Going Green(let): Concurrency without the Chaos!
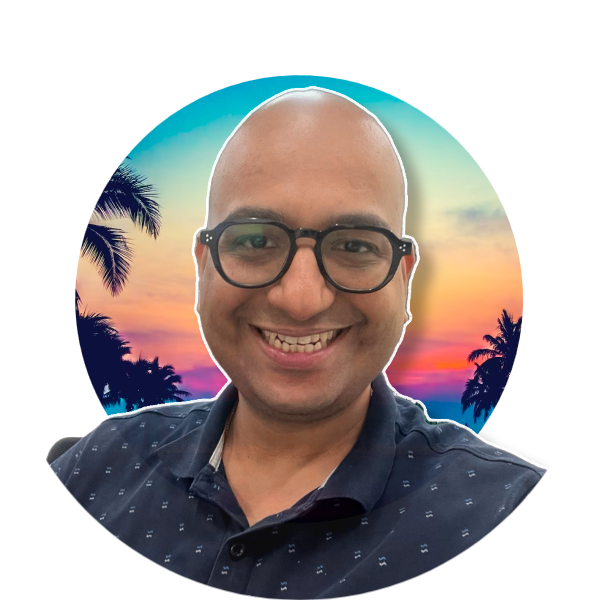
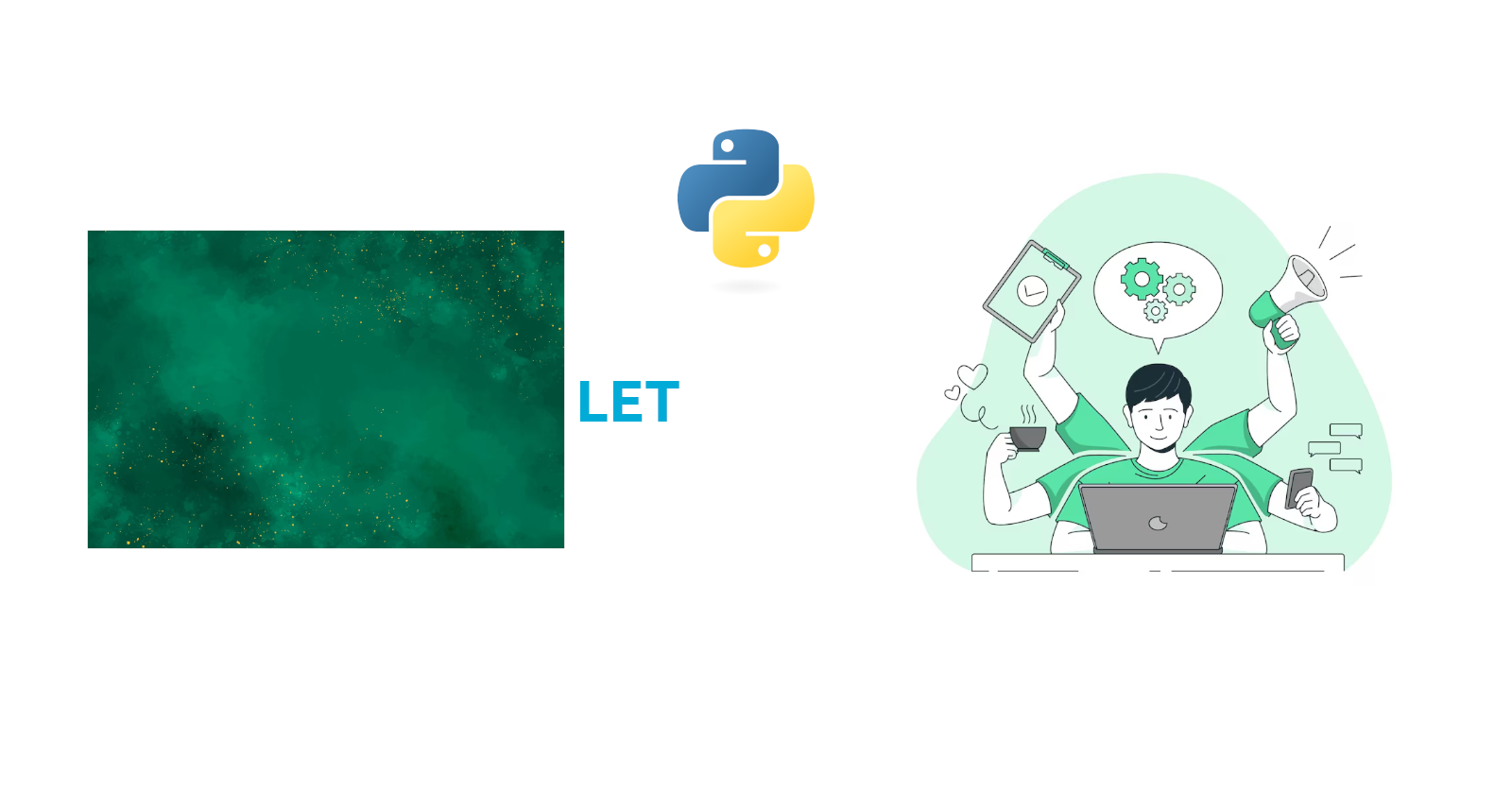
A greenlet is a lightweight, low-level coroutine-like primitive in Python, used primarily for concurrency. It is part of the greenlet
module, which is often associated with gevent, a library for asynchronous I/O operations.
