Basics of Python for DevOps Engineers

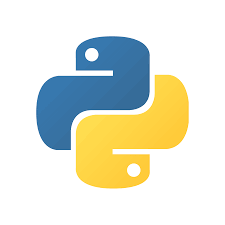
Getting Started with Python: Installation and Data Types
Step 1: Installing Python
Windows
Download Python:
- Visit the official Python website and download the latest version for Windows.
Install Python:
Run the installer and ensure you check the box that says “Add Python to PATH” to easily access Python from the command line.
Follow the installation instructions.
Check Python Version:
Open the Command Prompt (cmd).
Type the following command and press Enter:
python --version
Mac OS
Download Python:
- Go to the official Python website and download the latest version for macOS.
Install Python:
- Open the downloaded file and follow the instructions to install Python.
Check Python Version:
Open the Terminal.
Type the following command and press Enter:
python --version
Linux
Install Python:
Most Linux distributions come with Python pre-installed. You can check if it’s installed by opening the terminal :
python --version
If Python is not installed, you can install it using the package manager. For example :
sudo apt update sudo apt install python3.12
Check Python Version:
In the terminal, type:
python --version
Data Types in Python:
String (
str
):A string is a sequence of characters enclosed in quotes (single, double, or triple).
Example:
name = "Imran" greeting = 'Hello, world!'
Strings can include letters, numbers, and symbols.
Integer (
int
):Integers are whole numbers, either positive or negative, without a decimal point.
Example:
age = 23 year = -2024
Operations like addition, subtraction, and multiplication can be performed with integers.
Float (
float
):Floats represent real numbers with a decimal point.
Example:
temperature = 36.5 pi = 3.14159
Floats are useful for representing measurements, distances, or any value that requires precision.
List (
list
):A list is an ordered collection of elements, which can be of different data types, enclosed in square brackets
[]
. Lists are mutable, meaning they can be modified after creation.Example:
fruits = ["apple", "banana", "cherry"] numbers = [1, 2, 3, 4, 5]
Lists are great for storing multiple related items, like a series of tasks or results.
Tuple (
tuple
):A tuple is similar to a list but is immutable, meaning it cannot be changed once created. It’s enclosed in parentheses
()
.Example:
coordinates = (10, 20) person = ("Alice", 30)
Use tuples for fixed collections where data shouldn’t be modified.
Dictionary (
dict
):A dictionary stores data in key-value pairs, enclosed in curly braces
{}
. Each key must be unique, and values can be of any type.Example:
student = {"name": "Alice", "age": 23, "grade": "A"}
Dictionaries are great for representing structured data, like configurations or records.
Boolean (
bool
):Booleans represent two values:
True
orFalse
. They're often used in conditional statements or logic.Example:
is_active = True has_passed = False
Booleans are crucial for decision-making in code, like checking conditions.
Each of these data types has its own purpose and is useful in different scenarios in Python programming.
HappyLearning:)
Subscribe to my newsletter
Read articles from Imran Shaikh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
