๐งฉ Solving Request Context Mysteries in Spring Boot with Async Services ๐
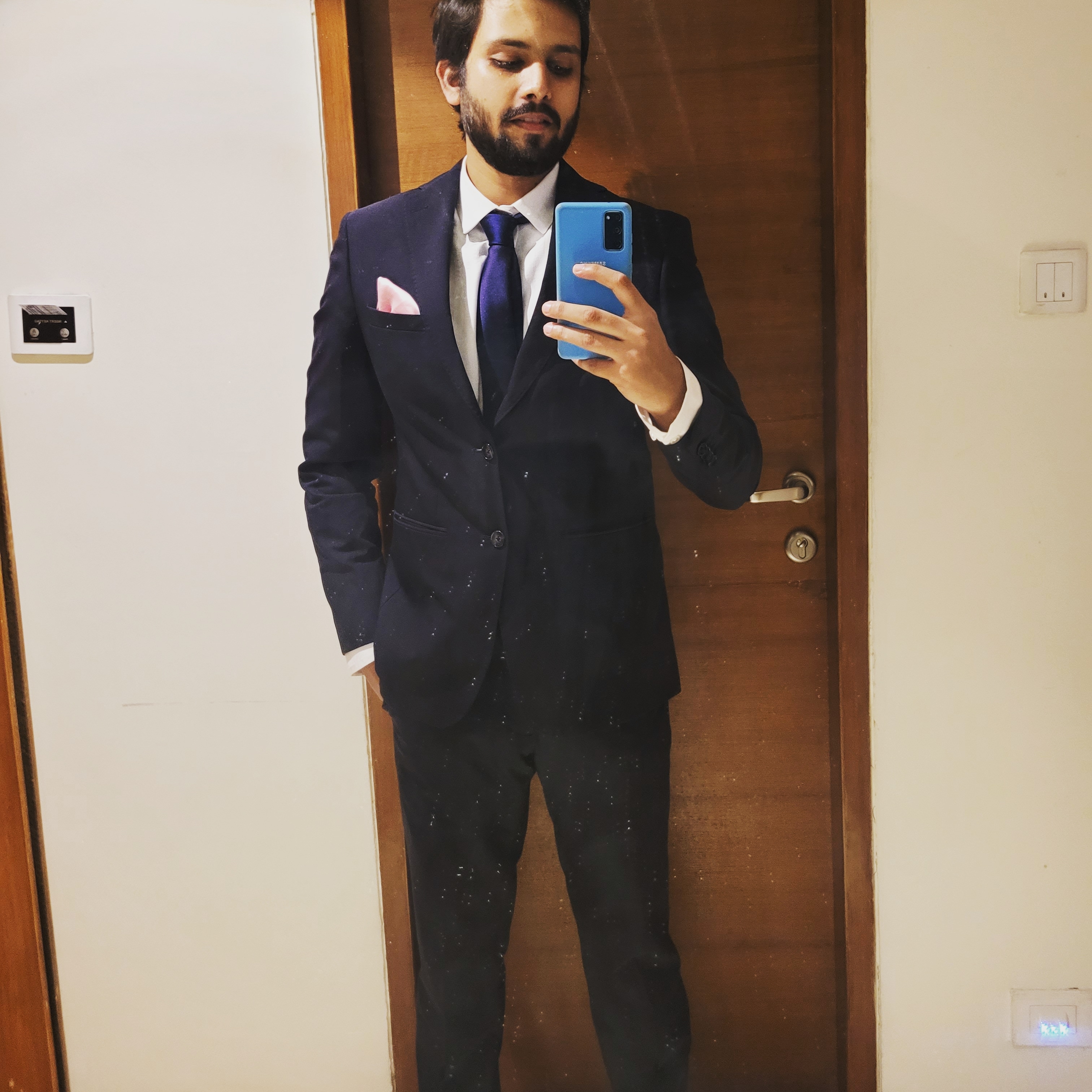
Table of contents
- ๐ The Problem: โHelp! My Request Context is Gone!โ ๐
- ๐ง My Initial Attempts and โSolutionsโ ๐ง
- โจ Adding the TaskDecorator Magic โจ
- ๐ง Setting Up the Async Configuration
- ๐ The Breakthrough: โJust Donโt Discard the Facade!โ ๐
- ๐ก The Solution: One Line of Code to Save the Day! ๐ชโจ
- ๐ Key Lessons Learned (a.k.a., How I Stopped Worrying and Learned to Love Async)
- ๐ค Why Did This Work?