Here are some key points about greenlets:
Micro-threading: Greenlets are like micro-threads that allow you to manage the execution of code blocks without creating actual OS-level threads. They are not parallel, but concurrent, meaning they give the appearance of multitasking by switching between different tasks.
Explicit switching: Unlike Python's built-in coroutines or async/await syntax, greenlets require explicit control over when to switch from one greenlet to another, which is done using the
greenlet.switch()
method.Use Cases:
Greenlets are often used in applications that require high concurrency but don't want the overhead of traditional multithreading.
They are commonly found in networking applications (for example, handling many client connections efficiently) through libraries like gevent.
Stack Saving: A greenlet can save the state of the current stack (variables, code location, etc.) and resume from that state later. This allows for cooperative multitasking between greenlets.
Simple Example:
from greenlet import greenlet
def task1():
print("Task 1 - Part 1")
g2.switch() # Switch to task 2
print("Task 1 - Part 2")
def task2():
print("Task 2 - Part 1")
g1.switch() # Switch back to task 1
print("Task 2 - Part 2")
g1 = greenlet(task1)
g2 = greenlet(task2)
g1.switch() # Start execution with task1
In this example, task1
and task2
switch between each other, creating a form of cooperative concurrency.
Related Libraries:
- gevent: A higher-level library that builds on greenlets and provides more abstract concurrency features like networking and I/O operations.
Greenlets are quite powerful but are not used as widely as Python's modern asynchronous capabilities, such as asyncio
, which provides a higher-level API for concurrency.
Resources
Image attribution
Subscribe to my newsletter
Read articles from Nikhil Akki directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
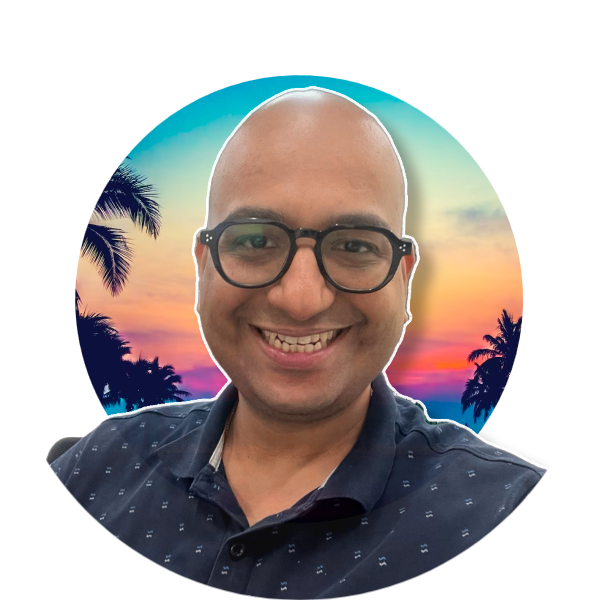
Nikhil Akki
Nikhil Akki
I am a Full Stack Solution Architect at Deloitte LLP. I help build production grade web applications on major public clouds - AWS, GCP and Azure.