Is JavaScript Synchronous or Asynchronous? 🤔 Let's Find Out! 🚀

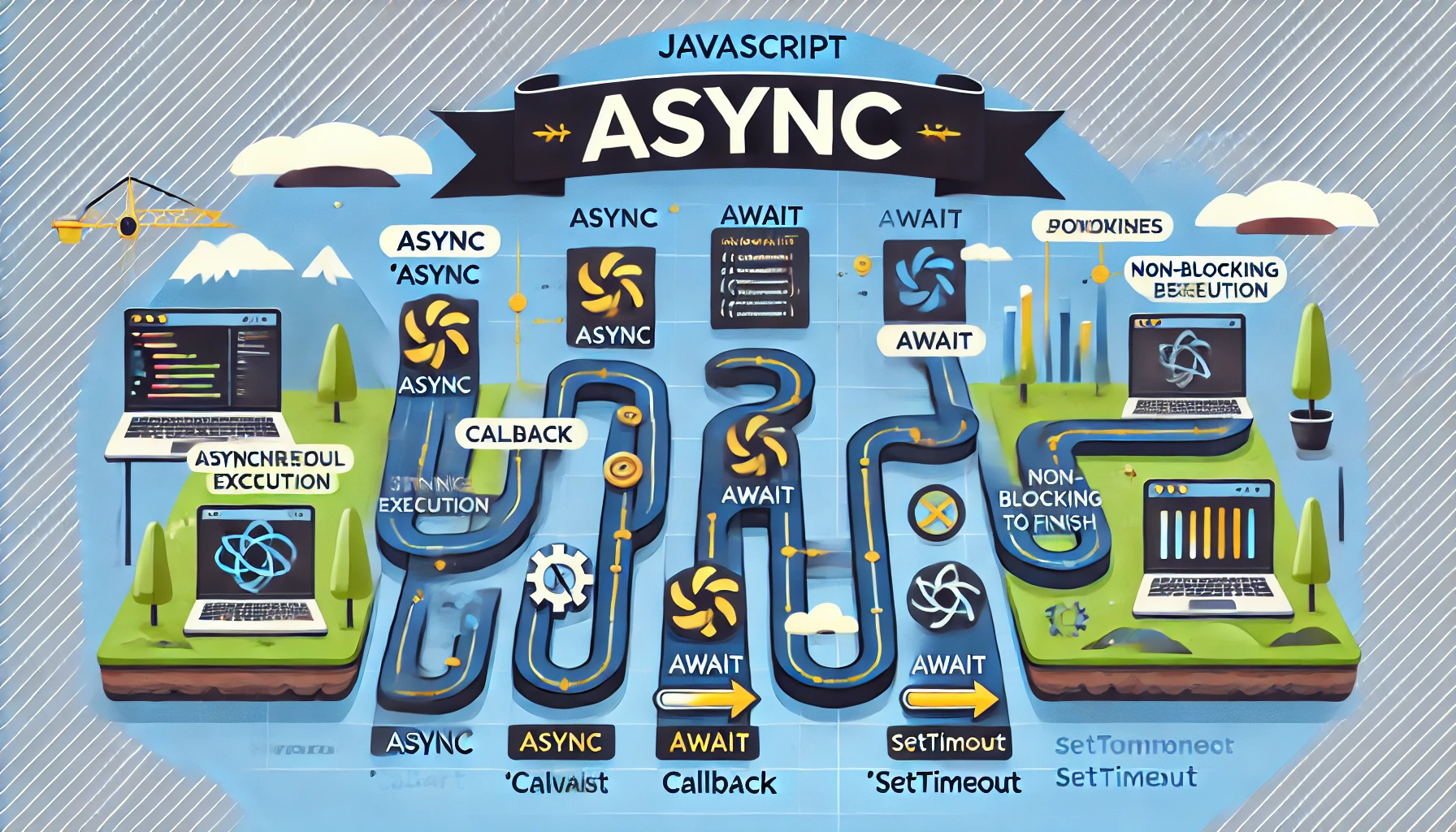
Hey there, curious coders! 👋 Today, we’re diving into one of the most asked questions in the JavaScript world:
Is JavaScript synchronous or asynchronous ?
Spoiler alert: It’s both! 🎉 Sounds confusing? Don’t worry! By the end of this post, you’ll totally get it, and I promise, no headaches! 🤯 So, let’s break it down—simple, practical, and fun (yes, I said fun 😎).
