9 Must-Know PHP and WordPress Hacks That Will Instantly Boost Your Development Workflow

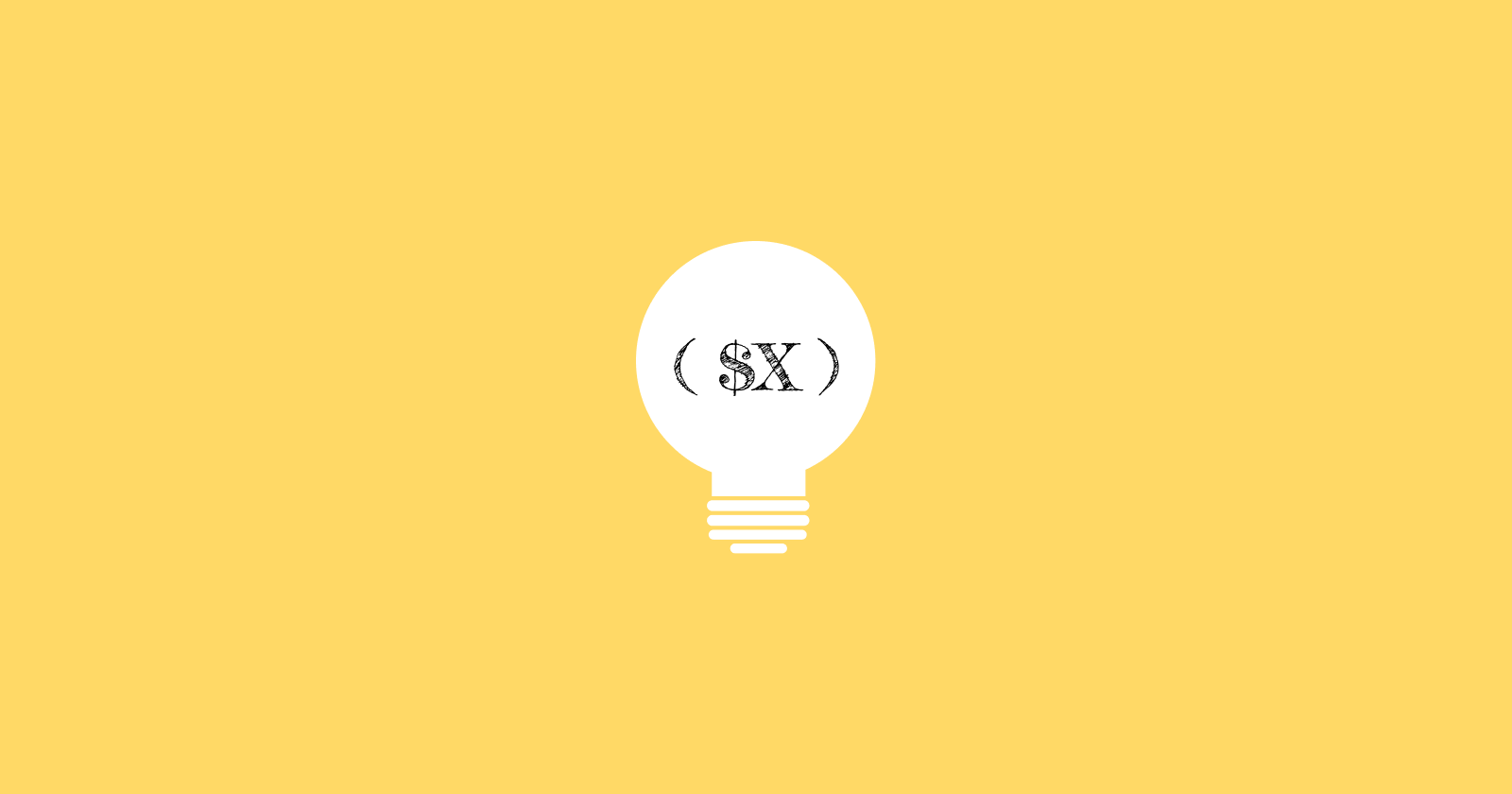
Efficiently handling data and managing PHP logic is key to smooth WordPress development. Sometimes, it’s the little tricks and techniques that can make all the difference in your workflow. This listicle focuses on tips to streamline your work with PHP arrays, dynamic variables, and content management in WordPress. These trusty snippets, though some might seem a bit old, still pack a punch when you need them.
(I’ve gathered these trusty code snippets from some of my older blog posts. Some might be a bit old, but they might still come in handy.)
General PHP and Data Management Tips
1. Array Change Key Case
When handling external data, especially data from APIs or third-party services, you’ll often come across array keys that aren’t in a format you’d prefer—sometimes they’re all uppercase or inconsistent with your internal structure. To solve this, you can use PHP's array_change_key_case()
function to quickly convert all array keys to either lowercase or uppercase. This makes your data easier to work with and ensures uniformity.
Here’s an example that converts an array’s keys to lowercase:
$array = [ 'P' => 42, 'POST_STATUS' => 'publish' ];
$lower_case_array = array_change_key_case( $array, CASE_LOWER );
print_r( $lower_case_array );
// Output:
// Array
// (
// [p] => 42
// [post_status] => publish
// )
2. Dynamic Variable Names in PHP
There are cases when you need to create variables dynamically within loops or repetitive structures, especially when dealing with user-generated data or flexible input formats. Using dynamic variable names allows you to generate new variables on the fly based on conditions or data, which can be handy in certain situations. However, use this technique cautiously, as it can make your code harder to debug.