- ๐ Wrapping Up: My Async Troubles Are Over... For Now ๐ป
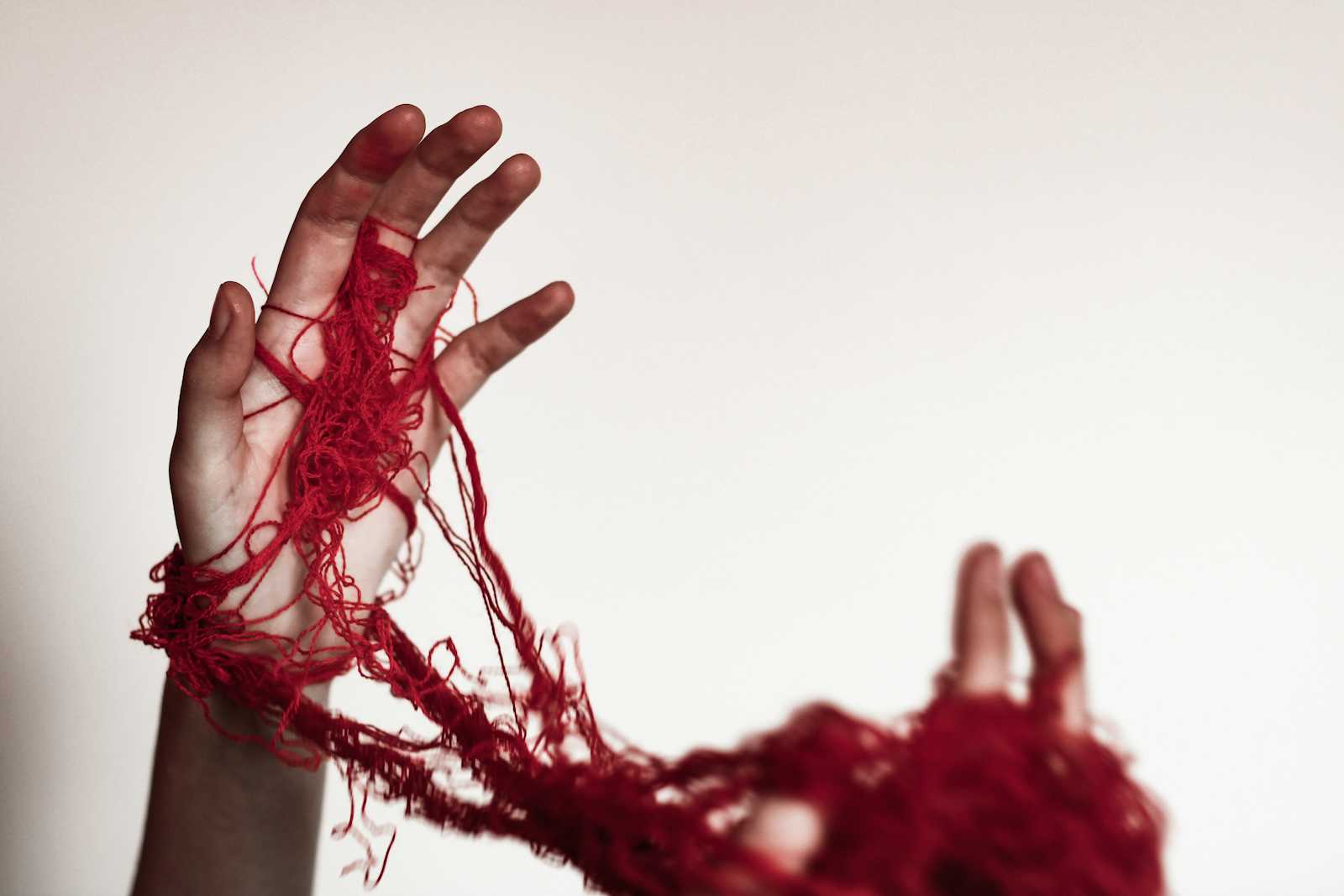
Ah, Spring Boot. It's like a magic wand for Java developers โ until it suddenly isnโt. Recently, I found myself in a bit of a pickle while working with Spring Boot (3.x) and Tomcat 10+. What started as a simple asynchronous service turned into a wild goose chase to get my hands on the request context. ๐คฏ
Let me take you on the journey of what went wrong, my troubleshooting adventures, and how I finally cracked the case. ๐ต๏ธโโ๏ธ๐
๐ The Problem: โHelp! My Request Context is Gone!โ ๐
Picture this: You have a Spring Boot controller that receives a request, calls an async service, and returns a response โ all while your async service works its magic in the background. ๐ฉโจ
Sounds great, right? Until I tried accessing the request context inside the async service. Every time, I hit a NullPointerException
faster than I could say โRequestContextHolder
โ ๐ฌ.
