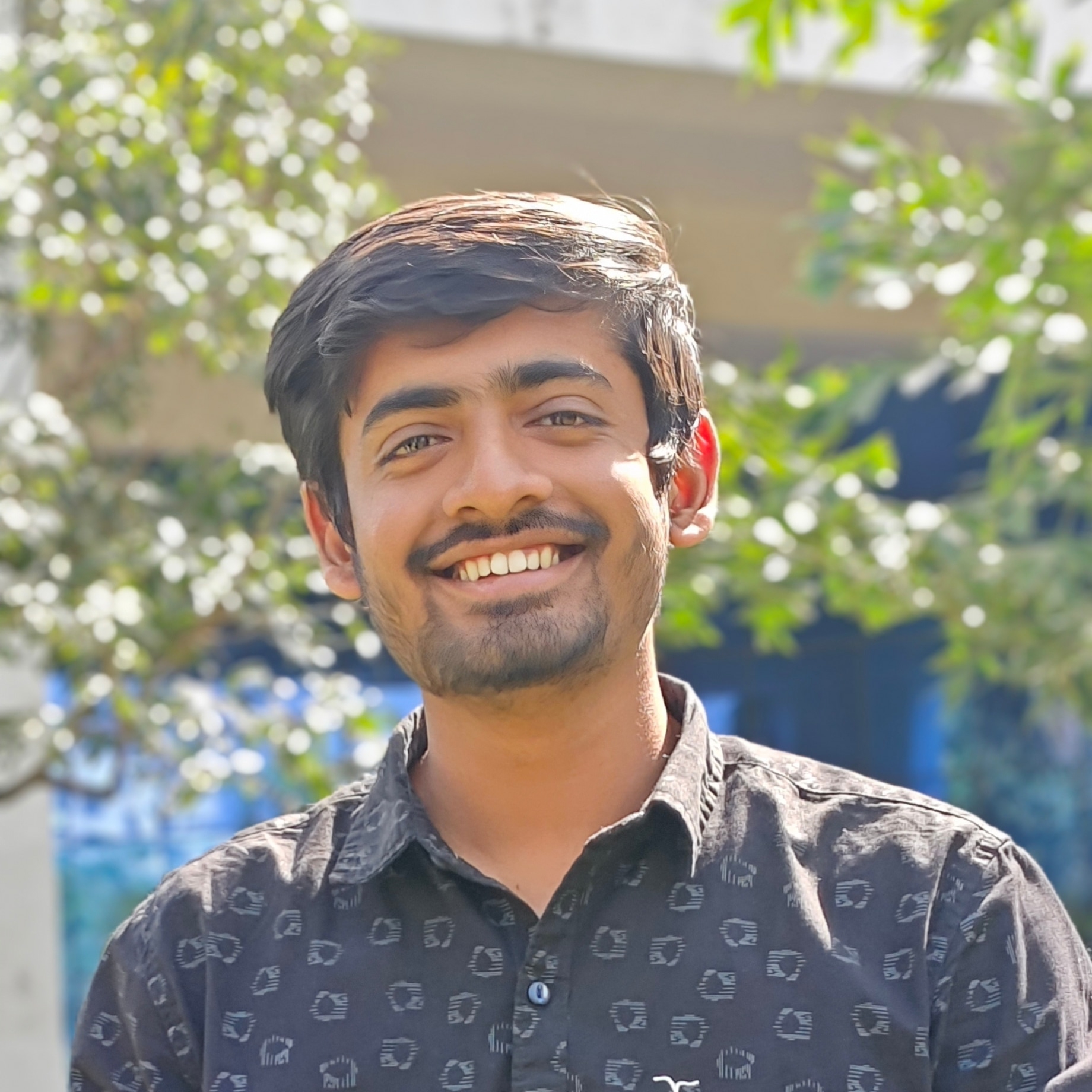
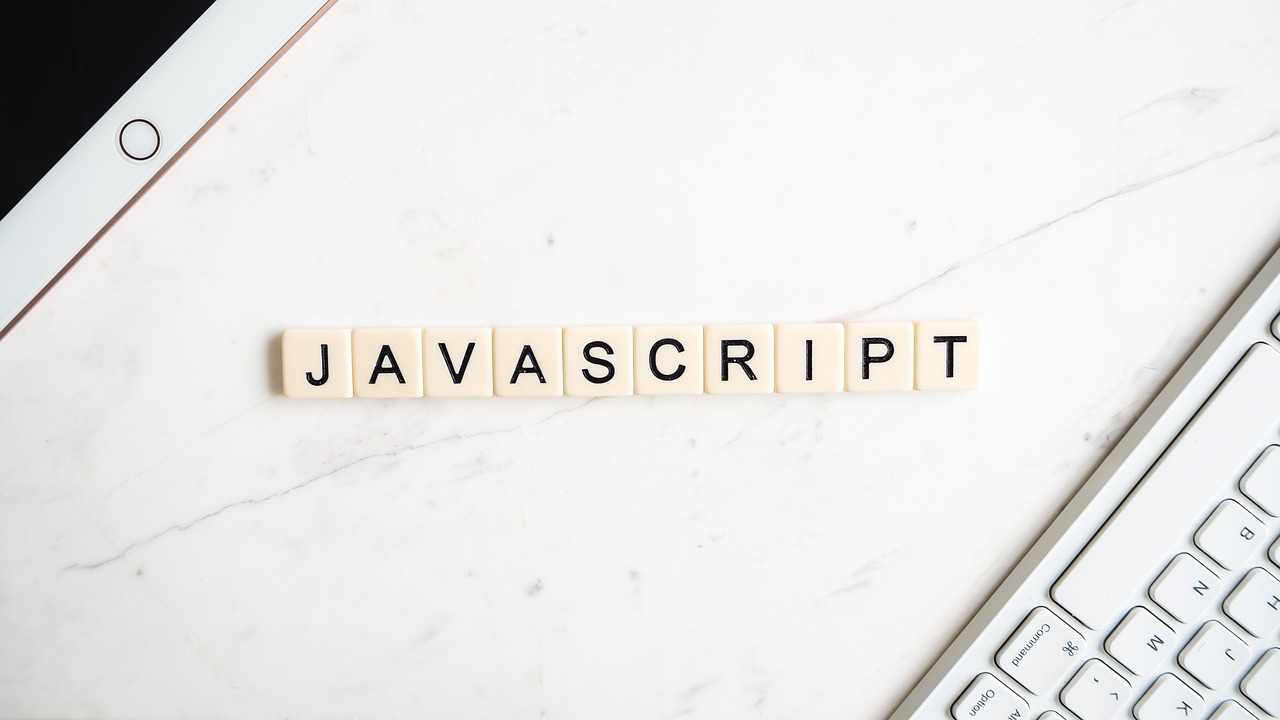
JavaScript has many guests at this party! Meet Numbers, Strings, Booleans, Arrays, and Objects. Today, you'll learn what makes each guest special and how to interact with them through code. Thereโs also a game at the end, where you'll guess the data type of some fun code snippets!
1. Meet the Guests (Data Types) ๐บ
1๏ธโฃ Numbers: The Math Whiz ๐งฎ
- Numbers love calculations! They can handle things like addition, subtraction, multiplication, and division.
javascriptCopy codelet age = 25; // Number
let pi = 3.14159; // Another Number
console.log(age + 5); // Output: 30 ๐
๐ก Fun Fact: You can even have negative and decimal numbers.
2๏ธโฃ Strings: The Party Singer ๐ค
- Strings are all about text. They hold words, sentences, and even emojis! ๐ธ
javascriptCopy codelet greeting = "Hello, world! ๐";
let favoriteHero = 'Spiderman ๐ธ๏ธ';
console.log(greeting); // Output: Hello, world! ๐
๐ก Pro Tip: Strings can be written with single quotes ('') or double quotes ("").
3๏ธโฃ Booleans: The Bouncer at the Door ๐ช
- Booleans can only say Yes (true) or No (false). They're perfect for making decisions.
javascriptCopy codelet isDoorOpen = true; // Yes, the door is open ๐ช
let isNightTime = false; // No, itโs not nighttime ๐
console.log(isDoorOpen); // Output: true
๐ก Fun Fact: Booleans control if-else logic in programs โ they decide what happens next!
