Automate Customer Support with Multi-Agents using OpenAI Swarm, Azure AI Search, and Azure OpenAI
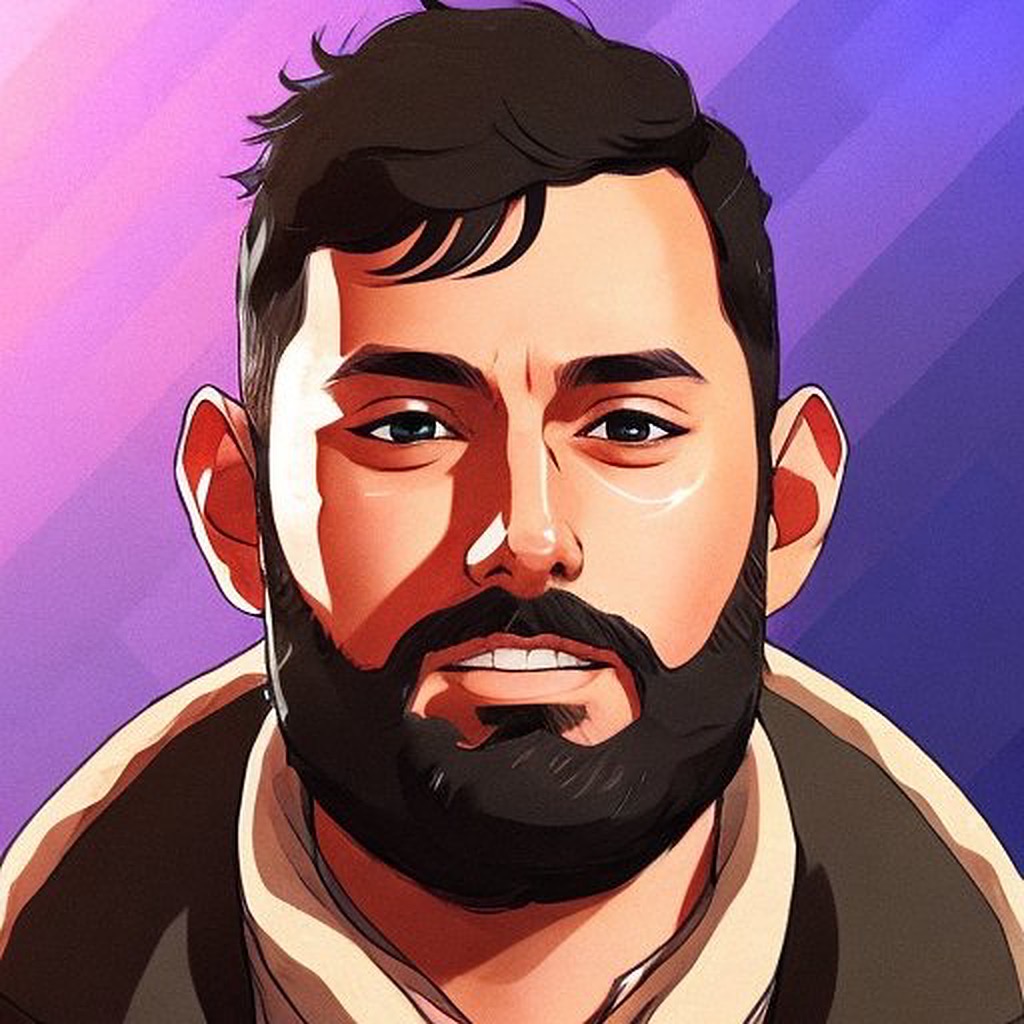
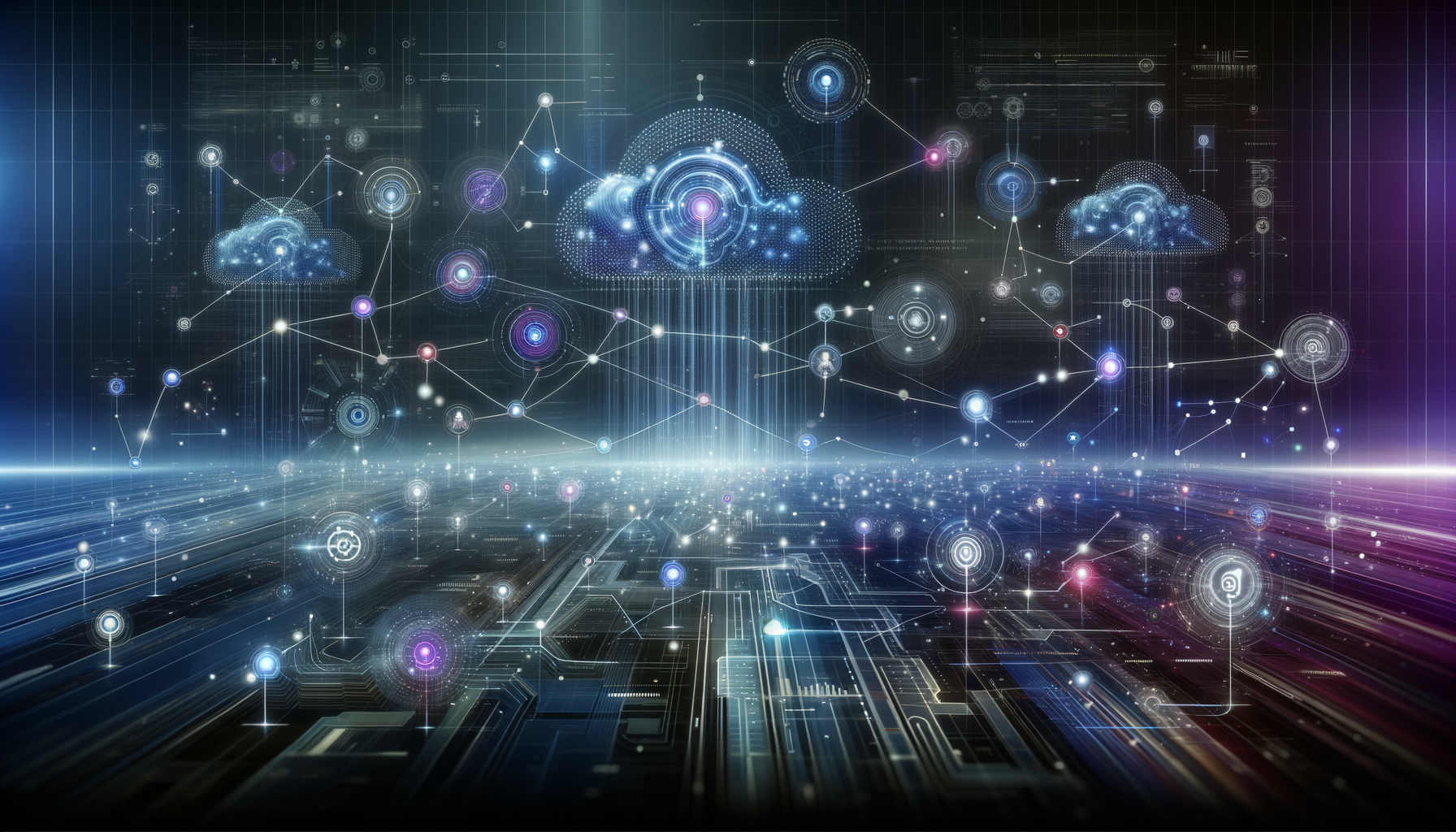
Introduction
Automating customer support can be a game changer for businesses, saving time, reducing costs, and improving customer satisfaction. Leveraging AI technologies like OpenAI Swarm, Azure AI Search, and Azure OpenAI, you can build a customer support agent that handles complex inquiries and performs various tasks like answering questions, submitting tickets, and even sending emails—all without human intervention. In this guide, we’ll walk you through creating a scalable, intelligent agent to streamline your customer service operations.
