Understanding JavaScript Scope: A Beginner's Guide to Variable Visibility
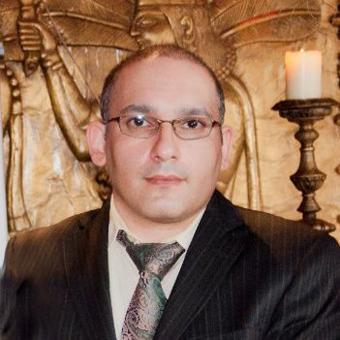
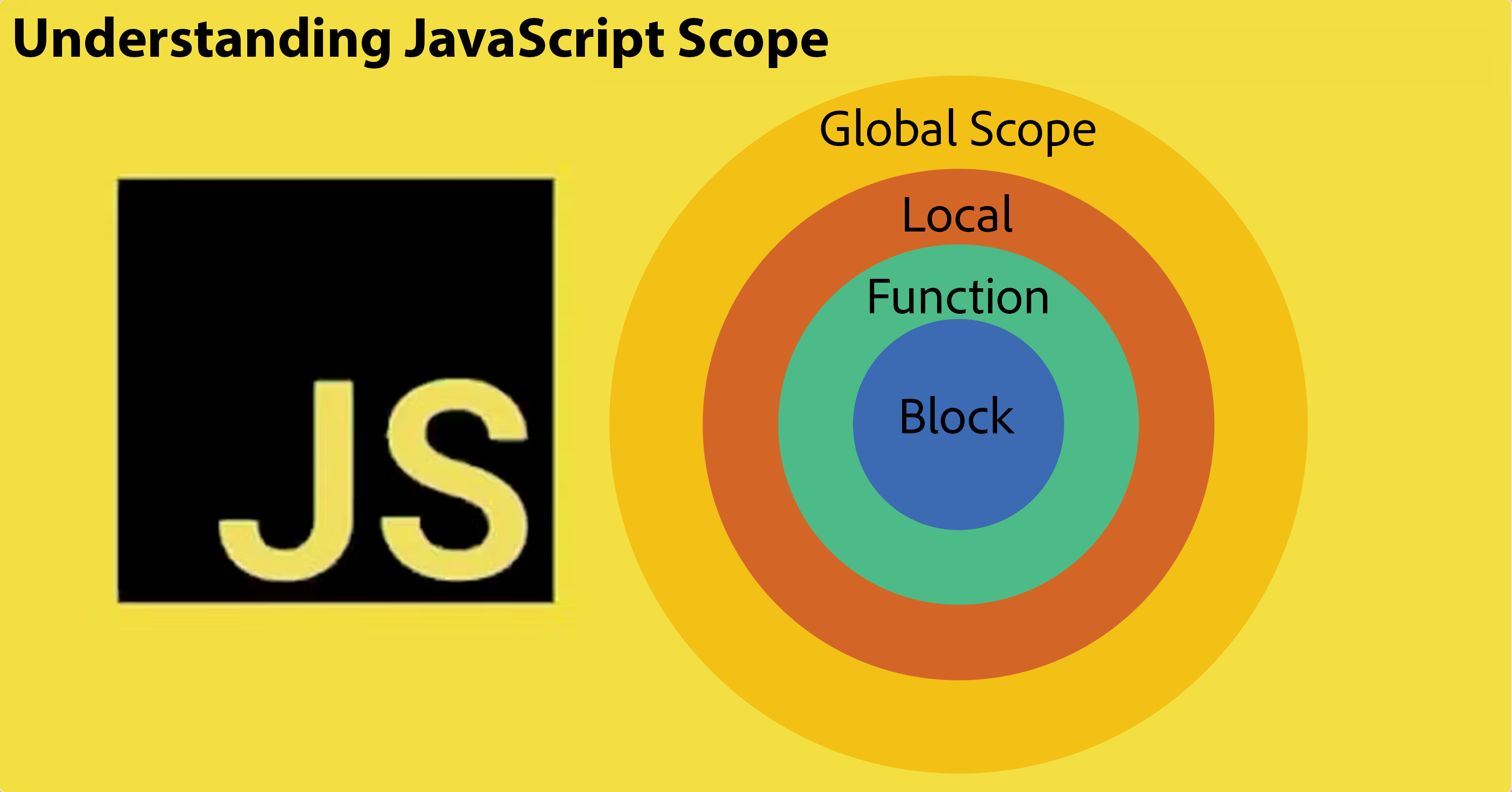
Ever found yourself scratching your head because a variable or function in your JavaScript code wasn’t working where you expected? It can feel like your code is playing hide and seek. The key to this mystery is something fundamental but often misunderstood—JavaScript Scope. In JavaScript, scope defines where your variables and functions live and breathe, determining where they can be accessed or modified throughout your program.
