Understanding and Leveraging Go's sync.Pool for Efficient Object Reuse
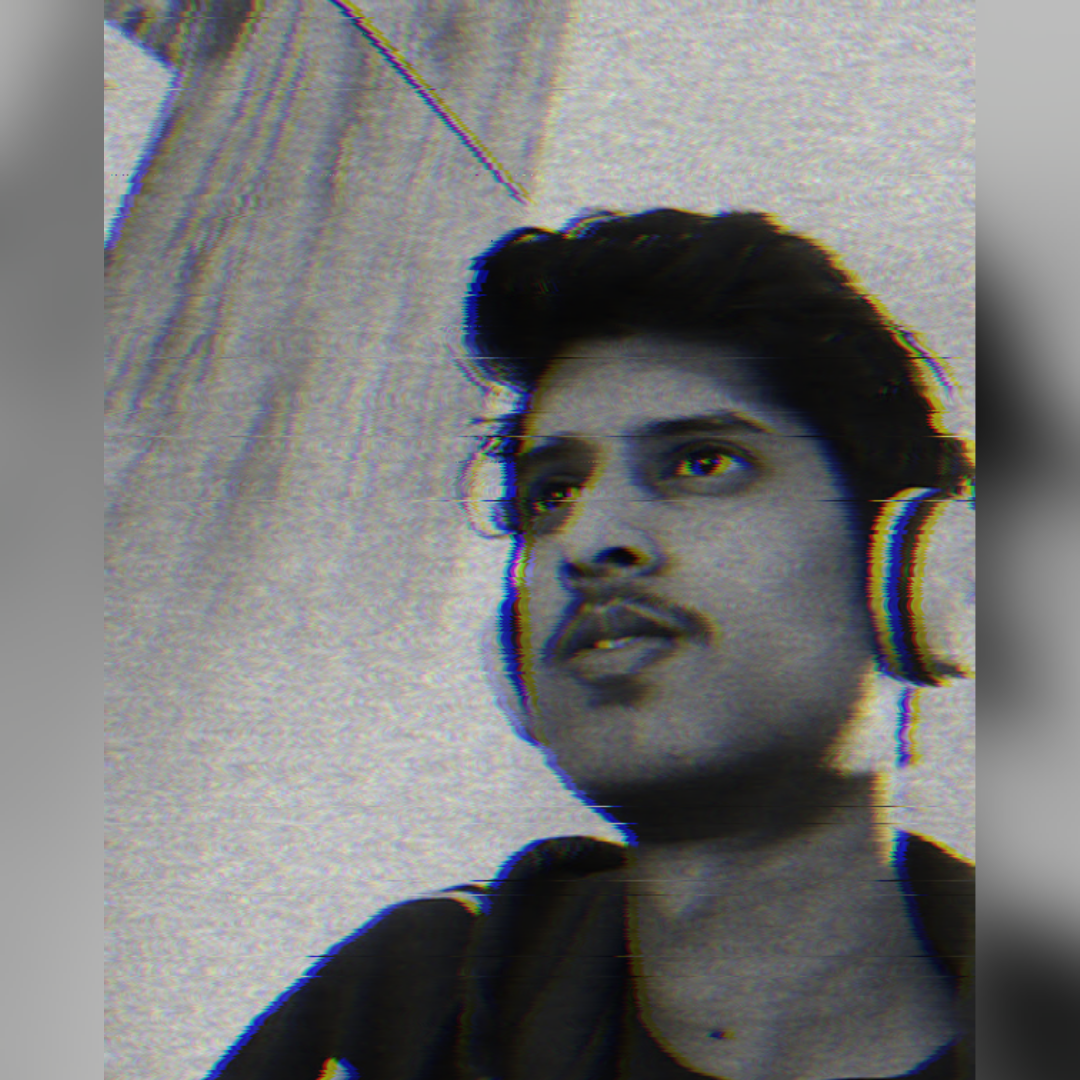
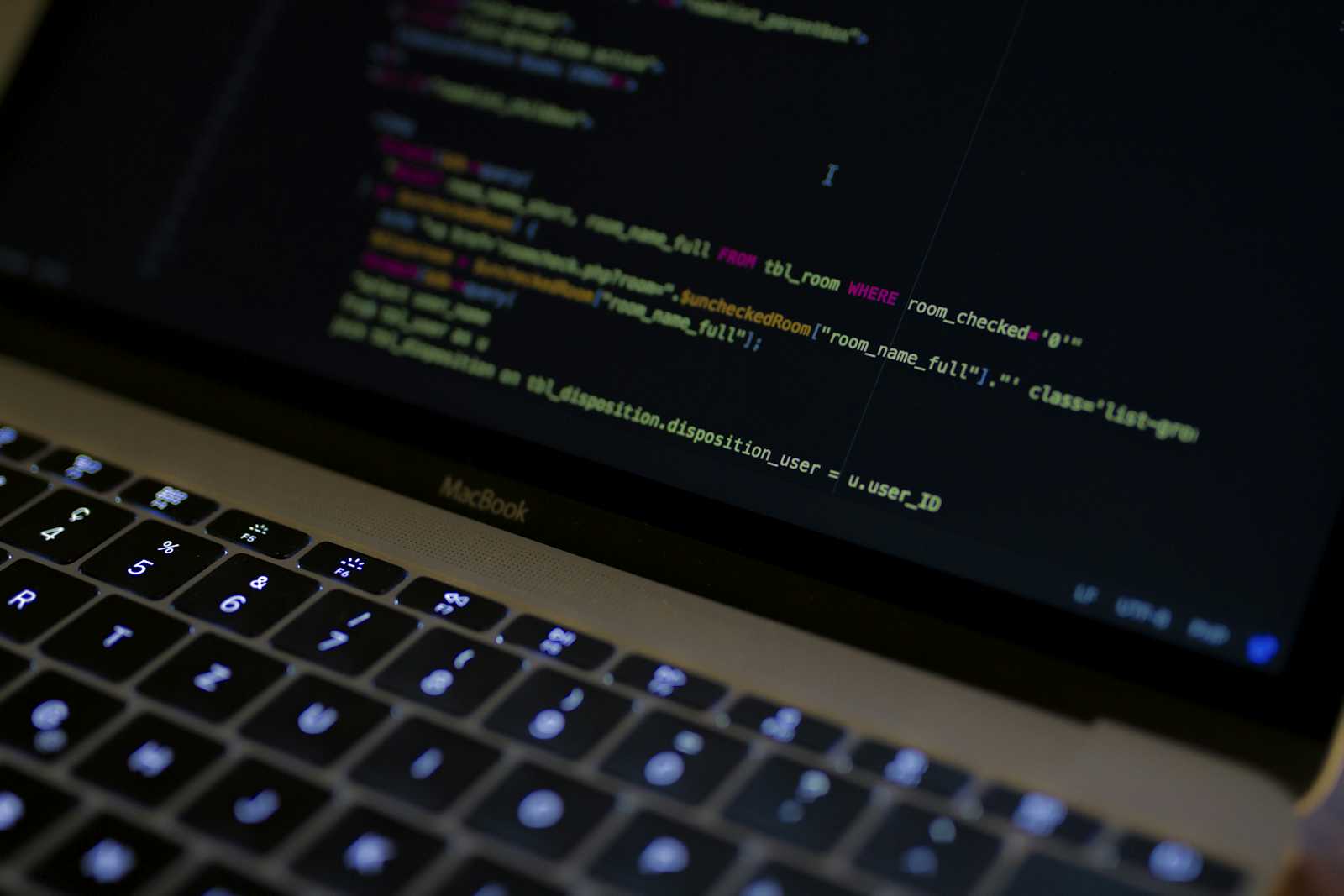
Go/Golang, is a statically typed, compiled language designed for simplicity and efficiency. One of its powerful concurrency features is the sync package, which includes various synchronization primitives. Among these is sync.Pool, a powerful tool for managing temporary objects in a concurrent environment.
What is sync.Pool?
sync.Pool
is a concurrent data structure designed for caching temporary objects to reduce memory allocation overhead. It allows multiple goroutines to share a pool of objects, providing a way to reuse objects instead of allocating and deallocating them repeatedly.
What is the need of sync.Pool?
Garbage collection (GC) is a crucial aspect of memory management in Go, automatically reclaiming memory that is no longer in use. However, frequent allocations and deallocations can place significant pressure on the garbage collector, leading to performance overhead. Here’s where sync.Pool
plays an essential role.
When objects are frequently allocated and deallocated (in cases where a same function is started as multiple go-routines that allocates/deallocates the same objects), they can cause spikes in memory usage and lead to increased garbage collection activity. Each time the garbage collector runs, it needs to identify which objects are no longer reachable and free them, which can be costly in terms of CPU cycles and pause times.
sync.Pool
mitigates these issues by allowing the reuse of objects. Instead of allocating new memory each time an object is needed, you can retrieve an object from the pool. This reduces the total number of allocations, helping to keep the memory footprint lower and minimizing GC activity.
Working with sync.Pool
sync.Pool
is a concurrent-safe object pool that allows multiple goroutines to share a pool of objects. It operates on a simple principle: objects are fetched from the pool when needed and returned to the pool when no longer in use.
Object Reuse: Allows for the reuse of objects, which can significantly improve performance in applications that require frequent allocations and deallocations.
Temporary Nature: Intended for temporary objects - in a
sync.Pool
can be garbage collected if they are not in use.The New() function is used to initialize the object. Note that this is an optional function and users need to do a
nil
check before type-casting it into respective type afterGet()
The objects can be retrieved using Get and can be put back into the pool using the Put method.
