🤔 Understanding the Nullish Coalescing Operator (??) vs. Logical OR (||) in JavaScript

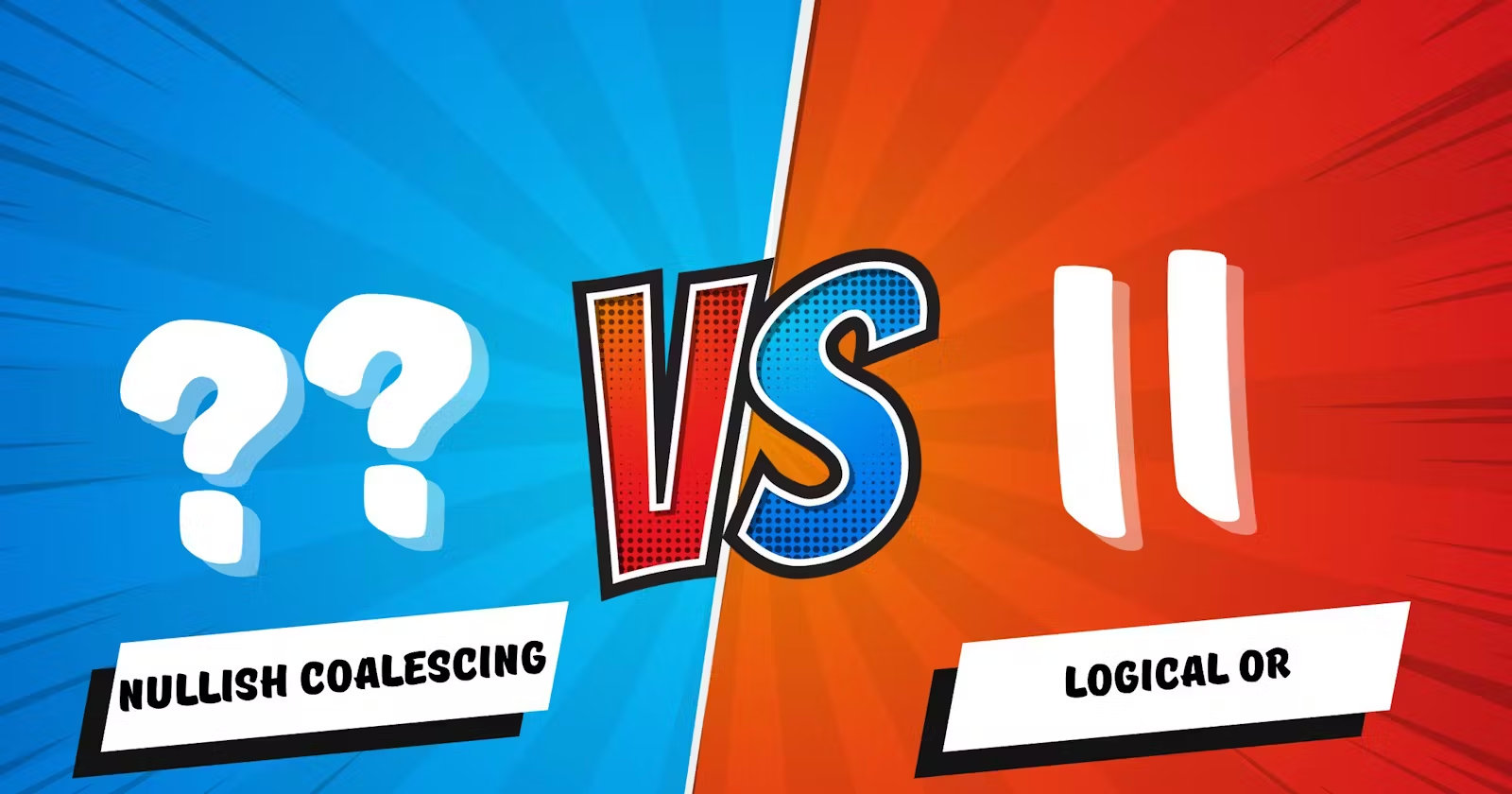
When building JavaScript applications, handling null
and undefined
values is often a crucial part of writing stable and user-friendly code. Two powerful operators can help with this: the Nullish Coalescing Operator (??
) and the Logical OR (||
) operator. While they might seem similar at first glance, they have fundamental differences that can impact how your application behaves.
🔍 What is the Logical OR (||
) Operator?
