Introduction to CSS Preprocessors
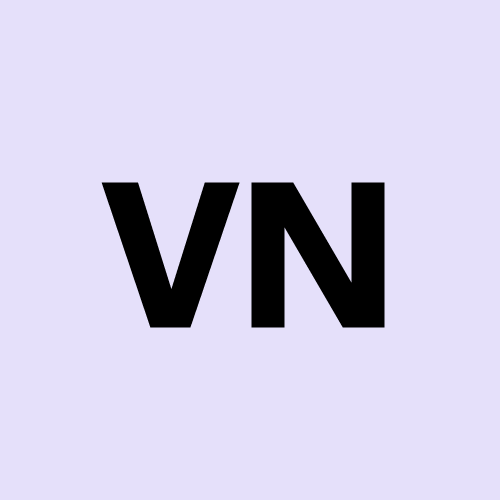
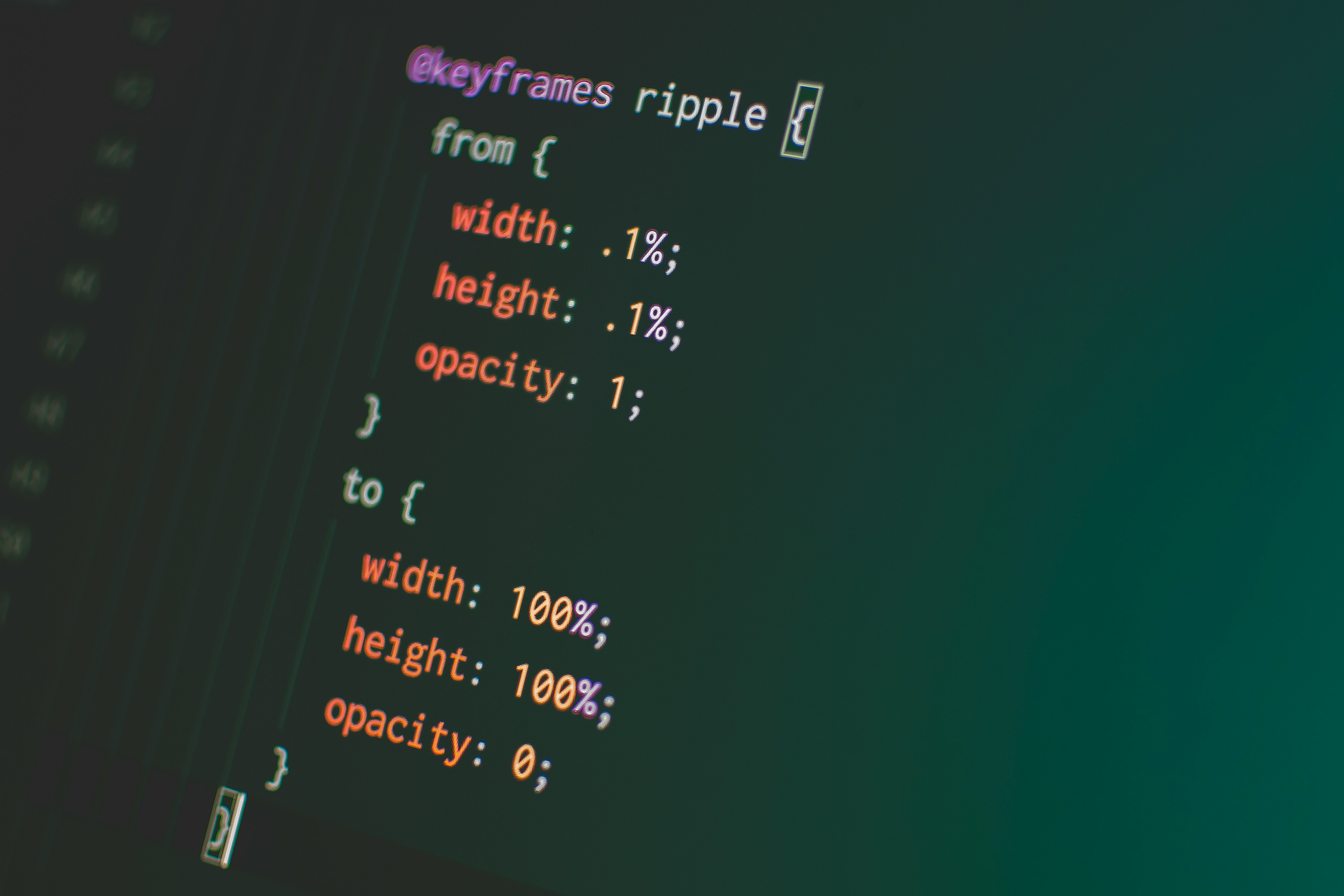
Sass and LESS
CSS preprocessors, such as Sass and LESS, offer an enhanced way of writing CSS that simplifies the process of building complex, scalable stylesheets. They introduce features like variables, nesting, mixins, and functions that make your code more maintainable and modular. In this article, we'll explore the benefits of using preprocessors, focusing on Sass and LESS, and provide examples to demonstrate their power.
What Are CSS Preprocessors?
