π Understanding Truthy and Falsy Values in JavaScript π€

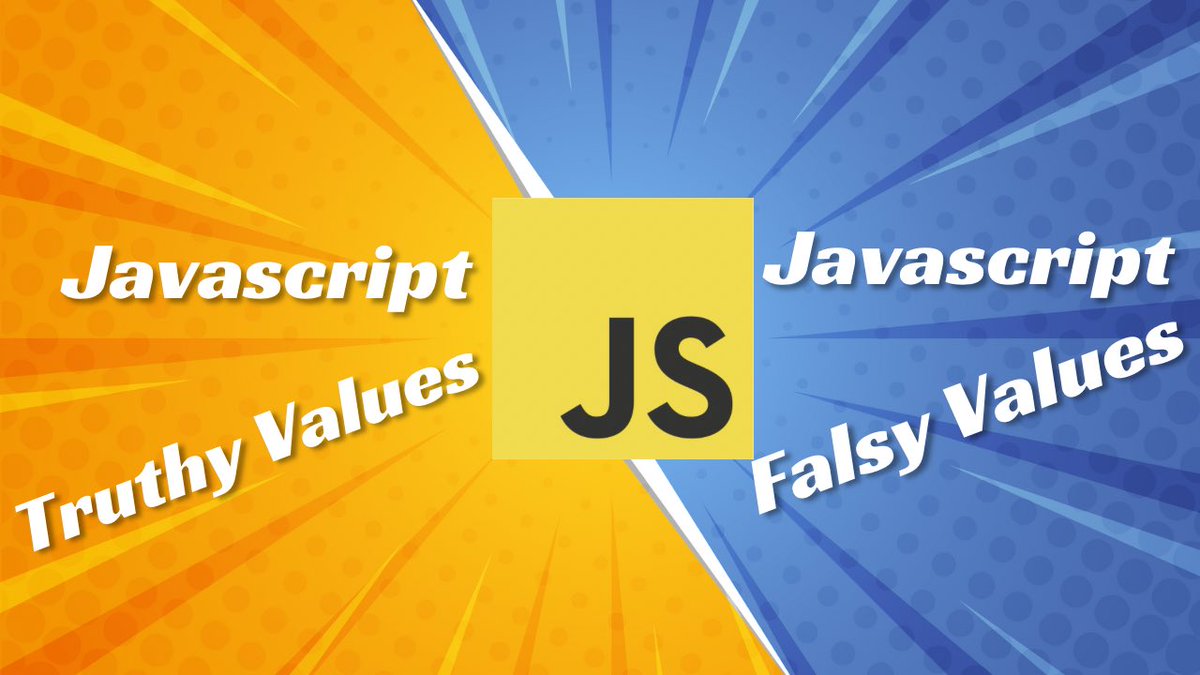
JavaScript is known for its quirks, and one of its interesting features is the concept of truthy and falsy values. Understanding these can make your code more efficient and elegant, allowing you to write cleaner logic with fewer bugs. Letβs dive into what these terms mean and explore some practical use cases. π‘
What Are Truthy and Falsy Values? π€
In JavaScript, a value is considered truthy if it evaluates to true
when converted to a Boolean. Conversely, a value is falsy if it evaluates to false
in a Boolean context.
Falsy Values in JavaScript β
There are only a few values that JavaScript considers falsy:
false
β0
(zero) β""
or''
(empty strings) πnull
π«undefined
βNaN
(Not a Number) π«π’
When any of these values are used in conditions, they are treated as false
. For example:
if (0) { console.log("This won't run"); }
Truthy Values in JavaScript βοΈ
Anything that is not falsy is considered truthy*. This includes:*
true
βAny non-zero number (e.g.,
1
,-1
) βNon-empty strings (e.g.,
"hello"
) βοΈArrays (even empty arrays
[]
) πObjects (even empty objects
{}
) π¦
For example:
if ("hello") { console.log("This will run"); }
π₯ Practical Use Cases of Truthy and Falsy Values
Knowing when a value is truthy or falsy can be incredibly useful when writing JavaScript. Here are a few scenarios where this comes in handy:
1. User Authentication Checks π
A common use case for truthy and falsy values is in determining a user's login status:
let isLoggedIn = true;
if (isLoggedIn) {
console.log("Welcome back, user!");
} else {
console.log("Please log in.");
}
Here, isLoggedIn
is a Boolean variable that checks whether a user is logged in. If itβs true
, the user is greeted; if itβs false
, theyβre prompted to log in. This pattern is widely used in authentication flows.
2. Providing Default Values Using Short-Circuiting π¬
JavaScript's ||
operator can be used to set default values for variables:
let userName = "";
console.log(userName || "Guest");
In this example, if userName
is an empty string (a falsy value), it falls back to "Guest"
. This is a handy way to ensure your application has sensible defaults.
3. Toggling UI States π
Another common use of true
and false
is toggling between states in user interfaces:
let isVisible = false;
// Toggling the state
isVisible = !isVisible;
console.log(isVisible); // Output: true
This is useful for showing or hiding elements, like modals, dropdowns, or sidebars, based on a userβs interaction.
4. Validating Form Inputs π
When building forms, you often need to validate user inputs:
let email = "";
if (!email) {
console.log("Email is required!");
}
Here, if the email
variable is an empty string (falsy), the message "Email is required!"
will be displayed, prompting the user to fill in their email.
Why It Matters π
Understanding truthy and falsy values helps avoid common pitfalls in JavaScript. It enables you to:
Write more concise and readable conditional statements. π
Use short-circuit evaluation to simplify setting default values. π
Create more user-friendly applications with proper state management. π§βπ»
By leveraging these concepts, you can make your code more robust and easier to maintain.
Conclusion π
Mastering the concept of truthy and falsy values in JavaScript is a small but powerful skill that can greatly improve the quality of your code. Whether you're handling user authentication, managing UI states, or validating inputs, knowing when to expect a truthy or falsy value will save you time and make your codebase cleaner.
Happy coding! π»β¨
Let's Connect π€
If you found this article helpful, feel free to connect with me on LinkedIn. I love discussing all things JavaScript and front-end development!
Subscribe to my newsletter
Read articles from Yasin Sarkar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Yasin Sarkar
Yasin Sarkar
Front-End Developer. I create dynamic web applications using HTML, Tailwind CSS, JavaScript, React, and Next.js. I share my knowledge on social media to help others enhance their tech skills. An Open Source Enthusiast and Writer.