7 Game-Changing WordPress Performance Hacks

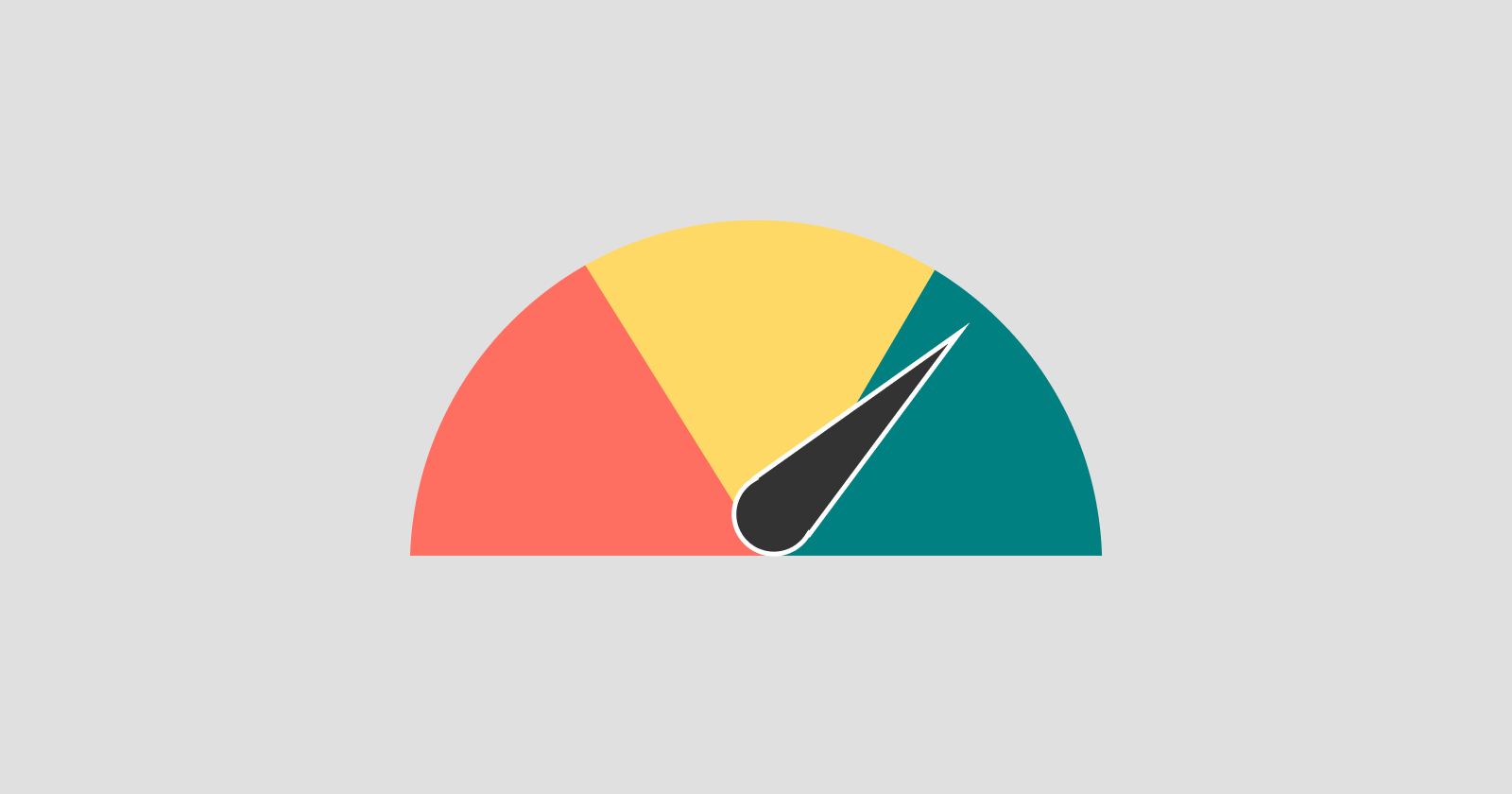
Performance in WordPress is like that elusive Holy Grail — always in demand, often hard to achieve, but oh so rewarding when you finally get it right. The good news? Optimizing your WordPress site doesn’t have to involve complex systems or in-depth reengineering. Sometimes, the smallest tweaks can make the biggest difference. Whether you're streamlining queries, optimizing database performance, or removing unnecessary features, these code snippets will help you improve your site's speed and responsiveness.
