SDET Java Questions - Post 1
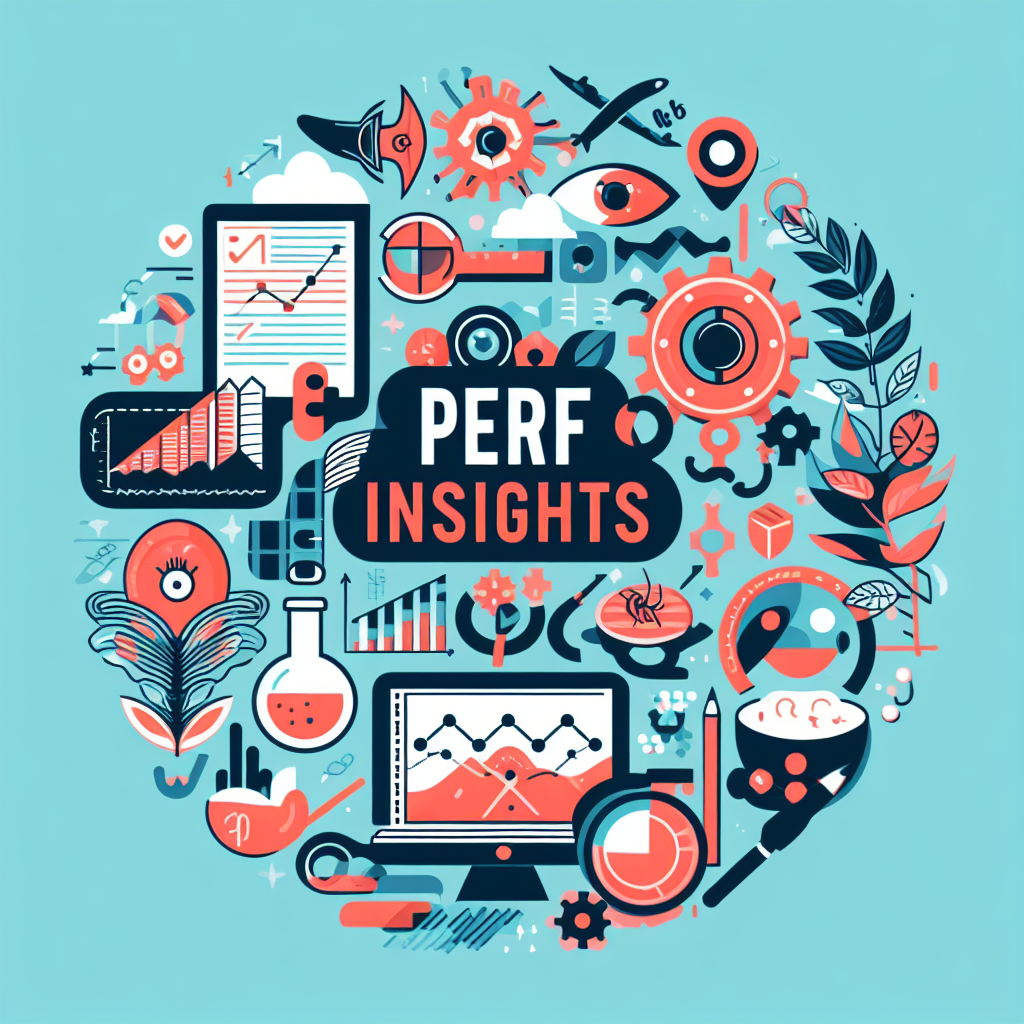
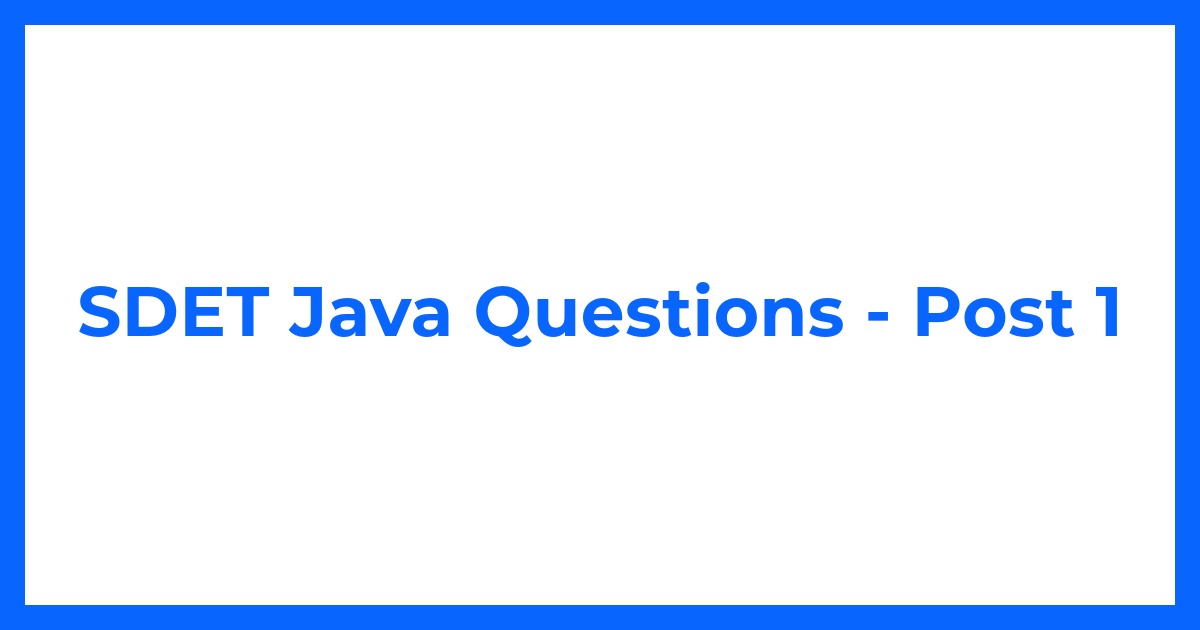
These two questions were posted by a LinkedIn user, and I thought of solving them. Two unique problems: one about converting a string from CamelCase to SnakeCase, and the other about finding common numbers in an array. In this blog, I’ll write my approach to solving these problems step by step.
Program 1 : Converting CamelCase to SnakeCase
Converting a camel case sentence to a snake case sentenced
Input -->sentence="MyNameIsAswin"
Output --> My_Name_Is_Aswin
My Approach:
To solve this, I wrote a simple method that traverses through the input string, checks for uppercase letters, and inserts an underscore before them to convert it to SnakeCase.
Link - LinkedIn | Post
// Question 1 Helper Method
public static String convertToSnakeCase(String str){
StringBuilder sb = new StringBuilder();
sb.append(str.charAt(0)); // Start with the first character
for (int i = 1; i < str.length(); i++) {
char ch = str.charAt(i);
if (Character.isUpperCase(ch)) {
sb.append("_"); // Add underscore before an uppercase letter
}
sb.append(ch); // Append the current character
}
return sb.toString();
}
Problem 2: Finding Common Numbers in an Array
Print the count of common numbers in an array with size 3
Input-->arr=[5,3,2]
