Doubly link list
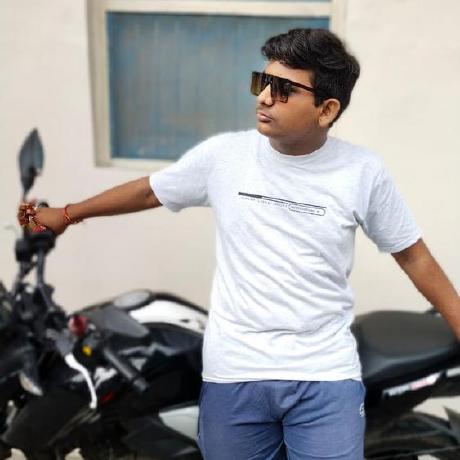
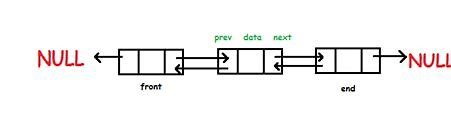
Like doubly link list it also contains address of next node and data but the change is that it also contains the address of previous node.
So here we have 3 block of
Previous node address
Data
Next node address
We will make it using 5 function:
Declaring a node
Creating a node
Inserting a data
Printing the data
Main function
Declaring a node
struct Node {
int data;//new data
struct Node* next;//address of the next node
struct Node* prev;//address of the previous node
};
Creating a node
struct Node* createNode(int data) {
struct Node* new_node = (struct Node*)malloc(sizeof(struct Node));
new_node->data = data;//assigning the data of link list
new_node->next = NULL;//addressing the node to next node.
new_node->prev = NULL;//addressing the node to previous node.
return new_node;
}
Inserting the data in node
void insert(struct Node** head, int new_data) {
struct Node* new_node = createNode(new_data); //creating a newNode
struct Node* last = *head; //Assingning the value to the last node
if (*head == NULL) { //checking if the linked list is empty
*head = new_node; //if empty assign the value
return;
}
while (last->next != NULL) { //traversing the link list
last = last->next;//forwarding the data of link list
}
last->next = new_node;
new_node->prev = last;
}
Here we are adding the address of last node for the link list.
Printing the linked list
// Function to print the doubly linked list
void printList(struct Node* node) {
while (node != NULL) {
printf("%d <-> ", node->data);
node = node->next;
}
printf("NULL\n");
}
This function is made to increase the readability of code and make it easier to understand for user.
Main function
int main() {
struct Node* head = NULL;
int n, value;
printf("Enter the number of nodes: ");
scanf("%d", &n);
for (int i = 0; i < n; i++) {
printf("Enter value for node %d: ", i + 1);
scanf("%d", &value);
insertEnd(&head, value);
}
printf("Original doubly linked list: ");
printList(head);
return 0;
}
In this code we are calling the function and getting the values from users that we have to use.
Complete code
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
struct Node* prev;
};
struct Node* createNode(int data) {
struct Node* new_node = (struct Node*)malloc(sizeof(struct Node));
new_node->data = data;
new_node->next = NULL;
new_node->prev = NULL;
return new_node;
}
void insertEnd(struct Node** head, int new_data) {
struct Node* new_node = createNode(new_data);
struct Node* last = *head;
if (*head == NULL) {
*head = new_node;
return;
}
while (last->next != NULL) {
last = last->next;
}
last->next = new_node;
new_node->prev = last;
}
void printList(struct Node* node) {
while (node != NULL) {
printf("%d <-> ", node->data);
node = node->next;
}
printf("NULL\n");
}
int main() {
struct Node* head = NULL;
int n, value;
printf("Enter the number of nodes: ");
scanf("%d", &n);
for (int i = 0; i < n; i++) {
printf("Enter value for node %d: ", i + 1);
scanf("%d", &value);
insertEnd(&head, value);
}
printf("Original doubly linked list: ");
printList(head);
return 0;
}
There are many more ways we can use doubly link list.
Questions for doubly link list are:
Traversal Doubly Link List
Reversal of Doubly Link List
Merge to link list
Deleting the value of doubly link list etc.
Subscribe to my newsletter
Read articles from Jalaj Singhal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
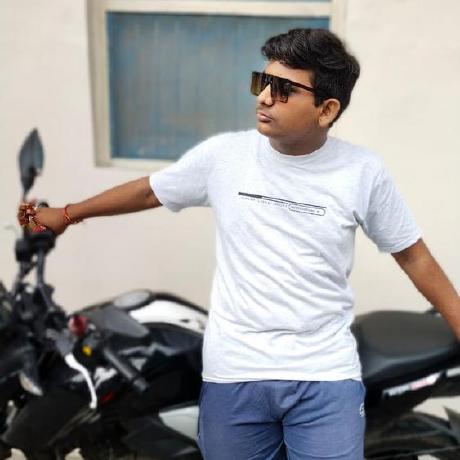
Jalaj Singhal
Jalaj Singhal
π Greetings, Jalaj Singhal here! π I'm an enthusiastic blogger who enjoys delving into the world of technology and imparting my knowledge to the community. π Having experience in HTML and CSS, I enjoy creating interesting and educational content that demystifies difficult ideas and gives readers the tools they need to advance their knowledge. π I try to contribute to the active tech community and encourage relevant discussions on Hash Node, where you can find my writings on the subject of web development. π‘ Together, let's connect and go out on this fascinating path of invention and learning!