In this tutorial, we’ll take a closer look at what scope is, how it works, and why mastering it is essential to writing cleaner, more efficient code. Whether you’ve struggled with global variables polluting your functions or wondered why your loops don’t behave as planned, understanding scope is the first step to solving these puzzles. By the end, you’ll have a solid grasp of scope in JavaScript and be equipped to tackle common scoping issues with confidence. Ready to take control of your code? Let’s dive in!
Scope is defined as the context in which the variable is visible or can be referenced.
In JavaScript, scope is the set of rules that defines where variables and functions are accessible. It determines which parts of your code can use or modify them. Simply put, where you declare a variable or function affects where you can use it later. For example, if you create a variable inside a function, that variable will only be available within that function and nowhere else.
If a variable or expression is not in the current scope, it will not be available for use. Scopes can also be layered in a hierarchy, so that child scopes have access to parent scopes, but not vice versa.
In JavaScript, variables and functions are categorized by scope, which determines where they can be accessed in your code. There are two primary types of scope: global scope and local scope.
Global scope means that a variable or function is accessible from anywhere in your code. These are typically declared outside of any function or block.
Local scope, on the other hand, refers to variables that are only accessible within a specific part of the code. Local scope can be further divided into two types:
Functional scope, where variables are accessible only within the function in which they are defined.
Block scope, where variables declared inside a block (such as an
if
statement or loop) are only accessible within that block.
By understanding the differences between these scopes, you’ll be able to manage where and how your variables and functions are accessible, helping you avoid common errors and write cleaner code.
Global Scope:
Variables or functions declared outside of any function or block are in the global scope. These are accessible from anywhere in the code.
var globalVar = "I'm global";
function showGlobal() {
console.log(globalVar); // Accessible anywhere
}
showGlobal(); // Outputs: I'm global
The variable globalVar
is declared outside of any function or block, which places it in the global scope. This means that the variable can be accessed from anywhere in the program, including inside the showGlobal
function. When the function is called, it outputs the value of globalVar
because global variables are accessible throughout your entire code.
Why Global Scope Is Considered Bad Practice
While global variables might seem convenient, they can lead to several issues, especially in larger programs:
Unintended Modifications: Since global variables can be accessed and modified from anywhere in your code, it's easy to accidentally change their values in one part of the program without realising the impact elsewhere. This can make debugging difficult.
Naming Conflicts: As your program grows, using too many global variables increases the risk of name collisions, where two variables with the same name can overwrite each other.
Memory Leaks: Variables in the global scope persist throughout the lifecycle of the program. Overusing global variables can lead to memory leaks, especially in long-running applications, as global variables are not garbage-collected until the program ends.
For these reasons, it's generally best to avoid using global variables whenever possible. Instead, prefer using local scope (functional or block scope) to limit the accessibility of your variables and keep your code more modular and predictable.
It’s important to mention here that in non-strict mode, if you forget to declare a variable explicitly (with var
, let
, or const
), JavaScript will automatically create the variable in the global scope. This happens even if the variable is defined inside a function or block, which can lead to unintended global variables.