The Logical OR (||
) operator is widely used in JavaScript to return the first truthy value it encounters from a pair of expressions. It is often used as a fallback mechanism when a variable might be undefined
or another falsy value.
Example of ||
:
let username = "";
let displayName = username || "Anonymous";
console.log(displayName); // Output: "Anonymous"
In this example, username
is an empty string (""
), which is considered falsy in JavaScript. As a result, the ||
operator evaluates the right-hand side and returns "Anonymous"
as the fallback value.
🤓 What is the Nullish Coalescing Operator (??
)?
The Nullish Coalescing Operator (??
) was introduced in ES2020 as a more specific way to handle null
or undefined
. It returns the right-hand operand only if the left-hand operand is nullish—meaning it is either null
or undefined
.
Example of ??
:
let username = "";
let displayName = username ?? "Anonymous";
console.log(displayName); // Output: ""
In this case, username
is still an empty string (""
), but the ??
operator treats empty strings as valid values. Thus, it does not consider them as nullish and returns the actual value of username
.
⚖️ Key Differences Between ||
and ??
The main difference between ||
and ??
lies in how they handle falsy values like 0
, NaN
, ""
, false
, and undefined
:
1. Falsy Values vs. Nullish Values:
• ||
treats all falsy values—such as 0
, ""
(empty string), false
, NaN
—as a reason to use the fallback.
• ??
is more restrictive and only considers null
and undefined
as reasons to fall back.
2. When to Use ||
:
• Use ||
if you want to handle a wide range of values that could be considered empty, such as 0
, ""
, or NaN
.
• Example: Setting default values when users have not provided inputs.
3. When to Use ??
:
• Use ??
if you want to ensure that only null
or undefined
triggers the fallback value.
• Example: Preserving 0
or ""
as valid user inputs while still providing a fallback for truly missing values.
🚀 Practical Use Cases
Let's explore a few real-world examples where using ??
or ||
makes a difference:
Example 1: Default Values for Numbers 💵
Imagine you have a price value that could be 0
:
let price = 0;
let displayPrice = price || 100;
console.log(displayPrice); // Output: 100
Here, price
is 0
, which is a falsy value. The ||
operator treats it as equivalent to false
, so it uses 100
as the fallback.
With the ??
operator:
let price = 0;
let displayPrice = price ?? 100;
console.log(displayPrice); // Output: 0
In this case, ??
respects that 0
is a valid value and does not use the fallback.
Example 2: Handling User Input 📝
Consider a form where users can provide an optional nickname:
let nickname = "";
let displayName = nickname || "Guest";
console.log(displayName); // Output: "Guest"
The empty string is treated as falsy, so ||
falls back to "Guest"
.
With ??
:
let nickname = "";
let displayName = nickname ?? "Guest";
console.log(displayName); // Output: ""
Using ??
, the empty string is seen as a valid value, so the user's input is preserved.
📊 Summary Table
Here's a quick comparison between the two operators:
🔍 Operator | ✔️ Checks for | 🚨 Returns Fallback For | 💡 Use Case | ||
All falsy values | All falsy values | ||||
?? | Only nullish values | null , undefined | Use when only null or undefined should trigger a fallback. |
🧠 Common Pitfalls
1. Using ||
Instead of ??
:
- Developers often use
||
when they want to handle default values, but it can be too aggressive, treating0
and empty strings as invalid. This can cause unintended behavior, especially when these values are meaningful.
2. Browser Compatibility:
- The
??
operator is relatively new (introduced in ES2020). If you are working with older JavaScript environments, ensure that your code is transpiled using tools like Babel for broader compatibility.
🎯 Conclusion
Understanding the difference between the ||
and ??
operators is crucial for writing clear and predictable JavaScript. While ||
is versatile and widely used, it can be too aggressive in treating values like 0
and empty strings as invalid. The ??
operator, on the other hand, provides a more refined approach, allowing you to handle null
and undefined
without sacrificing the validity of other values.
By choosing the right operator for your specific use case, you can make your JavaScript code more reliable and intuitive, enhancing the overall user experience. Happy coding! 💻✨
Feel free to inform me by comment if I make any mistakes so I can correct them.
Subscribe to my newsletter
Read articles from Yasin Sarkar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Yasin Sarkar
Yasin Sarkar
Front-End Developer. I create dynamic web applications using HTML, Tailwind CSS, JavaScript, React, and Next.js. I share my knowledge on social media to help others enhance their tech skills. An Open Source Enthusiast and Writer.