Example
var pool sync.Pool
type MyCustomStruct struct {
Buffer []byte
}
func init() {
pool.New = func() interface{} {
return &MyCustomStruct{
Buffer: make([]byte, 0, 1024),
}
}
}
func process() {
for i := 0; i < 10000; i++ {
obj := pool.Get().(*MyCustomStruct) // Get objects from the pool
// Note: if New() is not used when initializing, a nil check is required before type-casting
//Perform some operations on the object
// Reset the object before putting it back into the pool (A good practice)
pool.Put(obj) // Put the object back to the pool
}
}
In summary, while Go's garbage collector is a powerful, using a sync.Pool
can be beneficial in scenarios where you need to optimize performance, reduce GC pressure, or manage resources effectively.
Subscribe to my newsletter
Read articles from Nihal Wasim directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
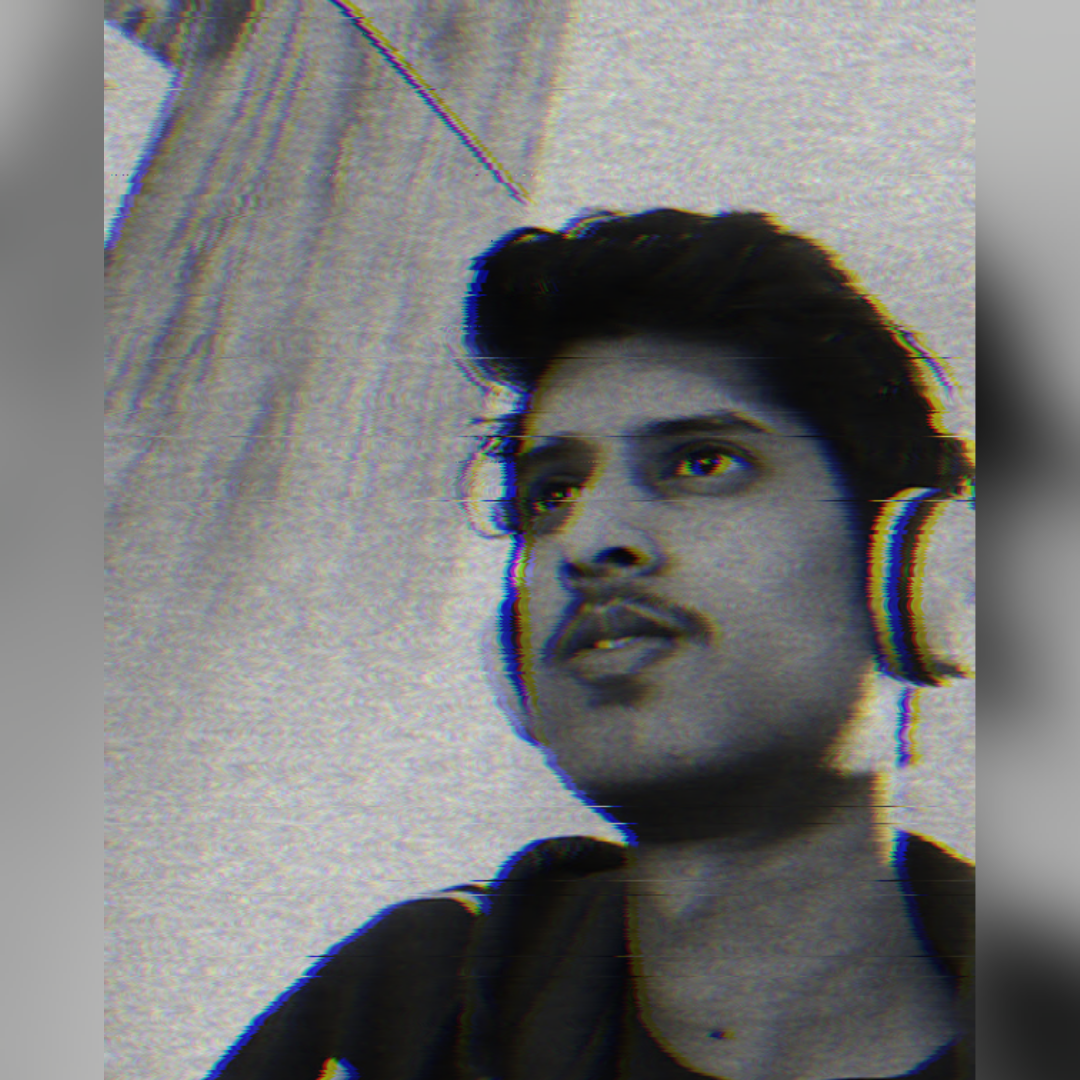