Automating AWS Resource Listing with a Simple Bash Script

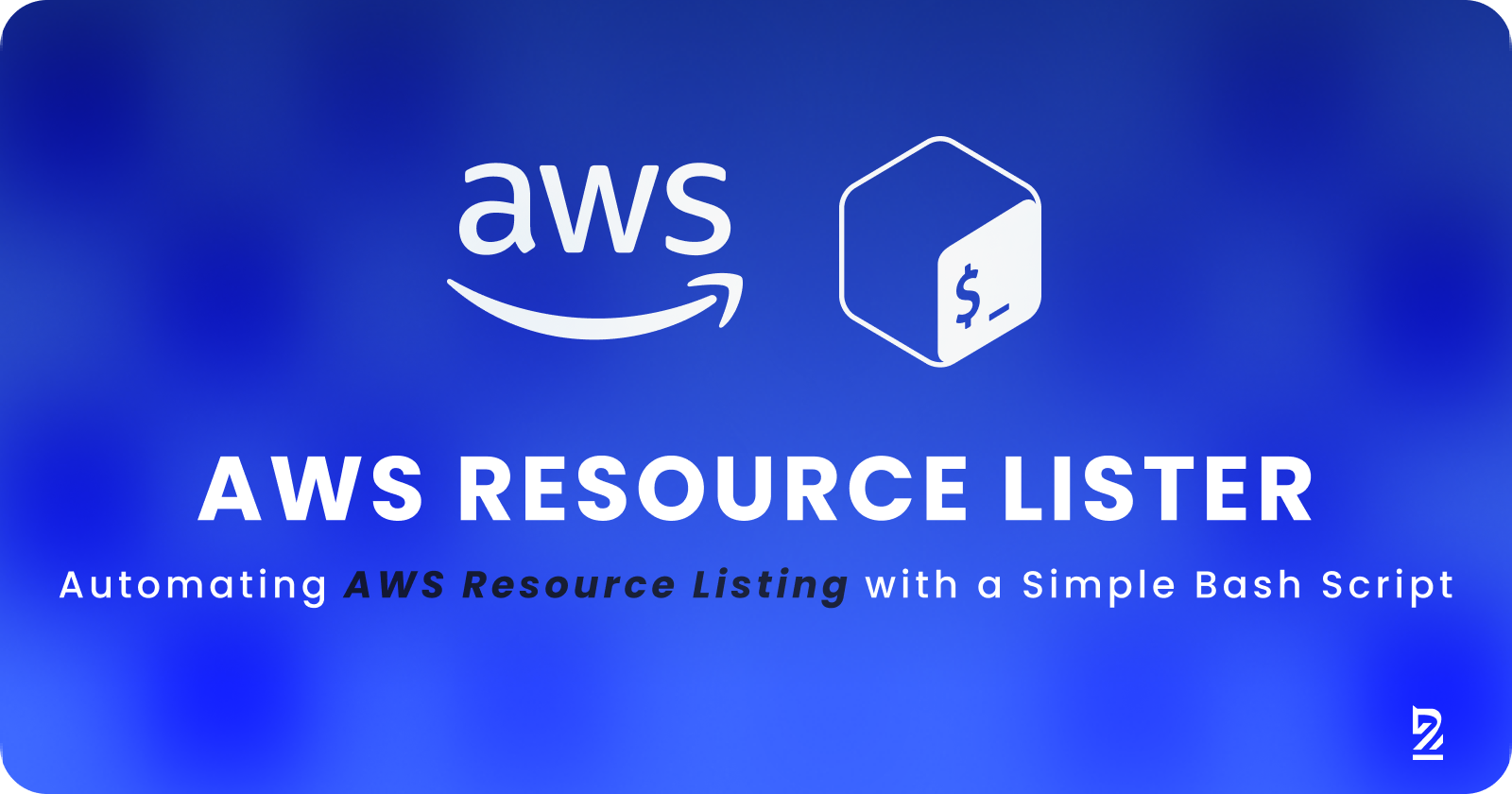
Managing AWS resources across multiple services and regions can be complex, but scripting provides an excellent opportunity to streamline this process while showcasing the power of automation in the cloud. In this blog, I present a script that automates the listing of various AWS resources. It’s a great and simple project to explore scripting capabilities with cloud services such as EC2, RDS, S3, and more.
Key Features of the Script
Supports 14 AWS Services: EC2, RDS, S3, Lambda, and more.
Region-Specific: Specify the region to get localized results.
Error Handling: Ensures AWS CLI availability and configuration before proceeding.
Lightweight and Customizable: Easily extendable to include other services as needed.