(I’ve gathered these trusty code snippets from some of my older blog posts. Some might be a bit old, but they might still come in handy.)
1. Array Chunking for Large Data Sets
Handling large datasets in WordPress can quickly become problematic if you try to process everything at once. A massive array of data can overwhelm your server, leading to slow page loads or even server crashes. By chunking arrays into smaller pieces, you can improve the way your server handles large datasets, optimizing performance and memory usage.
The following snippet demonstrates how to split a large array into smaller, more manageable chunks. This helps the server process data more efficiently, making your site faster and more responsive.
$array = [ 'Post 0', 'Post 1', 'Post 2', 'Post 3', 'Post 4', 'Post 5' ];
$chunked_array = array_chunk( $array, 2 );
print_r( $chunked_array );
// Output:
// Array
// (
// [0] => Array
// (
// [0] => Post 0
// [1] => Post 1
// )
// [1] => Array
// (
// [0] => Post 2
// [1] => Post 3
// )
// [2] => Array
// (
// [0] => Post 4
// [1] => Post 5
// )
// )
2. Disable Emoji Scripts
Emojis, while fun, aren’t essential for every website. Yet, WordPress loads emoji scripts by default, even on sites where they aren’t needed. These scripts add unnecessary weight to your pages, slightly slowing down your site’s load time. By disabling these emoji scripts, you can streamline your site and speed up page loads.
Here’s how you can remove those extra emoji scripts from your site’s header and styles, which will result in fewer HTTP requests and quicker page rendering.
function matt_watson_disable_emojis() {
remove_action( 'wp_head', 'print_emoji_detection_script', 7 );
remove_action( 'wp_print_styles', 'print_emoji_styles' );
}
add_action( 'init', 'matt_watson_disable_emojis' );
3. Query Optimization
Inefficient database queries can significantly slow down your WordPress site, especially when generated by plugins or themes. By analyzing these queries, you can refine them to make your database interactions faster and more efficient. This is particularly useful for large, content-heavy sites where unnecessary queries can impact performance.
The following snippet optimizes the SQL queries generated by WPML (WordPress Multilingual Plugin) by removing unnecessary post-type lookups, reducing query load, and improving page speed.
function matt_watson_remove_post_type_sql_search( $query ) : string {
global $wpdb;
$id_sql = "{$wpdb->posts}.ID = wpml_translations.element_id";
$post_type_sql = "AND wpml_translations.element_type = CONCAT('post_', {$wpdb->posts}.post_type)";
if ( ! str_contains( $query, $id_sql ) || ! str_contains( $query, $post_type_sql ) ) {
return $query;
}
$query = str_replace( $post_type_sql, '', $query );
return $query;
}
add_filter( 'query', 'matt_watson_remove_post_type_sql_search' );
4. XDebug Profiling for Slow Plugins
Plugins are a key feature of WordPress, but they can also be a source of performance bottlenecks. Some plugins load unnecessary resources or execute inefficient PHP processes, significantly slowing down your site’s response time. Using tools like XDebug allows you to profile your PHP processes, identifying which plugins or theme components are hogging resources.
Once you know which plugins are the main offenders, you can either disable them, find more efficient alternatives, or work on optimizing them directly. This process can greatly improve your Time to First Byte (TTFB) and overall site speed.
5. Leveraging Object Caching
WordPress allows you to cache objects, reducing the need for repeated database queries, but excessive autoloaded options can overwhelm your cache. When too many options are autoloaded, performance can degrade, especially if your hosting provider disables object caching altogether. By controlling which options are autoloaded, you can keep your cache lean and efficient, improving your site’s performance.
