Better Living with Patterns – Head First Approach
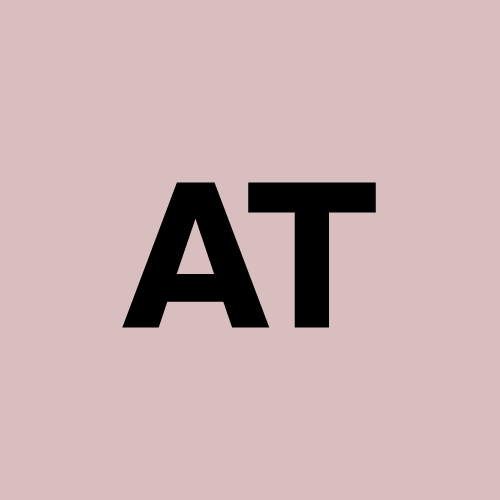
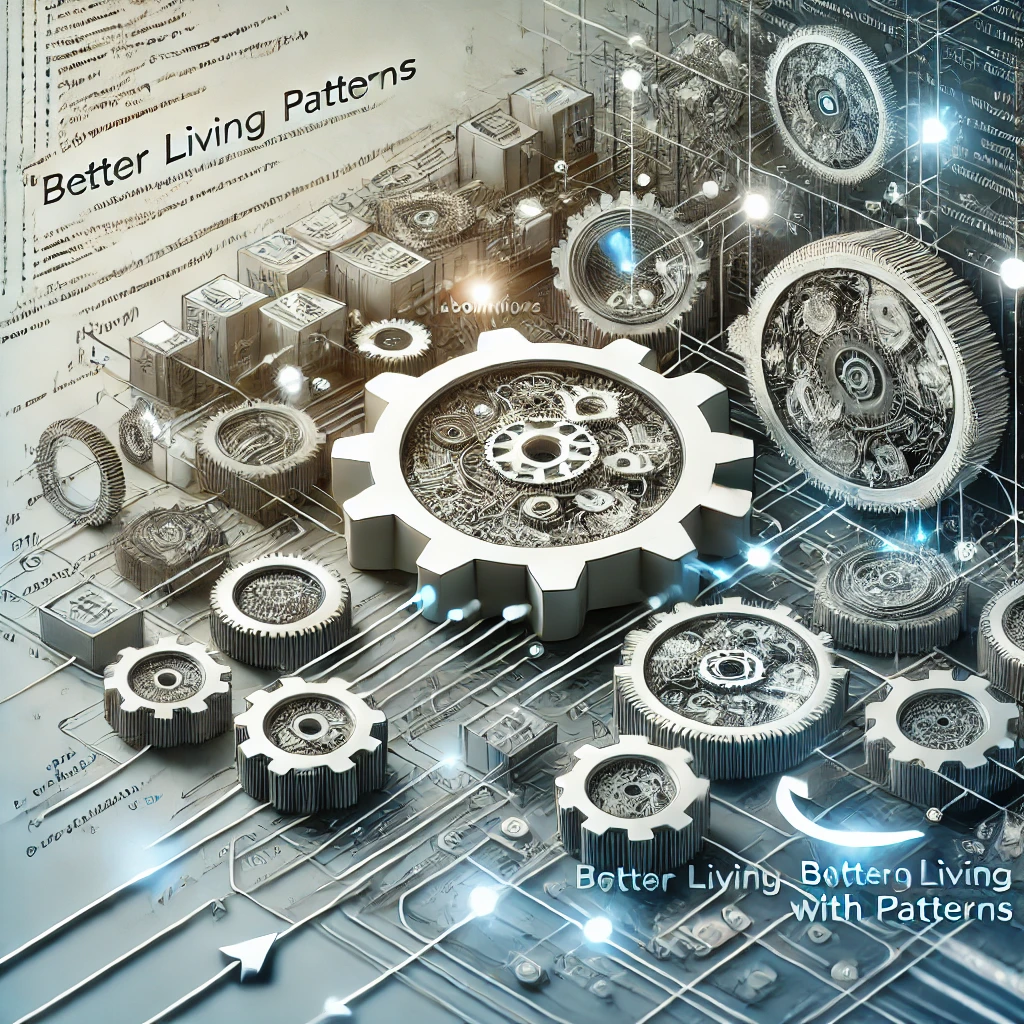
The final chapter of Head First Design Patterns offers a reflective look on how design patterns can improve your life as a developer by guiding you toward better, more maintainable code. It emphasizes the idea that patterns, when used wisely, can simplify complex software systems and make your code more adaptable to change.
What are Design Patterns?
Definition: Design patterns are proven solutions to common problems encountered in software development. They are not specific code implementations but rather templates that you can follow to solve recurring design issues.
Patterns help developers communicate ideas, reduce complexity, and build systems that are easier to maintain and extend.
Why "Better Living" with Patterns?