Script Breakdown
#!/bin/bash
This shebang line ensures that the script is executed in the Bash shell.
if [ $# -ne 2 ]; then
echo "Usage: ./aws_resource_list.sh <aws_region> <aws_service>"
exit 1
fi
The script requires two arguments: AWS region and service. This block ensures correct input is provided before proceeding.
if ! command -v aws &> /dev/null; then
echo "Error: AWS CLI is not installed."
exit 1
fi
Checks whether the AWS CLI is installed, an essential tool to interact with AWS services from the terminal.
if [ ! -d ~/.aws ]; then
echo "Error: AWS CLI is not configured."
exit 1
fi
Ensures that AWS credentials and configurations are set up properly for the CLI to function.
case $aws_service in
ec2)
aws ec2 describe-instances --region $aws_region
;;
s3)
aws s3api list-buckets
;;
# More services...
*)
echo "Error: Invalid service '$aws_service'"
exit 1
;;
esac
This case
block executes the correct AWS CLI command based on the service provided. The script is easily extendable to include additional services, making it highly customizable.
Usage
Prerequisites:
AWS CLI installed and configured.
Appropriate IAM permissions for accessing the desired AWS resources.
Run the Script: Example to list EC2 instances in the us-east-1
region:
./aws_resource_list.sh us-east-1 ec2
Example Outputs:
- For EC2
Listing EC2 Instances in us-east-1...
- For S3
Listing S3 Buckets...
Supported AWS Services:
EC2: Retrieve running EC2 instances.
RDS: List Relational Database Service instances.
S3: Show S3 buckets across all regions.
CloudFront: List CloudFront distributions.
Lambda: Display Lambda functions in the specified region.
IAM: List IAM users.
Route53: Show DNS hosted zones.
DynamoDB: List DynamoDB tables.
And more…
Why This Script?
This project serves as a great introduction to the intersection of cloud services and scripting. It highlights how you can leverage simple scripts to explore the vast capabilities of the cloud while making AWS resource management more efficient. By automating these listings, you can quickly assess resources without navigating through the AWS Management Console, all while improving your scripting skills.
Complete Script
# Check if the required number of arguments is provided
if [ $# -ne 2 ]; then
echo "Usage: ./aws_resource_list.sh <aws_region> <aws_service>"
echo "Example: ./aws_resource_list.sh us-east-1 ec2"
exit 1
fi
# Assign command-line arguments to variables
aws_region=$1
aws_service=$2
# Check for AWS CLI availability
if ! command -v aws &> /dev/null; then
echo "Error: AWS CLI is not installed. Please install the AWS CLI and try again."
exit 1
fi
# Verify if AWS CLI is configured
if [ ! -d ~/.aws ]; then
echo "Error: AWS CLI is not configured. Please configure the AWS CLI and try again."
exit 1
fi
# Execute the corresponding AWS CLI command based on the specified service
case $aws_service in
ec2)
echo "Listing EC2 Instances in $aws_region..."
aws ec2 describe-instances --region $aws_region
;;
rds)
echo "Listing RDS Instances in $aws_region..."
aws rds describe-db-instances --region $aws_region
;;
s3)
echo "Listing S3 Buckets..."
aws s3api list-buckets
;;
cloudfront)
echo "Listing CloudFront Distributions in $aws_region..."
aws cloudfront list-distributions --region $aws_region
;;
vpc)
echo "Listing VPCs in $aws_region..."
aws ec2 describe-vpcs --region $aws_region
;;
iam)
echo "Listing IAM Users..."
aws iam list-users
;;
route53)
echo "Listing Route53 Hosted Zones..."
aws route53 list-hosted-zones
;;
cloudwatch)
echo "Listing CloudWatch Alarms in $aws_region..."
aws cloudwatch describe-alarms --region $aws_region
;;
cloudformation)
echo "Listing CloudFormation Stacks in $aws_region..."
aws cloudformation describe-stacks --region $aws_region
;;
lambda)
echo "Listing Lambda Functions in $aws_region..."
aws lambda list-functions --region $aws_region
;;
sns)
echo "Listing SNS Topics..."
aws sns list-topics
;;
sqs)
echo "Listing SQS Queues..."
aws sqs list-queues
;;
dynamodb)
echo "Listing DynamoDB Tables in $aws_region..."
aws dynamodb list-tables --region $aws_region
;;
ebs)
echo "Listing EBS Volumes in $aws_region..."
aws ec2 describe-volumes --region $aws_region
;;
*)
echo "Error: Invalid service '$aws_service'. Please enter a valid service."
exit 1
;;
esac
Conclusion
This script is an excellent starting point for those looking to explore cloud automation through scripting. By customizing it further to suit your specific needs or expanding it to include additional services, you can unlock new ways of interacting with cloud infrastructure. Dive in, experiment, and let your creativity guide you!
Checkout My GitHub Repo
Subscribe to my newsletter
Read articles from Waseef Tauqueer directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Waseef Tauqueer
Waseef Tauqueer
☁️ Cloud & DevOps Enthusiast | Engineering Student 🔧 Passionate about automation, infrastructure, and scaling solutions 📝 Sharing insights and projects at Tech Crusading