The Ant Colony’s Network
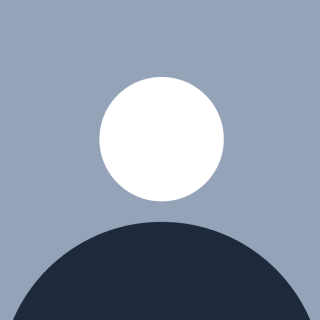
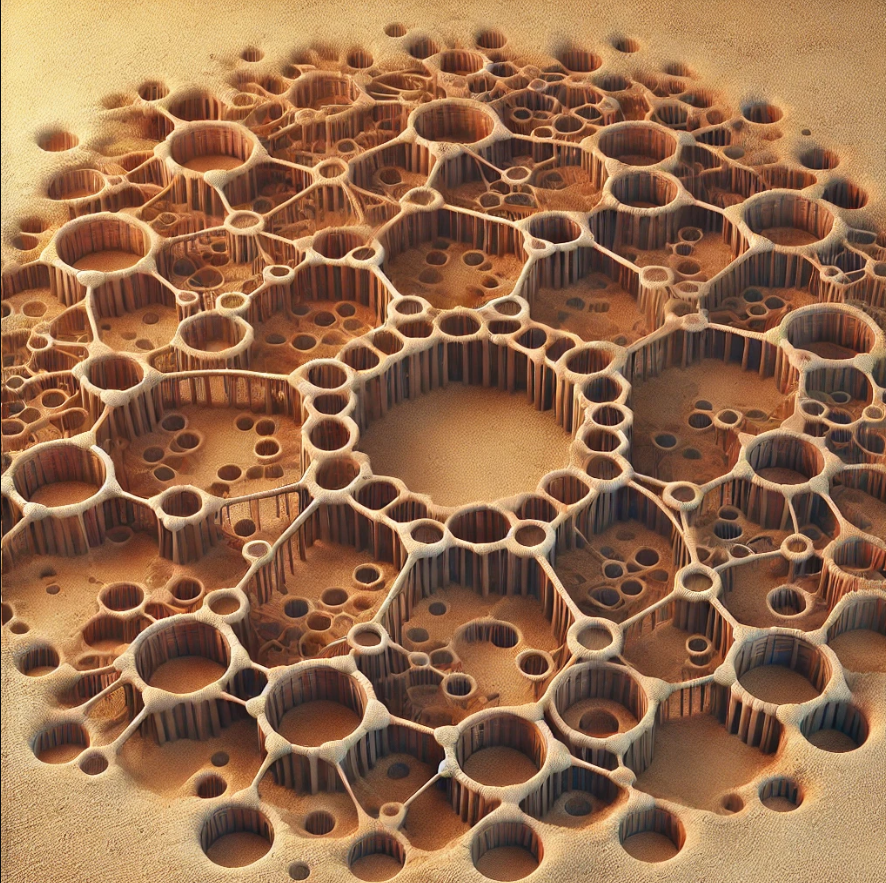
Imagine walking through a vast ant colony where thousands of chambers are interconnected by tunnels. Each tunnel leads to a different chamber, and every chamber has multiple paths leading out. This labyrinth is more than just a network of tunnels; it’s an organized structure that guides the ants’ journey.
Now, think of this ant colony as a graph, a powerful data structure used to model relationships between objects. Whether it’s mapping out social media connections, city road networks, or web page links, graphs help represent these relationships.
In this article, we’ll introduce you to the world of graphs through the lens of an ant colony. By the end, you’ll not only understand what a graph is but also know how to represent and build graphs using code.
Simple Graphs – The Ant Colony’s Tunnels
Let’s start with the basics. A graph is made up of nodes (also called vertices) and edges (connections between nodes). Think of the chambers in the ant colony as nodes, and the tunnels connecting them as edges. Every time the ants move through a tunnel, they’re traveling along an edge from one node to another.
But not all tunnels are the same:
Some tunnels are one-way only, meaning ants can only travel from one chamber to another but not back. This represents a directed edge in a graph.
Other tunnels allow two-way travel, where ants can move freely back and forth. This is an undirected edge.
In some cases, tunnels might be easier or harder for ants to traverse. These differences in difficulty are captured by assigning a weight to the edges.
Why Graphs Are So Useful
Graphs aren’t just abstract concepts; they represent real-world systems. From traffic networks to recommendation engines, graphs are behind many of the technologies we use every day. In a social media network, people are nodes, and their friendships are edges. In a city map, intersections are nodes, and roads are edges.