Here’s an example:
function myFunction() {
undeclaredVar = "I should have been declared";
}
myFunction();
console.log(undeclaredVar); // Outputs: "I should have been declared"
In the above example, undeclaredVar
was not explicitly declared with var
, let
, or const
, so JavaScript automatically made it a global variable, even though it was defined inside a function.
Why This Is Problematic:
Unintended Global Variables: Forgetting to declare a variable can create unwanted global variables, leading to potential bugs.
Naming Conflicts: Global variables can clash with other parts of your program, especially in large codebases, making debugging more difficult.
Strict Mode: In strict mode, JavaScript will prevent this behaviour and throw an error if you try to use an undeclared variable, helping avoid accidental global variables.
It’s always best to explicitly declare variables to avoid these issues. Would you like to explore more about strict mode or common scoping pitfalls?
To enable strict mode in JavaScript, you simply add the string
"use strict";
at the beginning of your script or function. This enforces a stricter parsing and error-checking mechanism, helping to avoid common mistakes such as accidentally creating global variables or using unsafe actions.
Local Scope
In addition to global scope, JavaScript also has local scope.
Local scope refers to variables that are accessible only within a specific part of your code—either within a function (functional scope) or within a block (block scope—inside blocks like loops or conditionals).
Functional scope refers to variables declared within a function. These variables are only accessible inside the function where they are defined. Once the function finishes execution, the variables are no longer available.
function showLocal() {
var localVar = "I'm local";
console.log(localVar); // Accessible inside the function
}
showLocal(); // Outputs: I'm local
console.log(localVar); // Error: localVar is not defined
In JavaScript, a function's scope is local to that function, meaning variables defined inside a function cannot be accessed from outside it. This concept is essential to understanding JavaScript's function scoping and is crucial for encapsulating data and avoiding variable name conflicts.
When a variable is declared within a function (using var
, let
, or const
), it is limited to that function’s scope. The variable doesn’t exist outside the function, so attempts to access it from outside will result in an error.
function myFunction() {
let localVariable = "I am inside the function";
console.log(localVariable); // This works; it's inside the function
}
myFunction(); // This runs fine and logs: "I am inside the function"
console.log(localVariable); // Error: localVariable is not defined
Explanation:
localVariable
is declared insidemyFunction
, so it only exists within that function.Trying to access
localVariable
outside ofmyFunction
results in aReferenceError
because it is out of scope.
Encapsulation and Data Privacy
Function scoping is helpful in encapsulating data to avoid unintentional changes or conflicts with other parts of the code. Consider this example:
function counter() {
let count = 0; // `count` is only accessible inside `counter`
count++;
console.log(count);
}
counter(); // Output: 1
counter(); // Output: 1 again, since `count` is reinitialized each time
console.log(count); // Error: count is not defined
Explanation:
Each time
counter()
is called, a newcount
variable is created within that specific function call.Outside the function, there is no
count
variable, protecting it from being modified elsewhere.
Block scope refers to variables declared within a block, which is any code enclosed in curly braces {}
—for example, inside loops, if
statements, or even within a function's block.
Variables declared with let
or const
inside a block are only accessible within that specific block. This is block scope.
Important! —Variables declared with
var
are not block-scoped, butlet
andconst
are.
if (true) {
let blockMessage = "I'm inside the block!";
console.log(blockMessage); // Accessible here
}
console.log(blockMessage); // Error: blockMessage is not defined
In both cases, variables are confined to their respective local scopes, whether that’s inside a function (functional scope) or a block of code (block scope). This helps prevent variables from being accessed or modified unintentionally outside of their intended context.
By understanding the differences between these scopes, you’ll be able to manage where and how your variables and functions are accessible, helping you avoid common errors and write cleaner code.
Lexical scope
In addition to global, functional, and block scope, JavaScript also follows something called lexical scope (also known as static scope). Lexical scope refers to the way JavaScript determines variable access based on where functions and variables are physically written in the code, not where they are called from.