4๏ธโฃ Arrays: The DJ with a Playlist ๐ง
- Arrays love to store lists. Think of them as your partyโs playlist of songs or guests.
javascriptCopy codelet favoriteSongs = ["Blinding Lights", "Levitating", "Good 4 U"];
console.log(favoriteSongs[0]); // Output: Blinding Lights ๐ต
๐ก Pro Tip: Arrays start counting at 0! The first item is at index 0.
5๏ธโฃ Objects: The Cool Organizer ๐๏ธ
- Objects can hold a bunch of related information in one place. Think of them as a guestโs profile at the party โ name, age, and hobbies, all bundled together!
javascriptCopy codelet spiderman = {
name: "Peter Parker",
age: 18,
powers: ["Wall-crawling", "Spider-sense", "Web-slinging"]
};
console.log(spiderman.name); // Output: Peter Parker ๐ท๏ธ
๐ก Pro Tip: Objects store key-value pairs, making them perfect for detailed information.
2. Game Time: Spot the Data Type! ๐ฒ
In this game, Iโll show you some mysterious code snippets. Your task is to guess the data type of the variable. Try to guess before running the code!
Round 1:
javascriptCopy codelet answer = 42;
Guess: ๐ค (Type: Number)
Answer: โ๏ธ Yes, itโs a Number! ๐
Round 2:
javascriptCopy codelet isFun = true;
Guess: ๐ค (Type: Boolean)
Answer: โ๏ธ Correct! Itโs a Boolean! ๐ฅ
Round 3:
javascriptCopy codelet hero = "Wonder Woman";
Guess: ๐ค (Type: String)
Answer: โ๏ธ Yes, itโs a String! ๐ค
Round 4:
javascriptCopy codelet tools = ["Hammer", "Wrench", "Screwdriver"];
Guess: ๐ค (Type: Array)
Answer: โ๏ธ You got it โ an Array! ๐ ๏ธ
Round 5:
javascriptCopy codelet car = { brand: "Tesla", model: "Model 3", electric: true };
Guess: ๐ค (Type: Object)
Answer: โ๏ธ Correct! Itโs an Object! ๐
3. Bonus Challenge: Build a Guest List ๐ฏ
Your task: Create a guest list for a party using an array and an object.
Store three guest names in an array.
Create an object for one of the guests, including their name, age, and favorite activity.
Sample Code:
javascriptCopy code// Array of guest names
let guests = ["Tony Stark", "Bruce Banner", "Natasha Romanoff"];
// Object for one guest
let guestProfile = {
name: "Tony Stark",
age: 45,
favoriteActivity: "Building suits"
};
console.log(`Guest: ${guestProfile.name}, Age: ${guestProfile.age}`);
4. Recap: What Did You Learn Today?
Numbers: For doing math and keeping track of ages.
Strings: For holding text and conversation.
Booleans: For making decisions with true/false.
Arrays: For storing lists like playlists or guest names.
Objects: For bundling information, like a guest's profile.
5. Final Challenge: Create Your Own Data Party ๐
Make a playlist array with 5 songs.
Create an object for yourself with your name, age, and three hobbies.
Use
console.log
to print everything!