Output-->0
Input-->arr=[2,5,2]
Output-->2
Input-->arr=[5,5,5]
Output-->3
My Approach:
I used a HashMap
to store the frequency of each element in the array and then returning the highest frequency.
// Question 2 Helper Method
public static int findCommonNum(int[] arr) {
Map<Integer, Integer> map = new HashMap<>();
// Count the frequency of each number
for (int ele : arr) {
map.put(ele, map.getOrDefault(ele, 0) + 1);
}
// Find the maximum frequency
int answer = 0;
for (int value : map.values()) {
if (value > answer) {
answer = value;
}
}
// Return 0 if all elements are unique
return (answer == 1) ? 0 : answer;
}
Full Code Example:
Here’s the complete code implementing both solutions:
import java.util.*;
class HelloWorld {
public static void main(String[] args) {
// Question 1
String sentence = "MyNameIsAswin";
String result = convertToSnakeCase(sentence);
System.out.println(result);
// Question 2
int[] input = {2, 1, 6};
int answer = findCommonNum(input);
System.out.println(answer);
}
// Question 1 Helper Method
public static String convertToSnakeCase(String str){
StringBuilder sb = new StringBuilder();
sb.append(str.charAt(0));
for (int i = 1; i < str.length(); i++) {
char ch = str.charAt(i);
if (Character.isUpperCase(ch)) {
sb.append("_");
}
sb.append(ch);
}
return sb.toString();
}
// Question 2 Helper Method