Here’s a snippet that prevents specific options from being autoloaded, reducing unnecessary load on the cache and improving database efficiency:
function matt_watson_set_autoload_to_no( $option ) {
global $wpdb;
$autoloaded_options = ['permalink-manager-uris', /* other options */];
if ( ! in_array( $option, $autoloaded_options ) ) {
return;
}
$wpdb->update(
$wpdb->prefix . "options",
['autoload' => 'no'],
['option_name' => $option]
);
}
add_action( 'updated_option', 'matt_watson_set_autoload_to_no' );
add_action( 'added_option', 'matt_watson_set_autoload_to_no' );
6. Advanced Conditional Plugin Loading for Elementor
Some plugins, like Elementor, can dramatically increase page load times if loaded on every page, even those that don’t require their functionality. By conditionally loading these plugins only when necessary, you can significantly reduce the load on your server and improve page speed for non-Elementor pages.
Here’s a detailed example of how to conditionally disable Elementor and its dependencies unless needed, such as for specific post types or Elementor edit modes:
function matt_watson_restrict_elementor_loading( $plugins ) : array {
if ( is_admin() || wp_doing_ajax() ) {
return $plugins;
}
if ( isset( $_GET['elementor-preview'] ) ) {
return $plugins;
}
$path = $_SERVER['REQUEST_URI'];
$path_base = basename( $path );
$rest_prefix = trailingslashit( rest_get_url_prefix() );
$is_rest_api_request = strpos( $path, $rest_prefix ) !== false;
if ( $is_rest_api_request ) {
return $plugins;
}
$post = get_page_by_path( $path_base, OBJECT, [ 'page', 'getting_started_hub' ] );
$is_elementor_page = is_object( $post ) && ! empty( get_post_meta( $post->ID, '_elementor_edit_mode', true ) );
if ( $is_elementor_page ) {
return $plugins;
}
$remove_plugins = [
'elementor/elementor.php',
'elementor-pro/elementor-pro.php',
'elemental-menu/elemental-menu.php',
'unlimited-elements-for-elementor-premium/unlimited-elements-pro.php',
];
foreach ( $remove_plugins as $remove_plugin ) {
$key = array_search( $remove_plugin , $plugins );
unset( $plugins[ $key ] );
}
return $plugins;
}
add_filter( 'option_active_plugins', 'matt_watson_restrict_elementor_loading' );
7. Remove WPML Distinct Strings in English
On a multilingual site powered by WPML, WordPress often loads distinct language strings, even when browsing the site in English. Since your default language is likely English, loading these strings is unnecessary and adds extra load to your database. By removing the query for distinct strings when viewing the English version, you can improve database performance and speed up page load times.
This code snippet stops WPML from loading distinct language strings on English pages, reducing the number of queries executed and optimizing site performance for English-speaking users:
function matt_watson_remove_wpml_distinct_strings_in_english( $query ) {
global $wpdb;
if ( is_admin() ) {
return $query;
}
$path = $_SERVER['REQUEST_URI'];
$path = explode( '/', $path );
$path = array_filter( $path );
$lang = reset( $path );
if ( isset( $lang ) && in_array( $lang, [ 'es', 'fr', 'it', 'de', 'br' ] ) ) {
return $query;
}
$id_sql = "FROM {$wpdb->prefix}icl_strings";
if ( false === strpos( $query, $id_sql ) && false === strpos( $query, 'DISTINCT' ) ) {
return $query;
}
return '';
}
add_filter( 'query', 'matt_watson_remove_wpml_distinct_strings_in_english' );
These seven tips may seem small individually, but combined, they can lead to significant performance improvements for your WordPress site. From chunking arrays and optimizing database queries to conditionally loading plugins and disabling unnecessary features, each tweak helps ensure your site runs as fast and efficiently as possible.
Subscribe to my newsletter
Read articles from Matt Watson directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Matt Watson
Matt Watson
Hello there! I’m Matt Watson — a developer, father, and husband, remotely coding from the charming landscapes of Yorkshire, UK. I’ve been crafting wonderful things with WordPress since 2006 and spinning webs (the good kind) since 1996 — yes, I survived the dial-up era! Solving (and occasionally committing) WordPress-based crimes, I juggle code by day and dad jokes by night. Remote worker since 2014, I’ve mastered the art of debugging in my slippers while keeping the coffee industry in business.