Protecting Your API with Unkey: A Guide to API Security and Rate Limiting
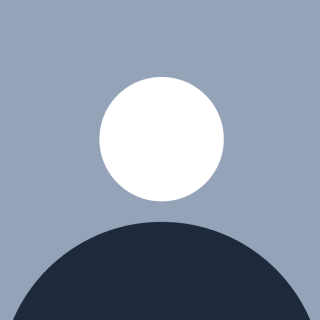
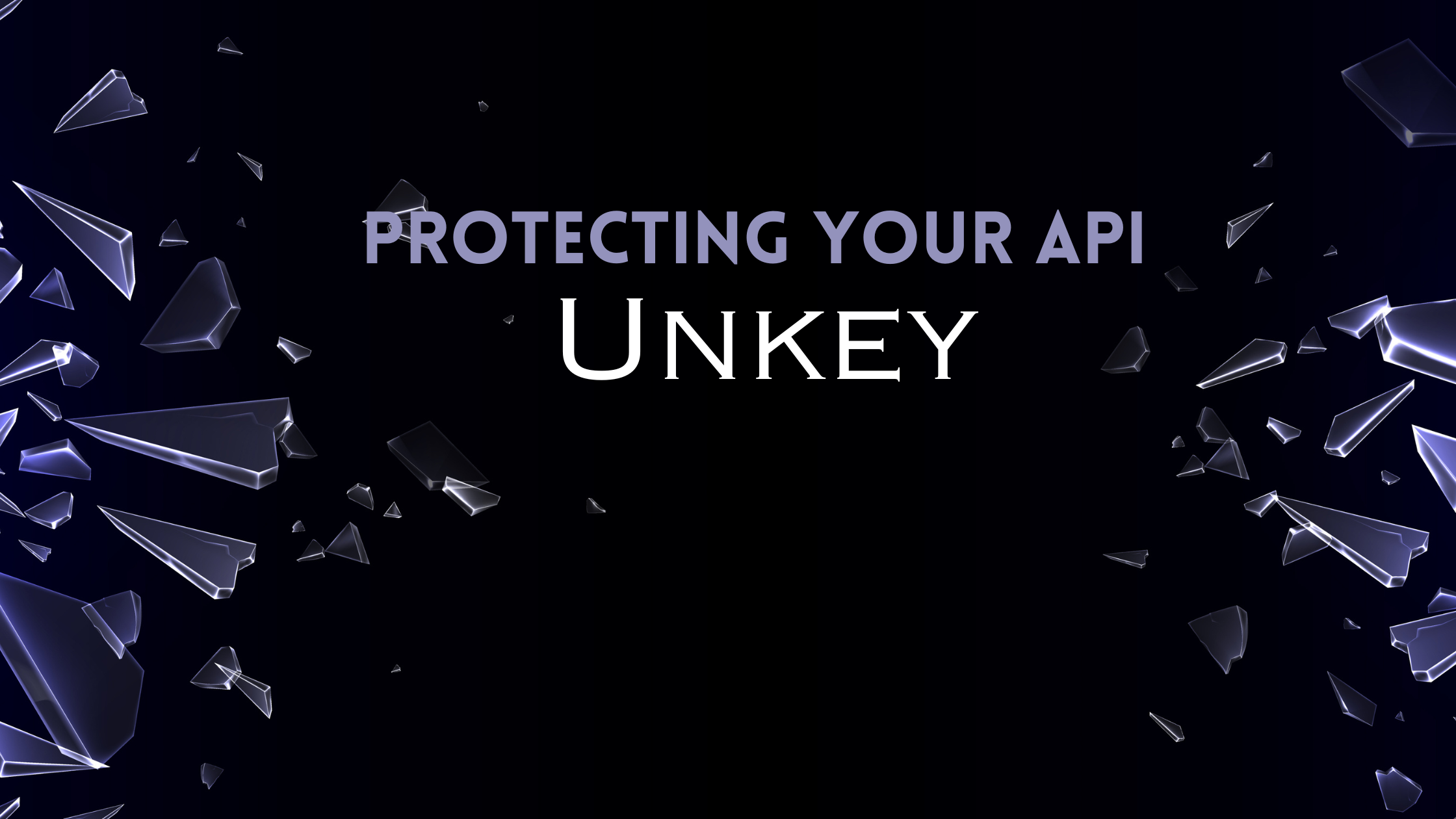
APIs are the backbone of modern applications, for seamless communication between different services.However, securing these APIs is crucial to prevent unauthorized access and abuse. Unkey offers a simple yet powerful solution for protecting APIs with authentication keys and rate limiting for user-triggered actions.
we will explore how to integrate Unkey into an Express.js application and demonstrate its features through code examples.