CSS preprocessors are scripting languages that extend CSS by allowing you to write code with advanced features. The preprocessor then compiles this code into standard CSS that browsers can interpret. Two of the most popular preprocessors are Sass (Syntactically Awesome Stylesheets) and LESS (Leaner Style Sheets).
Key Benefits:
Variables: Define reusable values (colors, fonts, sizes, etc.) that can be applied throughout your stylesheet.
Nesting: Structure your CSS in a hierarchical way, mirroring your HTML structure.
Mixins: Reusable blocks of styles that can be included anywhere in your stylesheet.
Inheritance/Extends: Share styles between different selectors without duplication.
Functions/Operations: Perform calculations or generate values dynamically within your stylesheets.
Sass
Sass is one of the most widely used CSS preprocessors, offering a wealth of features that make CSS more powerful and efficient.
Example 1: Variables
scssCopy code// Defining variables in Sass
$primary-color: #3498db;
$font-stack: Helvetica, sans-serif;
body {
font-family: $font-stack;
background-color: $primary-color;
}
Output in CSS:
cssCopy codebody {
font-family: Helvetica, sans-serif;
background-color: #3498db;
}
Explanation: In this example, we define variables for the primary color and font stack, which can be reused throughout the stylesheet. This makes it easier to maintain consistency and update values in one place.
Example 2: Nesting
scssCopy codenav {
ul {
margin: 0;
padding: 0;
list-style: none;
}
li {
display: inline-block;
}
a {
text-decoration: none;
color: $primary-color;
}
}
Output in CSS:
cssCopy codenav ul {
margin: 0;
padding: 0;
list-style: none;
}
nav li {
display: inline-block;
}
nav a {
text-decoration: none;
color: #3498db;
}
Explanation: Nesting in Sass allows you to structure your CSS in a way that mirrors your HTML. This makes it easier to see how the styles relate to the structure of the document.
Example 3: Mixins
scssCopy code// Defining a mixin
@mixin border-radius($radius) {
-webkit-border-radius: $radius;
-moz-border-radius: $radius;
border-radius: $radius;
}
button {
@include border-radius(5px);
}
Output in CSS:
cssCopy codebutton {
-webkit-border-radius: 5px;
-moz-border-radius: 5px;
border-radius: 5px;
}
Explanation: The mixin border-radius
allows you to reuse the code for adding a border radius across different elements, avoiding redundancy while keeping the code DRY (Don’t Repeat Yourself).
LESS
LESS operates similarly to Sass, but with a slightly different syntax. Let's look at some common examples in LESS.
Example 1: Variables
lessCopy code@primary-color: #3498db;
@font-stack: Helvetica, sans-serif;
body {
font-family: @font-stack;
background-color: @primary-color;
}
Explanation: LESS uses the @
symbol to define variables, but the concept is the same: reusable values that make your styles more consistent and easier to update.
Example 2: Nesting
lessCopy codenav {
ul {
margin: 0;
padding: 0;
list-style: none;
}
li {
display: inline-block;
}
a {
text-decoration: none;
color: @primary-color;
}
}
Explanation: Just like in Sass, LESS allows you to nest your CSS selectors to better reflect your HTML structure, reducing repetitive code and making your stylesheet more organized.
Example 3: Mixins
lessCopy code.border-radius(@radius) {
-webkit-border-radius: @radius;
-moz-border-radius: @radius;
border-radius: @radius;
}
button {
.border-radius(5px);
}
Explanation: LESS mixins work similarly to those in Sass. Here, you define reusable chunks of code with parameters and include them wherever needed.
Sass vs. LESS
While both Sass and LESS provide powerful features, they have some key differences:
Syntax: Sass offers two syntaxes: the indented syntax (Sass) and SCSS (Sassy CSS). LESS has a single, CSS-like syntax.
Functions: Sass offers more built-in functions and better support for advanced features like control directives (loops and conditionals).
Compilation: Sass requires Ruby or Node.js for compilation, while LESS is written in JavaScript and can be compiled directly in the browser.
Summary
CSS preprocessors like Sass and LESS enable developers to write cleaner, more efficient, and scalable CSS. Features like variables, mixins, and nesting save time and make maintaining large stylesheets easier. Both preprocessors are powerful tools, with Sass generally offering more advanced features, but LESS is also a strong option due to its simplicity.
Incorporating these preprocessors into your workflow can dramatically improve the maintainability of your CSS, particularly for larger projects. Give them a try and see how they can enhance your styling process!
Subscribe to my newsletter
Read articles from Victor Nite directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
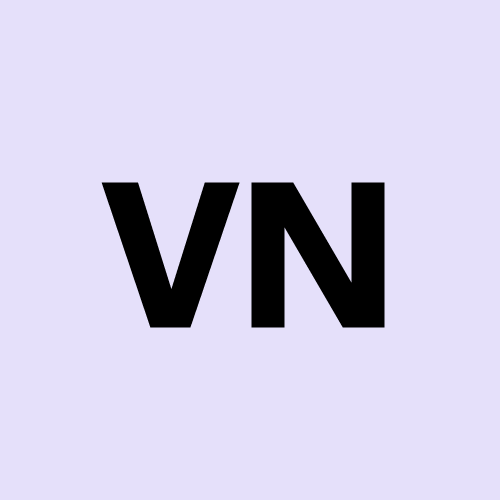