The Whale's Filtering Process
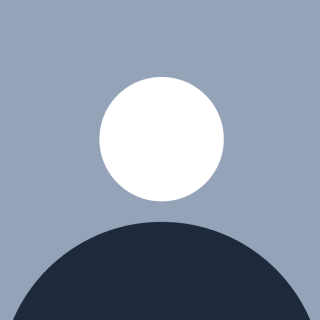
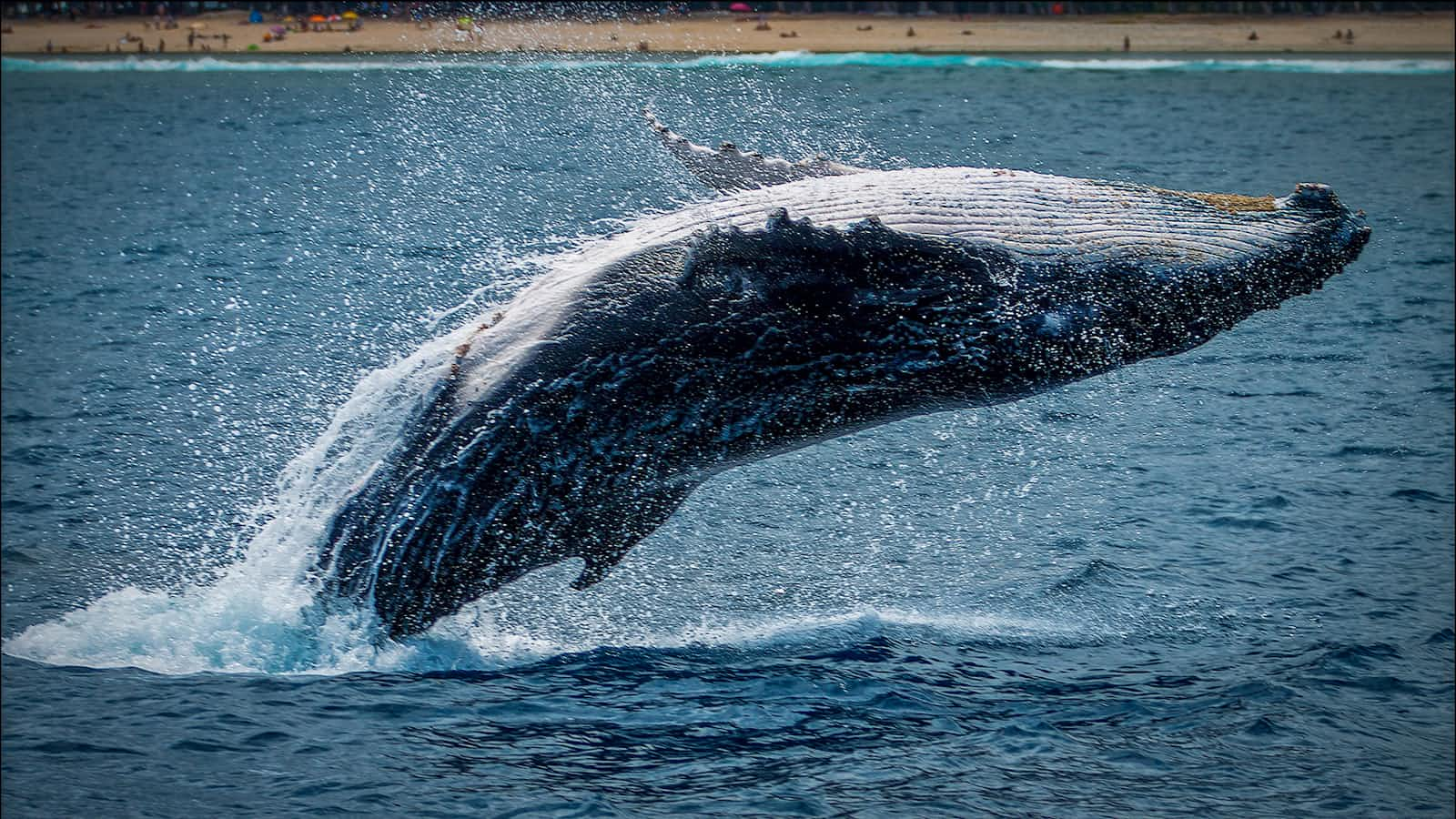
Imagine a whale gliding through the ocean, filtering gallons of water to capture food. The whale efficiently sifts through its environment by separating smaller prey into manageable portions. This process mirrors Bucket Sort, where the algorithm divides elements into different "buckets" (subsets) based on their value, sorts each bucket individually, and then combines them to create the final sorted list.