What is a Graph? A Journey Through the Ant Colony
Graphs help us map out and explore complex relationships, much like how ants navigate their colony.
Vertices (Nodes)
Each vertex represents a unique object or entity. In the ant colony, each chamber is a node, and ants can reside in any chamber.
Example:
Imagine a social network where each person is a node, and their connections to other people are edges. Your friends would be connected to you by edges, just like chambers in the ant colony are connected by tunnels.
Edges
Edges represent the connections between vertices. In the ant colony, these edges are the tunnels that allow ants to travel between chambers. But not all edges are the same:
Directed Edge: A tunnel that only allows one-way travel, like a one-way street in a city.
Undirected Edge: A tunnel that allows ants to travel back and forth freely, like a two-way road.
Weighted vs. Unweighted Graphs
Some tunnels are wide and easy to traverse, while others are narrow and difficult. In graphs, these differences are represented by weights. A weighted graph assigns a value (weight) to each edge, representing the difficulty of traveling along that edge.
Weighted Graphs: The tunnels in the ant colony might have different widths, which represent the difficulty (weight) of traveling through them. A larger tunnel is easier (lower weight), while a narrow tunnel is harder (higher weight).
Unweighted Graphs: All tunnels are equal. Ants don’t care how long or difficult the tunnel is—it’s the same no matter what.
Representing Graphs: Mapping the Colony with Adjacency Lists
Now that you understand what a graph is, how do we represent it in code? Imagine that the ants have a map of their colony, showing which chambers are connected by tunnels. There are different ways to build this map, but one of the most efficient methods is using an adjacency list.
Adjacency List: A Map for the Ants
An adjacency list is a compact way of representing a graph, especially when not every node is connected to every other node. Each node maintains a list of all the other nodes it’s directly connected to. It’s like the ants in each chamber keep a list of the tunnels that lead out to other chambers.
Code Example – Adjacency List:
class Graph:
def __init__(self):
self.graph = {} # Dictionary to store the adjacency list
def add_edge(self, u, v):
# If the node u is not in the graph, add it with an empty list
if u not in self.graph:
self.graph[u] = []
# Similarly, add v to u's list and vice versa for an undirected graph
if v not in self.graph:
self.graph[v] = []
self.graph[u].append(v)
self.graph[v].append(u) # Undirected graph, add edge in both directions
def display_graph(self):
for node in self.graph:
print(f"{node}: {self.graph[node]}")
# Example usage
ant_colony = Graph()
ant_colony.add_edge('A', 'B')
ant_colony.add_edge('A', 'C')
ant_colony.add_edge('B', 'D')
ant_colony.add_edge('C', 'D')
ant_colony.display_graph()
# Output:
# A: ['B', 'C']
# B: ['A', 'D']
# C: ['A', 'D']
# D: ['B', 'C']
Explanation
In this example, the ant colony is represented as an undirected graph. Each node (chamber) keeps a list of its neighbors (connected chambers). For instance, chamber A has tunnels to B and C, so its adjacency list shows
A: ['B', 'C']
.This method is memory efficient because it only stores the edges that exist. If a chamber doesn’t have many tunnels, the adjacency list will be short, which saves space.
Strengths of Adjacency Lists
Efficient for Sparse Graphs: If not every node is connected to many others, an adjacency list saves memory by only listing the edges that exist. For the ants, this means they don’t need to remember unnecessary tunnels between distant chambers they never visit.
Easy to Traverse: It’s easy to find neighbors of a node by simply looking at its list. For the ants, this means they can quickly see which other chambers they can reach.
Drawbacks of Adjacency Lists
- Edge Lookups Can Be Slow: If the ants need to check whether a specific tunnel exists between two chambers, they might have to search through the entire list of tunnels. This is not a problem for small colonies but could slow them down in larger ones.
Optimizing the Ants’ Map: Adjacency List Methods
Let’s dive deeper into what we can do with adjacency lists. Apart from adding edges, there are other common operations we can perform on our ant colony’s map.
Methods to Optimize the Ant Colony Map
Finding Neighbors: Given a chamber, we can easily return all the neighboring chambers using the adjacency list.
Removing Edges: If a tunnel collapses and ants can no longer use it, we need to remove the edge from the adjacency list.
Checking Connectivity: The ants might need to check if there’s a tunnel between two chambers. This involves searching the adjacency list for a connection.
