Java Messaging Service (JMS): Asynchronous Communication
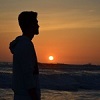
Introduction
Java Messaging Service (JMS) is an API for messaging systems that provides a standardised way for Java applications to create, send, receive, and read messages. It enables asynchronous communication between applications, allowing them to exchange data without blocking each other.
Core Concepts
Message: A unit of data that is exchanged between applications.
Message Producer: An application that creates and sends messages.
Message Consumer: An application that receives and processes messages.
Message Broker: A server that acts as an intermediary between producers and consumers, storing and forwarding messages.
Destination: A logical endpoint for messages, such as a queue or topic.
Message Destinations
Queue: A point-to-point destination where messages are delivered to one consumer at a time.
Topic: A publish-subscribe destination where messages are delivered to all interested consumers.
Message Properties
Headers: Standardised properties that provide information about the message, such as the destination, sender, and timestamp.
Body: The actual data content of the message.
Properties: Custom properties that can be added to the message to provide additional context or metadata.
JMS API
The JMS API provides interfaces for message producers, consumers, destinations, and message factories. It also defines message types (TextMessage, BytesMessage, etc.) and message delivery modes (PERSISTENT, NON_PERSISTENT).
Example: Using JMS for Asynchronous Communication
Java
import javax.jms.*;
public class JMSExample {
public static void main(String[] args) {
try {
// Create a connection factory
InitialContext ctx = new InitialContext();
ConnectionFactory connectionFactory = (ConnectionFactory) ctx.lookup("ConnectionFactory");
// Create a connection
Connection connection = connectionFactory.createConnection();
connection.start();
// Create a session
Session session = connection.createSession(false, Session.AUTO_ACKNOWLEDGE);
// Create a destination (queue or topic)
Destination destination
= (Destination) ctx.lookup("myQueue");
// Create a message producer
MessageProducer producer = session.createProducer(destination);
// Create a text message
TextMessage message = session.createTextMessage("Hello, JMS!");
// Send the message
producer.send(message);
// Close resources
producer.close();
session.close();
connection.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Benefits of JMS
Asynchronous communication: Improves application responsiveness and scalability.
Decoupling: Separates components, making them easier to develop, test, and maintain.
Reliability: Ensures message delivery even in case of network failures.
Scalability: Handles high message volumes and can be distributed across multiple servers.
Flexibility: Supports different messaging patterns and use cases.
JMS Implementations
ActiveMQ: An open-source JMS broker from Apache Software Foundation.
RabbitMQ: Another popular open-source message broker.
IBM MQ: A commercial JMS broker from IBM.
Conclusion
JMS provides a powerful and flexible way for Java applications to communicate asynchronously. By understanding the core concepts and using the JMS API effectively, developers can build robust and scalable messaging systems. For those in Delhi looking to further their expertise, a Java training institute in Noida, Delhi, Pune and other Indian cities can be a great resource to explore these concepts in depth.
Subscribe to my newsletter
Read articles from Sanjeet Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
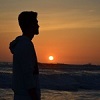
Sanjeet Singh
Sanjeet Singh
I am Sanjeet Singh, an IT professional with experience in the IT sector. I have a broad understanding of Data Analytics and proficiency across multiple layers of software development and testing, from the front end to the back end.