Understanding Null vs Undefined in JavaScript: Key Differences and Use Cases 🤔

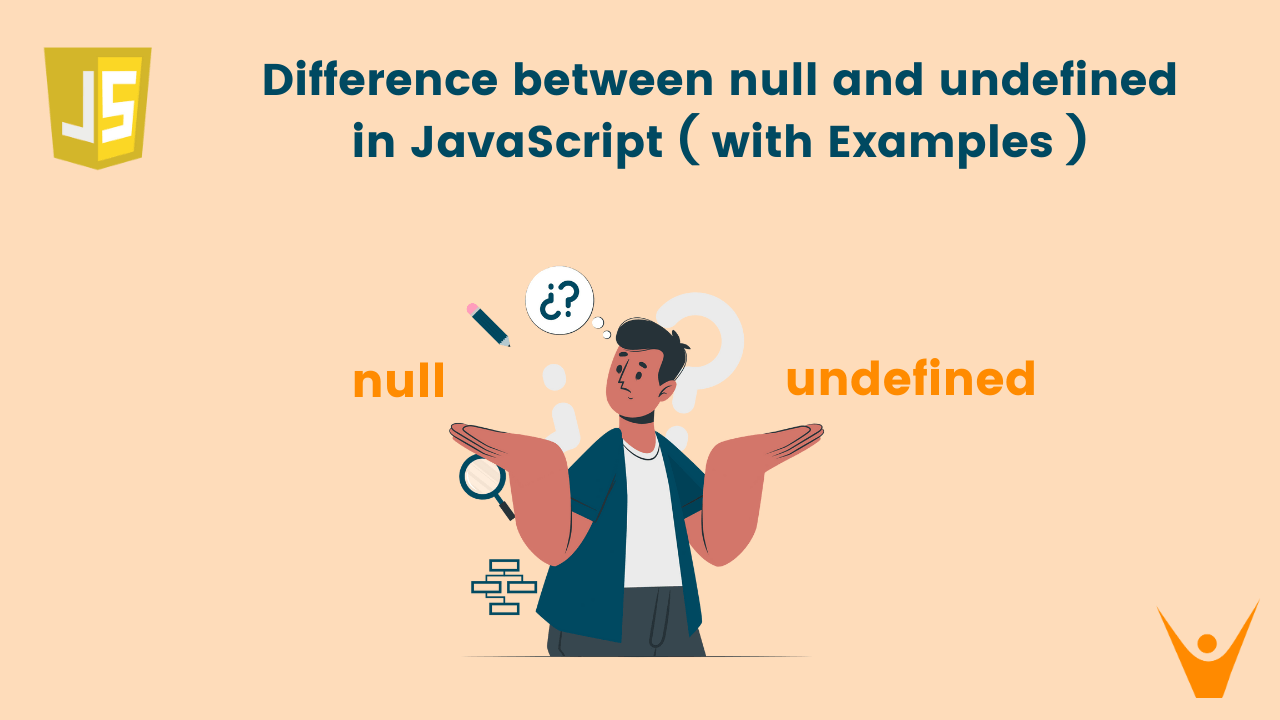
In JavaScript, encountering the terms null
and undefined
is common, but understanding the differences between them is crucial for writing clean and bug-free code. They may seem similar at first glance—both representing the absence of a value—but they serve different purposes. In this article, we’ll delve into the differences between null
and undefined
, explore their use cases, and provide examples to illustrate how each is used in JavaScript. 💡
What is undefined
? 🚫
undefined
is a built-in JavaScript primitive value that indicates a variable has been declared but has not yet been assigned a value. It is automatically assigned to variables when they are declared without an initial value.
Example:
let user;
console.log(user); // Output: undefined
In the above example, the user
variable is declared but not initialized with any value. Thus, JavaScript assigns undefined
to it.
• Other Scenarios of undefined
:
When a function does not return any value explicitly, it returns undefined
.
function greet() {
console.log('Hello!');
}
console.log(greet()); // Output: undefined
• Accessing a non-existent object property will also yield undefined
.
const person = { name: 'Alice' };
console.log(person.age); // Output: undefined
What is null
? 🕳️
null
is another primitive value in JavaScript that represents the intentional absence of any object value. It is explicitly assigned to a variable by the developer when they want to indicate that a variable should not have any value.
Example:
let user = null;
console.log(user); // Output: null
In this example, the user
variable is explicitly set to null
, indicating that it has no value or that its value is unknown.
Key Differences Between null
and undefined
🔍
Aspect | undefined | null |
Type | undefined is of type undefined . | null is of type object . |
Usage | Indicates an uninitialized variable. | Indicates an absence of value set intentionally. |
Assigned by | JavaScript automatically. | Developers explicitly. |
Conversion to Boolean | Boolean(undefined) results in false . | Boolean(null) results in false . |
Common Scenarios | Default value for uninitialized variables, missing properties, and non-returning functions. | Use when a variable should have no value. |
Comparing null
and undefined
🤝
In JavaScript, using the equality (==
) operator between null
and undefined
returns true
because they are considered loosely equal when compared with ==
. However, they are not strictly equal (===
), as they are of different types.
Example:
console.log(null == undefined); // Output: true
console.log(null === undefined); // Output: false
In the above example, null
and undefined
are loosely equal (==
) but not strictly equal (===
). When strict equality is important, always use ===
to avoid unexpected results.
When to Use null
and undefined
🧠
• Use undefined
when you want to check if a variable has been declared but not assigned a value, or when accessing object properties that may or may not exist.
function findUser(id) {
const user = database.find((user) => user.id === id);
return user ? user : undefined;
}
• Use null
when you want to explicitly indicate that a variable is empty or has no value, such as when resetting a variable or signaling the end of data processing.
let user = {
name: 'John',
age: 30,
};
// User logs out, so we set user to null.
user = null;
Checking for null
and undefined
Safely ✅
To avoid errors when dealing with null
and undefined
, you can use a strict equality check (===
) or typeof
checks.
Example:
if (user !== null && user !== undefined) {
// Safe to use `user` here.
}
Alternatively, you can use the ??
(nullish coalescing) operator, which returns the right-hand value if the left-hand side is null
or undefined
.
let username;
console.log(username ?? 'Guest'); // Output: 'Guest'
The ??
operator is useful when you want to provide default values for potentially null
or undefined
variables.
Conclusion 📝
Understanding the difference between null
and undefined
is key to handling data in JavaScript effectively. While both indicate the absence of a value, undefined
is used by JavaScript automatically for uninitialized variables, whereas null
is used intentionally by developers. By knowing when and how to use each, you can write more predictable and robust code.
Mastering these concepts will help you handle errors more gracefully and make your JavaScript code cleaner and easier to debug. Happy coding! 🎉
Subscribe to my newsletter
Read articles from Yasin Sarkar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Yasin Sarkar
Yasin Sarkar
Front-End Developer. I create dynamic web applications using HTML, Tailwind CSS, JavaScript, React, and Next.js. I share my knowledge on social media to help others enhance their tech skills. An Open Source Enthusiast and Writer.