Error message:Cannot invoke web.context.request.ServletRequestAttributes.getRequest() because "attributes" is null
Apparently, in an async method, the original request context doesnโt carry over to the new thread by default. In short, the request was out partying elsewhere, leaving me with nothing but null. ๐๐
๐ง My Initial Attempts and โSolutionsโ ๐ง
Passing the
HttpServletRequest
directly to the async method:
Seemed like a solid plan at first. But nope! As the async method was executed after the controller returned, the original request was long gone. ๐ถโโ๏ธ๐จUsing a
TaskDecorator
to manually copy the context:
This was like using duct tape to fix a leaky pipe โ it kind of worked but wasnโt reliable enough for certain request attributes. ๐ฉน๐
โจ Adding the TaskDecorator
Magic โจ
To keep the request context alive in async threads, I added a custom TaskDecorator
. Hereโs what it looked like:
@Component
public class ContextCopyingTaskDecorator implements TaskDecorator {
@Override
public Runnable decorate(Runnable runnable) {
// Capture the current request attributes
RequestAttributes context = RequestContextHolder.currentRequestAttributes();
return () -> {
try {
// Set the request attributes for this thread
RequestContextHolder.setRequestAttributes(context);
runnable.run();
} finally {
// Reset the request attributes after execution
RequestContextHolder.resetRequestAttributes();
}
};
}
}
This decorator captures the current request attributes and sets them for the new thread that runs the async task. Itโs like copying the keys to a new door so the request context can still get in. ๐ ๐
๐ง Setting Up the Async Configuration
Then, I configured the TaskDecorator
in my async setup:
@Configuration
public class AsyncConfig implements AsyncConfigurer {
@Autowired
private ContextCopyingTaskDecorator taskDecorator;
@Override
public Executor getAsyncExecutor() {
ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor();
executor.setCorePoolSize(5);
executor.setMaxPoolSize(10);
executor.setQueueCapacity(25);
executor.setTaskDecorator(taskDecorator);
executor.initialize();
return executor;
}
}
The custom TaskDecorator
worked well in most scenarios, but for some strange reason, I still occasionally found that the request context wasnโt available. ๐ค
๐ The Breakthrough: โJust Donโt Discard the Facade!โ ๐
After some deep digging and desperate Googling ๐โโ๏ธ๐ป, I stumbled upon the culprit: Tomcatโs discardFacade
setting.
By default, Tomcat throws away the request facades for security reasons, which is a problem when you want to access request info in an async context.
๐ก The Solution: One Line of Code to Save the Day! ๐ชโจ
The fix turned out to be surprisingly simple โ just a one-liner configuration to tell Tomcat to stop discarding facades:
@Bean
TomcatConnectorCustomizer disableFacadeDiscard() {
return (connector) -> connector.setDiscardFacades(false);
}
Yep, that's it! I created this bean in the configuration class to disable facade discard, and boom ๐ฅ! The request attributes were available even after the original request had completed. ๐๐
๐ Key Lessons Learned (a.k.a., How I Stopped Worrying and Learned to Love Async)
Context Doesnโt Follow You (Unless You Tell It To):
In async processing, the request context is like a pet cat ๐ฑ โ it does its own thing. If you need it to follow you around, youโll have to configure it correctly.Tomcat's Facade Management Matters:
Disabling thediscardFacade
property can be a game-changer when you need access to the request context in async scenarios.Avoid Overcomplicated Workarounds:
Custom decorators and directly passing theHttpServletRequest
might seem like clever ideas at first, but they can be unreliable. Sometimes, the simplest solutions really are the best. ๐งโ๐ง๐ง
๐ค Why Did This Work?
