Foreach vs. Map: A Comprehensive Comparison for JavaScript Developers 🚀

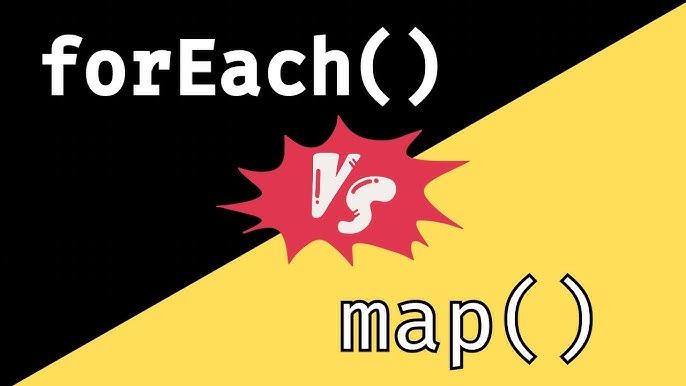
When working with arrays in JavaScript, you often need to iterate over each element. Two commonly used methods for this purpose are forEach
and map
. While they might seem similar at first glance, they serve different purposes and have distinct behaviors that can significantly impact your code. Understanding these differences will help you choose the right one for your specific needs. In this article, we’ll explore forEach
and map
in depth, including their syntax, use cases, and performance considerations.
1. Understanding forEach
👀
The forEach
method in JavaScript is used to execute a provided function once for each array element. It’s typically used when you want to perform side effects such as logging values, updating an external variable, or making API calls. The forEach
method does not return a new array; it simply iterates over each element and performs an action.
Syntax:
array.forEach((element, index, array) => {
// Your code here
});
element
: The current element being processed.index
: The index of the current element.array
: The original array being iterated over.
Example:
const numbers = [1, 2, 3, 4];
numbers.forEach((num) => {
console.log(num * 2);
});
// Output: 2, 4, 6, 8
In the example above, forEach
iterates over each element and logs its double to the console. Notice that it doesn’t create or return a new array; it just performs the action for each element.
Key Points:
forEach
does not return a new array.Ideal for side effects like logging or updating variables.
Does not support chaining, as it returns
undefined
.
2. Understanding map
🔄
The map
method is used to create a new array by applying a provided function to every element in the original array. It returns a new array containing the results of the function for each element, making it ideal for scenarios where you need to transform or modify data.
Syntax:
const newArray = array.map((element, index, array) => {
// Return the transformed element
return element * 2;
});
element
: The current element being processed.index
: The index of the current element.array
: The original array being iterated over.
Example:
const numbers = [1, 2, 3, 4];
const doubled = numbers.map((num) => num * 2);
console.log(doubled);
// Output: [2, 4, 6, 8]
In this example, map
creates a new array doubled
containing the doubled values of each element from the numbers
array. Unlike forEach
, map
is used here because we wanted to transform each element into a new form.
Key Points:
map
returns a new array.Useful for transformations where you want to create a new array.
Supports chaining, since it returns an array.
3. Key Differences Between forEach
and map
⚖️
Feature | forEach() | map() |
Return Value | undefined | New array with transformed elements |
Mutates Original Array | No | No |
Use Case | Side effects (logging, API calls) | Transforming data to a new array |
Chaining | Not possible | Supports chaining |
Performance | Faster for side effects | Might be slower due to array creation |
The choice between forEach
and map
depends on whether you need the result of the iteration. If you simply want to perform an action for each element without returning a new array, forEach
is more suitable. However, if you need to transform each element into a new form and store those values, map
is the way to go.
4. Performance Considerations ⚡
Performance is always a critical factor when choosing between these methods. Here are a few key points to consider:
Memory Usage:
map
creates a new array, which means it uses more memory thanforEach
. If memory usage is a concern, and you don't need a new array,forEach
may be more efficient.Speed: For simple operations,
forEach
can be slightly faster thanmap
because it doesn’t have to create a new array. However, the difference is often negligible unless you are working with large datasets.Readability: Code readability is also a crucial factor. Using
map
when you don't need the new array can make your code harder to understand. On the other hand, usingforEach
when you need to transform data might confuse other developers who expect a result.
5. When to Use forEach
vs. map
📅
• Use forEach
when:
You want to perform a side effect for each element (logging values, modifying external variables).
You don’t need to return a new array.
• Use map
when:
You need to transform each element and want a new array with the transformed data.
You plan to chain the method with other array functions like
filter
orreduce
.
Conclusion 🎉
Both forEach
and map
are valuable methods for iterating over arrays in JavaScript, each with its unique strengths. Understanding the key differences between them will allow you to write more efficient, readable, and maintainable code. Remember, choose forEach
for side effects and map
for transformations.
By selecting the right method for the job, you can ensure that your code is not only effective but also optimized for performance and clarity. Happy coding! 💻
I hope this article helps you understand when to use forEach
vs. map
in your JavaScript projects. If you have any questions or thoughts, feel free to leave a comment below. Happy coding, and keep exploring JavaScript! 🚀
Subscribe to my newsletter
Read articles from Yasin Sarkar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Yasin Sarkar
Yasin Sarkar
Front-End Developer. I create dynamic web applications using HTML, Tailwind CSS, JavaScript, React, and Next.js. I share my knowledge on social media to help others enhance their tech skills. An Open Source Enthusiast and Writer.