JAVA: Constructors

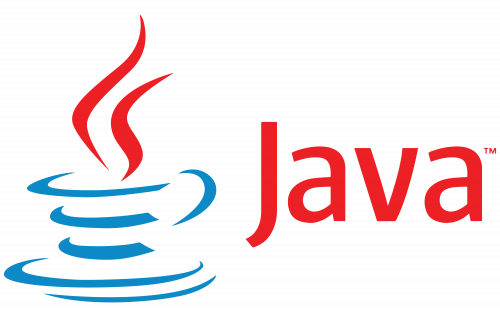
Prologue
Think of it as assembling furniture. You follow instructions to put all the parts together so it works. Similarly, when you create an object in Java, a constructor helps set it up correctly from the start. Without it, the object would be incomplete, just like furniture with missing screws. Constructors ensure everything is ready to use right away.
What are Constructors?
A constructor is a special method which is used to instantiate the object and allocate necessary resources to an object. It gets executed automatically each time the code for object creation gets executed
Key Points:
A constructors is invoked automatically
A constructor has same name as that of the class name
A constructor must not return a value, not even void
If there is no constructor in the class definition, then a default constructor is added in the compiled code file at the time of compilation. This default constructor initializes the instance variables (data members) to their default values, which are defined by their data types
Types of Constructors
There are mainly 3 different types of Constructors, they exist to offer flexibility and convenience when creating objects.
Default Constructors
This is the simplest type of constructor. The default constructor is provided by the compiler and initializes data members with default values. However, if we define a constructor, we can set specific values and include code to run while the object is being created.
A class can have only one default constructor, and it runs when no arguments are given during object creation.
class ClassName {
// Default constructor (No arguments)
ClassName() {
// Initialization code
}
}
Parameterized Constructors
A parameterized constructor is a constructor that accepts parameter(s) which is passed as argument(s) to initialize an object with specific values. This allows more flexibility, as you can pass different data to each object when it is created. A parameterized constructors can be overloaded (means, there can be more than one parameterized constructor in a given class definition).
class ClassName {
// Parameterized constructor with parameters
ClassName(Parameter List) {
// Constructor body: Initialize instance variables using the parameters
}
}
// Call to parameterized constructor
new ClassName(Argument List) // Argument List of Primitive Type
Copy Constructors
A copy constructor is a type of parameterized constructor where the parameter receives a reference to an object. It is invoked when an object is passed as an argument at the time of creating a new object. A copy constructor is used to initialize the fields of the new object with the values from an existing object, ensuring that the two objects are independent.
class ClassName {
// Copy constructor that takes an object of the same class as a parameter
ClassName(ClassName obj) {
// Initialize fields with the values from the passed object
}
}
ReferenceVariable2 = new ClassName(ReferenceVariable1);
// Call to Copy Constructor
Garbage Collection
Garbage collection (GC) in Java is an automatic memory management process. It frees up memory by removing objects that are no longer reachable or needed by the program. Java’s garbage collector prevents memory leaks by reclaiming the memory of unused objects, which helps applications run efficiently without manual intervention.
System.gc()
makes an explicit request to perform garbage collection, but it does not guarantee that garbage collection will run immediately or even at all. The JVM decides when and if to run the garbage collector based on internal memory management logic.
Finalization
Finalization refers to a mechanism where an object's finalize()
method is called just before the object is collected by the garbage collector. This gives objects a chance to perform any cleanup tasks, before they are removed from the memory. This method is called by garbage collector.
finalize()
for cleanup operations. Instead, Java encourages the use of the try-with-resources statement or explicit cleanup methods.Program
Program (Constructors)
class Student {
String name; // Instance variable for name
int age; // Instance variable for age
// Default Constructor
Student() {
System.out.println("Using Default Constructor");
name = "Unknown"; // Assign default values
age = 1;
}
// Parameterized Constructor
Student(String studentName, int studentAge) {
System.out.println(" ");
System.out.println("Using Parameterized Constructor");
name = studentName; // Assign parameter values to instance variables
age = studentAge;
}
// Copy Constructor
Student(Student otherStudent) {
System.out.println(" ");
System.out.println("Using Copy Constructor");
name = otherStudent.name; // Copy values from the other object
age = otherStudent.age;
}
// Method to display Student details
void display() {
System.out.println("Name: " + name + ", Age: " + age);
}
public static void main(String[] args) {
// Using Default Constructor
Student student1 = new Student();
student1.display();
// Using Parameterized Constructor
Student student2 = new Student("Amni", 20);
student2.display();
// Using Copy Constructor
Student student3 = new Student(student2);
student3.display();
}
}
OUTPUT:
What’s next?
So, today we learned about Conditional Statements, and there is much more to come. In the next part, we’ll learn about Inheritance, how they work, and what their importance is.
So, Stay tuned!!
PS: I am also learning Java while teaching it. I believe in continuous growth and am committed to learning as much as I am teaching. If you have any questions, suggestions, or just want to chat about Java, please leave a comment! I'm also new to blogging, so any feedback is appreciated as I strive to improve. Thank you for joining me on this journey! Let's learn and grow together.
Subscribe to my newsletter
Read articles from Sarthak Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sarthak Sharma
Sarthak Sharma
Hi, I’m Sarthak, a passionate tech enthusiast, a student and blogger. I’m on a mission to share the things that i learn or find interesting.