Java Project For Beginners

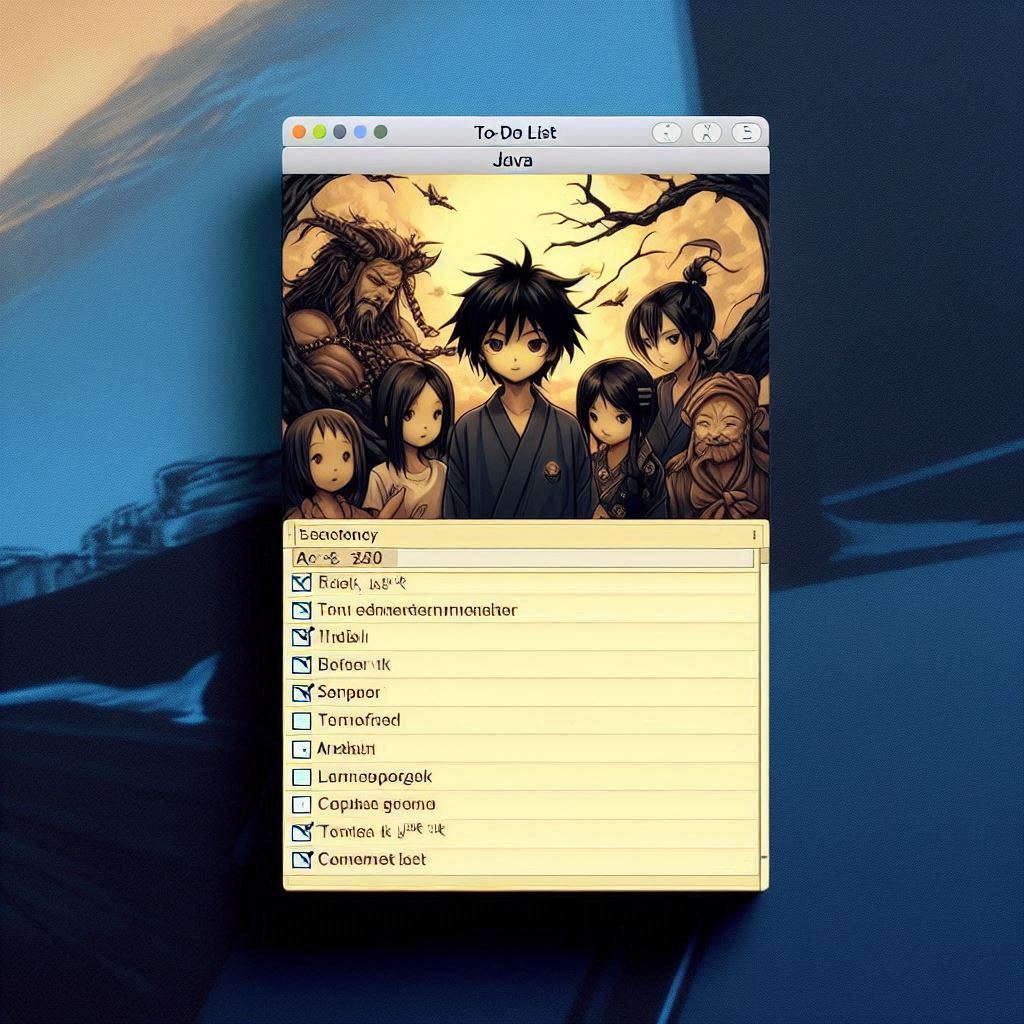
Overview
In this blog post, we'll walk through creating a straightforward to-do list application using Java.
The Simple To-Do List application allows users to add, view, update and delete tasks from a list.
This project will help you learn essential Java concepts and gain hands-on experience with fundamental operations.
Objectives
Learn to use
ArrayList
class to store dynamic data.Learn to handle simple CRUD (Create, Read, Update, Delete) operations using java.
Implements simple console based user interface.
Step-by-Step Guide
Step 1 : set up the project
create a new java project named simpleToDoList.
Step 2 : import necessary classes
import java.util.ArrayList;
import java.util.Scanner;
Step 3 : initialize to-do list
In a main method create an ArrayList
of type string to store the task.
ArrayList <String> toDoList = new ArrayList<>();
Scanner sc = new Scanner(System.in);
Step 4 : create a loop to display menu
Using while loop to display a menu and switch
case to map and process user input.
int choice = 0;
while (choice != 5) {
System.out.println("\n \n");
System.out.println("==================================");
System.out.println("|| Simple TO-Do list ||");
System.out.println("==================================");
System.out.println(" Select 1. Add Task ");
System.out.println(" Select 2. View Task ");
System.out.println(" Select 3. Update Task ");
System.out.println(" Select 4. Delete Task");
System.out.println(" Select 5. Exit ");
System.out.println("Choose an Option from above Menu: ");
choice = sc.nextInt();
sc.nextLine();
switch (choice) {
case 1:
// logic to Add task goes here
break;
case 2:
// logic to View task goes here
break;
case 3:
// logic to Update task goes here
break;
case 4:
// logic to Delete task goes here
break;
case 5:
System.out.println("========xxxxxxxxxxxxxx=======");
System.out.println("Exiting...");
System.out.println("========xxxxxxxxxxxxxx=======");
break;
default:
System.out.println("========xxxxxxxxxxxxxx=======");
System.out.println("Invalid Choice, Please Enter a valid Choice.");
System.out.println("========xxxxxxxxxxxxxx=======");
}
}
Step 5 : implementing Add operation (Insertion).