In other words, a function’s scope is defined by its location within the source code, and it has access to variables from its outer scope.
Lexical scope can be slightly more challenging to grasp compared to other types of scope. It is sometimes also called static scope or compile-time scope.
How Lexical Scope Works:
When a function is declared inside another function or block, it has access to variables in its own scope as well as the scopes of its parent functions, even after the parent function has finished executing.
For example:
function outerFunction() {
let outerVar = "I'm outside!";
function innerFunction() {
console.log(outerVar); // Can access outerVar due to lexical scope
}
innerFunction();
}
outerFunction(); // Outputs: I'm outside!
In this example, the innerFunction
is defined inside outerFunction
. Even though outerVar
is not declared inside innerFunction
, it can still access outerVar
because of lexical scope. JavaScript looks at where innerFunction
is defined (inside outerFunction
), so it can access any variables in outerFunction
's scope.
Lexical scope means that the scope of a variable is determined by its position in the code. In other words, variables are available in the same scope as their parent variables.
Why Lexical Scope Is Important:
Lexical scope is a key concept that enables closures in JavaScript, allowing inner functions to "remember" and access variables from their outer functions even after those outer functions have finished executing. This is crucial for building more complex functionality, such as callbacks or event handlers.
JavaScript does allow for an exception called a closure where an inner function can access variables from its parent function, even after the parent function has executed.
Remember in your earlier encapsulation example:
function counter() {
let count = 0; // `count` is only accessible inside `counter`
count++;
console.log(count);
}
counter(); // Output: 1
counter(); // Output: 1 again, since `count` is reinitialized each time
console.log(count); // Error: count is not defined
In the above example, Outside the counter()
function, there is no count
variable, protecting it from being modified elsewhere.
Closures offer an exception where inner functions can "remember" outer function variables for access even outside the initial scope.
In the closure example below, the inner function can access variables from its parent function, even after the parent function has executed.
function createCounter() {
let count = 0;
return function () {
count++;
return count;
};
}
const counter = createCounter();
console.log(counter()); // Output: 1
console.log(counter()); // Output: 2
Explanation:
Here,
count
is insidecreateCounter
and isn’t directly accessible from outside.However, the inner function returned by
createCounter
creates a closure, allowing it to "remember"count
across calls.
Scope is a fundamental concept in JavaScript that controls where variables and functions can be accessed. Variables declared with const
and let
follow the same scoping rules, whether they’re inside a block, function, or module. However, var
variables only follow these scoping rules when inside a function or module.
Using scope correctly helps improve both the security and reusability of your code. By preventing naming conflicts (namespace collisions), you can avoid bugs and make your code easier to maintain and reuse in future projects.
Now that you understand how scope works, you can apply it to write cleaner, more organized, and maintainable JavaScript code.
https://www.w3schools.com/js/js_scope.asp
https://developer.mozilla.org/en-US/docs/Glossary/Scope
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Closures
Subscribe to my newsletter
Read articles from Pedram Badakhchani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
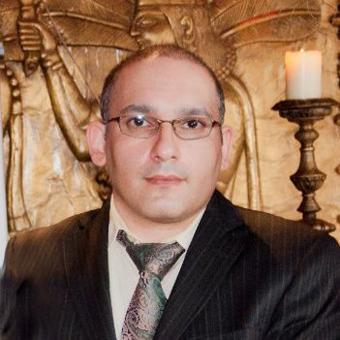
Pedram Badakhchani
Pedram Badakhchani
Working as an online tutor for the Bachelor of Computer Science Degree from Goldsmiths, University of London on the Coursera platform. This is the first undergraduate degree programme available on Coursera, one of the world’s leading online education providers. The programme has been designed to equip students to access careers in emerging technologies, providing opportunities for students to study machine learning, data science, virtual reality, game development and web programming to meet the needs of career changers in industry as well as those taking their first steps into the innovative computer science field.