By setting discardFacades
to false
, Tomcat doesnโt throw away the request data prematurely. This allows the async service to access the original request information even after the controller has returned the response. Itโs like giving the request a backstage pass to the async show. ๐ซ๐ค
๐ Wrapping Up: My Async Troubles Are Over... For Now ๐ป
So, if youโre ever struggling with Spring Boot, async services, and request context woes, give this solution a try. You might just save yourself hours of frustration โ and several cups of coffee. โ๏ธ๐
Subscribe to my newsletter
Read articles from Yash Raj Srivastav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
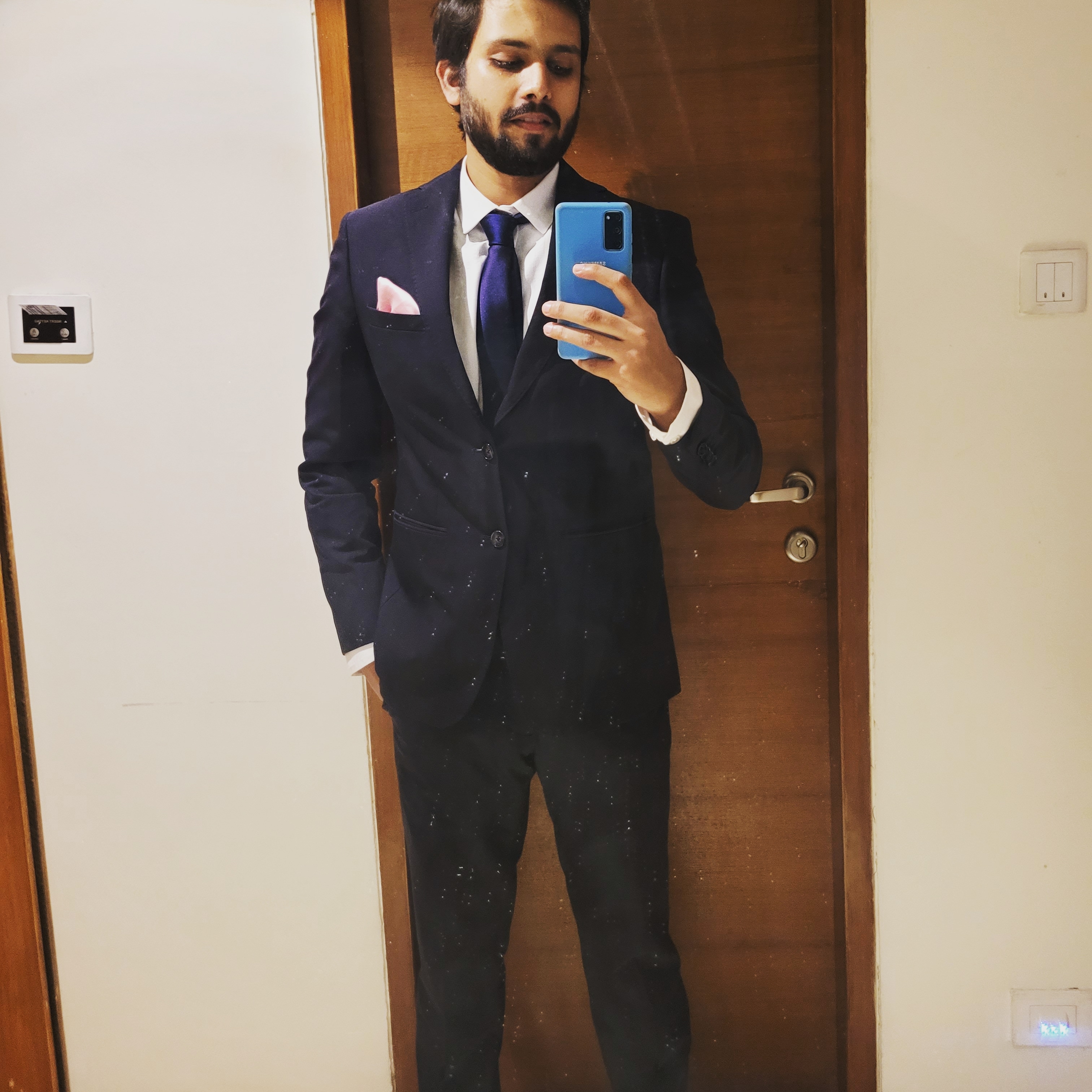
Yash Raj Srivastav
Yash Raj Srivastav
Software Developer + DevOps | Tech Blogger | DSA Practitioner | Java | SpringBoot(security, batch, cloud) | TypeScript | Python | Bash Script | Kafka | Redis | Payment Gateways | AWS | Azure | Docker | Kubernetes | Ansible | Jenkins | GitHub Actions