Inside a case 1 block, firstly we will display a message, then takes input from user and stored it to a local variable, and then use an inbuilt method of an ArrayList
class called add();
(which basically adds the item to a list) to add task in our to-do list.
System.out.println("========xxxxxxxxxxxxxx=======");
System.out.println(" Enter the Task ");
String task = sc.nextLine();
toDoList.add(task);
System.out.println("Task added Successfully!!");
System.out.println("========xxxxxxxxxxxxxx=======");
Step 6 : implementing View operation
Inside the case 2 block, we use a loop to keep track of task indexes and to display all the tasks added in our list.
System.out.println("========xxxxxxxxxxxxxx=======");
System.out.println(" Your Task: ");
for (int i = 0; i < toDoList.size(); i++) {
System.out.println((i + 1) + ". " + toDoList.get(i));
}
System.out.println("========xxxxxxxxxxxxxx=======");
Step 7 : implementing Update Operation (Updation).
Inside the case 3 block, Asks the user to enter the task ID they want to update, Captures the userβs input for the task ID, Calls sc.nextLine();
to consume the newline after reading the integer, Captures the new task description input from the user.
Updates the task in the list at the specified index.
System.out.println("========xxxxxxxxxxxxxx=======");
System.out.println("Enter the id to Update a task ");
int uId = sc.nextInt();
sc.nextLine();
System.out.println("Update a new task Description.");
String newTask = sc.nextLine();
toDoList.set(uId - 1, newTask);
System.out.println("Task Updated Successfully!!");
System.out.println("========xxxxxxxxxxxxxx=======");
Step 8 : implementing Delete operation (Deletion).
Inside case 4 block, Asks the user to enter the ID of the task they want to delete, Checks if the entered ID is valid (within the range of the list size), Removes the task if the ID is valid, otherwise, prints an error message.
Prints a success message if the task was deleted, or prompts for a valid ID if not.
System.out.println("========xxxxxxxxxxxxxx=======");
System.out.println("Enter the Task Id to Delete : ");
int taskNumber = sc.nextInt();
sc.nextLine();
if (taskNumber > 0 && taskNumber <= toDoList.size()) {
toDoList.remove(taskNumber - 1);
System.out.println("Task Deleted successfully!");
} else {
System.out.println("Enter Valid Task Id.");
}
System.out.println("========xxxxxxxxxxxxxx=======");
Step 8: Close the Scanner
After the loop close the scanner.
sc.close();
shuts down the scanner and releases any resources it was using. Always a good practice to avoid memory leaks when you're done with input operations!
sc.close();
Key Learnings
Here's the key learnings from simpleToDoList
Java program:
ArrayList Usage: Learn how to create and manipulate an
ArrayList
to store tasks.User Input: Use the
Scanner
class to capture user input.Control Flow: Implement control flow using a
while
loop to keep the program running until the user chooses to exit.Switch Statements: Use a
switch
statement to handle different user choices and perform corresponding actions.CRUD Operations: Perform basic CRUD (Create, Read, Update, Delete) operations on the task list.
Input Validation: Validate user input to ensure that the task ID is within a valid range.
Updating and Deleting Tasks: Update and delete tasks based on user input, demonstrating how to modify
ArrayList
elements.
These concepts are fundamental for managing data and user interactions in a Java application.
Possible Enhancements
You could consider a few improvements to your simple to-do list program:
Persistent Storage: Save tasks to a file so they persist between sessions. You can use file I/O to read/write tasks.
Task Completion Status: Add a way to mark tasks as completed and filter between completed and pending tasks.
User Interface: Develop a graphical user interface using JavaFX or Swing.
Error Handling: Improve error handling to provide more informative messages and prevent crashes.
These enhancements can make your to-do list application more robust and user-friendly.
By the end of this tutorial, you'll have a functional to-do list application and a solid understanding of core Java programming principles.
In the coming articles in the series we will deep dive into the development and implementation of more advance features.
do Follow Sahil Notes , If you find my articles useful, please leave a comment, and subscribe to us on hashnode for updates on our latest posts.
Happy coding!
Sahil Notes.
Subscribe to my newsletter
Read articles from Sahil Khan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sahil Khan
Sahil Khan
π¨βπ» Sahil- Software developer π¨βπ Hello there! I'am Sahil, a recent graduate software developer passionate about crafting efficient and scalable code.