Basic Accordion
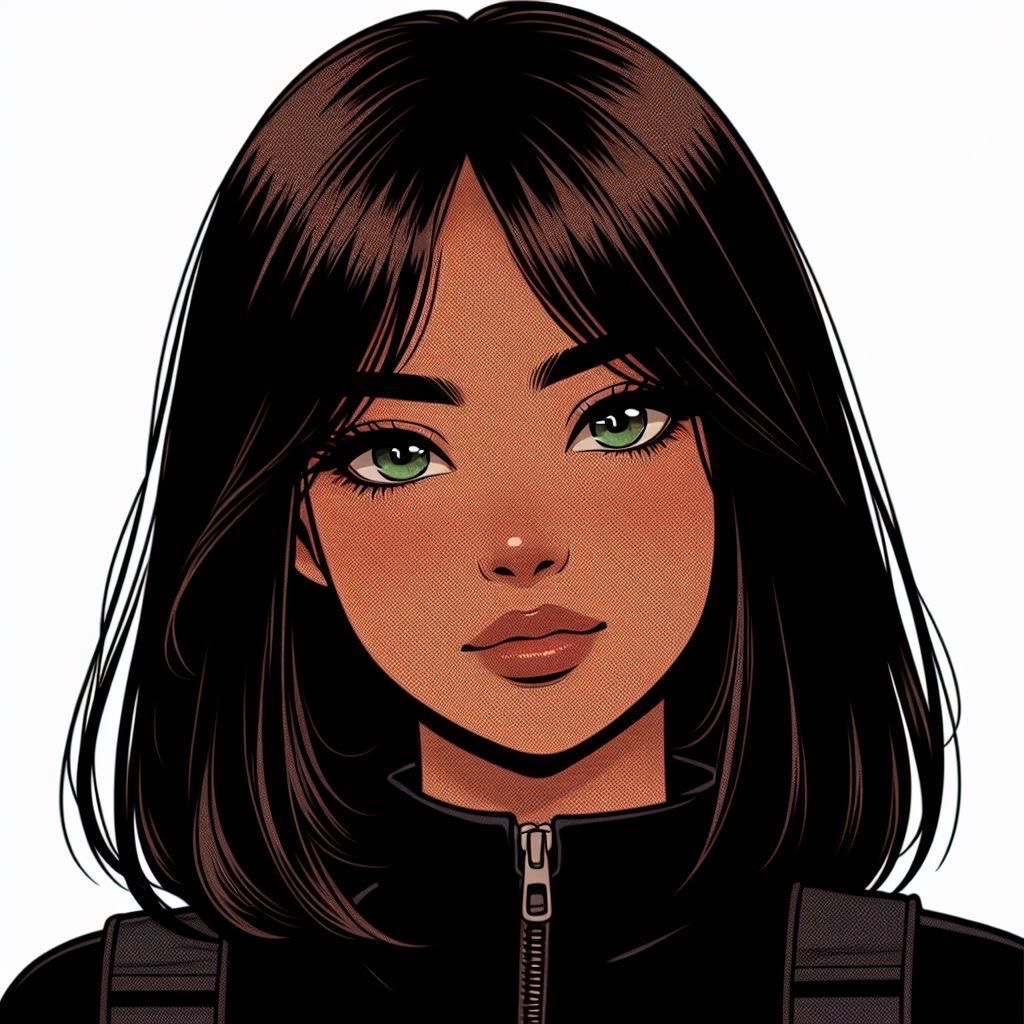
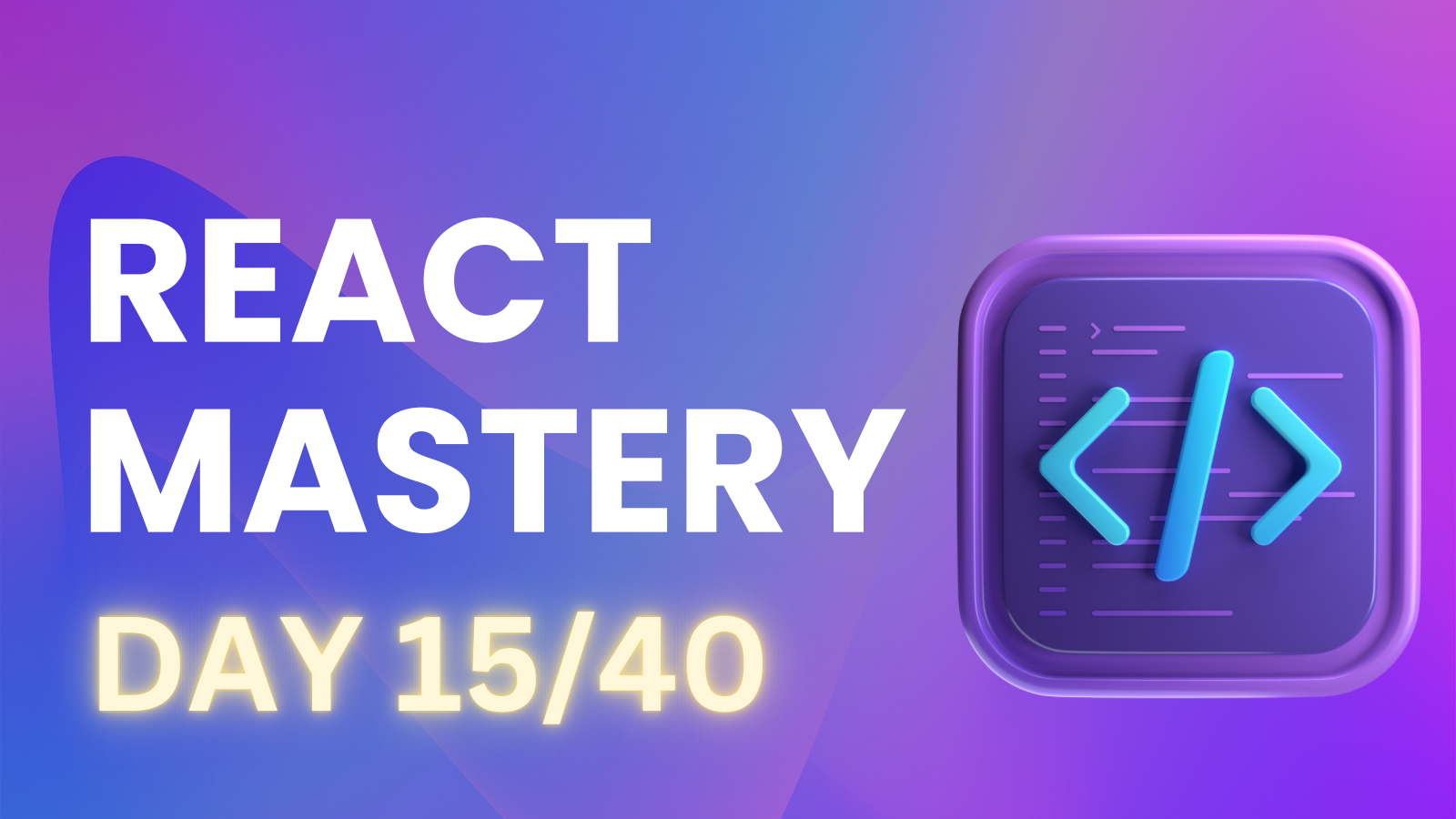
For the 15th day of my React journey, I decided to create a simple accordion. It was a fun exercise that helped me practice how to manage state and conditional rendering in React. Let me walk you through what I did.
Components/accordion/data.js
First, I created a file called data.js
where I stored an array of objects. Each object had an id
, a question
, and an answer
. The purpose was to use this data to populate my accordion.
Here's how the data.js
file looked:
const data = [
{
id: 1,
question: "Which Planet?",
answer: "Earth"
},
{
id: 2,
question: "Which Continent?",
answer: "Asia"
},
{
id: 3,
question: "Which Country?",
answer: "Japan"
},
];
export default data;
Components/accordion/index.jsx
Next, I created a component named Accordion
. Initially, this component was pretty basic, just containing a title:
export default function Accordion() {
return(
<div>
<h1>Accordion</h1>
</div>
);
}
This component acted as the main wrapper for the accordion functionality.