First Things First: What's Synchronous? 🤔
Imagine you’re in line at your favorite taco truck 🌮. You place your order, and you can’t do anything else until you get your tacos, right? The truck won’t let the next person order until your tacos are served. ⏳
That’s synchronous. One task finishes before the next starts. JavaScript is synchronous by default. When you write code, it goes line by line, executing one thing at a time—just like our taco truck. 🚚
Example of Synchronous Code
console.log('Order placed!');
console.log('Cooking your taco...');
console.log('Taco ready, enjoy! 🌮');
Output:
Order placed!
Cooking your taco...
Taco ready, enjoy! 🌮
No surprises here. Each step waits for the one before it to finish. Simple, right?
But Wait... What’s Asynchronous? 🕒
Now, let’s shake things up! What if our taco truck is super efficient? 🏃♂️ You place your order, but instead of making you wait, they give you a buzzer and say, “We’ll buzz you when your taco’s ready, do whatever you want until then!” 🤩
That's asynchronous. Tasks can start and then wait for something else (like a taco being ready) while other things happen in the background. JavaScript uses this a lot because waiting around isn’t cool, especially for things like network requests. 🕸️
Example of Asynchronous Code
console.log('Order placed!');
setTimeout(() => {
console.log('Taco ready, enjoy! 🌮');
}, 3000); // Taco takes 3 seconds
console.log('Meanwhile, you grab a drink! 🍹');
Output:
Order placed!
Meanwhile, you grab a drink! 🍹
Taco ready, enjoy! 🌮
See what happened here? While your taco was "cooking" in the background (thanks, setTimeout()
), you grabbed a drink without waiting around. 🍸 That's asynchronous magic! ✨
Why Does This Matter? 🤷♂️
Understanding synchronous vs. asynchronous is key because it helps you write more efficient code. JavaScript is single-threaded 🧵 (it can only do one thing at a time), but with the magic of asynchronous tasks like setTimeout()
, fetch()
, and Promises, you can make it look like it’s doing multiple things at once. 🧙♂️✨
Without asynchronous behavior, your web app could freeze while waiting for things like API responses or slow database queries. Imagine waiting 10 seconds just to load a page—yikes! 😱
console.log('Fetching taco ingredients... 🥑');
fetch('https://tacoapi.com/ingredients')
.then(response => response.json())
.then(data => {
console.log('Ingredients fetched! Let’s cook! 🌮');
});
console.log('Meanwhile, we prepare the tortillas! 🌯');
In this example, while waiting for taco ingredients to arrive from the API (which could take some time), the script keeps running and moves on to preparing the tortillas. No time wasted! ⏲️
How Does JavaScript Handle This? The Event Loop 🌀
JavaScript uses an event loop to manage asynchronous operations. Imagine the event loop as a super-organized chef 🧑🍳 who keeps track of all the food orders, so you don’t have to stand around waiting for each dish. The chef will buzz you when your taco is ready. Until then, they keep working on other tasks.
Quick Nerdy Diagram (Just for You 🤓)
| Call Stack | <- Synchronous code (single line kitchen order)
|------------|
| Event | <- Asynchronous code (kitchen prepares the taco and buzzes you later)
| Queue |
So, when an async task finishes (like an API call or a timeout), it goes to the event queue, and the event loop checks if it can safely add it back to the stack without messing up the flow. 💪 Pretty neat, huh?
Wrapping It All Up 🎁
To sum it up:
Synchronous: One task at a time (like waiting in line for tacos 🌮).
Asynchronous: Tasks happening in the background (like getting a buzzer while your taco is being prepared 🚀).
JavaScript is mostly synchronous, but thanks to cool features like setTimeout()
, Promises, and async functions, it can handle asynchronous tasks too!
So, next time you’re working on some JavaScript magic 🪄, remember: you can grab a drink while your taco is being made. 🍹
Happy coding, and may all your tacos be delicious! 🌮✨
What do you think? 😎 Let me know in the comments below!
Subscribe to my newsletter
Read articles from Harsh Goswami directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Harsh Goswami
Harsh Goswami
Harsh | Frontend Developer 👨💻 I’m a passionate frontend developer with 2 years of experience at Softcolon Pvt Ltd. I specialize in creating responsive, user-centric web applications using modern frameworks like React.js, Next.js, and Remix. Currently, I’m focused on enhancing my skills in web development and exploring new technologies. When I'm not coding, you’ll find me collaborating with developers worldwide, participating in hackathons, or working on my portfolio website. I’m also considering starting a blog to share insights and connect with the frontend community. Let’s build amazing things together!