Patterns are tools that go beyond just writing code. They help you think in higher-level abstractions, letting you focus on solving the core problems without getting bogged down by implementation details. When applied correctly, patterns can lead to:
Cleaner, more readable code: By following known solutions, your code becomes more organized and easier to understand.
Reusability: Patterns are designed to solve problems that occur frequently, allowing you to reuse their structures across multiple projects.
Extensibility: Patterns provide flexible architectures that are easier to extend when requirements change.
Reduced complexity: By breaking problems into smaller parts, patterns can help you tackle large software challenges without being overwhelmed.
Problem Scenario
Consider a situation where you are building an application that needs to scale over time, with potentially dozens of features added as the product evolves. Managing the complexity of such a system without careful planning can lead to fragile, tangled code that’s hard to extend. To ensure long-term maintainability and scalability, you turn to design patterns to guide your architecture.
How Patterns Can Help
Throughout Head First Design Patterns, we’ve learned many design patterns that can be applied to improve the structure of your code. Here’s a recap of the key patterns and their benefits:
Strategy Pattern: Encapsulates algorithms, making them interchangeable.
Observer Pattern: Defines a one-to-many relationship between objects, useful for building event-driven systems.
Decorator Pattern: Adds behavior to objects dynamically without modifying their structure.
Factory Pattern: Creates objects without specifying the exact class of object that will be created.
Singleton Pattern: Ensures a class has only one instance and provides a global point of access.
Command Pattern: Encapsulates a request as an object, allowing for the parameterization of clients with different requests.
Adapter Pattern: Converts one interface into another that a client expects.
Facade Pattern: Provides a simplified interface to a complex subsystem.
Template Method Pattern: Defines the skeleton of an algorithm, allowing subclasses to override certain steps without changing its structure.
Iterator Pattern: Provides a way to access elements of a collection sequentially without exposing the underlying representation.
State Pattern: Allows an object to alter its behavior when its internal state changes.
Proxy Pattern: Provides a surrogate or placeholder for another object to control access to it.
Compound Patterns: Combines multiple patterns to solve complex problems.
These patterns help address issues like code duplication, tight coupling, and inflexibility, which are common pain points in large systems.
Patterns in the Real World
Design patterns have been used in countless real-world applications. For instance, e-commerce websites rely heavily on patterns like Observer for handling notifications, Strategy for implementing payment methods, and Factory for managing product categories.
Even small projects benefit from applying patterns. A simple mobile app may use Decorator to handle different themes, Command for user interactions, and Singleton for managing shared resources like databases.
When to Use Patterns
While patterns are powerful, they should not be applied everywhere. Knowing when to use a pattern is key to success. You should use a design pattern when:
You are facing a problem that matches the problem a pattern is designed to solve.
You need to enhance code flexibility, maintainability, or scalability.
You want to communicate design decisions with other developers more effectively.
However, avoid using patterns just for the sake of it, as they can add unnecessary complexity when not needed.
Bullet Points
Patterns guide maintainable design: By following the structures of well-established patterns, you can write more maintainable and scalable code.
Reusability and flexibility: Patterns provide templates for solving problems that recur in software development.
Think in abstractions: Patterns allow you to think at a higher level, focusing on solving the core problem rather than implementation details.
Use patterns wisely: Patterns are a tool, not a silver bullet. Apply them thoughtfully, considering the specific challenges of your project.
Conclusion
Design patterns aren’t just about improving your code today—they’re about ensuring your code can grow and adapt in the future. Whether you’re building small projects or enterprise-level applications, patterns offer a way to make your code cleaner, more maintainable, and more flexible.
By mastering design patterns, you’re not just writing code; you’re crafting systems that can evolve gracefully as your project grows. As a developer, this is what “better living with patterns” truly means.
Subscribe to my newsletter
Read articles from Alyaa Talaat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
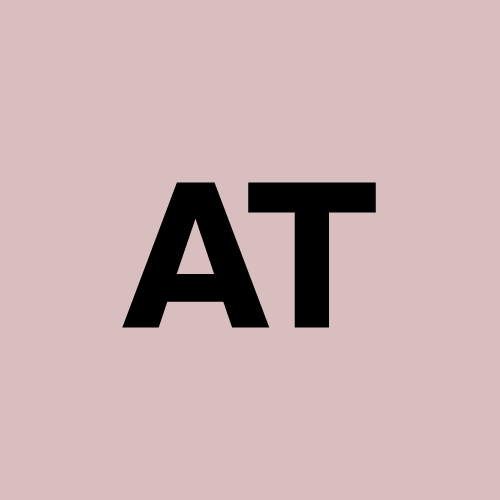
Alyaa Talaat
Alyaa Talaat
As a continuous learner, I’m always exploring new technologies and best practices to enhance my skills in software development. I enjoy tackling complex coding challenges, whether it's optimizing performance, implementing new features, or debugging intricate issues.