In this article, we’ll explore how Bucket Sort works, optimize it for different cases, and dive into some fun challenges. Let the whale guide you through this intelligent sorting process!
Simple Bucket Sort – The Whale’s Filtering Method
Bucket Sort 101 – Dividing and Conquering
Bucket Sort works by distributing elements into a number of buckets. Each bucket contains a subset of the elements that fall within a specific range, and each bucket is sorted individually (often using another sorting algorithm). Finally, the sorted buckets are concatenated to produce the sorted list.
Step-by-Step Example with Code
Let’s take a list of whale feeding zones, represented by the number of krill each zone has: [0.32, 0.12, 0.45, 0.91, 0.60]
.
Distribute Elements into Buckets:
The list is divided into buckets based on value. For example, if you have 5 buckets, each bucket holds values within specific ranges.Bucket 1:
[0.12]
Bucket 2:
[0.32]
Bucket 3:
[0.45]
Bucket 4:
[0.60]
Bucket 5:
[0.91]
Sort Each Bucket:
Sort the elements within each bucket (in this case, each bucket contains only one element, so no further sorting is needed).Concatenate the Buckets:
Combine the elements from each sorted bucket into a single list.
Final sorted list:[0.12, 0.32, 0.45, 0.60, 0.91]
.
Code Snippets for Simple Bucket Sort
def bucket_sort(arr):
bucket_count = len(arr)
buckets = [[] for _ in range(bucket_count)]
for num in arr:
index = int(num * bucket_count)
buckets[index].append(num)
for i in range(bucket_count):
buckets[i].sort()
sorted_arr = []
for bucket in buckets:
sorted_arr.extend(bucket)
return sorted_arr
# Example usage
whale_zones = [0.32, 0.12, 0.45, 0.91, 0.60]
print(bucket_sort(whale_zones))
function bucketSort(arr) {
let bucketCount = arr.length;
let buckets = Array.from({ length: bucketCount }, () => []);
for (let num of arr) {
let index = Math.floor(num * bucketCount);
buckets[index].push(num);
}
for (let bucket of buckets) {
bucket.sort((a, b) => a - b);
}
return buckets.flat();
}
// Example usage
let whaleZones = [0.32, 0.12, 0.45, 0.91, 0.60];
console.log(bucketSort(whaleZones));
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
void bucketSort(float arr[], int n) {
vector<float> buckets[n];
for (int i = 0; i < n; i++) {
int index = n * arr[i];
buckets[index].push_back(arr[i]);
}
for (int i = 0; i < n; i++)
sort(buckets[i].begin(), buckets[i].end());
int idx = 0;
for (int i = 0; i < n; i++)
for (int j = 0; j < buckets[i].size(); j++)
arr[idx++] = buckets[i][j];
}
int main() {
float whaleZones[] = {0.32, 0.12, 0.45, 0.91, 0.60};
int n = sizeof(whaleZones) / sizeof(whaleZones[0]);
bucketSort(whaleZones, n);
for (int i = 0; i < n; i++)
cout << whaleZones[i] << " ";
return 0;
}
Optimizing Bucket Sort – Fine-Tuning the Whale’s Strategy
Bucket Sort is already a very efficient sorting algorithm for data that is uniformly distributed over a range. Here’s how we can optimize it further:
Choosing the Number of Buckets:
The efficiency of Bucket Sort relies heavily on choosing the right number of buckets. For optimal performance, the number of buckets should generally be proportional to the number of elements in the list.Use of Efficient Sorting for Buckets:
Inside each bucket, using an efficient sorting algorithm like insertion sort or quick sort can reduce the overhead of sorting small subarrays.