Example – Finding Neighbors and Removing Edges:
class Graph:
def __init__(self):
self.graph = {}
def add_edge(self, u, v):
if u not in self.graph:
self.graph[u] = []
if v not in self.graph:
self.graph[v] = []
self.graph[u].append(v)
self.graph[v].append(u)
def remove_edge(self, u, v):
if u in self.graph:
self.graph[u].remove(v)
if v in self.graph:
self.graph[v].remove(u)
def get_neighbors(self, node):
return self.graph.get(node, [])
def display_graph(self):
for node in self.graph:
print(f"{node}: {self.graph[node]}")
# Example usage
ant_colony = Graph()
ant_colony.add_edge('A', 'B')
ant_colony.add_edge('A', 'C')
ant_colony.add_edge('B', 'D')
ant_colony.add_edge('C', 'D')
print("Neighbors of A:", ant_colony.get_neighbors('A')) # Output: ['B', 'C']
ant_colony.remove_edge('A', 'C')
ant_colony.display_graph()
# Output:
# A: ['B']
# B: ['A', 'D']
# C: ['D']
# D: ['B', 'C']
Explanation
Neighbors: The ants in chamber A can see that they are connected to B and C (
get_neighbors('A')
).Removing Edges: If the tunnel between A and C collapses, we can remove that edge using
remove_edge('A', 'C')
, and the adjacency list is updated accordingly.
The adjacency list is an efficient and flexible way for the ants to map out their colony. For graphs where not all nodes are connected, adjacency lists help conserve memory and make it easy to traverse the colony. However, for larger colonies, it can take time to search through the tunnels to find specific connections.
Mapping the Ant Colony with Adjacency Matrices
Adjacency lists are indeed a simple and memory-efficient way to track connections between chambers. But what if the colony is much larger and densely packed with tunnels? In such cases, adjacency lists may not be the most efficient option for quick lookups.
Enter the adjacency matrix—a grid-like representation that can make it much faster to check if two chambers are connected. Next, we’ll explore how adjacency matrices work, where they shine, and some of their limitations. By the end, you'll understand when to use adjacency matrices and how to work with them in code.
Adjacency Matrix: The Grid that Maps Every Tunnel
An adjacency matrix is a two-dimensional array where rows and columns represent nodes (chambers), and the value at each cell tells whether an edge (tunnel) exists between them.
Ant Colony Analogy:
Imagine a giant grid covering the entire colony. Each row and column represent a specific chamber, and each cell in the grid answers a simple question: Is there a tunnel between these two chambers? If there is, we mark the cell with a 1
; if there isn’t, we mark it with a 0
.
This matrix representation allows ants (and us) to quickly check if a tunnel exists between any two chambers by simply looking at the grid.
Code Example – Adjacency Matrix:
Let’s see how we can represent the ant colony using an adjacency matrix.
import numpy as np
class GraphMatrix:
def __init__(self, size):
# Initialize a size x size matrix filled with zeros
self.graph = np.zeros((size, size))
def add_edge(self, u, v):
# For an undirected graph, mark both directions
self.graph[u][v] = 1
self.graph[v][u] = 1
def display_graph(self):
print(self.graph)
# Example usage
ant_colony = GraphMatrix(4) # 4 chambers (nodes)
ant_colony.add_edge(0, 1) # Tunnel between chamber 0 and 1
ant_colony.add_edge(0, 2) # Tunnel between chamber 0 and 2
ant_colony.add_edge(1, 3) # Tunnel between chamber 1 and 3
ant_colony.add_edge(2, 3) # Tunnel between chamber 2 and 3
ant_colony.display_graph()
# Output (Adjacency Matrix):
# [[0. 1. 1. 0.]
# [1. 0. 0. 1.]
# [1. 0. 0. 1.]
# [0. 1. 1. 0.]]
Explanation:
In this matrix:
Rows and columns represent the chambers (nodes).
A
1
at the intersection of rowi
and columnj
means there’s a tunnel between chamberi
and chamberj
.A
0
means no tunnel exists between the two chambers.
For example, in the output above, there’s a tunnel between chambers 0 and 1 (represented by 1
in cell [0][1]
and [1][0]
), but no tunnel between chambers 0 and 3 (represented by 0
in cell [0][3]
).
Strengths and Weaknesses of Adjacency Matrices
Adjacency matrices are powerful for certain kinds of graphs but can become a burden for others. Let’s break down their strengths and weaknesses.
Strengths of Adjacency Matrices
Quick Lookups: The greatest strength of an adjacency matrix is that you can check if an edge exists between two nodes in constant time O(1). Just like how the ants can look at the matrix and instantly see if a tunnel exists between two chambers, this speed is perfect for dense graphs with lots of connections.
Better for Dense Graphs: If most chambers (nodes) are connected, adjacency matrices are efficient because we don’t waste time and memory listing every single connection like we do in an adjacency list.