This blog will explain step-by-step how to set up and integrate these powerful tools to build a agent that handles customer queries effectively. We’ll also cover how to manage multi-agent systems using OpenAI Swarm, and leverage Azure AI Search for document retrieval, backed by Azure OpenAI’s GPT4o-mini for language models and text-embedding-3-large for embedding models.
Prerequisites
Before we get started, make sure you have the following:
Python 3.10 or higher
OpenAI Swarm installed
Access to Azure OpenAI with the necessary models deployed
Azure AI Search with a populated index named
azure-search-docs
Azure OpenAI API Key
Azure AI Search service endpoint and API Key
How to Create an Azure AI Search Index in 60 seconds
Follow these steps to quickly index your documentation:
Visit the Azure Search Documentation.
Scroll down to the bottom left and click Download PDF.
Go to Blob Storage, create a new container, and upload the
azure-search.pdf
In the Azure Portal, navigate to your Azure AI Search service.
Once uploaded, click Import and Vectorize in the Azure AI Search interface and follow the wizard to configure the indexing process.
Wait for the indexing process to complete.
After completion, verify that the search index is created with at least the following fields:
title
,chunk
, andtext_vector
.
Why Use OpenAI Swarm, Azure AI Search, and Azure OpenAI?
These three technologies combined create a highly effective system for automating customer support. Here’s why:
OpenAI Swarm allows you to orchestrate multiple agents seamlessly. Agents can transfer tasks between each other (handoffs), ensuring specialized tasks are handled by the right agent.
Azure AI Search documentation | Microsoft Learn offers powerful search capabilities, enabling the agent to retrieve relevant documents and information to answer customer queries accurately.
Azure OpenAI enhances the agent’s ability to understand and process natural language, making interactions feel more human-like.
Step by Step Guide
Setting Up the Environment
Let’s start by setting up the necessary packages and services.
Step 1: Install Required Packages
You’ll need to install OpenAI Swarm and the Azure Search SDK.
!pip install git+https://github.com/openai/swarm.git
!pip install azure-search-documents==11.6.0b6
Step 2: Import Libraries
Once the packages are installed, import the libraries you need:
from swarm import Swarm, Agent
from azure.search.documents import SearchClient
from azure.core.credentials import AzureKeyCredential
from openai import AzureOpenAI
import json, re, os
import re
from azure.search.documents import SearchClient
from azure.search.documents.models import (
QueryLanguage,
QueryType,
VectorizableTextQuery,
)
Step 3: Configure API Credentials
Make sure you’ve got your API keys ready for Azure OpenAI and Azure AI Search.
# Azure OpenAI API configuration
aoai_client = AzureOpenAI(
api_key=os.getenv("AZURE_OPENAI_API_KEY"),
api_version="2024-09-01-preview",
azure_endpoint=os.getenv("AZURE_OPENAI_ENDPOINT")
)
# Azure AI Search configuration
search_endpoint = os.getenv("AZURE_SEARCH_SERVICE_ENDPOINT")
search_api_key = os.getenv("AZURE_SEARCH_ADMIN_KEY")
index_name = "azure-search-docs" # Your Azure Search index
Step 4: Initialize Azure AI Search Client
credential = AzureKeyCredential(search_api_key)
search_client = SearchClient(
endpoint=search_endpoint,
index_name=index_name,
credential=credential
)
Step 5: Initialize OpenAI Swarm Client
Now, initialize the Swarm client, which will coordinate the agents.
swarm_client = Swarm(client=aoai_client)
Building the Customer Support Agent
Your agent will consist of two main agents:
User Interface Agent: Handles the initial user interaction.
Help Center Agent: Uses Azure AI Search to retrieve documentation and perform actions like submitting tickets and sending emails.