Why Use Unkey?
Unkey provides a fast and flexible way to secure your APIs. It offers:
API Key Verification: Ensure that only authorized users can access your API.
Rate Limiting: Control the number of requests a user can make over a specific time period, preventing abuse and ensuring fair usage.
Serverless Caching: Unkey has implemented an efficient caching solution, which can be installed using
npm i @unkey/cache.
This feature allows for faster API responses and reduced server load, especially beneficial for serverless architectures.Audit Logs: Unkey now provides automatic audit trails for API activities, allowing developers to track changes and monitor API usage for security and compliance purposes.
Unkey helps you quickly implement API security without having to manage complex setups manually.
Why Use Unkey for Rate Limiting?
Rate limiting is an essential feature that prevents users from overwhelming your API with requests, which can lead to server crashes, increased costs, and a poor user experience. By using Unkey, you gain the ability to implement:
Global Rate Limits: Set limits that apply to all users collectively.
Key-Specific Rate Limits: Tailor limits based on individual users or API keys.
Setting Up Unkey in Your Application
First, ensure you have the necessary packages installed in your project.
npm install @unkey/api @unkey/ratelimit express dotenv
In your main application file (e.g., index.js
), set up your Express server and configure Unkey:
import express from "express";
import dotenv from "dotenv";
import appRoutes from "./routes/apiRoute.js";
import { Ratelimit } from "@unkey/ratelimit";
import { Unkey } from '@unkey/api';
dotenv.config();
export const unkey = new Unkey({token: process.env.UNKEY_KEY});
export const ratelimit = new Ratelimit({
rootKey: process.env.UNKEY_KEY,
namespace: "system-api",
limit: 100, // Global limit of 100 requests
duration: "1m", // Within a 1-minute window
async: true
});
export const app = express();
app.use(express.json());
app.use(appRoutes);
app.listen(3000, () => {
console.log("Server is running on port 3000");
});
Implement API Key Management
Create a controller to manage API keys. Here’s an example of how to create, retrieve, and update API keys:
// Testing Paid API controller
export const paidApiData = async (req, res) =>{
const globalIdentifier = "system-api"; // Global rate limit identifier
const {success} = (await ratelimit.limit(globalIdentifier))
if(!success){
return res.status(429).json({ message: "Global rate limit exceeded" });
}
return res.json({
message : "You can access Api"
})
}
Verifying API Keys
Create a middleware to verify the provided API keys.
import { verifyKey } from "@unkey/api";
async function verifyApiKey(req, res, next) {
const apiKey = req.headers['x-api-key'];
if (!apiKey) {
return res.status(401).json({ message: 'API key missing' });
}
const { error, result } = await verifyKey({ key: apiKey });
if (!result.valid) {
return res.status(403).json({ message: 'Invalid API key' });
}
if (error) {
return res.status(error.code).json({ message: error.message });
}
next();
}
export default verifyApiKey;
Defining API Routes
Now, define your routes for the API key management and rate limiting.
javascriptCopy codeimport express from "express";
import { createAPIKey, getAllKeys, paidApiData } from "../controller/apiController.js";
import verifyApiKey from "../middleware/keyVerification.js";
const router = express.Router();
router.get("/api", verifyApiKey, paidApiData);
export default router;
Analytics and Rate Limiting with Unkey
When using Unkey's rate limiter, one of the key features that sets it apart is the rich analytics it provides. This analytics data can be filtered around identifiers, giving you insights into how your application is being used. Whether it’s API keys, user actions, or global identifiers, this allows you to pinpoint what areas of your application receive the most traffic and which users or keys are driving that traffic.
Conclusion
By integrating Unkey into your Node.js application, you can easily secure your APIs with robust key management and implement rate limiting to prevent abuse.
For further details and to implement Unkey in your application, check out Unkey's documentation.
Subscribe to my newsletter
Read articles from Utkarsh Jaiswal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Utkarsh Jaiswal
Utkarsh Jaiswal
My name is Utkarsh. I am a software developer and computer science student passionate about programming. In recent years, I've worked with C++, JavaScript, TypeScript, and PHP, as well as frameworks and libraries such as Node.js, React.js, and Symfony. I have experience in e-commerce-related projects, including building admin dashboards, e-commerce frontends, and creating backend systems and database management. You can find me on LinkedIn, GitHub, and Hashnode.