Weaknesses of Adjacency Matrices
Memory Usage: In sparse graphs, where most nodes aren’t connected, adjacency matrices can become memory hogs. The matrix stores information for every possible connection, even if no tunnel exists. For large colonies with few tunnels, this is inefficient.
Difficult to Traverse: Traversing a node’s neighbors in an adjacency matrix is slower than with an adjacency list. In the matrix, the ants would have to scan through every cell in a row to find which nodes are connected to the current one.
When to Use Adjacency Matrices
Dense Graphs: When most nodes are connected to each other, adjacency matrices are the better option because of their quick edge lookups.
Graphs with Constant Edge Queries: If you need to frequently check if a specific connection exists, adjacency matrices can save you time compared to adjacency lists.
Working with Adjacency Matrices: Common Operations
Now that we know how adjacency matrices work, let’s look at some common operations we can perform on them.
Adding an Edge
In the code example above, adding an edge is as simple as updating the matrix. We mark the corresponding cell with a 1
for undirected graphs or just mark one direction for directed graphs.
Removing an Edge
Removing a tunnel is easy. We just reset the value in the matrix to 0
.
def remove_edge(self, u, v):
self.graph[u][v] = 0
self.graph[v][u] = 0 # For undirected graphs, remove the edge in both directions
Finding Neighbors
Unlike adjacency lists where neighbors are listed explicitly, finding neighbors in an adjacency matrix requires us to scan through a row in the matrix and look for 1
s.
def get_neighbors(self, node):
return [i for i in range(len(self.graph)) if self.graph[node][i] == 1]
# Example usage
print("Neighbors of chamber 0:", ant_colony.get_neighbors(0))
# Output: Neighbors of chamber 0: [1, 2]
Explanation:
- In this case, the ants are in chamber 0, and they want to see which chambers they can reach directly. By scanning the matrix, they find tunnels leading to chambers 1 and 2.
Adjacency Matrix vs. Adjacency List: Which Is Better for the Colony?
Both adjacency lists and matrices have their uses, but when should you use one over the other?
Adjacency List: Pros and Cons
Pros:
Efficient for sparse graphs where most nodes don’t have many connections.
Easy to traverse and find neighbors.
Cons:
- Checking if an edge exists between two nodes takes O(n) time, since you have to scan the entire neighbor list for the node.
Adjacency Matrix: Pros and Cons
Pros:
Fast edge lookups: Checking if an edge exists takes O(1) time.
Great for dense graphs, where most nodes are connected.
Cons:
Memory intensive: Requires O(n²) space, even if there are few connections.
Traversing neighbors is slower than with adjacency lists.
When to Choose Each:
If the ant colony is small and not many chambers are connected, go with an adjacency list. It saves memory and makes traversing easy.
If the colony is large and densely connected, and you frequently need to check if specific tunnels exist, an adjacency matrix is your best bet.
Optimizing the Colony: Tips for Efficient Graph Use
Use Adjacency Lists for Sparse Graphs: If your graph has relatively few edges, an adjacency list will help you save memory.
Leverage Adjacency Matrices for Dense Graphs: For densely connected graphs, matrices allow for quick lookups and are worth the memory overhead.
Directed vs. Undirected: Always consider whether your tunnels (edges) are directed or undirected. Directed edges take up less space in adjacency lists but can complicate traversal algorithms.
Challenge Time – Test Your Skills!
Challenge 1: Modify the adjacency list code to implement directed graphs, where some tunnels are one-way only.
Challenge 2: Implement a method to check if two nodes are directly connected by an edge in an adjacency list.
Challenge 3: Modify the adjacency matrix code to implement a directed graph, where some tunnels are one-way.
Challenge 4: Implement a function that finds and returns the total number of edges (tunnels) in an adjacency matrix.
Think you’ve mastered adjacency matrices? Try out the challenges and share your solutions!
The Colony’s Complete Map
In this article, we expanded the ant colony’s map by using adjacency matrices, a powerful tool for representing densely connected graphs. While adjacency lists are great for sparse colonies, adjacency matrices shine in dense ones where quick edge lookups are important. As you continue to work with graphs, choosing the right representation will help you balance memory usage and performance.
We have now laid the foundation for working with graphs by introducing these two fundamental representations. But this is just the beginning! Graphs are an incredibly versatile and powerful tool in computer science, with applications ranging from social networks to navigation systems.
Want to explore graph traversals next? Stay tuned for the next article where we dive into graph traversal algorithms like BFS and DFS!
Subscribe to my newsletter
Read articles from gayatri kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by