Here’s an example that dynamically creates variables based on an array’s values:
$custom_variable_array = ['name_1', 'name_2', 'name_3'];
foreach ( $custom_variable_array as $custom_variable ) {
$$custom_variable = 'Some Value';
}
echo $name_1;
3. Remove Duplicates from Arrays
Working with arrays often involves cleaning up data and ensuring there are no duplicate values. PHP’s array_unique()
function provides a simple way to eliminate duplicate entries from an array, leaving you with clean, unique data. This is particularly useful when you’re aggregating data from multiple sources or handling form input where duplicates might occur.
Here's how you can remove duplicate values from an array:
$array = [ 'apple', 'banana', 'apple', 'orange', 'banana' ];
$unique_array = array_unique( $array );
print_r( $unique_array );
// Output:
// Array
// (
// [0] => apple
// [1] => banana
// [3] => orange
// )
4. Merge Arrays Recursively
Merging arrays in PHP can be tricky when you have nested arrays or need to combine multidimensional arrays. The array_merge_recursive()
function comes in handy by merging arrays while preserving their nested structure. This is particularly useful when combining configurations or settings from different sources without losing any nested values.
Here’s an example that merges two multidimensional arrays:
$array1 = [
'settings' => [
'color' => 'blue',
'size' => 'medium',
]
];
$array2 = [
'settings' => [
'size' => 'large',
'shape' => 'round',
]
];
$merged_array = array_merge_recursive( $array1, $array2 );
print_r( $merged_array );
// Output:
// Array
// (
// [settings] => Array
// (
// [color] => blue
// [size] => Array
// (
// [0] => medium
// [1] => large
// )
// [shape] => round
// )
// )
Text and Content Management Tips
5. Filter Text Strings in WordPress Using gettext
Customizing text strings in themes or plugins can sometimes involve modifying the original code, which is neither practical nor update-friendly. Instead, WordPress provides the gettext
filter, allowing you to dynamically change text strings without touching the source files. This is particularly useful for making quick adjustments to text used by third-party themes or plugins.
Here’s how to modify a string using the gettext
filter:
function matt_watson_filter_text( $translated_text, $untranslated_text, $domain ) {
if ( 'String to translate' === $untranslated_text && 'mattwatson' === $domain ) {
return esc_html__( 'Translated Text', 'mattwatson' );
}
return $translated_text;
}
add_filter( 'gettext', 'matt_watson_filter_text', 10, 3 );
6. Sanitize Titles with sanitize_title()
When generating URLs or slugs from user inputs, it’s important to sanitize the title to ensure it's URL-friendly. WordPress offers the sanitize_title()
function, which removes unwanted characters and spaces, turning your input into a clean slug. This is useful for creating SEO-friendly URLs or unique identifiers for content.
Here’s an example of how to sanitize a title in WordPress:
$title = "This is My Awesome Post!";
$slug = sanitize_title( $title );
echo $slug; // Output: this-is-my-awesome-post
7. Programmatically Create WordPress Pages
If you’re setting up a WordPress site and need to automatically generate pages upon theme activation or plugin installation, you can programmatically create new pages using wp_insert_post()
. This allows you to set up default content or landing pages, saving time and ensuring consistency across installations.
Here’s an example of how to create a page programmatically:
function matt_watson_create_default_page() {
$page_data = [
'post_title' => 'Contact Us',
'post_content' => 'This is the contact page.',
'post_status' => 'publish',
'post_type' => 'page',
];
wp_insert_post( $page_data );
}
add_action( 'after_setup_theme', 'matt_watson_create_default_page' );
8. Automatically Shorten Excerpts in WordPress
By default, WordPress excerpts can be too long or inconsistent for certain themes. You can programmatically set the length of excerpts to ensure they’re a consistent size across your site. This is especially useful for improving the look and feel of archive pages, blog listings, or search results.
Here’s a simple function to control excerpt length:
function matt_watson_set_excerpt_length( $length ) {
return 20; // Limit excerpt to 20 words
}
add_filter( 'excerpt_length', 'matt_watson_set_excerpt_length', 999 );
9. Replace WordPress Shortcodes Programmatically
If you need to replace shortcodes dynamically in your content, WordPress provides a filter called do_shortcode_tag
that allows you to replace shortcodes with custom logic. This is especially useful if you want to swap out certain shortcodes or modify their output without changing the underlying content structure.
Here’s how you can replace a shortcode with a custom output:
function matt_watson_replace_shortcode_output( $output, $tag, $attr ) {
if ( 'old_shortcode' === $tag ) {
return 'This is the new output!';
}
return $output;
}
add_filter( 'do_shortcode_tag', 'matt_watson_replace_shortcode_output', 10, 3 );
These nine tips and tricks will help streamline your WordPress development process, whether you're working with PHP arrays or fine-tuning your WordPress content. By mastering these techniques, you’ll be able to work faster, more efficiently, and create better-performing websites with less effort.
Subscribe to my newsletter
Read articles from Matt Watson directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Matt Watson
Matt Watson
Hello there! I’m Matt Watson — a developer, father, and husband, remotely coding from the charming landscapes of Yorkshire, UK. I’ve been crafting wonderful things with WordPress since 2006 and spinning webs (the good kind) since 1996 — yes, I survived the dial-up era! Solving (and occasionally committing) WordPress-based crimes, I juggle code by day and dad jokes by night. Remote worker since 2014, I’ve mastered the art of debugging in my slippers while keeping the coffee industry in business.