Python Code for Optimized Bucket Sort
def insertion_sort(bucket):
for i in range(1, len(bucket)):
key = bucket[i]
j = i - 1
while j >= 0 and key < bucket[j]:
bucket[j + 1] = bucket[j]
j -= 1
bucket[j + 1] = key
return bucket
def bucket_sort_optimized(arr):
bucket_count = len(arr)
buckets = [[] for _ in range(bucket_count)]
for num in arr:
index = int(num * bucket_count)
buckets[index].append(num)
for i in range(bucket_count):
insertion_sort(buckets[i])
sorted_arr = []
for bucket in buckets:
sorted_arr.extend(bucket)
return sorted_arr
Time and Space Complexity – Understanding Bucket Sort’s Efficiency
Time Complexity:
Best Case (O(n + k)): In the ideal case, where the data is uniformly distributed and each bucket contains a small number of elements, Bucket Sort performs very efficiently with time complexity of O(n + k), where
n
is the number of elements andk
is the number of buckets.Worst Case (O(n²)): In the worst case, where all elements fall into the same bucket, Bucket Sort degrades to O(n²) as the bucket itself needs to be sorted using a slower sorting algorithm.
Space Complexity:
- Space Complexity (O(n + k)): Bucket Sort requires additional space for the buckets, resulting in a space complexity of O(n + k), where
n
is the number of elements andk
is the number of buckets.
Drawbacks and Real-World Applications
Drawbacks of Bucket Sort
Non-Uniform Data: Bucket Sort performs poorly when the input data is not uniformly distributed, as it may result in uneven bucket sizes, with some buckets being overloaded.
Memory Overhead: The need to create multiple buckets can lead to higher memory consumption, especially for large datasets.
Real-World Applications of Bucket Sort
Sorting Floating-Point Numbers: Bucket Sort is particularly well-suited for sorting floating-point numbers that are uniformly distributed over a range.
Grading Systems: Bucket Sort can be used in grading systems where students' scores are distributed over a specific range (e.g., 0–100), making it easier to assign grades.
Hash-Based Systems: Bucket Sort is effective in hash-based systems where data can be distributed into predefined ranges (or “buckets”) before being sorted or processed.
Challenge Time – Test Your Skills!
Challenge: Sorting Whale Krill Intake
You’re a marine biologist studying whale feeding habits, and you need to sort krill intake data:[0.42, 0.24, 0.75, 0.63, 0.92]
. Use Bucket Sort to arrange the values.Challenge: Sorting Rainfall Data
Sort a list of rainfall amounts (in inches) for different regions using Bucket Sort:[2.1, 0.8, 3.2, 1.9, 0.5]
.Challenge: Grading Exam Scores
You’ve been given a list of student exam scores to sort using Bucket Sort:[72, 85, 90, 67, 81, 78, 92, 88, 75]
. Sort the scores and group them into ranges (buckets) to easily assign grades.
Think you've mastered Bucket Sort? Try the challenges and share your solutions!
The Whale’s Filtering Efficiency in Sorting
Just like a whale filters through the ocean to gather food, Bucket Sort filters and organizes data into manageable portions, allowing for efficient sorting of elements. You’ve now learned how Bucket Sort works, when to use it, and how to optimize it for specific data types.
Ready for the next sorting challenge? Subscribe to stay updated on Counting Sort!
Subscribe to my newsletter
Read articles from gayatri kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by