Example:
javascriptCopy codelet myPlaylist = ["Shape of You", "Peaches", "Rockstar"];
let myProfile = {
name: "Risharth",
age: 22,
hobbies: ["Coding", "Watching Anime", "Reading Manga"]
};
console.log(myPlaylist);
console.log(`Hey! I'm ${myProfile.name}, and I love ${myProfile.hobbies[1]}.`);
Great job! Youโve partied hard with JavaScript data types today! Tomorrow, we dive into Operators Showdown ๐คบ, where youโll learn to perform operations like a pro. ๐ฏ
Subscribe to my newsletter
Read articles from Risharth pardeshi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
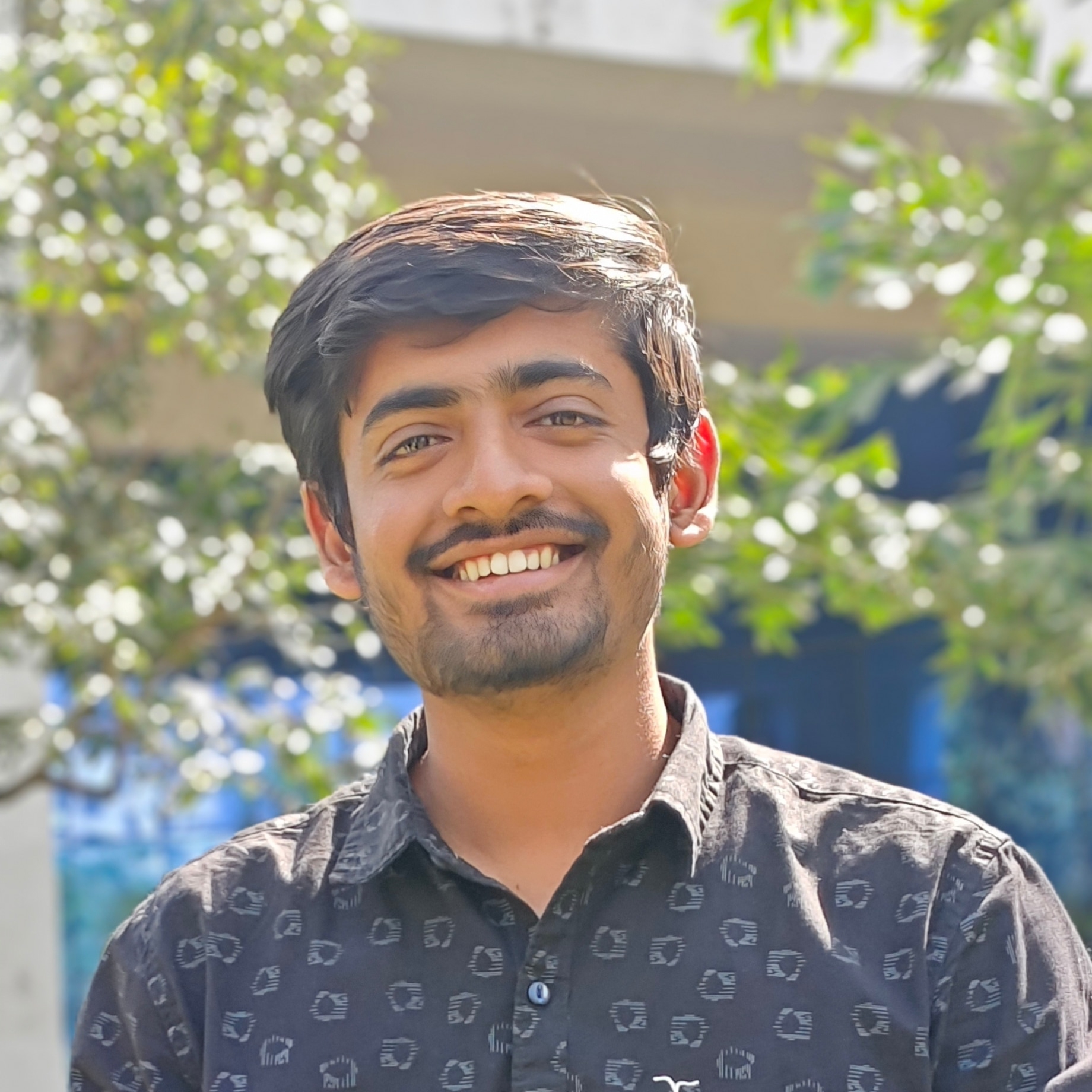
Risharth pardeshi
Risharth pardeshi
I hope this message finds you well. I am Risharth Pardeshi I am passionate about Computer Science and Engineering, and I have a strong foundation in this field. I graduated with a Bachelor of Engineering from the Laxmi Narayan College of Technology in Indore, India, in July 2024, with a CGPA of 7.98. Here is a brief overview of my technical strengths: Languages: C++, HTML, CSS, JavaScript, SQL Frameworks: React.js, Next.js, Tailwind CSS, Node.js Databases/OS: MySQL, MongoDB, Firebase, Linux Technical Skills: Data Structures and Algorithms, Git, DBMS, OS, Object-Oriented Programming, Computer Networking, Vercel, Postman