Step 1: Define Helper Functions
We’ll create some basic functions that the agent can use during interactions.
- Query Documents: Search Azure AI Search for relevant information.
def query_docs(query: str):
vector_query = VectorizableTextQuery(
text=query,
k_nearest_neighbors=3,
fields="text_vector"
)
results = search_client.search(
search_text=query,
vector_queries=[vector_query],
top=3
)
return results
- Submit Ticket: Simulate submitting a support ticket.
def submit_ticket(description: str):
return {"response": f"Ticket created with description: '{description}'."}
- Send Email: Simulate sending a confirmation email.
def send_email(email_address: str, message: str):
return {"response": f"Email sent to {email_address} with message: {message}."}
Step 2: Create Agents
- Help Center Agent
help_center_agent = Agent(
name="Help Center Agent",
instructions="You assist users by retrieving information or submitting tickets.",
functions=[query_docs, submit_ticket, send_email],
model='gpt-4o-mini' # Replace with your Azure OpenAI model deployment
)
- User Interface Agent
user_interface_agent = Agent(
name="User Interface Agent",
instructions="You handle initial user queries and can transfer to the Help Center Agent.",
functions=[transfer_to_help_center],
model='gpt-4o-mini'
)
Step 3: Set Up Multi-Agent Interaction
Now, let’s define the interaction loop, where the User Interface Agent can transfer the query to the Help Center Agent if needed.
# Initialize context variables
context_variables = {"name": "Farzad", "user_id": 12345}
# Initialize messages and set the starting agent
messages = []
agent = user_interface_agent # Start with the User Interface Agent
def pretty_print_messages(messages):
for message in messages:
if message.get("content") is None:
continue
sender = message.get("sender", message.get("role", "assistant"))
print(f"{sender.capitalize()}: {message['content']}")
def is_valid_email(email: str) -> bool:
pattern = r"[^@]+@[^@]+\.[^@]+"
return re.match(pattern, email) is not None
# Interaction loop with print-based logging
print("You can start chatting with the assistant. Type 'exit' or 'quit' to end the conversation.")
try:
while True:
user_input = input("> ")
print(f"User Input: {user_input}") # Logging user input
if user_input.lower() in ["exit", "quit"]:
print("Assistant: Goodbye!")
break
messages.append({"role": "user", "content": user_input})
# Check if the user provided an email address
if is_valid_email(user_input):
context_variables["email_address"] = user_input.strip()
# Inform the assistant that the email address has been provided
messages.append({"role": "system", "content": f"User email address is {user_input.strip()}"})
response = swarm_client.run(
agent=agent,
messages=messages,
context_variables=context_variables
)
# Update messages with the new messages from the response
messages.extend(response.messages)
# Update agent if there's a handoff
agent = response.agent
# Update context variables if they have changed
context_variables.update(response.context_variables)
# Print the assistant's response
pretty_print_messages(response.messages[-len(response.messages):])
except KeyboardInterrupt:
print("\nAssistant: Goodbye!")
Here is what OpenAI Swarm in action looks like:
Business Value of Automating Customer Support
By building an automated customer support agent, you can:
Save Time and Resources: Handle repetitive queries automatically, allowing human agents to focus on complex issues.
24/7 Availability: Agents can provide round-the-clock support, ensuring no customer query is missed.
Improved Customer Satisfaction: Faster response times and relevant information retrieval lead to a better customer experience.
Scalability: The system can easily scale to handle a high volume of requests without needing to hire more human agents.
Conclusion
By integrating OpenAI Swarm, Azure AI Search, and Azure OpenAI, you can build powerful and scalable agents that automates routine tasks, retrieves detailed information, and seamlessly interacts with customers. This agent is not just a tool for reducing costs, but a strategic asset for improving customer satisfaction and scaling your support operations.
Ready to supercharge your customer support? Start building with these technologies today!