public static int findCommonNum(int[] arr) {
Map<Integer, Integer> map = new HashMap<>();
for (int ele : arr) {
map.put(ele, map.getOrDefault(ele, 0) + 1);
}
int answer = 0;
for (int value : map.values()) {
if (value > answer) {
answer = value;
}
}
return (answer == 1) ? 0 : answer;
}
}
Subscribe to my newsletter
Read articles from Gulshan Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
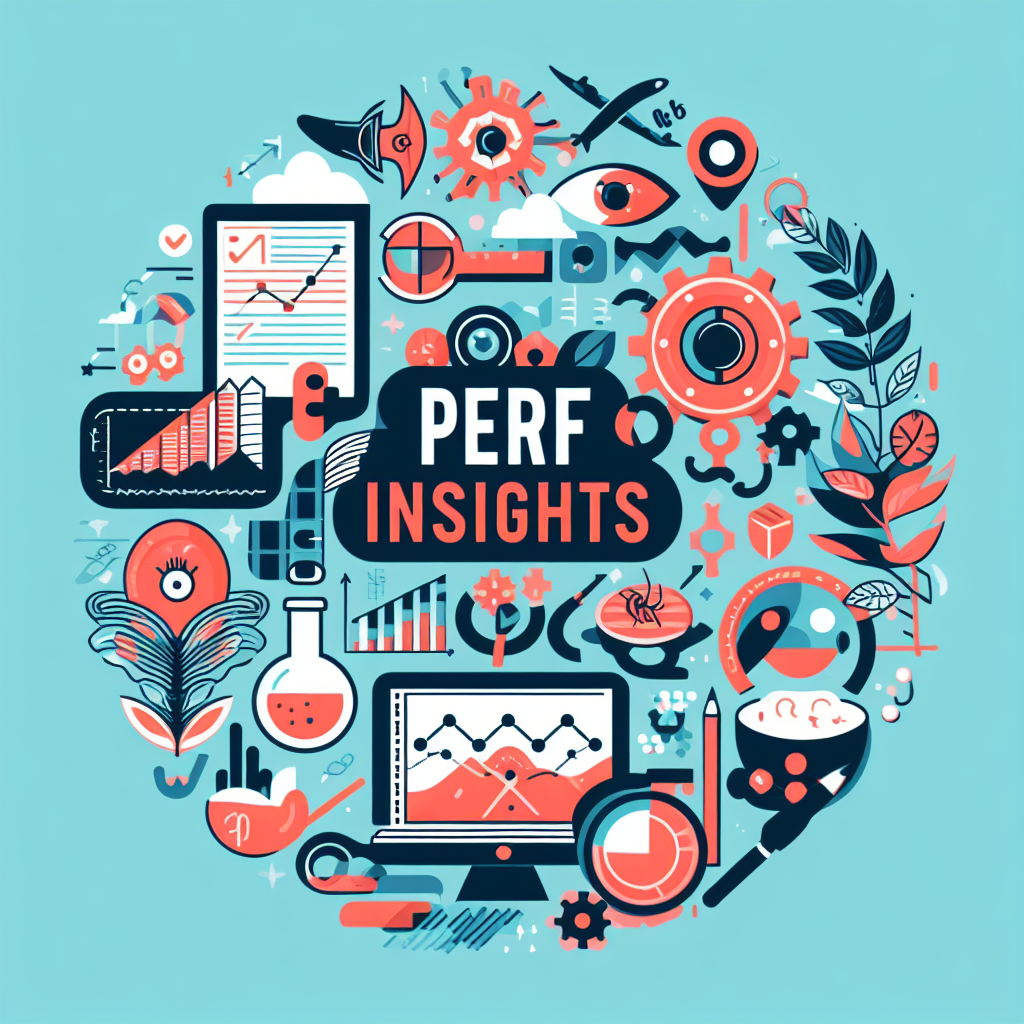
Gulshan Kumar
Gulshan Kumar
As a Systems Engineer at Tata Consultancy Services, I deliver exceptional software products for mobile and web platforms, using agile methodologies and robust quality maintenance. I am experienced in performance testing, automation testing, API testing, and manual testing, with various tools and technologies such as Jmeter, Azure LoadTest, Selenium, Java, OOPS, Maven, TestNG, and Postman. I have successfully developed and executed detailed test plans, test cases, and scripts for Android and web applications, ensuring high-quality standards and user satisfaction. I have also demonstrated my proficiency in manual REST API testing with Postman, as well as in end-to-end performance and automation testing using Jmeter and selenium with Java, TestNG and Maven. Additionally, I have utilized Azure DevOps for bug tracking and issue management.