Understanding the reduce Method in JavaScript: A Powerful Tool for Array Manipulation π

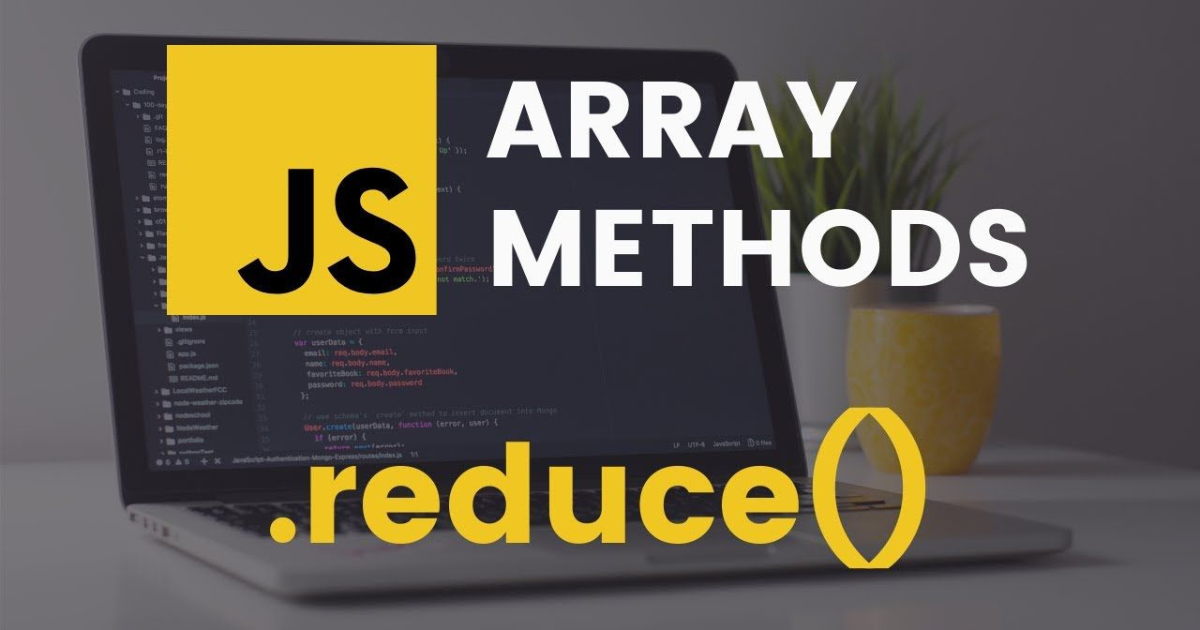
JavaScript offers a variety of methods to work with arrays, and one of the most powerful and versatile is the reduce
method. In this article, we'll explore what reduce
does, how it works, and some practical examples to help you understand its capabilities. Let's dive in! πββοΈ
What is the reduce
Method? π€
The reduce
method executes a reducer function on each element of the array, resulting in a single output value. It takes two parameters:
Reducer Function: A callback function that processes each element.
Initial Value (optional): The value to use as the first argument to the first call of the callback.
Syntax
array.reduce(callback(accumulator, currentValue, currentIndex, array), initialValue);
callback: The function to execute on each element.
accumulator: The accumulated value previously returned in the last invocation.
currentValue: The current element being processed.
currentIndex: The index of the current element (optional).
array: The array
reduce
was called upon (optional).
How Does reduce
Work? βοΈ
The reduce
method iterates through the array, applying the callback function and updating the accumulator. If an initial value is provided, it will be used as the first accumulator value. Otherwise, the first element of the array will be used.
Example 1: Summing an Array of Numbers β
Letβs say we have an array of numbers, and we want to calculate the sum:
const numbers = [1, 2, 3, 4, 5];
const sum = numbers.reduce((accumulator, currentValue) => {
return accumulator + currentValue;
}, 0);
console.log(sum); // Output: 15
In this example, we start with an initial value of 0
. The reduce
method adds each number in the array to the accumulator, resulting in a total sum of 15
.
Example 2: Flattening an Array of Arrays π¦
The reduce
method is also great for flattening an array of arrays. Hereβs how you can do it:
const nestedArray = [[1, 2], [3, 4], [5]];
const flatArray = nestedArray.reduce((accumulator, currentValue) => {
return accumulator.concat(currentValue);
}, []);
console.log(flatArray); // Output: [1, 2, 3, 4, 5]
In this example, we start with an empty array []
. The reduce
method concatenates each sub-array into a single flat array.
Example 3: Counting Instances of Values in an Object π’
You can also use reduce
to count instances of values in an array:
const fruits = ['apple', 'banana', 'orange', 'apple', 'orange', 'banana', 'banana'];
const fruitCount = fruits.reduce((accumulator, fruit) => {
accumulator[fruit] = (accumulator[fruit] || 0) + 1;
return accumulator;
}, {});
console.log(fruitCount);
// Output: { apple: 2, banana: 3, orange: 2 }
Here, we create an object that counts how many times each fruit appears in the array.
When to Use reduce
? π
Transforming Data: When you need to compute a single value from an array.
Accumulating Values: Great for tasks like summing numbers or counting occurrences.
Complex Data Structures: Useful for transforming arrays into objects or other arrays.
Conclusion π
The reduce
method is a powerful addition to your JavaScript toolkit. It simplifies complex data manipulations and provides a clear way to work with arrays. Whether you're summing values, flattening arrays, or counting occurrences, reduce
can help you achieve your goals efficiently.
Now that you know how to use the reduce
method, go ahead and experiment with it in your projects! Happy coding! π
Feel free to comment to modify any part of the article to better fit your style or add more examples if needed!
Subscribe to my newsletter
Read articles from Yasin Sarkar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Yasin Sarkar
Yasin Sarkar
Front-End Developer. I create dynamic web applications using HTML, Tailwind CSS, JavaScript, React, and Next.js. I share my knowledge on social media to help others enhance their tech skills. An Open Source Enthusiast and Writer.