Subscribe to my newsletter
Read articles from Farzad Sunavala directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
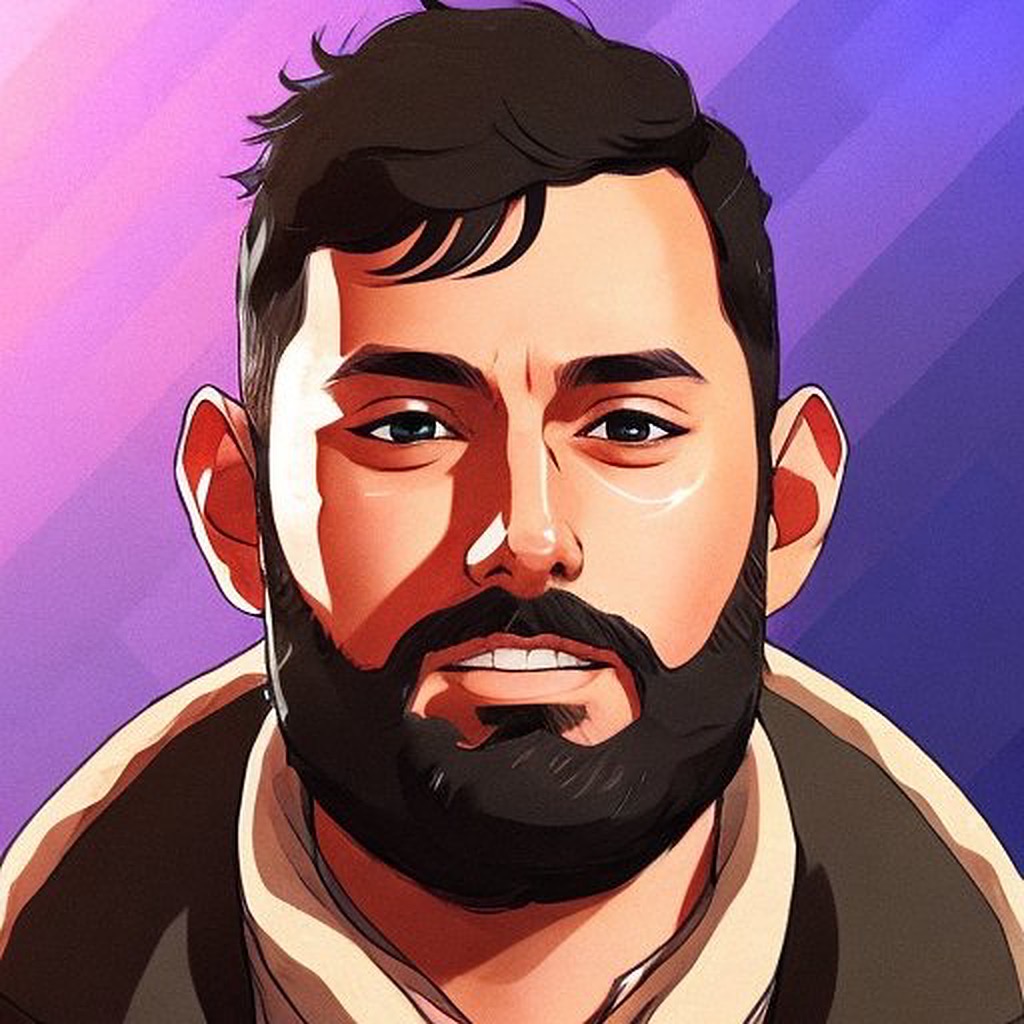
Farzad Sunavala
Farzad Sunavala
I am a Principal Product Manager at Microsoft, leading RAG and Vector Database capabilities in Azure AI Search. My passion lies in Information Retrieval, Generative AI, and everything in between—from RAG and Embedding Models to LLMs and SLMs. Follow my journey for a deep dive into the coolest AI/ML innovations, where I demystify complex concepts and share the latest breakthroughs. Whether you're here to geek out on technology or find practical AI solutions, you've found your tribe.