app.jsx
The most interesting part was where I integrated everything in app.jsx
. Here's what I did:
Importing the Necessary Files: I imported the
Accordion
component, the data, and some CSS for styling:import './App.css'; import Accordion from './components/accordion'; import data from './components/accordion/data'; import { useState } from 'react'; import './components/accordion/styles.css';
State Management: I used React's
useState
to keep track of which accordion item was selected:const [selected, setSelected] = useState([]);
Handle Selection: I created a function
handleSingleSelection
to handle which item is expanded or collapsed:function handleSingleSelection(getCurrentId) { setSelected(getCurrentId === selected ? null : getCurrentId); }
Rendering the Accordion Items: Using
.map()
, I looped through thedata
and displayed each question. When a question is clicked, it toggles to show or hide the answer:return ( <> <div className='wrapper'> <div className='accordion'> {data && data.length > 0 ? ( data.map((item) => ( <div key={item.id} className='items'> <div onClick={() => handleSingleSelection(item.id)} className='title'> <h3>{item.question}</h3> <span>+</span> </div> { selected === item.id ? <div> <h3>{item.answer}</h3> </div> : null } </div> )) ) : (<div>No data</div>) } </div> </div> </> );
What I Learned
Creating this accordion taught me several things:
State Management: Using
useState
to track which item is expanded helped me understand how to manage state for individual components.Conditional Rendering: I learned how to show or hide parts of a component based on a condition. Here, I used
selected ===
item.id
to decide whether to display the answer.
Overall, this was a simple yet valuable exercise that enhanced my understanding of React components and state.
Subscribe to my newsletter
Read articles from Aaks directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
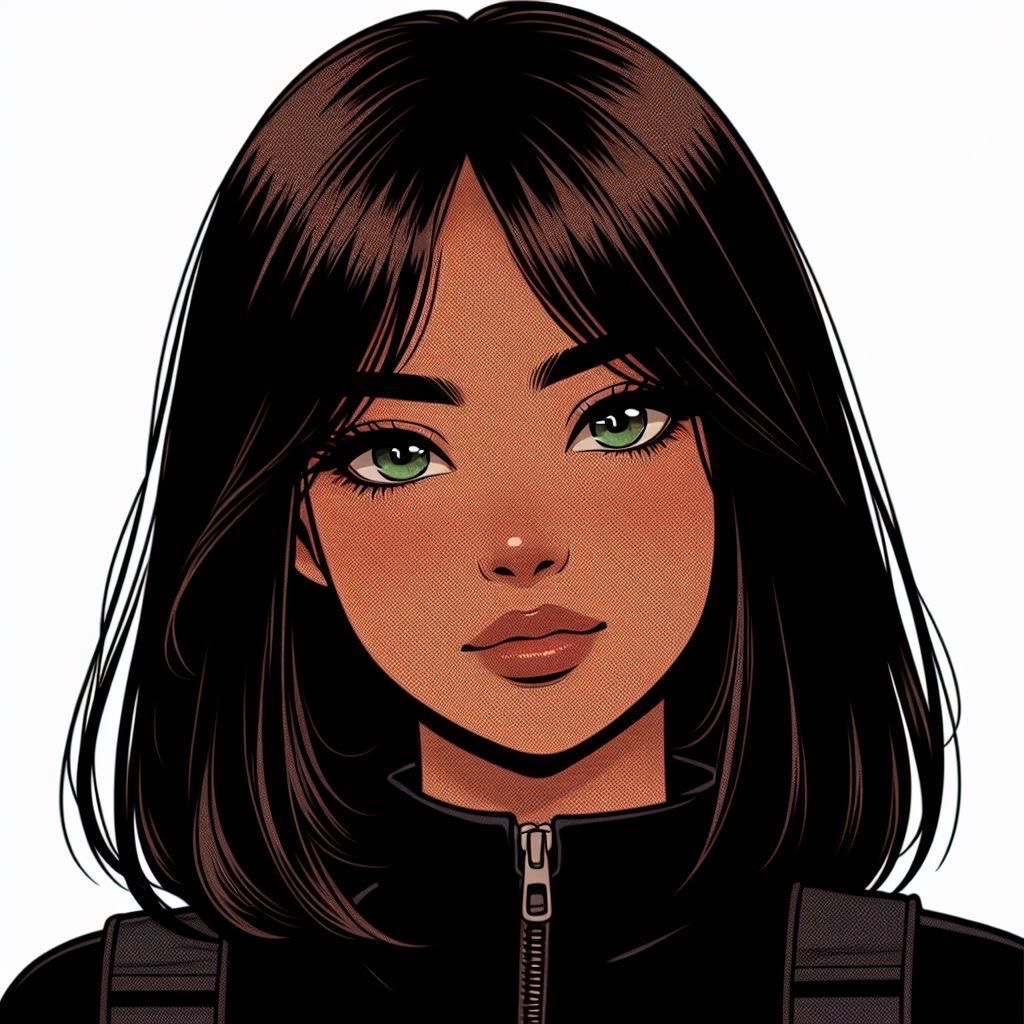