Advanced Linux Shell Scripting for DevOps Engineers

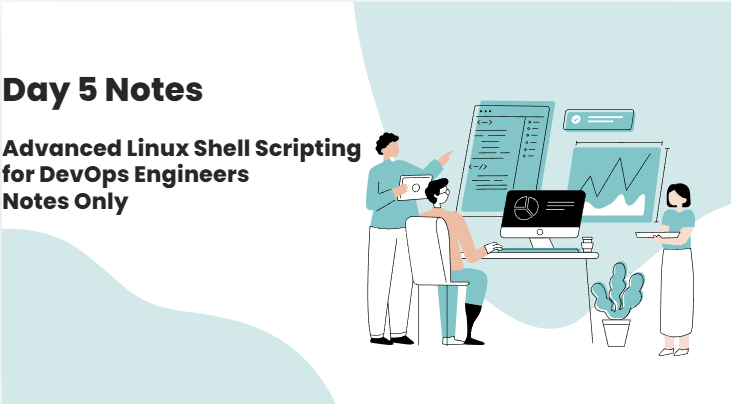
Shell scripting is an essential skill for system administrators, developers, and anyone working with Linux/Unix-based systems. It allows you to automate repetitive tasks, manipulate files, and enhance productivity by writing small programs. Let’s dive into Shell scripting, from the basics to advanced topics.
1. What is Shell?
The shell is a command-line interpreter that provides a way for users to interact with the operating system. Some popular shells are:
Bash (Bourne Again Shell) – Default on most Linux systems.
Zsh – A more feature-rich shell.
Ksh (KornShell) – Useful in many Unix systems.
In shell scripting, we write commands in a file (script) which the shell can interpret.
2. Getting Started with a Simple Shell Script
Creating a Script: Create a text file and include commands you want to execute.
#!/bin/bash echo "Hello, World!"
Execution:
3. Basic Shell Commands in Scripts
echo: Prints text to the terminal.
echo "This is a message"
Variables: Store data for reuse.
NAME="John" echo "My name is $NAME"
Command Substitution: Capture the output of commands.
CURRENT_DATE=$(date) echo "Today is $CURRENT_DATE"
Comments: Use
#
for comments.# This is a comment
4. Conditional Statements (if-else)
If-Else Structure
Syntax:
if [ condition ]; then # Commands elif [ another_condition ]; then # Commands else # Commands fi
Example:
AGE=25 if [ $AGE -ge 18 ]; then echo "Adult" else echo "Minor" fi
Comparison Operators:
-eq
(equal),-ne
(not equal)-lt
(less than),-gt
(greater than)-le
(less than or equal to),-ge
(greater than or equal to)
String Comparison:
=
(equal),!=
(not equal),-z
(empty string)
5. Loops
For Loop:
Syntax:
for item in list; do # Commands done
Example:
for i in {1..5}; do echo "Iteration $i" done
While Loop:
Syntax:
while [ condition ]; do # Commands done
Example:
COUNTER=1 while [ $COUNTER -le 5 ]; do echo "Count: $COUNTER" ((COUNTER++)) done
Until Loop:
Syntax:
until [ condition ]; do # Commands done
6. Functions in Shell Scripts
Functions allow us to write reusable blocks of code.
Syntax:
codefunction_name() { # Commands }
Example:
codegreet() { echo "Hello, $1!" } greet "Alice"
In this example,
$1
represents the first argument passed to the function.
7. Handling Input and Output
Reading Input from the User:
read -p "Enter your name: " NAME
echo "Welcome, $NAME"
Redirecting Output:
>
: Redirects output to a file (overwrites).>>
: Appends output to a file.echo "This is a log entry" >> logfile.txt
Redirecting Errors:
2>
: Redirects standard error.ls nonexistentfile 2> error.log
Piping: Passes output of one command as input to another.
ls | grep "filename"
8. Advanced Shell Scripting
Arrays in Shell:
Defining an Array:
MY_ARRAY=("item1" "item2" "item3")
Accessing Elements:
echo ${MY_ARRAY[1]} # Output: item2
Looping Through Arrays:
for ITEM in "${MY_ARRAY[@]}"; do echo $ITEM done
Case Statements:
Syntax:
case $variable in pattern1) # Commands ;; pattern2) # Commands ;; *) # Default commands ;; esac
Example:
read -p "Enter a number between 1 and 3: " NUMBER case $NUMBER in 1) echo "You entered one" ;; 2) echo "You entered two" ;; 3) echo "You entered three" ;; *) echo "Invalid number" ;; esac
9. File Handling
Creating and Writing Files:
echo "This is a test" > testfile.txt
Reading Files:
while read line; do echo $line done < filename.txt
10. Trap Command
Trap allows you to catch signals and perform cleanups or special commands when the script is interrupted.
Syntax:
trap "commands" signal
Example:
trap "echo 'Caught CTRL+C'; exit" SIGINT
11. Working with Cron Jobs
Shell scripts can be automated using cron.
Cron Job Format:
* * * * * /path/to/script.sh
- The format represents minute hour day-of-month month day-of-week.
Example (Every day at 5 PM):
0 17 * * * /path/to/backup.sh
12. Debugging Shell Scripts
Using
set
command:set -x
: Enables debugging (prints commands before execution).set +x
: Disables debugging.
Example:
set -x echo "This will be debugged" set +x echo "This will not"
13. Shell Scripting Best Practices
Use Comments: Explain your code.
Error Handling: Use
||
,&&
, andif
blocks to manage errors.Always Check Exit Status:
$?
gives the status of the last command executed (0 means success).cp file.txt /backup || echo "Copy failed!"
Use Functions: Break down tasks into functions for better code structure.
Avoid Hardcoding: Use variables and arguments.
14. Conclusion
Mastering shell scripting requires practice, but with this foundation, you can write scripts that automate your daily tasks, handle complex workflows, and improve productivity. Start with simple scripts and progressively explore more advanced topics like file handling, arrays, and automation with cron.
Subscribe to my newsletter
Read articles from Birva Gangurde directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
